[SinGuLaRiTy] 2017 百度之星程序设计大赛 初赛A
【SinGuLaRiTy-1036】 Copyright (c) SinGuLaRiTy 2017. All Rights Reserved.
小C的倍数问题

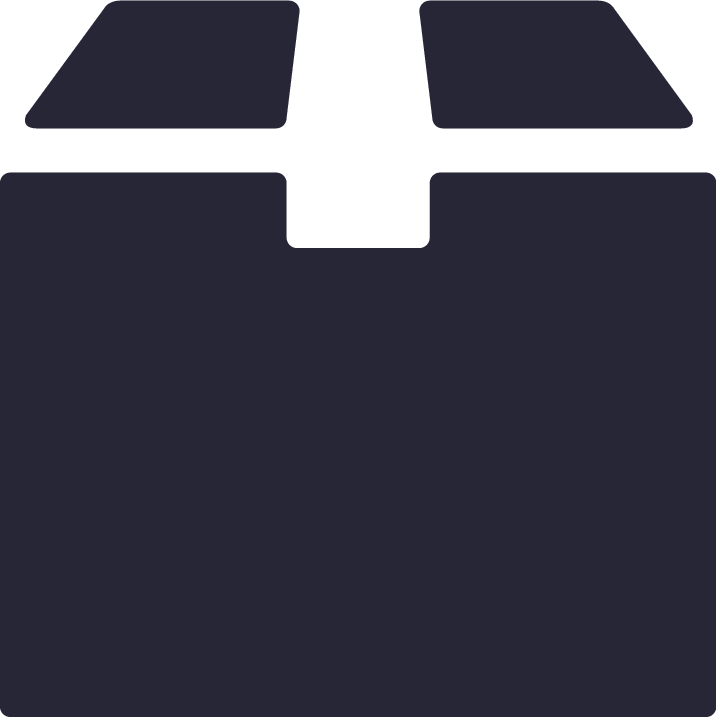
Problem Description
根据小学数学的知识,我们知道一个正整数x是3的倍数的条件是x每一位加起来的和是3的倍数。反之,如果一个数每一位加起来是3的倍数,则这个数肯定是3的倍数。
现在给定进制P,求有多少个B满足P进制下,一个正整数是B的倍数的充分必要条件是每一位加起来的和是B的倍数。
Input
第一行一个正整数T表示数据组数(1<=T<=20)。
接下来T行,每行一个正整数P(2 < P < 1e9),表示一组询问。
Output
对于每组数据输出一行,每一行一个数表示答案。
Sample Input
1
10
Sample Output
3
Code
数学(这道题不算是"数论"吧......)
#include<cstring>
#include<cmath>
#include<algorithm>
#include<iostream>
#include<cstdio>
#include<cstdlib> using namespace std; int T; int main()
{
cin>>T;
for(int i=;i<=T;i++)
{
int n,cnt=;
scanf("%d",&n);
n=n-;
for(int i=;i*i<=n;i++)
{
if(n%i==)
cnt+=;
if(i*i==n)
cnt--;
}
printf("%d\n",cnt);
}
return ;
}
数据分割

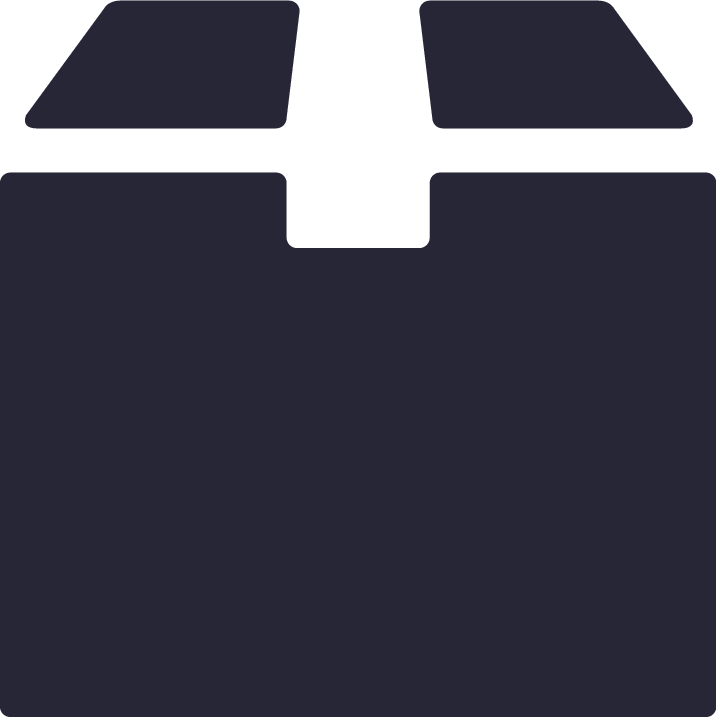
Problem Description
小w来到百度之星的赛场上,准备开始实现一个程序自动分析系统。
这个程序接受一些形如x_i = x_j 或x_i≠x_j 的相等/不等约束条件作为输入,判定是否可以通过给每个 w 赋适当的值,来满足这些条件。
输入包含多组数据。 然而粗心的小w不幸地把每组数据之间的分隔符删掉了。 他只知道每组数据都是不可满足的,且若把每组数据的最后一个约束条件去掉,则该组数据是可满足的。
请帮助他恢复这些分隔符。
Input
第1行:一个数字L,表示后面输入的总行数。
之后L行,每行包含三个整数,i,,j,e描述一个相等/不等的约束条件,若e=1,则该约束条件为x_i = x_j若e=0,则该约束条件为x_i!=x_j 。
i,j,L≤100000
Lxi,xj≤L
Output
输出共T+1行。
第一行一个整数T,表示数据组数。
接下来T行的第i行,一个整数,表示第i组数据中的约束条件个数。
Sample Input
6
2 2 1
2 2 1
1 1 1
3 1 1
1 3 1
1 3 0
Sample Output
1
6
Code
并查集
#include<cstdio>
#include<cstdlib>
#include<cstring>
#include<cmath>
#include<algorithm>
#include<set> #define maxn 200011 using namespace std; set<int> s[maxn]; int T,n=;
int cnt,vis[maxn],sta[maxn],top; struct Node
{
int fa[maxn];
Node()
{
for(int i=;i<=n;i++)
fa[i]=i;
}
int find(int x)
{
return x==fa[x]?x:(fa[x]=find(fa[x]));
}
void Union(int &x,int &y)
{
x=find(x);
y=find(y);
if(x!=y)
fa[x]=y;
}
}node; int x,y,id;
int ans[maxn];
int lans=,Case; void clear()
{
while (top)
{
int now=sta[top--];
node.fa[now]=now;
s[now].clear();
}
ans[cnt++]=Case;
} int main()
{
scanf("%d",&T);
memset(vis,,sizeof(vis));
top=;
cnt=;
for (Case=;Case<=T;Case++)
{
scanf("%d%d%d",&x,&y,&id);
if(vis[x]!=cnt)
vis[x]=cnt,sta[++top]=x;
if(vis[y]!=cnt)
vis[y]=cnt,sta[++top]=y;
x=node.find(x);
y=node.find(y);
if(s[x].size()>s[y].size())
{
int t=x;
x=y;
y=t;
}
if(id)
{
if(s[x].find(y)!=s[x].end())
{
clear();
continue;
}
node.Union(x,y);
for(set<int>::iterator i=s[x].begin();i!=s[x].end();i++)
{
int now=*i;
now=node.find(now);
s[now].erase(x);
s[y].insert(now);
s[now].insert(y);
}
s[x].clear();
}
else
{
if(x==y)
{
clear();
continue;
}
s[x].insert(y);
s[y].insert(x);
}
}
printf("%d\n",cnt-);
for(int i=;i<cnt;i++)
printf("%d\n",ans[i]-ans[i-]);
return ;
}
路径交
Problem Description
给定一棵n个点的树,以及m条路径,每次询问第L条到第R条路径的交集部分的长度(如果一条边同时出现在2条路径上,那么它属于路径的交集)。
Input
第一行一个数n(n<=500,000)接下来n-1行,每行三个数x,y,z,表示一条从x到y并且长度为z的边第n+1行一个数m(m<=500,000)接下来m行,每行两个数u,v,表示一条从u到v的路径接下来一行一个数Q,表示询问次数(Q<=500,000)接下来Q行,每行两个数L和R
Output
Q行,每行一个数表示答案。
Sample Input
4
1 2 5
2 3 2
1 4 3
2
1 2
3 4
1
1 2
Sample Output
Code
线段树区间合并+LCA
#include<cstdlib>
#include<cmath>
#include<cstdio>
#include<cstring>
#include<iostream>
#include<algorithm> #define lson x<<1
#define rson x<<1|1 using namespace std; const int maxn=;
typedef long long ll; struct path
{
int a,b,c;
path(){}
path(int A,int B,int C)
{
a=A,b=B,c=C;
}
}p[maxn],s[maxn<<]; int n,m,q,cnt;
int mn[][maxn<<],Log[maxn<<],pos[maxn],dep[maxn],fa[maxn];
int head[maxn],nxt[maxn<<],val[maxn<<],to[maxn<<];
int cs[]; ll len[maxn]; int MN(int a,int b)
{
return dep[a]<dep[b]?a:b;
} int lca(int a,int b)
{
int x=pos[a],y=pos[b];
if(x>y)
swap(x,y);
int k=Log[y-x+];
return MN(mn[k][x],mn[k][y-(<<k)+]);
} bool cmp(int a,int b)
{
return dep[a]<dep[b];
} path mix(path x,path y)
{
if(!x.c||!y.c)
return path(,,);
cs[]=lca(x.a,y.a);
cs[]=lca(x.a,y.b);
cs[]=lca(x.b,y.a);
cs[]=lca(x.b,y.b);
sort(cs+,cs+,cmp);
int md=max(dep[x.c],dep[y.c]),nd=min(dep[x.c],dep[y.c]);
if(dep[cs[]]<nd||dep[cs[]]<md)
return path(,,);
else
return path(cs[],cs[],lca(cs[],cs[]));
} inline int read()
{
int ret=,f=;
char gc=getchar();
while(gc<''||gc>''){if(gc=='-')f=-f; gc=getchar();}
while(gc>=''&&gc<='') ret=ret*+gc-'',gc=getchar();
return ret*f;
} void add(int a,int b,int c)
{
to[cnt]=b;
val[cnt]=c;
nxt[cnt]=head[a];
head[a]=cnt++;
} void dfs(int x)
{
pos[x]=++pos[];
mn[][pos[]]=x;
for(int i=head[x];i!=-;i=nxt[i])
{
if(to[i]!=fa[x])
{
fa[to[i]]=x,dep[to[i]]=dep[x]+,len[to[i]]=len[x]+val[i],dfs(to[i]);
mn[][++pos[]]=x;
}
}
} void build(int l,int r,int x)
{
if(l==r)
{
s[x]=p[l];
return ;
}
int mid=l+r>>;
build(l,mid,lson),build(mid+,r,rson);
s[x]=mix(s[lson],s[rson]);
} path query(int l,int r,int x,int a,int b)
{
if(a<=l&&r<=b)
return s[x];
int mid=l+r>>;
if(b<=mid)
return query(l,mid,lson,a,b);
if(a>mid)
return query(mid+,r,rson,a,b);
return mix(query(l,mid,lson,a,b),query(mid+,r,rson,a,b));
} int main()
{
n=read();
int i,j,a,b,c;
memset(head,-,sizeof(head));
for(i=;i<n;i++)
{
a=read(),b=read(),c=read();
add(a,b,c),add(b,a,c);
}
dep[]=;
dfs();
for(i=;i<=*n-;i++)
Log[i]=Log[i>>]+;
for(j=;(<<j)<=*n-;j++)
for(i=;i+(<<j)-<=*n-;i++)
mn[j][i]=MN(mn[j-][i],mn[j-][i+(<<j-)]);
m=read();
for(i=;i<=m;i++)
{
p[i].a=read(),p[i].b=read();
p[i].c=lca(p[i].a,p[i].b);
}
build(,m,);
q=read();
for(i=;i<=q;i++)
{
a=read(),b=read();
path ans=query(,m,,a,b);
printf("%I64d\n",len[ans.a]+len[ans.b]-*len[ans.c]);
}
return ;
}
迷宫出逃
Time Limit: 2000/1000 MS (Java/Others)
Memory Limit: 32768/32768 K (Java/Others)
Problem Description
小明又一次陷入了大魔王的迷宫,在无人机的帮忙下,小明获得了整个迷宫的草图。
不同于一般的迷宫,魔王在迷宫里安置了机关,一旦触碰,那么四个方向所在的格子,将翻转其可达性(原先可通过的格子不可通过,反之亦然,机关可以反复触发)。为了防止小明很容易地出逃,魔王在临走前把钥匙丢在了迷宫某处,只有拿到钥匙,小明才能开门在出口处离开迷宫。
万般无奈之下,小明想借助聪明的你,帮忙计算是否有机会离开这个迷宫,最少需要多少时间。(每一单位时间只能向四邻方向走一步)
Input
第一行为 T,表示输入数据组数。
下面 T 组数据,对于每组数据:
第一行是两个数字 n, m(2 < n * m <= 64),表示迷宫的长与宽。
接下来 n 行,每行 m 个字符,‘.’表示空地可以通过,‘x’表示陷阱,‘*’表示机关,‘S’代表起点,‘E’代表出口,‘K’表示钥匙(保证存在且只有一个)。
Output
对第 i 组数据,输出
Case #i:
然后输出一行,仅包含一个整数,表示最少多少步能够拿到钥匙并走出迷魂阵,如果不能则打出-1。
Sample Input
...*x..
...x...
xEx....
*x...K.
.x*...S K..*x..
...x...
xEx....
*x.....
.x*...S ..K*x..
..*x*..
xEx....
*x.....
.x*...S ..K*x..
.*xx*..
*E*....
xx.....
.x*...S S*..
**..
...E
...K
View Sample Input
Sample Output
Case #1:
11
Case #2:
13
Case #3:
13
Case #4:
11
Case #5:
-1
Code
状态压缩+BFS
#include<iostream>
#include<cstdio>
#include<queue>
#include<cstring>
#include<vector> typedef unsigned long long LL; using namespace std; int tx[]={-,,,};
int ty[]={,,-,};
int ii=; struct datatype
{
int bs;
LL zt1;
LL zt2;
int x;
int y;
datatype()
{
bs=;
zt1=;
x=;
y=;
zt2=;
}
};
queue<datatype> team; int maps[][]; struct mapdata
{
int x,y;
LL zt1;
int zt2;
LL count()
{
LL ans=zt1%;
ans=(ans+zt2)%;
ans=(ans+x)%;
ans=(ans+y)%;
return ans;
}
bool operator<(const mapdata &b)const
{
if(this->x!=b.x)
return this->x<b.x;
if(this->y!=b.y)
return this->y<b.y;
if(this->zt1!=b.zt1)
return this->zt1<b.zt1;
return this->zt2<b.zt2;
}
bool operator==(const mapdata &b)const
{
if(this->x!=b.x)
return this->x == b.x;
if(this->y!=b.y)
return this->y == b.y;
if(this->zt1!=b.zt1)
return this->zt1==b.zt1;
return this->zt2==b.zt2;
}
}; char s[][]; vector<mapdata> has[]; void deal()
{
memset(s,,sizeof(s));
memset(maps,,sizeof(maps));
int n,m;
while(!team.empty())
team.pop();
datatype pt;
cin>>n>>m;
for(int i=;i<=;i++)
has[i].clear();
for(int i=;i<=n;i++)
scanf("%s",s[i]+);
int qdx,qdy;
for(int i=;i<=n;i++)
{
for(int j=;j<=m;j++)
{
if(s[i][j]=='.') maps[i][j]=;
if(s[i][j]=='x') maps[i][j]=;
if(s[i][j]=='E') maps[i][j]=-;
if(s[i][j]=='*') maps[i][j]=-;
if(s[i][j]=='K') maps[i][j]=-;
if(s[i][j]=='S')
{
maps[i][j]=,qdx=i,qdy=j;
pt.bs=,pt.zt1=,pt.zt2=,pt.x=qdx,pt.y=qdy;
team.push(pt);
}
}
}
datatype pd;
int ans=-;
while(!team.empty())
{
pt=team.front();
team.pop();
if(maps[pt.x][pt.y]==-)
{
if(pt.zt2==)
{
ans=pt.bs;
break;
}
}
for(int i=;i<;i++)
{
pd.bs=pt.bs+;
pd.zt1=pt.zt1;
pd.zt2=pt.zt2;
pd.x=pt.x+tx[i];
pd.y=pt.y+ty[i];
if(pd.x<=||pd.y<=||pd.x>n||pd.y>m)
continue;
LL cj=(LL)(m)*(pd.x-)+pd.y;
LL zt=((pd.zt1>>(LL)(cj-))&(LL));
if(zt==&&maps[pd.x][pd.y]==)
continue;
if(zt==)
{
if(maps[pd.x][pd.y] != )
continue;
}
LL ws1,ws2,ws3,ws4;
ws1=;ws2=;ws3=;ws4=;
ws1=(pd.x-)*(m)+pd.y;
ws2=(pd.x)*m+(pd.y);
ws3=(pd.x-)*m+(pd.y-);
ws4=(pd.x-)*m+(pd.y+);
if(maps[pd.x][pd.y]==-)
{
if(pd.x->)
{
if((pd.zt1>>(ws1-(LL)))&(LL))
pd.zt1=pd.zt1-((LL)<<(ws1-(LL)));
else
pd.zt1=pd.zt1+((LL)<<(ws1-(LL)));
}
if(pd.x+<=n)
{
if((pd.zt1>>(ws2-(LL)))&(LL))
pd.zt1=pd.zt1-((LL)<<(ws2-(LL)));
else
pd.zt1=pd.zt1+((LL)<<(ws2-(LL)));
}
if(pd.y->)
{
if((pd.zt1>>(ws3-(LL)))&(LL))
pd.zt1=pd.zt1-((LL)<<(ws3-(LL)));
else
pd.zt1=pd.zt1+((LL)<<(ws3-(LL)));
}
if(pd.y+<=m)
{
if((pd.zt1>>(ws4-(LL)))&(LL))
pd.zt1=pd.zt1-((LL)<<(ws4-(LL)));
else
pd.zt1=pd.zt1+((LL)<<(ws4-(LL)));
}
}
if(maps[pd.x][pd.y]==-)
{
pd.zt2=;
}
mapdata h;
h.x=pd.x;h.y=pd.y;h.zt1=pd.zt1;h.zt2=pd.zt2;
int zzt=h.count();
int vvv=;
for(int k=;k<has[zzt].size();k++)
{
if(has[zzt][k]==h)
{
vvv=;
break;
}
}
if(vvv)
continue;
has[zzt].push_back(h);
team.push(pd);
}
}
printf("Case #%d:\n",ii);
cout<<ans<<endl;
}
int main()
{
int t;
cin>>t;
while(t--)
{
ii++;
deal();
}
return ;
}
今夕何夕

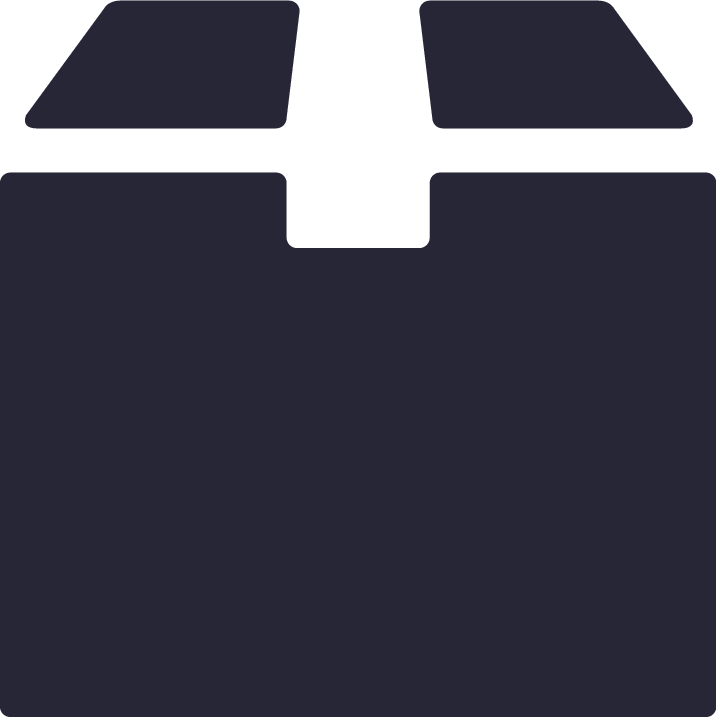
Problem Description
今天是2017年8月6日,农历闰六月十五。
小度独自凭栏,望着一轮圆月,发出了“今夕何夕,见此良人”的寂寞感慨。
为了排遣郁结,它决定思考一个数学问题:接下来最近的哪一年里的同一个日子,和今天的星期数一样?比如今天是8月6日,星期日。下一个也是星期日的8月6日发生在2023年。
小贴士:在公历中,能被4整除但不能被100整除,或能被400整除的年份即为闰年。
Input
第一行为T,表示输入数据组数。
每组数据包含一个日期,格式为YYYY-MM-DD。
1 ≤ T ≤ 10000
YYYY ≥ 2017
日期一定是个合法的日期
Output
对每组数据输出答案年份,题目保证答案不会超过四位数。
Sample Input
3
2017-08-06
2017-08-07
2018-01-01
Sample Output
2023
2023
2024
Code
暴力枚举
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cmath>
#include<algorithm> using namespace std; typedef long long ll; bool isrun(int year)
{
if (year%==&&year%!=||year%==)
return true;
return false;
} int a[][][];
int month[]={,,,,,,,,,,,,}; int main()
{
memset(a,-,sizeof(a));
int p=;
for(int year=;year<=;year++)
{
bool run=isrun(year);
for(int m=;m<=;m++)
{
if(run&&m==)
{
for(int d=;d<=month[];d++)
{
a[year][m][d]=p;
p++;
p%=;
}
continue;
}
for(int d=;d<=month[m];d++)
{
a[year][m][d]=p;
p++;
p%=;
}
}
}
int t;
scanf("%d",&t);
while (t--)
{
int y,m,d;
scanf("%d-%d-%d",&y,&m,&d);
for(int i=y+;i<=;i++)
{
if(a[i][m][d]==a[y][m][d])
{
printf("%d\n",i);
break;
}
}
}
return ;
}
度度熊的01世界

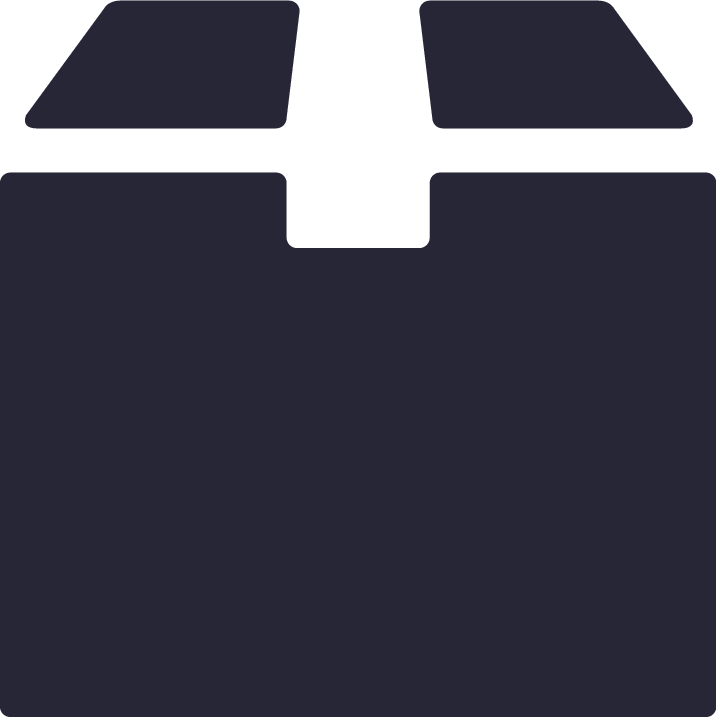
Problem Description
度度熊是一个喜欢计算机的孩子,在计算机的世界中,所有事物实际上都只由0和1组成。
现在给你一个n*m的图像,你需要分辨他究竟是0,还是1,或者两者均不是。
图像0的定义:存在1字符且1字符只能是由一个连通块组成,存在且仅存在一个由0字符组成的连通块完全被1所包围。
图像1的定义:存在1字符且1字符只能是由一个连通块组成,不存在任何0字符组成的连通块被1所完全包围。
连通的含义是,只要连续两个方块有公共边,就看做是连通。
完全包围的意思是,该连通块不与边界相接触。
Input
本题包含若干组测试数据。 每组测试数据包含: 第一行两个整数n,m表示图像的长与宽。 接下来n行m列将会是只有01组成的字符画。
满足1<=n,m<=100
Output
如果这个图是1的话,输出1;如果是0的话,输出0,都不是输出-1。
Sample Input
View Sample Input
Sample Output
0
1
-1
Code
BFS判连通包含数量
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cmath>
#include<algorithm>
#include<queue> #define ms(x,y) memset(x,y,sizeof(x)) using namespace std; typedef long long ll;
const int dx[]={,,,-},dy[]={,,-,}; struct node
{
int x, y;
node(int p,int q)
{
x=p;
y=q;
}
}; int n,m;
char a[][];
bool book[][]; int bfs(int x,int y,char c)
{
int bao=;
queue<node> que;
que.push(node(x,y));
book[x][y]=;
while(que.size())
{
node p=que.front();
que.pop();
for (int i=;i<;i++)
{
int px,py;
px=p.x+dx[i];
py=p.y+dy[i];
if(px>=&&px<n&&py>=&&py<m)
{
if(!book[px][py]&&a[px][py]==c)
{
book[px][py]=;
que.push(node(px,py));
}
}
else
bao=;
}
}
return bao;
}
int main()
{
while(~scanf("%d%d",&n,&m))
{
ms(book,);
ms(a,);
for(int i=;i<n;i++)
{
for(int j=;j<m;j++)
{
scanf(" %c",&a[i][j]);
}
}
int num0=,num1=;
int bao1=,bao0=;
for(int i=;i<n;i++)
{
for(int j=;j<m;j++)
{
if(!book[i][j])
{
if(a[i][j]=='')
{
num0++;
bao1+=bfs(i,j,'');
}
else
{
num1++;
bao0+=bfs(i,j,'');
}
}
}
}
if(num1==&&bao1==)
{
printf("1\n");
}
else if(num1==&&bao1==)
{
printf("0\n");
}
else
printf("-1\n");
}
return ;
}
Time: 2017-08-12
[SinGuLaRiTy] 2017 百度之星程序设计大赛 初赛A的更多相关文章
- [SinGuLaRiTy] 2017 百度之星程序设计大赛 初赛B
[SinGuLaRiTy-1037] Copyright (c) SinGuLaRiTy 2017. All Rights Reserved. Chess Time Limit: 2000/1000 ...
- HDU 6118 度度熊的交易计划 【最小费用最大流】 (2017"百度之星"程序设计大赛 - 初赛(B))
度度熊的交易计划 Time Limit: 12000/6000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total S ...
- HDU 6119 小小粉丝度度熊 【预处理+尺取法】(2017"百度之星"程序设计大赛 - 初赛(B))
小小粉丝度度熊 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Sub ...
- HDU 6114 Chess 【组合数】(2017"百度之星"程序设计大赛 - 初赛(B))
Chess Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submi ...
- HDU 6109 数据分割 【并查集+set】 (2017"百度之星"程序设计大赛 - 初赛(A))
数据分割 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submis ...
- HDU 6108 小C的倍数问题 【数学】 (2017"百度之星"程序设计大赛 - 初赛(A))
小C的倍数问题 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Sub ...
- HDU 6122 今夕何夕 【数学公式】 (2017"百度之星"程序设计大赛 - 初赛(A))
今夕何夕 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submis ...
- HDU 6113 度度熊的01世界 【DFS】(2017"百度之星"程序设计大赛 - 初赛(A))
度度熊的01世界 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- 2017"百度之星"程序设计大赛 - 初赛(A) [ hdu 6108 小C的倍数问题 ] [ hdu 6109 数据分割 ] [ hdu 6110 路径交 ] [ hdu 6112 今夕何夕 ] [ hdu 6113 度度熊的01世界 ]
这套题体验极差. PROBLEM 1001 - 小C的倍数问题 题 OvO http://acm.hdu.edu.cn/showproblem.php?pid=6108 (2017"百度之星 ...
随机推荐
- 从request获取各种路径总结 request.getRealPath("url")
转载:http://blog.csdn.net/piaoxuan1987/article/details/8541839 equest.getRealPath() 这个方法已经不推荐使用了,代替方法是 ...
- Linux - 创建用户的相关文件
创建一个用户会与 6 个文件相关 /etc/passwd 储存了所有用户的相关信息 第一行中,从左往右 root 为用户名,: 为分隔符,x 为密码,0 为 uid,0 为 gid,root 为用户的 ...
- ctf中检测和分离隐藏的文件
使用binwalk检测是否隐藏了文件 root@sch01ar:~# binwalk '/root/桌面/test.jpg' 还藏了一个zip文件,接下来用foremost来分离文件 root@sch ...
- IDEA实用的第三方插件和工具介绍设置
一:grep console grep-console插件可以让idea显示多颜色调试日志,使Log4j配置输出的不同级别error warn info debug fatal显示不同颜色 开发起来区 ...
- django 基于正则表达式的url
方式一: urls.py from mytest import views urlpatterns = [ url(r'^index-(\d+)-(\d+).html', views.Index.as ...
- ubuntu12上部署Django1.8.4+uwsgi+nginx超级详细流程配置到云服务器
环境: 系统:ubuntu12,系统自带默认有python2.7 框架:Django1.8.4,需要python2.7以上才能支持 前言: 用户浏览器发送http请求->nginx(静态文件 ...
- Python绘图matplotlib
转自http://blog.csdn.net/ywjun0919/article/details/8692018 Python图表绘制:matplotlib绘图库入门 matplotlib 是pyth ...
- Subscript & Inheritance
[Subscript] 1.subscript的定义: 2.Subscript的使用: 3.可以定义多维subscript: 多维Subscript的使用: [Inheritance] 1.overr ...
- linux tcpdump
简介 用简单的话来定义tcpdump,就是:dump the traffic on a network,根据使用者的定义对网络上的数据包进行截获的包分析工具. tcpdump可以将网络中传送的数据包的 ...
- 用Eclipse Memory Analyzer查找内存泄露
写在CSDN里面了 http://blog.csdn.net/dayulxl/article/details/78164301