💈 A Cross-Thread Call Helper Class
Conmajia © 2012, 2018
Published on August 5th, 2012
Updated on February 2nd, 2019
Introduction
While working on background threads, you may encounter updating values of frontend GUI controls. Then you probably will face one particular problem: invalid operation between threads. As shown in figure 1, this issue throws an InvalidOperationException
. It occurs every time when accesses properties/methods from other threads but the one which owns them.
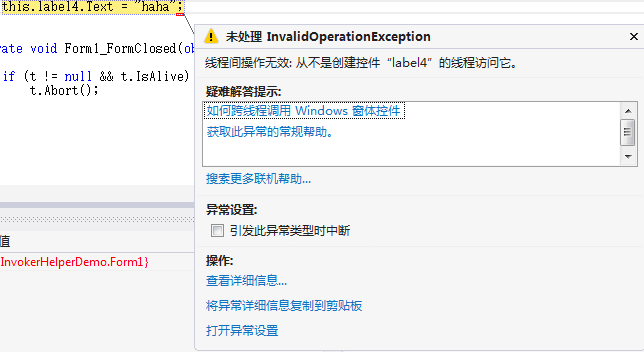
In .NET Framework, version 2.0 as I refer, every Control
class contains an InvokeRequired
property and an Invoke
method to accomplish cross-thread operations. Some typical call code is listed below.
public void DoWork() {
if (control.InvokeRequired) {
control.Invoke(DoWork);
}
else {
// do work
}
}
My Approach
I wrote a helper class InvokeHelper
which granted me the power to access assets between different threads. The class has 3 methods:
Invoke()
- to call methods of a control.
InvokeHelper.Invoke(<control>, "<method>"[, <param1>[,<param2>,...]]);
Get()
- to get properties of a control.
InvokeHelper.Get(<control>, "<property>");
Set()
- to set properties of a control.
InvokeHelper.Set(<control>, "<property>", <value>);
Demonstration
In the demo, I used a forever looping background thread (t
) to show how the InvokeHelper
helps threads to interact. Thread t
updates the frontend thread (the GUI) every 500 milliseconds.
Thread t;
private void button1_Click(object sender, EventArgs e) {
if(t == null) {
t = new Thread(multithread);
t.Start();
label4.Text = string.Format("Thread state:\n{0}", t.ThreadState.ToString());
}
}
public void DoWork(string msg) {
this.label3.Text = string.Format("Invoke method: {0}", msg);
}
int count = 0;
void multithread() {
while(true) {
InvokeHelper.Set(this.label1, "Text", string.Format("Set value: {0}", count));
InvokeHelper.Set(this.label1, "Tag", count);
string value = InvokeHelper.Get(this.label1, "Tag")
.ToString();
InvokeHelper.Set(this.label2, "Text", string.Format("Get value: {0}", value));
InvokeHelper.Invoke(this, "DoWork", value);
Thread.Sleep(500);
count++;
}
}
Results shown in animated figure 2. Obviously, the frontend is not blocked despite t
never stops.
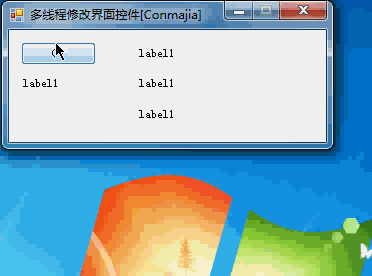
InvokeHelper
Demonstration (animation)Other Approaches
There is a built-in option in the VisualStudio IDE that disables the cross-thread access check: CheckForIllegalCrossThreadCalls
. I found it slightly slower than my helper class. Figure 3 shows the test result.
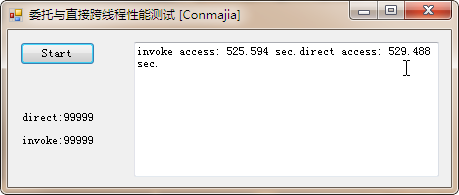
CheckForIllegalCrossThreadCalls
The whole procedure of the test is recorded in figure 4. (The video lasts for 8'51")
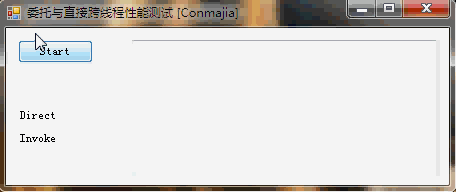
CheckForIllegalCrossThreadCalls
(8'51")Appendix
Source code of the InvokeHelper
is listed below. Zipped .NET project files: Download
public class InvokeHelper {
private delegate object MethodInvoker(Control control, string methodName, params object[] args);
private delegate object PropertyGetInvoker(Control control, object noncontrol, string propertyName);
private delegate void PropertySetInvoker(Control control, object noncontrol, string propertyName, object value);
private static PropertyInfo GetPropertyInfo(Control control, object noncontrol, string propertyName) {
if (control != null && !string.IsNullOrEmpty(propertyName)) {
PropertyInfo pi = null;
Type t = null;
if (noncontrol != null) t = noncontrol.GetType();
else t = control.GetType();
pi = t.GetProperty(propertyName);
if (pi == null) throw new InvalidOperationException(string.Format("Can't find property {0} in {1}.", propertyName, t.ToString()));
return pi;
} else throw new ArgumentNullException("Invalid argument.");
}
public static object Invoke(Control control, string methodName, params object[] args) {
if (control != null && !string.IsNullOrEmpty(methodName))
if (control.InvokeRequired) return control.Invoke(new MethodInvoker(Invoke), control, methodName, args);
else {
MethodInfo mi = null;
if (args != null && args.Length > 0) {
Type[] types = new Type[args.Length];
for (int i = 0; i
References
- Sergiu Josan, Making Controls Thread-safely, May 2009
- vicoB, Extension of safeInvoke, July 2010
The End. \(\Box\)
💈 A Cross-Thread Call Helper Class的更多相关文章
- update the UI property cross thread
this.Invoke((MethodInvoker)delegate { txtResult.Text = sbd.ToString(); // runs on UI thread });
- Flink - Checkpoint
Flink在流上最大的特点,就是引入全局snapshot, CheckpointCoordinator 做snapshot的核心组件为, CheckpointCoordinator /** * T ...
- [转]How to display the data read in DataReceived event handler of serialport
本文转自:https://stackoverflow.com/questions/11590945/how-to-display-the-data-read-in-datareceived-event ...
- c#子线程线程中操作窗体更新的报错
用 在执行上传时,由于操作较长窗体界面卡住,于是用task解决 Task t1 = new Task(manage.UploadData); t1.Start(); 结果不卡了,程序也传完了,运行到更 ...
- 进程创建过程详解 CreateProcess
转载请您注明出处:http://www.cnblogs.com/lsh123/p/7405796.html 0x01 CreateProcessW CreateProcess的使用有ANSI版本的Cr ...
- C#委托+回调详解
今天写不完,明天会接着写的,,,, 学习C#有一段时间了,不过C#的委托+回调才这两天才会用,以前只是知道怎么用.前面的一篇文章,函数指针,其实是为这个做铺垫的,说白了委托就相当于C语言中的函数指针, ...
- windows中的进程和线程
今天咱们就聊聊windows中的进程和线程 2016-09-30 在讨论windows下的进程和线程时,我们先回顾下通用操作系统的进程和线程.之所以称之为通用是因为一贯的本科或者其他教材都是这么说的: ...
- [MethodImpl(MethodImplOptions.Synchronized)]、lock(this)与lock(typeof(...))
对于稍微有点经验的.NET开发人员来说,倘若被问及如何保持线程同步,我想很多人都能说好好几种.在众多的线程同步的可选方式中,加锁无疑是最为常用的.如果仅仅是基于方法级别的线程同步,使用System.R ...
- Xamarin.Forms客户端第一版
Xamarin.Forms客户端第一版 作为TerminalMACS的一个子进程模块,目前完成第一版:读取展示手机基本信息.联系人信息.应用程序本地化. 功能简介 详细功能说明 关于TerminalM ...
- [源码解析] 深度学习流水线并行 PipeDream(5)--- 通信模块
[源码解析] 深度学习流水线并行 PipeDream(5)--- 通信模块 目录 [源码解析] 深度学习流水线并行 PipeDream(5)--- 通信模块 0x00 摘要 0x01 前言 0x02 ...
随机推荐
- MySQL Command Line Client显示中文的部分为空
一连接数据库的时候就设置如下: 先设置 set names gbk,然后再插入就显示中文
- arcgis地图服务之 identify 服务
arcgis地图服务之 identify 服务 在近期的一次开发过程中,利用IdentityTask工具查询图层的时候,请求的参数中ImageDisplay的参数出现了错误,导致查询直接不能执行,百度 ...
- 从arduino到32单片机的转型
#include "stm32f10x.h" #include "led.h" #include "delay.h" int main(vo ...
- Linux指令--which,whereis,locate,find
原文出处:http://www.cnblogs.com/peida/archive/2012/12/05/2803591.html.感谢作者无私分享 which 我们经常在linux要查找某个文件,但 ...
- alibaba架包FastJson使用例子
alibaba的架包FastJson可以对json字符串进行快捷的类型转换.下面是一些各种类型转换的使用例子. 一.下载FastJson的架包,并导入项目中,如下: Maven项目pom.xml配置如 ...
- 使用sed替换一行内多个括号内的值
1. 括号在同一行 # cat test2good morning (good afternoon) (good evening) (goodgood) (good morning) # cat se ...
- OSSEC初探
OSSEC初探 概念: OSSEC是一款开源的基于主机的入侵检测系统(HIDS),它可以执行日志分析.完整性检验.windows注册表监控.隐匿性检测和实时告警.它可以运行在各种不同的操作系统上,包括 ...
- Electron应用使用electron-builder配合electron-updater实现自动更新(windows + mac)
发客户端一定要做的就是自动更新模块,否则每次版本升级都是一个头疼的事.下面是Electron应用使用electron-builder配合electron-updater实现自动更新的解决方案. 1.安 ...
- 淘宝地址爬取及UI展示
淘宝地址爬取及UI展示 淘宝国家省市区街道获取 参考 foxiswho 的 taobao-area-php 部分代码,改由c#重构. 引用如下: Autofac MediatR Swagger Han ...
- 所不为人知的Python装饰器
装饰器可以说是Python中非常重要的特性之一.有些人要么从没使用过装饰器,要么就是对装饰器的使用一知半解.也有些人觉得装饰器很简单:"装饰器不就是那些把函数作为参数并输出一个函数的函数&q ...