POJ1328 Radar Installation 【贪心·区间选点】
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 54593 | Accepted: 12292 |
Description
sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write
a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
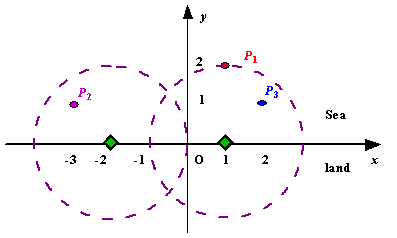
Figure A Sample Input of Radar Installations
Input
two integers representing the coordinate of the position of each island. Then a blank line follows to separate the cases.
The input is terminated by a line containing pair of zeros
Output
Sample Input
3 2
1 2
-3 1
2 1 1 2
0 2 0 0
Sample Output
Case 1: 2
Case 2: 1
Source
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <algorithm> #define maxn 1010
using namespace std; struct Node {
double u, v;
friend bool operator<(const Node& a, const Node& b) {
return a.u < b.u;
}
} E[maxn];
int N, D; int main() {
int i, ok, id, ans, cas = 1;
double x, y, d, flag;
while(scanf("%d%d", &N, &D), N) {
printf("Case %d: ", cas++);
ok = 1; id = 0;
for(i = 0; i < N; ++i) {
scanf("%lf%lf", &x, &y);
if(y > D) ok = 0;
if(!ok) continue;
d = sqrt(D * D - y * y);
E[id].u = x - d;
E[id++].v = x + d;
} if(!ok) {
printf("-1\n");
continue;
} sort(E, E + id); flag = E[0].v; ans = 1;
for(i = 1; i < N; ++i) {
if(E[i].u <= flag) {
if(E[i].v <= flag) flag = E[i].v;
continue;
}
++ans; flag = E[i].v;
} printf("%d\n", ans);
}
return 0;
}
POJ1328 Radar Installation 【贪心·区间选点】的更多相关文章
- poj1328 Radar Installation —— 贪心
题目链接:http://poj.org/problem?id=1328 题解:区间选点类的题目,求用最少的点以使得每个范围都有点存在.以每个点为圆心,r0为半径,作圆.在x轴上的弦即为雷达可放置的范围 ...
- UVALive 2519 Radar Installation 雷达扫描 区间选点问题
题意:在坐标轴中给出n个岛屿的坐标,以及雷达的扫描距离,要求在y=0线上放尽量少的雷达能够覆盖全部岛屿. 很明显的区间选点问题. 代码: /* * Author: illuz <iilluzen ...
- POJ1328 Radar Installation(贪心)
题目链接. 题意: 给定一坐标系,要求将所有 x轴 上面的所有点,用圆心在 x轴, 半径为 d 的圆盖住.求最少使用圆的数量. 分析: 贪心. 首先把所有点 x 坐标排序, 对于每一个点,求出能够满足 ...
- [POJ1328]Radar Installation
[POJ1328]Radar Installation 试题描述 Assume the coasting is an infinite straight line. Land is in one si ...
- POJ - 1328 Radar Installation(贪心区间选点+小学平面几何)
Input The input consists of several test cases. The first line of each case contains two integers n ...
- POJ--1328 Radar Installation(贪心 排序)
题目:Radar Installation 对于x轴上方的每个建筑 可以计算出x轴上一段区间可以包含这个点 所以就转化成 有多少个区间可以涵盖这所有的点 排序之后贪心一下就ok 用cin 好像一直t看 ...
- zoj1360/poj1328 Radar Installation(贪心)
对每个岛屿,能覆盖它的雷达位于线段[x-sqrt(d*d-y*y),x+sqrt(d*d+y*y)],那么把每个岛屿对应的线段求出来后,其实就转化成了经典的贪心法案例:区间选点问题.数轴上有n个闭区间 ...
- POJ1328——Radar Installation
Radar Installation Description Assume the coasting is an infinite straight line. Land is in one side ...
- POJ 1328 Radar Installation 贪心 A
POJ 1328 Radar Installation https://vjudge.net/problem/POJ-1328 题目: Assume the coasting is an infini ...
随机推荐
- JavaScript中数组Array.sort()排序方法详解
JavaScript中数组的sort()方法主要用于对数组的元素进行排序.其中,sort()方法有一个可选参数.但是,此参数必须是函数. 数组在调用sort()方法时,如果没有传参将按字母顺序(字符编 ...
- centos7安装gitlab与gitlab的汉化
Gitlab概述 GitLab是一个利用 Ruby on Rails 开发的开源应用程序,实现一个自托管的Git项目仓库,可通过Web界面进行访问公开的或者私人项目. GitLab拥有与Github ...
- 在ros下使用tf
tf真是一个好东西,把坐标变换都简化了 首先tf需要有一个broadcaster #include <ros/ros.h> #include <tf/transform_broadc ...
- CDH-5.7.0:基于Parcels方式离线安装配置
http://shiyanjun.cn/archives/1728.html https://www.waitig.com/cdh%E5%AE%89%E8%A3%85.html
- [BZOJ1044][HAOI2008]木棍分割 二分+贪心+dp+前缀和优化
1044: [HAOI2008]木棍分割 Time Limit: 10 Sec Memory Limit: 162 MB Submit: 4112 Solved: 1577 [Submit][St ...
- 牛客网 牛客练习赛7 A.骰子的游戏
A.骰⼦的游戏 时间限制:C/C++ 1秒,其他语言2秒空间限制:C/C++ 32768K,其他语言65536K64bit IO Format: %lld 题目描述 在Alice和Bob面前的是两个骰 ...
- luogu P1340 兽径管理
题目描述 约翰农场的牛群希望能够在 N 个(1<=N<=200) 草地之间任意移动.草地的编号由 1到 N.草地之间有树林隔开.牛群希望能够选择草地间的路径,使牛群能够从任一 片草地移动到 ...
- POJ 1769 Minimizing maximizer(DP+zkw线段树)
[题目链接] http://poj.org/problem?id=1769 [题目大意] 给出一些排序器,能够将区间li到ri进行排序,排序器按一定顺序摆放 问在排序器顺序不变的情况下,一定能够将最大 ...
- SQL表操作习题6 36~45题
- Ubuntu 16.04服务器版查看DHCP自动分配的IP、网关、DNS
说明: 1.在服务器版本中,没有想桌面版一样的NetworkManager工具,所以的一切都是在命令行上操作的. 2.本文只针对DHCP默认分配的IP进行查看. 方法: 1.如果要使用DHCP,那么需 ...