POJ2676Sudoku(类似于八皇后)
Time Limit: 2000MS | Memory Limit: 65536K | |||
Total Submissions: 16444 | Accepted: 8035 | Special Judge |
Description
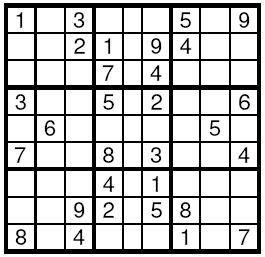
Input
Output
Sample Input
1
103000509
002109400
000704000
300502006
060000050
700803004
000401000
009205800
804000107
Sample Output
143628579
572139468
986754231
391542786
468917352
725863914
237481695
619275843
854396127
Source
题意:每行每列每个小的九宫格 每个数字只出现一次;
看着很高大上的题目做起来这么好玩,搜索真是很神奇!应该是第一次写带有返回值的搜索
转载请注明出处:優YoU http://user.qzone.qq.com/289065406/blog/1303713313
大致题意:
九宫格问题,也有人叫数独问题
把一个9行9列的网格,再细分为9个3*3的子网格,要求每行、每列、每个子网格内都只能使用一次1~9中的一个数字,即每行、每列、每个子网格内都不允许出现相同的数字。
0是待填位置,其他均为已填入的数字。
要求填完九宫格并输出(如果有多种结果,则只需输出其中一种)
如果给定的九宫格无法按要求填出来,则输出原来所输入的未填的九宫格
解题思路:
DFS试探,失败则回溯
用三个数组进行标记每行、每列、每个子网格已用的数字,用于剪枝
bool row[10][10]; //row[i][x] 标记在第i行中数字x是否出现了
bool col[10][10]; //col[j][y] 标记在第j列中数字y是否出现了
bool grid[10][10]; //grid[k][x] 标记在第k个3*3子格中数字z是否出现了
row 和 col的标记比较好处理,关键是找出grid子网格的序号与 行i列j的关系
即要知道第i行j列的数字是属于哪个子网格的
首先我们假设子网格的序号如下编排:
由于1<=i、j<=9,我们有: (其中“/”是C++中对整数的除法)
令a= i/3 , b= j/3 ,根据九宫格的 行列 与 子网格 的 关系,我们有:
不难发现 3a+b=k
即 3*(i/3)+j/3=k
又我在程序中使用的数组下标为 1~9,grid编号也为1~9
因此上面的关系式可变形为 3*((i-1)/3)+(j-1)/3+1=k
有了这个推导的关系式,问题的处理就变得非常简单了,直接DFS即可
#include <iostream>
#include <cstring>
#include <algorithm>
#include <cstdio> using namespace std;
int row[][],col[][],grid[][];
int g[][];
bool dfs(int x,int y)
{
if(x == )
{
return true;
}
bool flag = false;
if(g[x][y])
{
if(y == )
{
flag = dfs(x + , );
}
else
{
flag = dfs(x, y + );
}
if(flag)
return true;
else
return false;
}
else if(g[x][y] == )
{
for(int i = ; i <= ; i++)
{
int k = (x - ) / * + (y - ) / + ;
if(col[y][i] == && row[x][i] == && grid[k][i] == )
{
g[x][y] = i;
col[y][i] = ;
row[x][i] = ;
grid[k][i] = ;
if(y < )
{
flag = dfs(x,y + );
}
else
{
flag = dfs(x + , );
}
if(flag == false)
{
g[x][y] = ;
col[y][i] = ;
row[x][i] = ;
grid[k][i] = ;
}
else
return true;
}
}
}
return false;
}
int main()
{
int t;
scanf("%d", &t);
getchar();
while(t--)
{
memset(row,,sizeof(row));
memset(col,,sizeof(col));
memset(grid,,sizeof(grid));
memset(g,,sizeof(g));
char ch;
for(int i = ; i <= ; i++)
{
for(int j = ; j <= ; j++)
{
scanf("%c", &ch);
if(ch != '')
{
g[i][j] = ch - '';
row[i][ch - ''] = ;
col[j][ch - ''] = ;
int k = (i - ) / * + (j - ) / + ;
grid[k][ch - ''] = ;
}
}
getchar();
}
dfs(,);
for(int i = ; i <= ; i ++)
{
for(int j = ; j <= ; j++)
{
printf("%d",g[i][j]);
}
printf("\n");
}
}
return ;
}
POJ2676Sudoku(类似于八皇后)的更多相关文章
- POJ 1321 棋盘问题【DFS/回溯/放与不放/类似n皇后】
棋盘问题 Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 62164 Accepted: 29754 Description 在一 ...
- 回溯算法——解决n皇后问题
所谓回溯(backtracking)是通过系统地搜索求解问题的方法.这种方法适用于类似于八皇后这样的问题:求得问题的一个解比较困难,但是检查一个棋局是否构成解很容易. 不多说,放上n皇后的回溯问题代码 ...
- 2n皇后问题-------递归 暴力求解题与分布讨论题
问题描述 给定一个n*n的棋盘,棋盘中有一些位置不能放皇后.现在要向棋盘中放入n个黑皇后和n个白皇后,使任意的两个黑皇后都不在同一行.同一列或同一条对角线上,任意的两个白皇后都不在同一行.同一列或同一 ...
- 二模12day1解题报告
T1.笨笨与电影票(ticket) 有n个1和m个0,求每个数前1的个数都大于等于0的个数的排列数. 非常坑的一道题,推导过程很烦.首先求出所有排列数是 C(n+m,m),然后算不合法的个数. 假设存 ...
- Codevs p1004 四子连棋
四子连棋 题目描述 Description 在一个4*4的棋盘上摆放了14颗棋子,其中有7颗白色棋子,7颗黑色棋子,有两个空白地带,任何一颗黑白棋子都可以向 ...
- leetcode 39 Combination Sum --- java
Given a set of candidate numbers (C) and a target number (T), find all unique combinations in C wher ...
- HDU 1045(炮台安置 DFS)
题意是在 n*n 的方格中进行炮台的安置,炮台不能处于同一行或同一列(类似于八皇后问题),但若是炮台间有墙壁阻挡,则可以同时安置这对炮台.问图中可以安放的最大炮台数目. 用深搜的方法,若此处为空地,则 ...
- POJ1321 棋盘问题 —— DFS回溯
题目链接:http://poj.org/problem?id=1321 棋盘问题 Time Limit: 1000MS Memory Limit: 10000K Total Submissions ...
- USACO1.5 Checker Challenge(类n皇后问题)
B - B Time Limit:1000MS Memory Limit:16000KB 64bit IO Format:%lld & %llu Description E ...
随机推荐
- Jython概要
1.安装jython 1.1 进入http://www.jython.org/downloads.html ,网页上会显示当前最稳定的版本(The most current stable releas ...
- php基础21:上传文件
<?php /* 通过使用 PHP 的全局数组 $_FILES,你可以从客户计算机向远程服务器上传文件 第一个参数是表单的 input name,第二个下标可以是 "name" ...
- [WEB API] CLIENT 指定请求及回应格式(XML/JSON)
[Web API] Client 指定请求及响应格式(xml/json) Web API 支持的格式请参考 http://www.asp.net/web-api/overview/formats-an ...
- python调用windows api
import ctypes # 方式一 ctypes.windll.user32.MessageBoxA(None, 'message', 'title', 0) # 方式二 ctypes.WinDL ...
- matlab中fopen 和 fprintf函数总结
matlab中fopen函数在指定文件打开的实例如下: *1)"fopen"打开文件,赋予文件代号. 语法1:FID= FOPEN(filename,permission) 用指定 ...
- [CareerCup] 9.4 Subsets 子集合
9.4 Write a method to return all subsets of a set. LeetCode上的原题,请参见我之前的博客Subsets 子集合和Subsets II 子集合之 ...
- CodeIgniter框架中关于URL(index.php)的那些事
最近,在做自己的个人网站时,采用了轻量级的php框架CodeIgniter.乍一看上去,代码清晰简洁,MVC模型非常容易维护.开发时我采用的工具是Netbeans IDE 8.0,当然,本文的内容和开 ...
- 如何将Gate One嵌入我们的Web应用中
参考文档http://liftoff.github.io/GateOne/Developer/embedding.html 从https://github.com/liftoff/GateOne下载的 ...
- 关于#define预处理指令的一个问题
背景:由于经常需要在远程服务端和测试服务端进行切换,所以将接口的地址定义为了一个预处理变量,例如 //#define APIDOMAIN @"http://10.0.0.2" #d ...
- WebGame开发总结
不知不觉我们的项目开发有2年了,这两年来走了很多弯路,也收获了很多,今天在这里做一个总结. 项目基本情况: 服务器端采用c++和c#混合开发,网络层采用c++开发,业务逻辑用c#开发.客户端采用sil ...