iOS:选择器控件UIPickerView的详解和演示
选择器控件UIPickerView:
@protocol UIPickerViewDataSource, UIPickerViewDelegate;
@interface UIPickerView : UIView <NSCoding>
@property(nonatomic,assign) id<UIPickerViewDataSource> dataSource; // default is nil,设置代理
@property(nonatomic,assign) id<UIPickerViewDelegate> delegate; // default is nil,设置代理
@property(nonatomic) BOOL showsSelectionIndicator; // default is NO
@property(nonatomic,readonly) NSInteger numberOfComponents; //列数
二、协议UIPickerViewDataSource
@protocol UIPickerViewDataSource<NSObject>
@required //必须要实现的方法
// 返回的列显示的数量。
- (NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView;
//返回行数在每个组件(每一列)
- (NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component;
@end
三、协议UIPickerViewDelegate
@protocol UIPickerViewDelegate<NSObject>
@optional //可以选择执行的方法
//每一列组件的列宽度
- (CGFloat)pickerView:(UIPickerView *)pickerView widthForComponent:(NSInteger)component;
//每一列组件的行高度
- (CGFloat)pickerView:(UIPickerView *)pickerView rowHeightForComponent:(NSInteger)component;
// 返回每一列组件的每一行的标题内容
- (NSString *)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component;
// 返回每一列组件的每一行的标题内容的属性
- (NSAttributedString *)pickerView:(UIPickerView *)pickerView attributedTitleForRow:(NSInteger)row forComponent:(NSInteger)component;
// 返回每一列组件的每一行的视图显示
- (UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row forComponent:(NSInteger)component reusingView:(UIView *)view;
//执行选择某列某行的操作
- (void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component;
@end
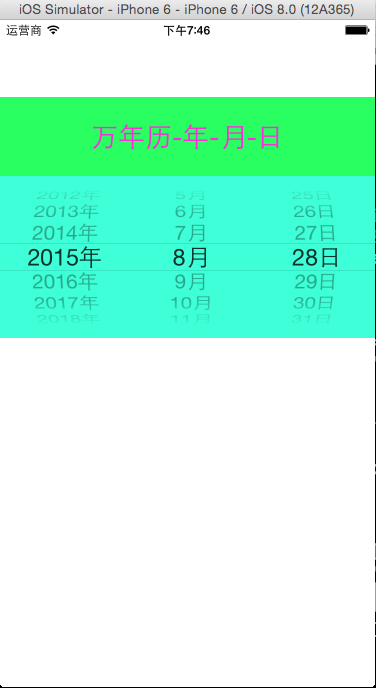
#import <UIKit/UIKit.h> @interface ViewController : UIViewController<UIPickerViewDataSource,UIPickerViewDelegate>
@property(nonatomic,strong)NSArray *years;
@property(nonatomic,strong)NSArray *months;
@property(nonatomic,strong)NSArray *days; @end
#import "ViewController.h" @interface ViewController ()
@property (weak, nonatomic) IBOutlet UIPickerView *pickerView; @end @implementation ViewController - (void)viewDidLoad
{
[super viewDidLoad];
//初始化数据
NSMutableArray *multYears = [NSMutableArray array];//年
for(int i=; i<; i++)
{
NSString *year = [NSString stringWithFormat:@"20%02d年",i+];
[multYears addObject:year];
}
self.years = multYears; NSMutableArray *multMonths = [NSMutableArray arrayWithCapacity:];//月
for(int i=; i<=; i++)
{
NSString *month = [NSString stringWithFormat:@"%d月",i];
[multMonths addObject:month];
}
self.months = multMonths; NSMutableArray *multDays = [NSMutableArray arrayWithCapacity:];//日
for(int i=; i<=; i++)
{
NSString *day = [NSString stringWithFormat:@"%d日",i];
[multDays addObject:day];
}
self.days = multDays; //设置pickerView的数据源和代理
self.pickerView.dataSource = self;
self.pickerView.delegate = self; //显示当前日期
NSDate *now = [NSDate date];
//分解日期
NSCalendar *calendar = [[NSCalendar alloc]initWithCalendarIdentifier:NSCalendarIdentifierGregorian]; NSCalendarUnit unitFlags = NSCalendarUnitYear|NSCalendarUnitMonth|NSCalendarUnitDay;
NSDateComponents *components = [calendar components:unitFlags fromDate:now]; //设置pickerView显示当前日期
NSInteger year = [components year];
[self.pickerView selectRow:year-- inComponent: animated:year]; NSInteger month = [components month];
[self.pickerView selectRow:month- inComponent: animated:month]; NSInteger day = [components day];
[self.pickerView selectRow:day- inComponent: animated:day];
} #pragma mark - pickerView的代理方法
-(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView
{
return ;
}
-(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component
{
NSInteger row = ;
switch (component)
{
case :
row = self.years.count;
break;
case :
row = self.months.count;
break;
case :
row = self.days.count;
break;
}
return row;
} -(NSString*)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component
{
NSString *title;
switch (component)
{
case :
title = self.years[row];
break;
case :
title = self.months[row];
break;
case :
title = self.days[row];
break;
}
return title;
}
-(void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component
{
NSString *strDate = [NSString stringWithFormat:@"%@-%@-%@",
self.years[[pickerView selectedRowInComponent:]],
self.months[[pickerView selectedRowInComponent:]],
self.days[[pickerView selectedRowInComponent:]]];
NSLog(@"%@",strDate);
}
@end
演示二:制作简单的字体表,包括字体类型、大小、颜色
源码如下:
#import <UIKit/UIKit.h> @interface ViewController : UIViewController<UIPickerViewDataSource,UIPickerViewDelegate>
@property(nonatomic,strong) NSArray *fontNames;
@property(nonatomic,strong) NSArray *fontSizes;
@property(nonatomic,strong) NSArray *fontColors;
@end
#import "ViewController.h" @interface ViewController ()
@property (weak, nonatomic) IBOutlet UILabel *label;
@property (weak, nonatomic) IBOutlet UIPickerView *pickerView; @end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
self.fontNames = [UIFont familyNames];//字体名字 NSMutableArray *mutsize = [NSMutableArray arrayWithCapacity:];//字体大小
for(int i=; i<=; i++)
{
[mutsize addObject:[NSString stringWithFormat:@"%d",+i]];
}
self.fontSizes = mutsize; self.fontColors = @[
@{@"name":@"红色",@"color":[UIColor redColor]},
@{@"name":@"黑色",@"color":[UIColor blackColor]},
@{@"name":@"蓝色",@"color":[UIColor blueColor]},
@{@"name":@"黄色",@"color":[UIColor yellowColor]},
@{@"name":@"绿色",@"color":[UIColor greenColor]}
];
//设置pickerView的代理和数据源
self.pickerView.dataSource = self;
self.pickerView.delegate = self; //设置label的font
self.label.font = [UIFont fontWithName:self.fontNames[] size:[self.fontSizes[] integerValue]]; self.label.textColor = [self.fontColors[] objectForKey:@"color"];
} #pragma mark - pickerView的代理方法
-(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView
{
return ;
}
-(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component
{
NSInteger row = ;
switch (component)
{
case :
row = self.fontNames.count;
break;
case :
row = self.fontSizes.count;
break;
case :
row = self.fontColors.count;
break;
}
return row;
}
-(NSString*)pickerView:(UIPickerView *)pickerView titleForRow:(NSInteger)row forComponent:(NSInteger)component
{
NSString *title;
switch (component)
{
case :
title = self.fontNames[row];
break;
case :
title = self.fontSizes[row];
break;
case :
title = [self.fontColors[row] objectForKey:@"name"];
break;
}
return title;
}
//设置每一列的宽度
-(CGFloat)pickerView:(UIPickerView *)pickerView widthForComponent:(NSInteger)component
{
CGFloat width = 0.0f;
switch (component)
{
case :
width = pickerView.frame.size.width/;
break;
case :
width = pickerView.frame.size.width/;
break;
case :
width = pickerView.frame.size.width/;
break;
}
return width;
} -(void)pickerView:(UIPickerView *)pickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component
{
//设置label的font
self.label.font = [UIFont fontWithName:self.fontNames[[pickerView selectedRowInComponent:]] size:[self.fontSizes[[pickerView selectedRowInComponent:]] integerValue]]; self.label.textColor = [self.fontColors[[pickerView selectedRowInComponent:]] objectForKey:@"color"];
}
@end
演示三:制作简单的图库浏览器
源码如下:
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController<UIPickerViewDelegate,UIPickerViewDataSource>
@end
#import "ViewController.h" @interface ViewController ()
@property (weak, nonatomic) IBOutlet UIPickerView *pickerView;
@property (strong,nonatomic)NSArray *imageNames;
@end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
NSMutableArray *mutImageNames = [NSMutableArray arrayWithCapacity:];
for(int i=; i<; i++)
{
[mutImageNames addObject:[NSString stringWithFormat:@"%d.png",i]];
}
self.imageNames = mutImageNames; //设置pickerView的数据源和代理
self.pickerView.dataSource = self;
self.pickerView.delegate = self; }
#pragma mark - pickerview代理方法
-(NSInteger)numberOfComponentsInPickerView:(UIPickerView *)pickerView
{
return ;
}
-(NSInteger)pickerView:(UIPickerView *)pickerView numberOfRowsInComponent:(NSInteger)component
{
return self.imageNames.count;
}
//设置行高
-(CGFloat)pickerView:(UIPickerView *)pickerView rowHeightForComponent:(NSInteger)component
{
UIImage *image = [UIImage imageNamed:self.imageNames[]];
return image.size.height;
}
//设置每行显示的视图
-(UIView *)pickerView:(UIPickerView *)pickerView viewForRow:(NSInteger)row forComponent:(NSInteger)component reusingView:(UIView *)view
{
UIImage *image = [UIImage imageNamed:self.imageNames[row]];
//UIImageView *imageView = [[UIImageView alloc]initWithImage:image];
//imageView.frame = CGRectMake(0, 0, image.size.width, image.size.height);
//return imageView; UIButton *button = [[UIButton alloc]initWithFrame:CGRectMake(, ,image.size.width, image.size.height)];
[button setBackgroundImage:image forState:UIControlStateNormal];
[button addTarget:self action:@selector(buttonClicked:) forControlEvents:UIControlEventTouchUpInside];
return button;
}
-(void)buttonClicked:(UIButton *)sender
{
NSLog(@"button clicked");
}
@end
iOS:选择器控件UIPickerView的详解和演示的更多相关文章
- 《手把手教你》系列技巧篇(三十八)-java+ selenium自动化测试-日历时间控件-下篇(详解教程)
1.简介 理想很丰满现实很骨感,在应用selenium实现web自动化时,经常会遇到处理日期控件点击问题,手工很简单,可以一个个点击日期控件选择需要的日期,但自动化执行过程中,完全复制手工这样的操作就 ...
- iOS:提示框(警告框)控件UIActionSheet的详解
提示框(警告框)控件2:UIActionSheet 功能:当点击按钮或标签等时,弹出一个提示框,显示必要的提示,然后通过添加的按钮完成需要的功能.它与导航栏类似,它继承自UIView. 风格类型: ...
- iOS:提示框(警告框)控件UIAlertView的详解
提示框(警告框)控件:UIAlertView 功能:当点击按钮或标签等时,弹出一个提示框,显示必要的提示,然后通过添加的按钮完成需要的功能. 类型:typedef NS_ENUM(NSInte ...
- iOS:下拉刷新控件UIRefreshControl的详解
下拉刷新控件:UIRefreshControl 1.具体类信息: @interface UIRefreshControl : UIControl //继承控制类 - (instancetype)ini ...
- iOS:网页视图控件UIWebView的详解
网页视图控件:UIWebView 功能:它是继承于UIView的,是一个内置的浏览器控件,以用来浏览从网络下载下来的网页或者本地上加载下来的文档. 枚举: //网页视图导航类型 typedef NS_ ...
- iOS:图像选取器控制器控件UIImagePickerController的详解
图像选择控制器:UIImagePickerController 功能:用于选取相册或相机等里面的照片. @interface UIImagePickerController : UINavigatio ...
- 《手把手教你》系列技巧篇(三十七)-java+ selenium自动化测试-日历时间控件-上篇(详解教程)
1.简介 我们在实际工作中,有可能遇到有些web产品,网页上有一些时间选择,然后支持按照不同时间段范围去筛选数据.网页上日历控件一般,是一个文本输入框,鼠标点击,就会弹出日历界面,可以选择具体日期.这 ...
- delphi控件属性大全-详解-简介
http://blog.csdn.net/u011096030/article/details/18716713 button 组件: CAPTION 属性 :用于在按钮上显示文本内容 Cancel ...
- 【VB技巧】VB ListView 控件功能使用详解
来源:http://lcx.cc/?i=494 ListView控件 在工具箱上击鼠标右键,选择快捷菜单的Components(部件)项,在控件列表中选择Microsoft Windows Commo ...
随机推荐
- 逻辑回归(Logistic Regression)
转载请注明出自BYRans博客:http://www.cnblogs.com/BYRans/ 本文主要讲解分类问题中的逻辑回归.逻辑回归是一个二分类问题. 二分类问题 二分类问题是指预测的y值只有两个 ...
- Python yield 使用浅析
转载来自: http://www.ibm.com/developerworks/cn/opensource/os-cn-python-yield/ 初学 Python 的开发者经常会发现很多 Pyth ...
- 【Java EE 学习 29 下】【JDBC编程中操作Oracle数据库】【调用存储过程的方法】
疑问:怎样判断存储过程执行之后返回值是否为空. 一.连接oracle数据库 1.需要的jar包:在安装的oracle中就有,所以不需要到官网下载,我的oracle11g下:D:\app\kdyzm\p ...
- redis非特定类型命令
1. key查询 keys my* #获取当前数据库中符合模式的所有key exists mykey #查看key是否还存在 2. 数据库操作 redis默认一个实例的数据库是16个[db0-db15 ...
- CSS3动画效果-7.13
例如: <body> <div class="div1"></div> </body> CSS: @keyframes myfirs ...
- js实现去重字符串
实现去重字符串主要是把重复的字符与原来的字符(先push()进入一个数组存起来)相匹配,如果match返回的不是null则说明重复,就删除掉: <script> var str = pro ...
- 分配问题与Hungarian算法
分配问题与Hungarian算法 分配问题 指派问题 匈牙利算法 匈牙利方法是一种能够在多项式时间内解决分配问题(assignment problem)的组合优化算法.它由Harold Kuhn 与1 ...
- 自己写了一个无缝滚动的插件(jQuery)
效果图: html代码: 1 <h1>无缝滚动,向右滚动</h1> 2 <ul id="guoul1"> 3 <li><img ...
- XVI Open Cup named after E.V. Pankratiev. GP of SPB
A. Bubbles 枚举两个点,求出垂直平分线与$x$轴的交点,答案=交点数+1. 时间复杂度$O(n^2\log n)$. #include<cstdio> #include<a ...
- Sass和compass 安装 和配合grunt实时显示 [Sass和compass学习笔记]
demo 下载http://vdisk.weibo.com/s/DOlfkrAWjkF/1401192855 为什么要学习Sass和compass ?提高站独立和代码产品化的绝密武器,尤其是程序化cs ...