Mybatis-Plus - 条件构造器 QueryWrapper 的使用
前言
记录下Mybatis-Plus
中条件构造器Wrapper
的一些基本用法。
查询示例
- 表结构
CREATE TABLE `product` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(100) COLLATE utf8_unicode_ci DEFAULT NULL,
`create_time` datetime DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=35 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci
CREATE TABLE `product_item` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(100) COLLATE utf8_unicode_ci DEFAULT NULL,
`product_id` int(10) unsigned NOT NULL,
`create_time` datetime DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci
- 实现需求:根据
product - id
查询product
实例及其关联的product_item
,如下:
基础代码
ProductController.java
@GetMapping("/{id}")
public ProductWithItemsVo getWithItems(@PathVariable Integer id) {
return productService.getWithItems(id);
}
ProductService.java
public interface ProductService {
ProductWithItemsVo getWithItems(Integer id);
}
ProductServiceImpl.java
@Service
public class ProductServiceImpl extends ServiceImpl<ProductMapper, Product> implements ProductService {
@Autowired
private ProductItemMapper productItemMapper;
@Override
public ProductWithItemsVo getWithItems(Integer id) {
// 实现代码
}
}
mapper
@Repository
public interface ProductMapper extends BaseMapper<Product> {
}
@Repository
public interface ProductItemMapper extends BaseMapper<ProductItem> {
}
model
@Getter
@Setter
@TableName("product")
public class Product {
private Integer id;
private String title;
@JsonIgnore
private Date createTime;
}
@Getter
@Setter
@TableName("product_item")
public class ProductItem {
private Integer id;
private Integer productId;
private String title;
@JsonIgnore
private Date createTime;
}
vo
出参
@Data
@NoArgsConstructor
public class ProductWithItemsVo {
private Integer id;
private String title;
List<ProductItem> items;
/**
* 构造ProductWithItemsVo对象用于出参
* @param product
* @param items
*/
public ProductWithItemsVo(Product product, List<ProductItem> items) {
BeanUtils.copyProperties(product, this);
this.setItems(items);
}
}
QueryWrapper 的基本使用
@Override
public ProductWithItemsVo getWithItems(Integer id) {
Product product = this.getById(id);
if (Objects.isNull(product)) {
System.out.println("未查询到product");
return null;
}
/**
* wrapper.eq("banner_id", id)
* banner_id 数据库字段
* id 判断相等的值
*/
QueryWrapper<ProductItem> wrapper = new QueryWrapper<>();
wrapper.eq("product_id", id);
List<ProductItem> productItems = productItemMapper.selectList(wrapper);
return new ProductWithItemsVo(product, productItems);
}
- 如上代码,通过条件构造器
QueryWrapper
查询出当前product
实例及其关联的product_item
QueryWrapper 的lambada写法
@Override
public ProductWithItemsVo getWithItems(Integer id) {
Product product = this.getById(id);
if (Objects.isNull(product)) {
System.out.println("未查询到product");
return null;
}
QueryWrapper<ProductItem> wrapper = new QueryWrapper<>();
/**
* lambda方法引用
*/
wrapper.lambda().eq(ProductItem::getProductId, id);
List<ProductItem> productItems = productItemMapper.selectList(wrapper);
return new ProductWithItemsVo(product, productItems);
}
- 如上代码,通过条件构造器
QueryWrapper
的lambda
方法引用查询出当前product
实例及其关联的product_item
LambadaQueryWrapper 的使用
LambadaQueryWrapper
用于Lambda
语法使用的QueryWrapper
- 构建
LambadaQueryWrapper
的方式:
/**
* 方式一
*/
LambdaQueryWrapper<ProductItem> wrapper1 = new QueryWrapper<ProductItem>().lambda();
wrapper1.eq(ProductItem::getProductId, id);
List<ProductItem> productItems1 = productItemMapper.selectList(wrapper1);
/**
* 方式二
*/
LambdaQueryWrapper<ProductItem> wrapper2 = new LambdaQueryWrapper<>();
wrapper2.eq(ProductItem::getProductId, id);
List<ProductItem> productItems2 = productItemMapper.selectList(wrapper2);
- 完整代码
@Override
public ProductWithItemsVo getWithItems(Integer id) {
Product product = this.getById(id);
if (Objects.isNull(product)) {
System.out.println("未查询到product");
return null;
}
LambdaQueryWrapper<ProductItem> wrapper = new LambdaQueryWrapper<>();
wrapper.eq(ProductItem::getProductId, id);
List<ProductItem> productItems = productItemMapper.selectList(wrapper);
return new ProductWithItemsVo(product, productItems);
}
- 如上代码,通过条件构造器
LambdaQueryWrapper
查询出当前product
实例及其关联的product_item
LambdaQueryChainWrapper 的链式调用
@Override
public ProductWithItemsVo getWithItems(Integer id) {
Product product = this.getById(id);
if (Objects.isNull(product)) {
System.out.println("未查询到product");
return null;
}
/**
* 链式调用
*/
List<ProductItem> productItems =
new LambdaQueryChainWrapper<>(productItemMapper)
.eq(ProductItem::getProductId, id)
.list();
return new ProductWithItemsVo(product, productItems);
}
- 如上代码,通过链式调用查询出当前
product
实例及其关联的product_item
- End -
﹀
﹀
﹀
梦想是咸鱼
关注一下吧
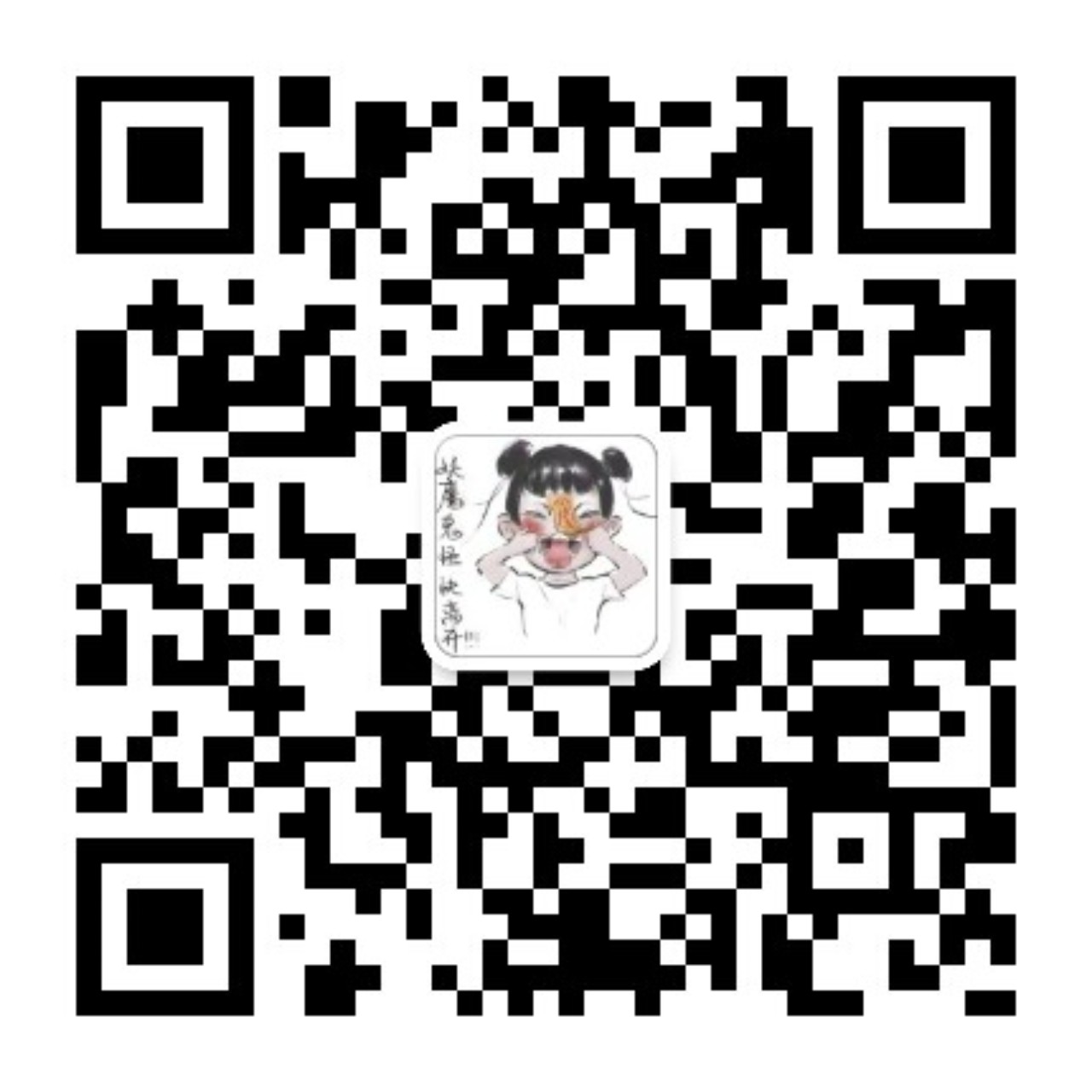
Mybatis-Plus - 条件构造器 QueryWrapper 的使用的更多相关文章
- MyBatis:条件构造器QueryWrapper方法详解
QueryWrapper 说明: 继承自 AbstractWrapper ,自身的内部属性 entity 也用于生成 where 条件及 LambdaQueryWrapper, 可以通过 n ...
- mybatis plus的条件构造器
我们在使用条件构造器的时候要使用QueryWrapper或者UpdateWrapper来充当条件语句来进行构造 QueryWrapper(LambdaQueryWrapper) 和 UpdateWra ...
- MyBatis:MyBatis-Plus条件构造器EntityWrapper
EntityWrapper 简介 1. MybatisPlus 通过 EntityWrapper(简称 EW,MybatisPlus 封装的一个查询条件构造器)或者 Condition(与 EW 类似 ...
- 小书MybatisPlus第2篇-条件构造器的应用及总结
一.条件构造器Wrapper Mybatis Plus为我们提供了如下的一些条件构造器,我们可以利用它们实现查询条件.删除条件.更新条件的构造. 条件构造器用于给如下的Mapper方法传参,通常情况下 ...
- MyBatisPlus性能分析插件,条件构造器,代码自动生成器详解
性能分析插件 我们在平时的开发中,会遇到一些慢sql,测试,druid MP(MyBatisPlus)也提供性能分析插件,如果超过这个时间就停止 不过官方在3.2版本的时候取消了,原因如下 条件构造器 ...
- Mybatis-Plus 实战完整学习笔记(十一)------条件构造器删除,修改,conditon
1.修改功能--其他过滤方式跟select一样 /** * 修改条件构造器 * @throws SQLException */ @Test public void selectUpdate() thr ...
- Mybatis-Plus 实战完整学习笔记(十)------条件构造器核心用法大全(下)
31.升序orderByAsc 31.升序orderByAsc List<Employee> employeeList = employeeMapper.selectList(new Qu ...
- Mybatis-Plus 实战完整学习笔记(九)------条件构造器核心用法大全(上)
一.Mybatisplus通用(公共方法)CRUD,一共17种(3.0.3版),2.3系列也是这么多,这个新版本一定程度进行了改造和删减. 二.构造器UML图(3.0.3)-----实体包装器,主要用 ...
- MybatisPlus学习(四)条件构造器Wrapper方法详解
文章目录 1.条件构造器 2.QueryWrapper 2.1.eq.ne 2.2.gt.ge.lt.le 2.3.between.notBetween 2.4.like.notLike.likeLe ...
随机推荐
- ondblclick="return showCodeList 分析思路
<Div id="divApproveRejectReasonInput" style="display:none"><input class ...
- Tom_No_02 Servlet向流中打印内容,之后在调用finsihResponse,调用上是先发送了body,后发送Header的解释
上次在培训班学上网课的时候就发现了这个问题,一直没有解决,昨天又碰到了,2-3小时也未能发现点端倪,今早又仔细缕了下,让我看了他的秘密 1.Servlet向流中打印内容,之后在调用finsihResp ...
- C# MongoDB添加索引
场景: 在最近的项目中,用到了Mongodb,用它来保存大量工业数据.同时是会根据用户自动建立对应的数据表.这要求同时建立索引来加快查询. 解决: 1.在Nuget包中查询"mongocsh ...
- php cookie赋值使用
setcookie('username',$user,time()+3600*24); //cookie赋值 public function login(){ //cookie 使用 ...
- fastjson 1.2.24 反序列化导致任意命令执行漏洞
漏洞检测 区分 Fastjson 和 Jackson {"name":"S","age":21} 和 {"name":& ...
- Mybatis学习笔记-Mybatis简介
如何获得Mybatis 中文文档 https://github.com/tuguangquan/mybatis Github https://github.com/mybatis/mybatis-3 ...
- ListPopupWindow和Popupwindow的阴影相关问题demo总结
Popupwindow: 优点:可以通过setBackgroundDrawable()来重新设置阴影. 缺点:当AnchorView是可移动的,比如移动到屏幕的左右边界.左下角.右下角时,Popupw ...
- 使用AVPro Video在Unity中播放开场视频(CG)笔记
游戏中的开场CG(播放视频),采用的插件为AVPro Video1.x(和W的版本一致),Unity版本为2018.4.0f1 Asset Store:AVPro Video - Core Andro ...
- LeetCode通关:栈和队列六连,匹配问题有绝招
刷题路线参考: https://github.com/chefyuan/algorithm-base https://github.com/youngyangyang04/leetcode-maste ...
- Java之Listener
Java之Listener Listener监听器 监听器有很多种,大部分还是在GUI用的比较多,这里简单记录一点关于HttpSessionListener的 统计session count List ...