IOS详解TableView——内置刷新,EGO,以及搜索显示控制器
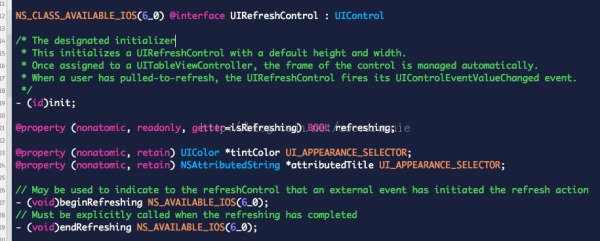
- /******内置刷新的常用属性设置******/
- UIRefreshControl *refresh = [[UIRefreshControl alloc] init];
- refresh.attributedTitle = [[NSAttributedString alloc] initWithString:@"下拉刷新"];
- refresh.tintColor = [UIColor blueColor];
- [refresh addTarget:self action:@selector(pullToRefresh) forControlEvents:UIControlEventValueChanged];
- self.refreshControl = refresh;
- //下拉刷新
- - (void)pullToRefresh
- {
- //模拟网络访问
- [UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
- self.refreshControl.attributedTitle = [[NSAttributedString alloc] initWithString:@"刷新中"];
- double delayInSeconds = 1.5;
- dispatch_time_t popTime = dispatch_time(DISPATCH_TIME_NOW, (int64_t)(delayInSeconds * NSEC_PER_SEC));
- dispatch_after(popTime, dispatch_get_main_queue(), ^(void){
- _rowCount += 5;
- [self.tableView reloadData];
- //刷新结束时刷新控件的设置
- [self.refreshControl endRefreshing];
- self.refreshControl.attributedTitle = [[NSAttributedString alloc] initWithString:@"下拉刷新"];
- [UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
- _bottomRefresh.frame = CGRectMake(0, 44+_rowCount*RCellHeight, 320, RCellHeight);
- });
- }
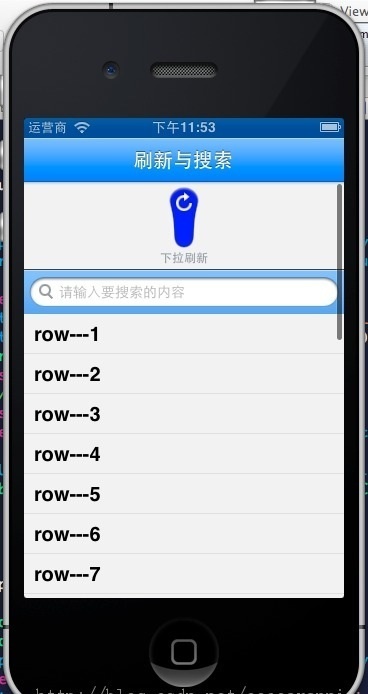
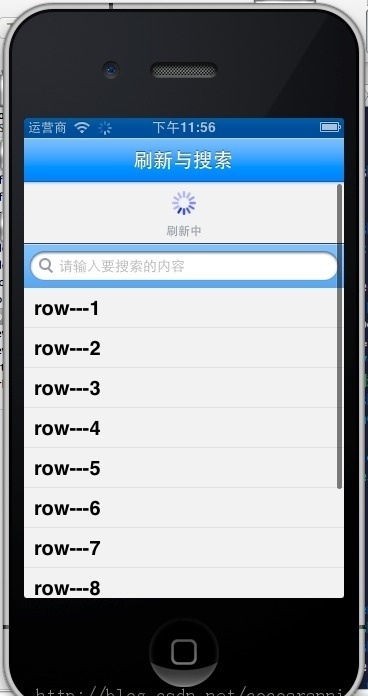
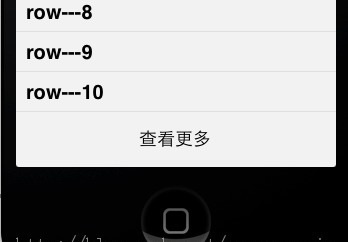
- /******自定义查看更多属性设置******/
- _bottomRefresh = [UIButton buttonWithType:UIButtonTypeCustom];
- [_bottomRefresh setTitle:@"查看更多" forState:UIControlStateNormal];
- [_bottomRefresh setTitleColor:[UIColor blackColor] forState:UIControlStateNormal];
- [_bottomRefresh setContentEdgeInsets:UIEdgeInsetsMake(15, 0, 0, 0)];
- [_bottomRefresh addTarget:self action:@selector(upToRefresh) forControlEvents:UIControlEventTouchUpInside];
- _bottomRefresh.frame = CGRectMake(0, 44+_rowCount*RCellHeight, 320, RCellHeight);
- [self.tableView addSubview:_bottomRefresh];
- //上拉加载
- - (void)upToRefresh
- {
- _bottomRefresh.enabled = NO;
- [SVProgressHUD showWithStatus:@"加载中..."];
- [UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
- double delayInSeconds = 1.5;
- dispatch_time_t popTime = dispatch_time(DISPATCH_TIME_NOW, (int64_t)(delayInSeconds * NSEC_PER_SEC));
- dispatch_after(popTime, dispatch_get_main_queue(), ^(void){
- _rowCount += 5;
- [self.tableView reloadData];
- [UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
- [SVProgressHUD showSuccessWithStatus:@"加载完成"];
- _bottomRefresh.frame = CGRectMake(0, 44+_rowCount*RCellHeight, 320, RCellHeight);
- _bottomRefresh.enabled = YES;
- });
- }
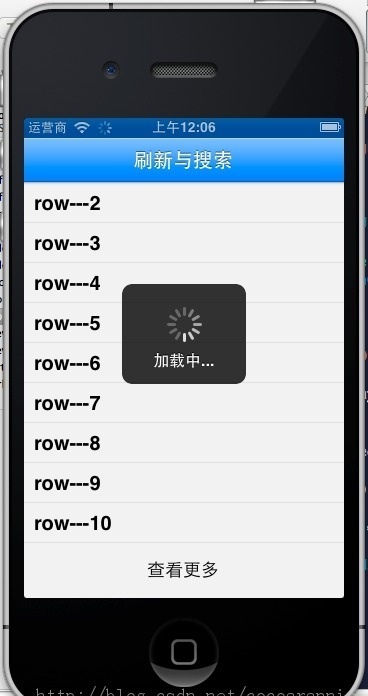
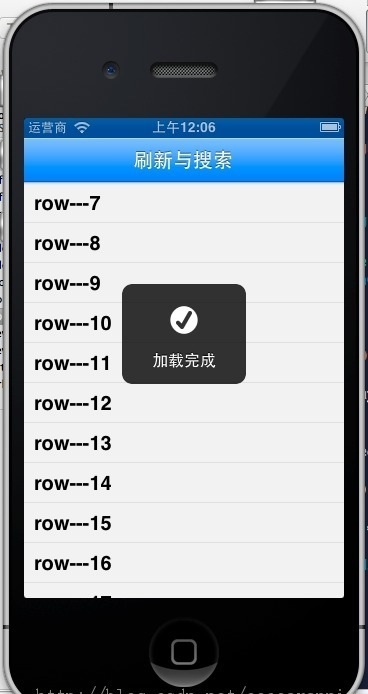
- /******头部的下拉刷新******/
- _headerRefreshView = [[EGORefreshTableHeaderView alloc] initWithFrame:CGRectMake(0, 0 - self.tableView.frame.size.height, 320, self.tableView.frame.size.height) withType:EGORefreshHeader];
- _headerRefreshView.delegate = self;
- [self.tableView addSubview:_headerRefreshView];
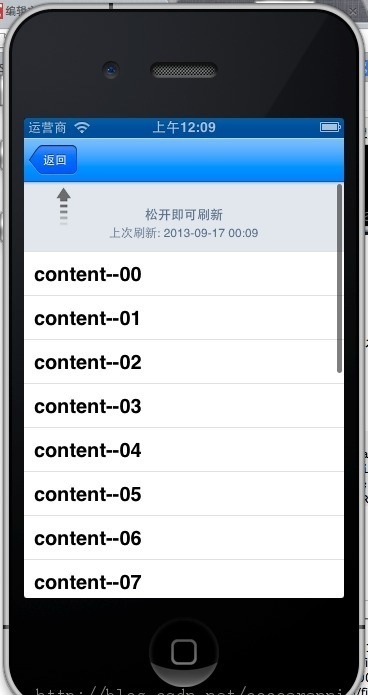
- #pragma mark -
- #pragma mark EGORefresh Delegate
- - (BOOL)egoRefreshTableDataSourceIsLoading:(UIView *)view
- {
- return _isRefreshing;
- }
- - (NSDate *)egoRefreshTableDataSourceLastUpdated:(UIView *)view
- {
- return [NSDate date];
- }
- - (void)egoRefreshTableDidTriggerRefresh:(UIView*)view{
- [self reloadTableViewDataSource];
- double delayInSeconds = 1.5;
- dispatch_time_t popTime = dispatch_time(DISPATCH_TIME_NOW, (int64_t)(delayInSeconds * NSEC_PER_SEC));
- dispatch_after(popTime, dispatch_get_main_queue(), ^(void){
- [self performSelector:@selector(doneLoadingTableViewData)];
- });
- }
- - (void)reloadTableViewDataSource
- {
- _rowCount += 5;
- _isRefreshing = YES;
- }
- - (void)doneLoadingTableViewData
- {
- // model should call this when its done loading
- _isRefreshing = NO;
- [self.tableView reloadData];
- [_headerRefreshView egoRefreshScrollViewDataSourceDidFinishedLoading:self.tableView];
- //header
- _headerRefreshView.frame = CGRectMake(0, 0-self.tableView.bounds.size.height- 44, self.tableView.frame.size.width, self.tableView.bounds.size.height + 44);
- }
- - (void)scrollViewDidScroll:(UIScrollView *)scrollView
- {
- [_headerRefreshView egoRefreshScrollViewDidScroll:scrollView];
- }
- - (void)scrollViewDidEndDragging:(UIScrollView *)scrollView willDecelerate:(BOOL)decelerate
- {
- [_headerRefreshView egoRefreshScrollViewDidEndDragging:scrollView];
- }
- - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
- {
- if (tableView == self.searchDisplayController.searchResultsTableView)
- {
- return _searchList.count;
- }
- else
- {
- return _rowCount;
- }
- }
- - (BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString
- {
- NSPredicate *predicate = [NSPredicate predicateWithFormat:@"self CONTAINS %@", searchString];
- if (_searchList)
- {
- _searchList = nil;
- }
- _searchList = [NSMutableArray arrayWithArray:[_dataList filteredArrayUsingPredicate:predicate]];
- return YES;
- }
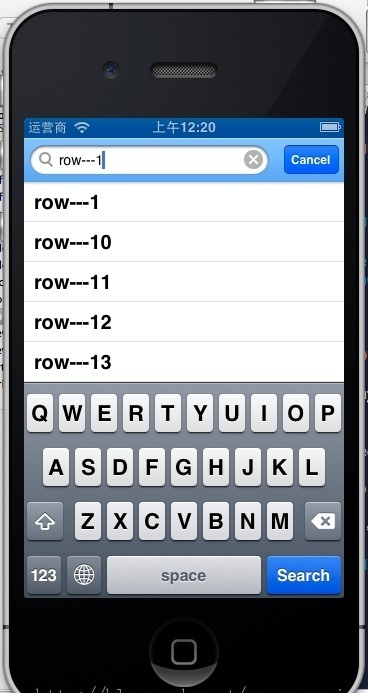
响应表视图点击的方法也需要判断,然后根据需求实现
IOS详解TableView——内置刷新,EGO,以及搜索显示控制器的更多相关文章
- IOS详解TableView——选项抽屉(天猫商品列表)
在之前的有篇文章讲述了利用HeaderView来写类似QQ好友列表的表视图. 这里写的天猫抽屉其实也可以用该方法实现,具体到细节每个人也有所不同.这里采用的是点击cell对cell进行运动处理以展开“ ...
- IOS详解TableView——对话聊天布局的实现
上篇博客介绍了如何使用UITableView实现类似QQ的好友界面布局.这篇讲述如何利用自定义单元格来实现聊天界面的布局. 借助单元格实现聊天布局难度不大,主要要解决的问题有两个: 1.自己和其他人说 ...
- iOS:详解MJRefresh刷新加载更多数据的第三方库
原文链接:http://www.ios122.com/2015/08/mjrefresh/ 简介 MJRefresh这个第三方库是李明杰老师的杰作,这个框架帮助我们程序员减轻了超级多的麻烦,节约了开发 ...
- 详解DevExpress.LookUpEdit控件实现自动搜索定位功能(转)
转载自csdn博客 爱拼才会赢 的博客 地址是详解DevExpress.LookUpEdit控件实现自动搜索定位功能(转)
- 详解Linux内核红黑树算法的实现
转自:https://blog.csdn.net/npy_lp/article/details/7420689 内核源码:linux-2.6.38.8.tar.bz2 关于二叉查找树的概念请参考博文& ...
- 干货:Java多线程详解(内附源码)
线程是程序执行的最小单元,多线程是指程序同一时间可以有多个执行单元运行(这个与你的CPU核心有关). 在java中开启一个新线程非常简单,创建一个Thread对象,然后调用它的start方法,一个 ...
- IOS系统唤醒微信内置地图
针对前一篇文章 唤醒微信内置地图 后来发现在IOS系统中运行 唤醒地图会无效的问题.因为在IOS上无法解析这俩个字符串的问题! 需要对经纬度 使用 “parseFloat()”进行转换 返回一个浮点数 ...
- iOS 详解NSXMLParser方法解析XML数据方法
前一篇文章已经介绍了如何通过URL从网络上获取xml数据.下面介绍如何将获取到的数据进行解析. 下面先看看xml的数据格式吧! <?xml version="1.0" enc ...
- Vue实战041:获取当前客户端IP地址详解(内网和外网)
前言 我们经常会有需求,希望能获取的到当前用户的IP地址,而IP又分为公网ip(也称外网)和私网IP(也称内网IP),IP地址是IP协议提供的一种统一的地址格式,每台设备都设定了一个唯一的IP地址”, ...
随机推荐
- where过滤字句
无条件为真 为假 别名不能直接用来当做查询来用 只能如此 下面这些语句是可以使用的,使用的是排序规则
- 【转】发布一个基于NGUI编写的UI框架
发布一个基于NGUI编写的UI框架 1.加载,显示,隐藏,关闭页面,根据标示获得相应界面实例 2.提供界面显示隐藏动画接口 3.单独界面层级,Collider,背景管理 4.根据存储的导航信息完成界面 ...
- js检测浏览器是否支持某属性
以检测浏览器是否支持 input 标签的 required 属性为例: var isSupport = 'required' in document.createElement('input');
- iOS开发小技巧
1. 解析详情页(是webView)遇到的3个问题: 1.图片太大,超出屏幕范围 2.怎么在webView上面添加一行文字 3.文字太小 1.解决方法 webView.scalesPageToFit ...
- Android (二维码)关于java.lang.UnsatisfiedLinkError的小案例
在许多项目中我们都会用到第三方动态库.so文件,但是往往会引来很多烦恼,比如:Java.lang.UnsatisfiedLinkError - ::-/com.ishow.scan E/Android ...
- .net开发中要注意的事项
1.尽量少用static 当对象被定义为static时,这个对象所占有的内存将不会被回收.有时我们会将经常调用的对象(变量)定义为static,以便提高程序的运行性能.所以,不常用的就不要再定义为st ...
- Comet 反Ajax: jQuery与PHP实现Ajax长轮询
原文地址(http://justcode.ikeepstudying.com/2016/08/comet-%E5%8F%8Dajax-%E5%9F%BA%E4%BA%8Ejquery%E4%B8%8E ...
- Spark+Hadoop+Hive集群上数据操作记录
[rc@vq18ptkh01 ~]$ hadoop fs -ls / drwxr-xr-x+ - jc_rc supergroup 0 2016-11-03 11:46 /dt [rc@vq18ptk ...
- [原创] Linux下几种文件传输命令 sz rz sftp scp介绍
Linux下几种文件传输命令 sz rz sftp scp介绍 1.sftp Secure Ftp 是一个基于SSH安全协议的文件传输管理工具.由于它是基于SSH的,会在传输过程中对用户的密码.数据等 ...
- C++ 学习笔记(2) —— float 和 double 的精度
Size Range Precision 4 bytes ±1.18 x 10-38 to ±3.4 x 1038 6-9 significant digits, typically 7 8 byte ...