NSFetchedResultController与UITableView
1 #import "AppDelegate.h"
#import "Book.h"
@interface AppDelegate ()
@end
@implementation AppDelegate
-(void)addBookWithTitle:(NSString *)title andAuthor:(NSString *)author andPrice:(NSNumber *)price
{
Book *book = [NSEntityDescription insertNewObjectForEntityForName:NSStringFromClass([Book class]) inManagedObjectContext:self.managedObjectContext];
book.title = title;
book.author = author;
book.price = price;
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
//如果不想每次执行时测试数据都重新插入一遍,可以使用偏好设置,如果已经存在了,就不再进行插入了。
NSUserDefaults *userDefaults = [NSUserDefaults standardUserDefaults];
BOOL isInserted = [userDefaults boolForKey:@"inInserted"];
if (!isInserted)
{
//插入数据
[self addBookWithTitle:@"*文*" andAuthor:@"徐" andPrice:@10000.1];
[self addBookWithTitle:@"西游记" andAuthor:@"吴承恩" andPrice:@20.5];
[self addBookWithTitle:@"水浒传" andAuthor:@"施耐庵" andPrice:@5.1];
[self addBookWithTitle:@"三国演义" andAuthor:@"罗贯中" andPrice:@10.2];
[self addBookWithTitle:@"史记" andAuthor:@"司马迁" andPrice:@45.3];
[self addBookWithTitle:@"资治通鉴" andAuthor:@"司马光" andPrice:@56.5];
[self saveContext];
//保存偏好设置
[userDefaults setBool:YES forKey:@"inInserted"];
//自动步更新
[userDefaults synchronize];
}
return YES;
}
#import "BookTableViewController.h"
#import "Book.h"
#import "AppDelegate.h"
@interface BookTableViewController ()<NSFetchedResultsControllerDelegate>
@property(strong,nonatomic)NSFetchedResultsController *fetchedRC;
@property(strong,nonatomic)NSManagedObjectContext *managedObjectContext;
@end @implementation BookTableViewController - (void)viewDidLoad {
[super viewDidLoad];
//获取应用代理
AppDelegate *delegate = [[UIApplication sharedApplication]delegate];
//本次案例需要对CoreData的内容进行修改,涉及到managedObjectContext,但是magagedObjetContext属于Appdelegate的属性,此处使用协议获取创建新的managedObjectContext;
self.managedObjectContext = delegate.managedObjectContext;
//使用fetchedRC获取数据
NSError *error = nil;
[self.fetchedRC performFetch:&error];
if (error) {
NSLog(@"NSFetchedResultsController获取数据失败");
}
}
-(NSFetchedResultsController *)fetchedRC
{
//判断fetchRC是否存在,如果不存在则创建新的,否则直接返回
if (!_fetchedRC) {
//使用NSFetchRequest进行获取数据
NSFetchRequest *request = [NSFetchRequest fetchRequestWithEntityName:NSStringFromClass([Book class])];
request.fetchBatchSize = ;
//设置以某个字段进行排序,此案例以:price价格大小进行排序
NSSortDescriptor *priceSort = [NSSortDescriptor sortDescriptorWithKey:@"price" ascending:YES];
//对获取的数据进行排序
[request setSortDescriptors:@[priceSort]];
//创建新的fetchedRC
_fetchedRC = [[NSFetchedResultsController alloc]initWithFetchRequest:request managedObjectContext:self.managedObjectContext sectionNameKeyPath:nil cacheName:nil];
_fetchedRC.delegate = self;
}
return _fetchedRC;
} #pragma mark - Table view data source
//设置tableView的分组数
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
//分组的数据取决于创建sectionNameKeyPath的设置;
return self.fetchedRC.sections.count;
}
//设置tableView每组有多少行
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
id sectionsInfo = [self.fetchedRC.sections objectAtIndex:section];
return [sectionsInfo numberOfObjects];
}
//自定义方法,设置单元格的显示内容
-(void)configCell:(UITableViewCell *)cell andIndexPath:(NSIndexPath *)indexPath
{
//获取选中的对象
Book *book = [self.fetchedRC objectAtIndexPath:indexPath];
cell.textLabel.text = [NSString stringWithFormat:@"%@ %@",book.title,book.author];
cell.detailTextLabel.text = [NSString stringWithFormat:@"%@",book.price];
}
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//1.根据reuseindentifier先到对象池中去找重用的单元格
static NSString *reuseIndetifier = @"bookCell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:reuseIndetifier];
//2.如果没有找到需要自己创建单元格对象
if (cell == nil) {
cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:reuseIndetifier];
}
//3.设置单元格对象的内容
[self configCell:cell andIndexPath:indexPath];
return cell;
}
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
// Return NO if you do not want the specified item to be editable.
return YES;
}
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete)
{
Book *book = [self.fetchedRC objectAtIndexPath:indexPath];
//1.先删除CoreData中的相应数据
[self.managedObjectContext deleteObject:book];
//插入新的记录
AppDelegate *delegate = [[UIApplication sharedApplication]delegate];
[delegate addBookWithTitle:@"唐诗三百首" andAuthor:@"李白等" andPrice:@12.3];
book.price = @([book.price doubleValue]+);
NSError *error = nil;
[self.managedObjectContext save:&error];
if(error) {
NSLog(@"失败");
}
} else if (editingStyle == UITableViewCellEditingStyleInsert)
{
}
}
#pragma mark - NSFetchedResultsController代理方法
-(void)controllerWillChangeContent:(NSFetchedResultsController *)controller
{
[self.tableView beginUpdates];
}
-(void)controllerDidChangeContent:(NSFetchedResultsController *)controller
{
[self.tableView endUpdates];
}
/**
* 以下方法共进行了两项操作:
1.判断操作的类型
2.对修改的数据、或新插入的数据位置进行局部刷新
*/
-(void)controller:(NSFetchedResultsController *)controller didChangeObject:(id)anObject atIndexPath:(NSIndexPath *)indexPath forChangeType:(NSFetchedResultsChangeType)type newIndexPath:(NSIndexPath *)newIndexPath
{
if (type == NSFetchedResultsChangeDelete)//删除操作
{
[self.tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationAutomatic];
}
else if (type == NSFetchedResultsChangeInsert)//插入操作
{
[self.tableView insertRowsAtIndexPaths:@[newIndexPath] withRowAnimation:UITableViewRowAnimationAutomatic];
}
else if (type == NSFetchedResultsChangeUpdate)//更新操作
{
//首先获取cell;
UITableViewCell *cell = [self.fetchedRC objectAtIndexPath:indexPath];
//调用configCell方法
[self configCell:cell andIndexPath:indexPath];
//重新加载指定行
[self.tableView reloadRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationAutomatic];
}
}
@end
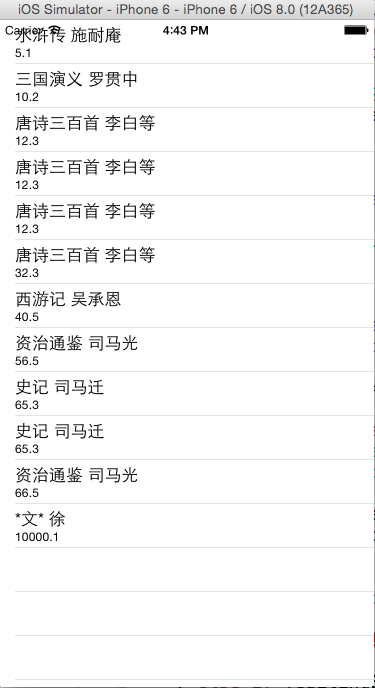
一般来说,你会创建一个NSFetchedResultsController实例作为tableview的成员变量。初始化的时候,你提供四个参数:
1。 一个fetchrequest.必须包含一个sortdescriptor用来给结果集排序。
2。 一个managedobject context。 控制器用这个context来执行取数据的请求。
3。 一个可选的keypath作为sectionname。控制器用keypath来把结果集拆分成各个section。(传nil代表只有一个section)
//初始化fetchedRC必须要排序
request.fetchBatchSize = 20;
NSSortDescriptor *priceSort = [NSSortDescriptor sortDescriptorWithKey:@"price" ascending:YES];
AppDelegate *appDelegate = [[UIApplication sharedApplication]delegate];
//如果要对上下文内容进行修改,可managedObjectContent存在于Appdelegate中,须通过协议进行引用。
NSFetchedResultController与UITableView的更多相关文章
- iOS UITableView 与 UITableViewController
很多应用都会在界面中使用某种列表控件:用户可以选中.删除或重新排列列表中的项目.这些控件其实都是UITableView 对象,可以用来显示一组对象,例如,用户地址薄中的一组人名.项目地址. UITab ...
- UITableView(二)
#import "ViewController.h" @interface ViewController () @end @implementation ViewControlle ...
- iOS: 在UIViewController 中添加Static UITableView
如果你直接在 UIViewController 中加入一个 UITableView 并将其 Content 属性设置为 Static Cells,此时 Xcode 会报错: Static table ...
- iOS 编辑UITableView(根据iOS编程编写)
上个项目我们完成了 JXHomepwner 简单的应用展示,项目地址.本节我们需要在上节项目基础上,增加一些响应用户操作.包括添加,删除和移动表格. 编辑模式 UITableView 有一个名为 e ...
- 使用Autolayout实现UITableView的Cell动态布局和高度动态改变
本文翻译自:stackoverflow 有人在stackoverflow上问了一个问题: 1 如何在UITableViewCell中使用Autolayout来实现Cell的内容和子视图自动计算行高,并 ...
- iOS - UITableView中Cell重用机制导致Cell内容出错的解决办法
"UITableView" iOS开发中重量级的控件之一;在日常开发中我们大多数会选择自定Cell来满足自己开发中的需求, 但是有些时候Cell也是可以不自定义的(比如某一个简单的 ...
- UITableView cell复用出错问题 页面滑动卡顿问题 & 各杂七杂八问题
UITableView 的cell 复用机制节省了内存,但是有时对于多变的自定义cell,重用时会出现界面出错(例如复用出错,出现cell混乱重影).滑动卡顿等问题,这里只简单敲下几点复用出错时的解决 ...
- UITableview delegate dataSource调用探究
UITableview是大家常用的UIKit组件之一,使用中我们最常遇到的就是对delegate和dataSource这两个委托的使用.我们大多数人可能知道当reloadData这个方法被调用时,de ...
- UITableView点击每个Cell,Cell的子内容的收放
关于点击TableviewCell的子内容收放问题,拿到它的第一个思路就是, 方法一: 运用UITableview本身的代理来处理相应的展开收起: 1.代理:- (void)tableView:(UI ...
随机推荐
- 用于ARM上的FFT与IFFT源代码-C语言
/********************************************************************************* 程序名称:快速傅里叶变换(FFT) ...
- AndroidManifest.xml详细分析
原文地址: http://my.eoe.cn/1087692/archive/5927.html 一.关于AndroidManifest.xmlAndroidManifest.xml 是每个andro ...
- scrapy 简介
Scrapy是用纯Python实现一个为了爬取网站数据.提取结构性数据而编写的应用框架. Scrapy架构图(绿线是数据流向): Scrapy Engine(引擎): 负责Spider.ItemPip ...
- CentOS7环境下在/离线安装GCC与GCC-C++
前几天在准备CentOS7下的编译环境,在线安装GCC和GCC-C++非常简单,只要机器是联网的在Terminal窗口中按顺序分别输入 yum install gcc yum install gcc- ...
- 循环遍历完成后再进行else判断
有时需要在循环遍历完后再进行else操作,通常的办法是在循环前设置一个flag,用于判断.具体例子如下:
- 【转】开发者应该了解的API技术清单
[转载贴] 作为一名开发者,诚然编写代码如同作家提笔挥毫,非常有成就感与乐趣,但同时我也觉得删除代码是件不相伯仲的美事.为什么呢?因为在进行删除工作 时,意味着自己找出了造成干扰的位置,意味着找到了冗 ...
- splay tree成段更新,成段查询poj3466
线段树入门题,换成splay tree 来搞搞. #include <stdio.h> #include <string.h> #include <algorithm&g ...
- 用户画像 销量预测 微观 宏观 bi
w 目前我们没有自己的平台 第三方平台又不会给任何我们想要的数据 没有用户的注册信息 全天候的行为信息 用户画像没法做 针对我们业务的bi做的思路是什么呢 数据中心怎么做销量预测呢 ...
- BigDecimal使用整理
BigDecimal使用整理 一. BigDecimal简介 计算机计算中无论是float还是double都是浮点数,由于计算机是二进制的,导致在在浮点数计算时会出现精度丢失,因此引入BigD ...
- 一篇搞定spring Jpa操作数据库
开始之前你必须在项目配置好数据库,本文使用的spring boot,相比spring,spring boot省去了很多各种对以来组件复杂的配置,直接在pom配置组件,完后会自动帮我们导入组件 < ...