The Balance POJ 2142 扩展欧几里得
Description
You are asked to help her by calculating how many weights are required.
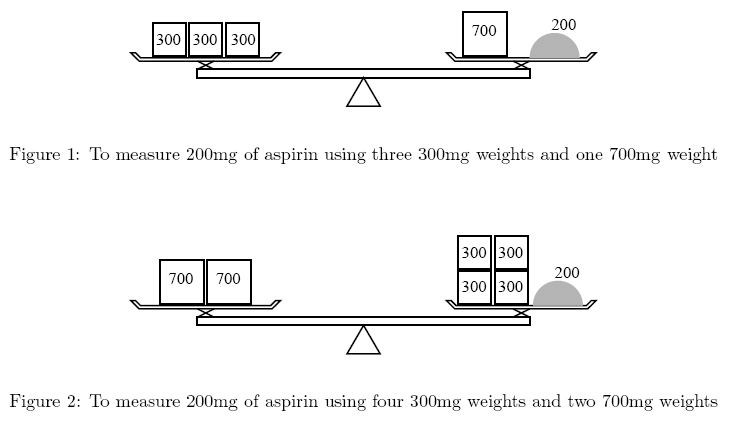
Input
The end of the input is indicated by a line containing three zeros separated by a space. It is not a dataset.
Output
- You can measure dmg using x many amg weights and y many bmg weights.
- The total number of weights (x + y) is the smallest among those pairs of nonnegative integers satisfying the previous condition.
- The total mass of weights (ax + by) is the smallest among those pairs of nonnegative integers satisfying the previous two conditions.
No extra characters (e.g. extra spaces) should appear in the output.
Sample Input
700 300 200
500 200 300
500 200 500
275 110 330
275 110 385
648 375 4002
3 1 10000
0 0 0
Sample Output
1 3
1 1
1 0
0 3
1 1
49 74
3333 1
#include<cstdio>
#include<set>
#include<map>
#include<cstring>
#include<algorithm>
#include<queue>
#include<iostream>
#include<string>
#include<cmath>
#include<vector>
using namespace std;
typedef long long LL;
/*
a*x = b*y + d
a*x + b*y = d
|x|+|y| 的最小值
|x|+|y|==|x0+b/d*t|+|y0-a/d*t|,我们规定 a>b(如果 a<b,我们便交换 a b),从这个式子中,
我们可以轻易的发现:|x0+b/d*t|是单调递增的,|y0-a/d*t|是单调递减的,而由于我们规定了 a>b,
那么减的斜率边要大于增的斜率,于是整个函数减少的要比增加的快,但是由于绝对值的符号的作用,
最终函数还是递增的。
也就是说,函数是凹的,先减小,再增大。那么什么时候最小呢?很显然是 y0-a/d*t==0 的时候,
于是我们的最小值|x|+|y|也一定是在 t=y0*d/a附近了,只要在附近枚举几个值就能找到最优解了。
*/
typedef long long LL;
#define INF 0x3f3f3f3f
LL ex_gcd(LL a,LL b,LL &x,LL &y)
{
if(b==)
{
x = ;
y = ;
return a;
}
LL ans = ex_gcd(b,a%b,x,y);
LL tmp = x;
x = y;
y = tmp - a/b*x;
return ans;
}
void cal(LL a,LL b,LL c)//求ax+by==c 的一组|x|+|y| 最小的解
{
bool f = false;
if(a<b)
{
f = true;
swap(a,b);
}
LL x=,y=;
LL gcd = ex_gcd(a,b,x,y);
x *= c/gcd;
y *= c/gcd;
LL t = y*gcd/a,ans=INF;
LL kx,ky;
for(LL i=t-;i<=t+;i++)
{
LL t1 = x + (b/gcd)*i;
LL t2 = y - (a/gcd)*i;
if( abs((long)t1)+abs((long)t2)<ans)
{
ans = abs((long)t1)+abs((long)t2);
kx = (t1>)?t1:-t1;
ky = (t2>)?t2:-t2;
}
}
if(!f)
printf("%lld %lld\n",kx,ky);
else
printf("%lld %lld\n",ky,kx);
}
int main()
{
LL a,b,c;
while(scanf("%lld%lld%lld",&a,&b,&c),a+b+c)
{
cal(a,b,c);
}
}
The Balance POJ 2142 扩展欧几里得的更多相关文章
- poj 2142 扩展欧几里得解ax+by=c
原题实际上就是求方程a*x+b*y=d的一个特解,要求这个特解满足|x|+|y|最小 套模式+一点YY就行了 总结一下这类问题的解法: 对于方程ax+by=c 设tm=gcd(a,b) 先用扩展欧几里 ...
- poj 2891 扩展欧几里得迭代解同余方程组
Reference: http://www.cnblogs.com/ka200812/archive/2011/09/02/2164404.html 之前说过中国剩余定理传统解法的条件是m[i]两两互 ...
- poj 1061 扩展欧几里得解同余方程(求最小非负整数解)
题目可以转化成求关于t的同余方程的最小非负数解: x+m*t≡y+n*t (mod L) 该方程又可以转化成: k*L+(n-m)*t=x-y 利用扩展欧几里得可以解决这个问题: eg:对于方程ax+ ...
- poj 1061(扩展欧几里得定理求不定方程)
两只青蛙在网上相识了,它们聊得很开心,于是觉得很有必要见一面.它们很高兴地发现它们住在同一条纬度线上,于是它们约定各自朝西跳,直到碰面为止.可是它们出发之前忘记了一件很重要的事情,既没有问清楚对方的特 ...
- poj 2115 扩展欧几里得
题目链接:http://poj.org/problem?id=2115 题意: 给出一段循环程序,循环体变量初始值为 a,结束不等于 b ,步长为 c,看要循环多少次,其中运算限制在 k位:死循环输出 ...
- poj 2142 拓展欧几里得
#include <cstdio> #include <algorithm> #include <cstring> #include <iostream> ...
- POJ 1061 扩展欧几里得
#include<stdio.h> #include<string.h> typedef long long ll; void gcd(ll a,ll b,ll& d, ...
- 扩展欧几里得(E - The Balance POJ - 2142 )
题目链接:https://cn.vjudge.net/contest/276376#problem/E 题目大意:给你n,m,k,n,m代表当前由于无限个质量为n,m的砝码.然后当前有一个秤,你可以通 ...
- POJ - 2142 The Balance(扩展欧几里得求解不定方程)
d.用2种砝码,质量分别为a和b,称出质量为d的物品.求所用的砝码总数量最小(x+y最小),并且总质量最小(ax+by最小). s.扩展欧几里得求解不定方程. 设ax+by=d. 题意说不定方程一定有 ...
随机推荐
- 【懒人专用系列】Xind2TestCase的初步探坑
公司最近说要弄Xind2TestCase,让我们组先试用一下 解释:https://testerhome.com/topics/17554 github项目:https://github.com/zh ...
- webservice 权限控制
webservice 如何限制访问,权限控制?1.服务器端总是要input消息必须携带用户名.密码信息 如果不用cxf框架,SOAP消息(xml片段)的生成.解析都是有程序员负责 2.拦截器 为了让程 ...
- java dom4j xml生成,解析
1. 用Java代码生成xml文档 package com.test.dom; import java.io.FileOutputStream; import java.io.IOException; ...
- docker血一样的教训,生成容器的时候一定要设置数据卷,把数据文件目录,配置文件目录,日志文件目录都要映射到宿主机上保存啊!!!
打个比方,比如mysql,如果你想重新设置一下mysql的配置,不小心配错里,启动容器失败,已启动就停止了. 根本进不去mysql的容器里.如果之前run容器的时候,没有把数据文件,日志文件,配置文件 ...
- 全面学习ORACLE Scheduler特性(8)Application抛出的Events
4.2 Application抛出的Events 首先要说明,这里所说的Application是个代词,即可以表示ORACLE数据库之外的应用程序,也可以是ORACLE数据库中的PROCEDURE等对 ...
- 391 Perfect Rectangle 完美矩形
有 N 个与坐标轴对齐的矩形, 其中 N > 0, 判断它们是否能精确地覆盖一个矩形区域.每个矩形用左下角的点和右上角的点的坐标来表示.例如, 一个单位正方形可以表示为 [1,1,2,2]. ( ...
- 创建http对象
package test; import java.net.HttpURLConnection;import java.net.URL; import javax.servlet.http.HttpS ...
- [Windows Server 2003] 手工创建安全网站
★ 欢迎来到[护卫神·V课堂],网站地址:http://v.huweishen.com★ 护卫神·V课堂 是护卫神旗下专业提供服务器教学视频的网站,每周更新视频.★ 本节我们将带领大家:手工创建安全站 ...
- python学习笔记(4)——list[ ]
发现个问题,python与C.JAVA等语言相比学习障碍最大差别居然在于版本更迭!这是python官方造的虐啊... 有时针对某问题去google答案,或者自己去博客找共性解答,会出现相互矛盾或者与你 ...
- 定时器tasktimer
1.web.xml中配置 <servlet> <servlet-name>TaskTimer</servlet-name> <servlet-class> ...