POJ1149(最大流)
PIGS
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 21678 | Accepted: 9911 |
Description
All data concerning customers planning to visit the farm on that particular day are available to Mirko early in the morning so that he can make a sales-plan in order to maximize the number of pigs sold.
More precisely, the procedure is as following: the customer arrives, opens all pig-houses to which he has the key, Mirko sells a certain number of pigs from all the unlocked pig-houses to him, and, if Mirko wants, he can redistribute the remaining pigs across the unlocked pig-houses.
An unlimited number of pigs can be placed in every pig-house.
Write a program that will find the maximum number of pigs that he can sell on that day.
Input
The next line contains M integeres, for each pig-house initial number of pigs. The number of pigs in each pig-house is greater or equal to 0 and less or equal to 1000.
The next N lines contains records about the customers in the following form ( record about the i-th customer is written in the (i+2)-th line):
A K1 K2 ... KA B It means that this customer has key to the pig-houses marked with the numbers K1, K2, ..., KA (sorted nondecreasingly ) and that he wants to buy B pigs. Numbers A and B can be equal to 0.
Output
Sample Input
3 3
3 1 10
2 1 2 2
2 1 3 3
1 2 6
Sample Output
7
Source
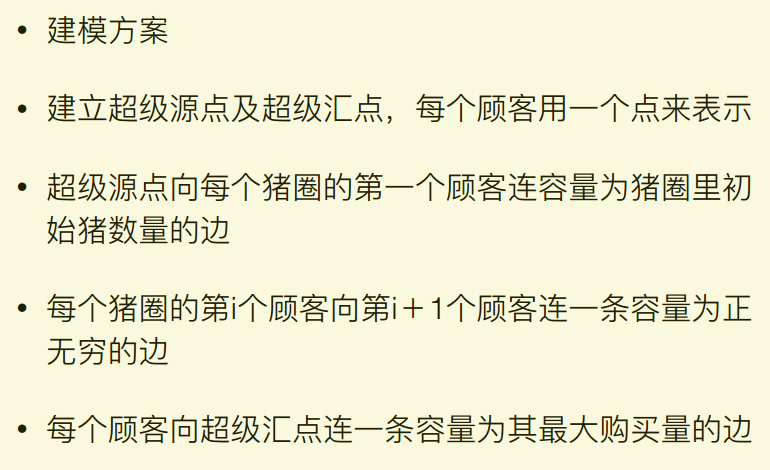
//2017-08-23
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
#include <queue>
#include <vector> using namespace std; const int N = ;
const int M = ;
const int INF = 0x3f3f3f3f;
int head[N], tot;
struct Edge{
int next, to, w;
}edge[N<<]; void add_edge(int u, int v, int w){
edge[tot].w = w;
edge[tot].to = v;
edge[tot].next = head[u];
head[u] = tot++; edge[tot].w = ;
edge[tot].to = u;
edge[tot].next = head[v];
head[v] = tot++;
} struct Dinic{
int level[N], S, T;
void init(int _S, int _T){
S = _S;
T = _T;
tot = ;
memset(head, -, sizeof(head));
}
bool bfs(){
queue<int> que;
memset(level, -, sizeof(level));
level[S] = ;
que.push(S);
while(!que.empty()){
int u = que.front();
que.pop();
for(int i = head[u]; i != -; i = edge[i].next){
int v = edge[i].to;
int w = edge[i].w;
if(level[v] == - && w > ){
level[v] = level[u]+;
que.push(v);
}
}
}
return level[T] != -;
}
int dfs(int u, int flow){
if(u == T)return flow;
int ans = , fw;
for(int i = head[u]; i != -; i = edge[i].next){
int v = edge[i].to, w = edge[i].w;
if(!w || level[v] != level[u]+)
continue;
fw = dfs(v, min(flow-ans, w));
ans += fw;
edge[i].w -= fw;
edge[i^].w += fw;
if(ans == flow)return ans;
}
if(ans == )level[u] = ;
return ans;
}
int maxflow(){
int flow = ;
while(bfs())
flow += dfs(S, INF);
return flow;
} }dinic; int house[M]; int main()
{
std::ios::sync_with_stdio(false);
//freopen("input.txt", "r", stdin);
int n, m;
while(cin>>m>>n){
int s = , t = n+;
dinic.init(s, t);
for(int i = ; i <= m; i++)
cin>>house[i];
int k, v;
int book[M];
memset(book, , sizeof(book));
for(int i = ; i <= n; i++){
cin>>k;
int weight = ;
while(k--){
cin>>v;
if(!book[v]){
book[v] = i;
weight += house[v];
}else{
add_edge(book[v], i, INF);
}
}
if(weight)add_edge(s, i, weight);
cin>>v;
add_edge(i, t, v);
}
cout<<dinic.maxflow()<<endl;
}
return ; }
POJ1149(最大流)的更多相关文章
- poj1149最大流经典构图神题
题意:n个顾客依次来买猪,有n个猪房,每个顾客每次可以开若干个房子,买完时,店主可以调整这位顾客 开的猪房里的猪,共m个猪房,每个猪房有若干猪,求最多能卖多少猪. 构图思想:顾客有先后,每个人想要的猪 ...
- POJ1149 最大流经典建图PIG
题意: 有一个人,他有m个猪圈,每个猪圈里都有一定数量的猪,但是他没有钥匙,然后依次来了n个顾客,每个顾客都有一些钥匙,还有他要卖猪的数量,每个顾客来的时候主人用顾客的钥匙打开相应的门,可 ...
- poj 1273.PIG (最大流)
网络流 关键是建图,思路在代码里 /* 最大流SAP 邻接表 思路:基本源于FF方法,给每个顶点设定层次标号,和允许弧. 优化: 1.当前弧优化(重要). 1.每找到以条增广路回退到断点(常数优化). ...
- POJ-1149 PIGS---最大流+建图
题目链接: https://vjudge.net/problem/POJ-1149 题目大意: M个猪圈,N个顾客,每个顾客有一些的猪圈的钥匙,只能购买这些有钥匙的猪圈里的猪,而且要买一定数量的猪,每 ...
- POJ1149 PIGS 【最大流 + 构图】
题目链接:http://poj.org/problem?id=1149 PIGS Time Limit: 1000MS Memory Limit: 10000K Total Submissions ...
- POJ1149 PIGS(最大流)
题意: 有一个人,他有m个猪圈,每个猪圈里面有一定数量的猪,但是每个猪圈的门都是锁着的,他自己没有钥匙,只有顾客有钥匙,一天依次来了n个顾客,(记住是依次来的)他们每个人都有一些钥匙,和他 ...
- POJ1149 PIGS [最大流 建图]
PIGS Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 20662 Accepted: 9435 Description ...
- 【POJ1149&BZOJ1280】PIGS(最大流)
题意:Emmy在一个养猪场工作.这个养猪场有M个锁着的猪圈,但Emmy并没有钥匙. 顾客会到养猪场来买猪,一个接着一个.每一位顾客都会有一些猪圈的钥匙,他们会将这些猪圈打开并买走固定数目的猪. 所有顾 ...
- poj1149 PIGS 最大流(神奇的建图)
一开始不看题解,建图出错了.后来发现是题目理解错了. if Mirko wants, he can redistribute the remaining pigs across the unlock ...
随机推荐
- 跟着刚哥学习Spring框架--事务配置(七)
事务 事务用来保证数据的完整性和一致性. 事务应该具有4个属性:原子性.一致性.隔离性.持久性.这四个属性通常称为ACID特性.1.原子性(atomicity).一个事务是一个不可分割的工作单位,事务 ...
- webApp开发中的总结
meta标签: H5页面窗口自动调整到设备宽度,并禁止用户缩放页面 <meta name="viewport" content="width=device-wid ...
- cytoscape.js在vue项目中的安装及案例
1. 安装: npm i cytoscape --save 2. 引入:main.js import cytoscape from 'cytoscape'; Vue.prototype.$cytosc ...
- copy代码的时候,如何去掉代码前边的编号
从网页上拷贝下来的代码前面总有编号,如何去掉! 1.使用正则表达式:在editorplus(notepad++)里按ctrl+h,弹出框里勾选上“正则表达式(regular expression)”, ...
- Oracle 获取本周、本月、本季、本年的第一天和最后一天
Oracle 获取本周.本月.本季.本年的第一天和最后一天 --本周 select trunc(sysdate, 'd') + 1 from dual; select trunc(sysdate, ' ...
- C#:多线程、线程同步与死锁
推荐阅读: C#线程系列讲座(1):BeginInvoke和EndInvoke方法 C#线程系列讲座(2):Thread类的应用 C#线程系列讲座(3):线程池和文件下载服务器 C#线程系列讲座(4) ...
- OpenShift Redhat 搭建NodeJS环境
https://openshift.redhat.com/ OpenShift 是 redhat 公司推出的一个 PaaS 云计算应用平台,开发者可在上面构建.测试.部署和运行应用程序,它支持 Jav ...
- asp.net core web 添加角色管理
新建asp.net core web应用 添加RolesAdminController [Authorize(Roles = "Admin")] public class Role ...
- java+hibernate+mysql
实体类News package org.mythsky.hibernatedemo; import javax.persistence.*; @Entity @Table(name="new ...
- 全网最详细的启动zkfc进程时,出现INFO zookeeper.ClientCnxn: Opening socket connection to server***/192.168.80.151:2181. Will not attempt to authenticate using SASL (unknown error)解决办法(图文详解)
不多说,直接上干货! at org.apache.zookeeper.ClientCnxnSocketNIO.doTransport(ClientCnxnSocketNIO.java:) at org ...