function declarations are hoisted and class declarations are not 变量提升
Classes - JavaScript | MDN https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes
Hoisting
An important difference between function declarations and class declarations is that function declarations are hoisted and class declarations are not. You first need to declare your class and then access it, otherwise code like the following will throw aReferenceError
:
const p = new Rectangle(); // ReferenceError
class Rectangle {}
https://developer.mozilla.org/en-US/docs/Glossary/Hoisting
Hoisting
Hoisting is a term you will not find used in any normative specification prose prior to ECMAScript® 2015 Language Specification. Hoisting was thought up as a general way of thinking about how execution contexts (specifically the creation and execution phases) work in JavaScript. However, the concept can be a little confusing at first.
Conceptually, for example, a strict definition of hoisting suggests that variable and function declarations are physically moved to the top of your code, but this is not in fact what happens. Instead, the variable and function declarations are put into memory during the compile phase, but stay exactly where you typed them in your code.
Learn moreSection
Technical exampleSection
One of the advantages of JavaScript putting function declarations into memory before it executes any code segment is that it allows you to use a function before you declare it in your code. For example:
function catName(name) {
console.log("My cat's name is " + name);
}
catName("Tigger");
/*
The result of the code above is: "My cat's name is Tigger"
*/
The above code snippet is how you would expect to write the code for it to work. Now, let's see what happens when we call the function before we write it:
catName("Chloe");
function catName(name) {
console.log("My cat's name is " + name);
}
/*
The result of the code above is: "My cat's name is Chloe"
*/
Even though we call the function in our code first, before the function is written, the code still works. This is because of how context execution works in JavaScript.
Hoisting works well with other data types and variables. The variables can be initialized and used before they are declared.
Only declarations are hoistedSection
JavaScript only hoists declarations, not initializations. If a variable is declared and initialized after using it, the value will be undefined. For example:
console.log(num); // Returns undefined
var num;
num = 6;
If you declare the variable after it is used, but initialize it beforehand, it will return the value:
num = 6;
console.log(num); // returns 6
var num;
The below two examples demonstrate the same behavior.
var x = 1; // Initialize x
console.log(x + " " + y); // '1 undefined'
var y = 2; // Initialize y
// The above example is implicitly understood as this:
var x; // Declare x
var y; // Declare y
// End of the hoisting.
x = 1; // Initialize x
console.log(x + " " + y); // '1 undefined'
y = 2; // Initialize y
Technical referenceSection
- JavaScript: Understanding the Weird Parts — Udemy.com Course
- var statement — MDN
- function statement — MDN
Document Tags and Contributors
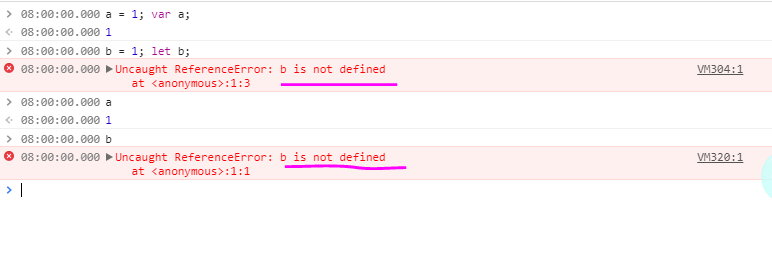
function declarations are hoisted and class declarations are not 变量提升的更多相关文章
- JS中的函数声明和函数表达式的区别,即function(){}和var function(){},以及变量提升、作用域和作用域链
一.前言 Uncaught TypeError: ... is not a function function max(){}表示函数声明,可以放在代码的任何位置,也可以在任何地方成功调用: var ...
- JavaScript 风格指导(Airbnb版)
JavaScript 风格指导(Airbnb版) 用更合理的方式写 JavaScript 原文 翻译自 Airbnb JavaScript Style Guide . 目录 类型 引用 对象 数组 解 ...
- Airbnb JavaScript Style Guide
Airbnb JavaScript Style Guide() { 用更合理的方式写 JavaScript ES5 的编码规范请查看版本一,版本二. 翻译自 Airbnb JavaScrip ...
- ES6 开发规范-最佳实践
ES6 开发规范(最佳实践) 本文为开发规范,收集方便日后查看. [开发规范]https://blog.csdn.net/zzzkk2009/article/details/53171058?utm_ ...
- ES6 学习笔记(1)
恰逢换工作之际,新公司的是以 ES6 + webpack + vue 为技术栈, 正好ES6是我下个学习目标, 因此买了阮老师的 ES6标准入门,也当是支持阮老师了. 笔记将会照着这本书的阅读展开而做 ...
- Javascript 优化项目代码技巧之语言基础(一)
Javascript的弱类型以及函数作用域等规则使用编写Javascript代码极为容易,但是编写可维护.高质量的代码却变得十分困难,这个系列的文章将总结在项目开发过程中,能够改善代码可读性. ...
- ( 译、持续更新 ) JavaScript 上分小技巧(四)
后续如有内容,本篇将会照常更新并排满15个知识点,以下是其他几篇译文的地址: 第一篇地址:( 译.持续更新 ) JavaScript 上分小技巧(一) 第二篇地址:( 译.持续更新 ) JavaScr ...
- Javascript 优化
Javascript 优化 作者:@gzdaijie本文为作者原创,转载请注明出处:http://www.cnblogs.com/gzdaijie/p/5324489.html 目录 1.全局变量污染 ...
- JavaScript(五):函数(闭包,eval)
1.函数的申明:三种方法: function命令 函数表达式:变量赋值 Function构造函数 //method 1: function命令 function test(){ console.log ...
随机推荐
- JMeter进行http接口测试
Jmter工具设计之初是用于做性能测试的,它在实现对各种接口的调用方面已经做的比较成熟,因此,本次直接使用Jmeter工具来完成对Http接口的测试. 一.开发接口测试案例的整体方案: 第一步:我们要 ...
- Delphi Integer 转成单字节
整形不能超过256 b:=Byte(StrToInt(n)); var s: string; b: Byte; begin s := Edit1.Text; b := Byte(Str ...
- Docker 创建image
images 是containers的基础.每次使用docker run 命令都要指定image. 列出本地images zane@zane-V:~$ docker images REPO ...
- C++中的void类型
Technorati 标签: void,指针 1.1. void类型 void类型其实是一种用于语法性的类型,而不是数据类型,主要用于作为函数的参数或返回值,或者定义void指针,表示一种未知类型. ...
- angular js 使用$location问题整理
angular js 自带的$location方法十分强大,通过使用$location方法.我们能够获取到server的port.杂乱连接中的path()部分(/所包括的部分). 例: // give ...
- zabbix学习系列之触发器
触发器的简介 监控项仅负责收集数据,而通常收集数据的目的还包括在某指标对应的数据超出合理范围时给相关人员发送告警信息,"触发器"正式 用于为监控项所收集的数据定义阈值 每一个触发器 ...
- .NET中XML 注释 SandCastle 帮助文件.hhp 使用HTML Help Workshop生成CHM文件
一.摘要 在本系列的第一篇文章介绍了.NET中XML注释的用途, 本篇文章将讲解如何使用XML注释生成与MSDN一样的帮助文件.主要介绍NDoc的继承者:SandCastle. .SandCastle ...
- Win7如何修复开机画面
将下面文件保存为"修复Win7开机画面.bat"双击运行即可 bcdedit /set {current} locale zh-CN
- 我用select做多路复用踩到的坑
既然说是用select踩到的坑,那么就先直接贴一段使用select的代码上来瞅一下: bool SocketAction(int fd, const char* buf, size_t len, ui ...
- python 查询,子查询以及1对多查询
1.添加数据: # 方法1:对象.save() book = Book(**kwargs) book.save() # 方法2:类.create(**kwargs) Book.create(**kwa ...