POJ1251 Jungle Roads(Kruskal)(并查集)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 23882 | Accepted: 11193 |
Description
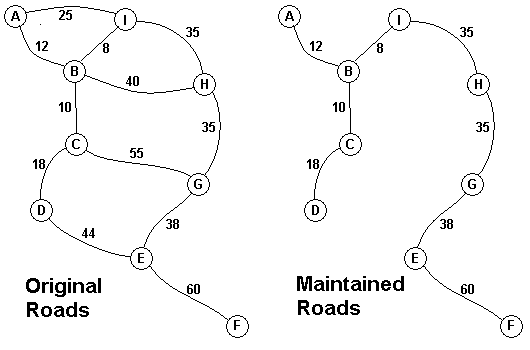
The Head Elder of the tropical island of Lagrishan has a problem. A
burst of foreign aid money was spent on extra roads between villages
some years ago. But the jungle overtakes roads relentlessly, so the
large road network is too expensive to maintain. The Council of Elders
must choose to stop maintaining some roads. The map above on the left
shows all the roads in use now and the cost in aacms per month to
maintain them. Of course there needs to be some way to get between all
the villages on maintained roads, even if the route is not as short as
before. The Chief Elder would like to tell the Council of Elders what
would be the smallest amount they could spend in aacms per month to
maintain roads that would connect all the villages. The villages are
labeled A through I in the maps above. The map on the right shows the
roads that could be maintained most cheaply, for 216 aacms per month.
Your task is to write a program that will solve such problems.
Input
input consists of one to 100 data sets, followed by a final line
containing only 0. Each data set starts with a line containing only a
number n, which is the number of villages, 1 < n < 27, and the
villages are labeled with the first n letters of the alphabet,
capitalized. Each data set is completed with n-1 lines that start with
village labels in alphabetical order. There is no line for the last
village. Each line for a village starts with the village label followed
by a number, k, of roads from this village to villages with labels later
in the alphabet. If k is greater than 0, the line continues with data
for each of the k roads. The data for each road is the village label for
the other end of the road followed by the monthly maintenance cost in
aacms for the road. Maintenance costs will be positive integers less
than 100. All data fields in the row are separated by single blanks. The
road network will always allow travel between all the villages. The
network will never have more than 75 roads. No village will have more
than 15 roads going to other villages (before or after in the alphabet).
In the sample input below, the first data set goes with the map above.
Output
output is one integer per line for each data set: the minimum cost in
aacms per month to maintain a road system that connect all the villages.
Caution: A brute force solution that examines every possible set of
roads will not finish within the one minute time limit.
Sample Input
9
A 2 B 12 I 25
B 3 C 10 H 40 I 8
C 2 D 18 G 55
D 1 E 44
E 2 F 60 G 38
F 0
G 1 H 35
H 1 I 35
3
A 2 B 10 C 40
B 1 C 20
0
Sample Output
216
30
【分析】话不多说,又是最小生成树,直接套模板。
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cmath>
#include <algorithm>
#include <climits>
#include <cstring>
#include <string>
#include <set>
#include <map>
#include <queue>
#include <stack>
#include <vector>
#include <list>
#include<functional>
#define mod 1000000007
#define inf 0x3f3f3f3f
#define pi acos(-1.0)
using namespace std;
typedef long long ll;
const int N=;
const int M=; struct Edg {
int v,u;
int w;
} edg[M];
bool cmp(Edg g,Edg h) {
return g.w<h.w;
}
int n,m,maxn,cnt;
int parent[N];
void init() {
for(int i=; i<n; i++)parent[i]=i;
}
void Build() {
int w,k;
char u,v;
for(int i=; i<n-; i++) {
cin>>u>>k;
while(k--) {
cin>>v>>w;
edg[++cnt].u=u-'A';
edg[cnt].v=v-'A';
edg[cnt].w=w;
}
}
sort(edg,edg+cnt+,cmp);
}
int Find(int x) {
if(parent[x] != x) parent[x] = Find(parent[x]);
return parent[x];
}//查找并返回节点x所属集合的根节点
void Union(int x,int y) {
x = Find(x);
y = Find(y);
if(x == y) return;
parent[y] = x;
}//将两个不同集合的元素进行合并
void Kruskal() {
int sum=;
int num=;
int u,v;
for(int i=; i<=cnt; i++) {
u=edg[i].u;
v=edg[i].v;
if(Find(u)!=Find(v)) {
sum+=edg[i].w;
num++;
Union(u,v);
}
//printf("!!!%d %d\n",num,sum);
if(num>=n-) {
printf("%d\n",sum); break;
}
}
}
int main() {
while(~scanf("%d",&n)&&n) {
cnt=-;
init();
Build();
Kruskal();
}
return ;
}
POJ1251 Jungle Roads(Kruskal)(并查集)的更多相关文章
- POJ1251 Jungle Roads Kruskal+scanf输入小技巧
Jungle Roads The Head Elder of the tropical island of Lagrishan has a problem. A burst of foreign ai ...
- poj1251 Jungle Roads Kruskal算法+并查集
时限: 1000MS 内存限制: 10000K 提交总数: 37001 接受: 17398 描述 热带岛屿拉格里山的首长有个问题.几年前,大量的外援花在了村庄之间的额外道路上.但是丛林不断地超 ...
- TOJ 2815 Connect them (kruskal+并查集)
描述 You have n computers numbered from 1 to n and you want to connect them to make a small local area ...
- Minimum Spanning Tree.prim/kruskal(并查集)
开始了最小生成树,以简单应用为例hoj1323,1232(求连通分支数,直接并查集即可) prim(n*n) 一般用于稠密图,而Kruskal(m*log(m))用于系稀疏图 #include< ...
- Connect the Campus (Uva 10397 Prim || Kruskal + 并查集)
题意:给出n个点的坐标,要把n个点连通,使得总距离最小,可是有m对点已经连接,输入m,和m组a和b,表示a和b两点已经连接. 思路:两种做法.(1)用prim算法时,输入a,b.令mp[a][b]=0 ...
- poj1251 Jungle Roads(Prime || Kruskal)
题目链接 http://poj.org/problem?id=1251 题意 有n个村庄,村庄之间有道路连接,求一条最短的路径能够连接起所有村庄,输出这条最短路径的长度. 思路 最小生成树问题,使用普 ...
- POJ1251 Jungle Roads (最小生成树&Kruskal&Prim)题解
题意: 输入n,然后接下来有n-1行表示边的加边的权值情况.如A 2 B 12 I 25 表示A有两个邻点,B和I,A-B权值是12,A-I权值是25.求连接这棵树的最小权值. 思路: 一开始是在做莫 ...
- HDU1301&&POJ1251 Jungle Roads 2017-04-12 23:27 40人阅读 评论(0) 收藏
Jungle Roads Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 25993 Accepted: 12181 De ...
- hdu 1863 畅通工程(Kruskal+并查集)
畅通工程 Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total Submi ...
随机推荐
- [luoguP3644] [APIO2015]八邻旁之桥(权值线段树)
传送门 首先如果起点终点都在同一侧可以直接处理,如果需要过桥答案再加1 对于k等于1的情况 桥的坐标为x的话,a和b为起点和终点坐标 $ans=\sum_{1}^{n} abs(a_{i}-x)+ab ...
- 版本7以上IE以文件夹视图方式打开FTP的解决
一.问题的提出 版本7以上IE浏览器打开FTP时只出现列表 二.问题的解决 设置ie浏览器选项即可,以ie9为例,设置步骤如下: 1.启动ie,点击设置按钮,弹出菜单选择internet选项命令: 2 ...
- Codeforces Round #487 (Div. 2) A Mist of Florescence (暴力构造)
C. A Mist of Florescence time limit per test 1 second memory limit per test 256 megabytes input stan ...
- css做中划线与文字排版
html: <div class="spilt"> <span class="left"></span> < ...
- Lucene4.6查询时完全跳过打分,提高查询效率的实现方式
由于索引的文件量比较大,而且应用中不需要对文档进行打分,只需要查询出所有满足条件的文档.所以需要跳过打分来提高查询效率.一开始想用ConstantScoreQuery,但是测试发现这个类虽然让所有返回 ...
- 归档普通对象Demo示例程序源代码
源代码下载链接:06-归档普通对象.zip34.2 KB // MJPerson.h // // MJPerson.h // 06-归档普通对象 // // Created by apple o ...
- A trick in Exploit Dev
学习Linux BOF的时候,看了这个文章,https://sploitfun.wordpress.com/2015/06/23/integer-overflow/ ,原文给出的exp无法成功, 此时 ...
- vue 数组、对象 深度拷贝和赋值
由于此对象的引用类型指向的都是一个地址(除了基本类型跟null,对象之间的赋值,只是将地址指向同一个,而不是真正意义上的拷贝) 数组: let a = [11,22,33]; let b = a; / ...
- glib windows下编译
记录的比较粗糙,但是绝对可行的 一些小的瑕疵以后再解决吧 (android版本的过几天再贴,移植到android已经通过) msys+mingw包下载: http://sourceforge.net/ ...
- appium===报错adb server version (31) doesn’t match this client (39); killing…的解决办法
当使用在cmd窗口调用adb shell命令的时候 提示如下: adb server version (31) doesn't match this client (39); killing...er ...