How to Export to Excel
https://simpleisbetterthancomplex.com/tutorial/2016/07/29/how-to-export-to-excel.html
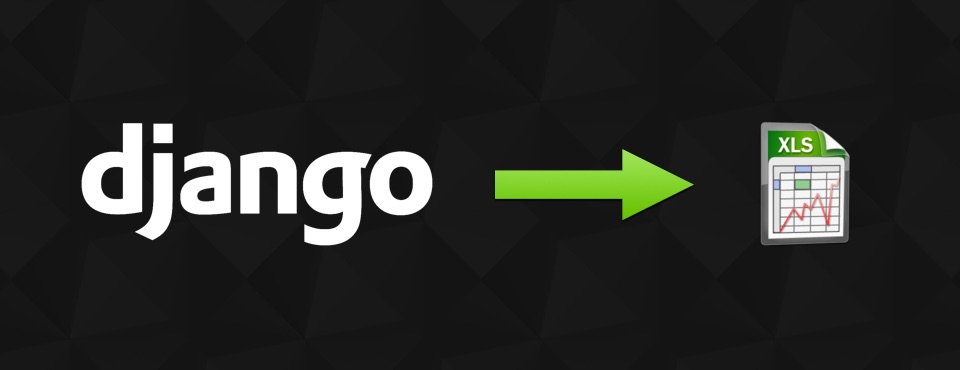
Export data to excel is a common requirement on many web applications. Python makes everything easier. But, nevertheless, it is the kind of task I need to look for references whenever I have to implement.
I will give you two options in this tutorial: (1) export data to a .csv file using Python’s csv module; (2) export data to a .xls file using a third-party module named xlwt.
For both cases, consider we are exporting django.contrib.auth.models.User
data.
Export Data to CSV File
Easiest way. No need to install dependencies, which is a great thing. Use it if you do not need any fancy formating.
views.py
import csv
from django.http import HttpResponse
from django.contrib.auth.models import User
def export_users_csv(request):
response = HttpResponse(content_type='text/csv')
response['Content-Disposition'] = 'attachment; filename="users.csv"'
writer = csv.writer(response)
writer.writerow(['Username', 'First name', 'Last name', 'Email address'])
users = User.objects.all().values_list('username', 'first_name', 'last_name', 'email')
for user in users:
writer.writerow(user)
return response
urls.py
import views
urlpatterns = [
...
url(r'^export/csv/$', views.export_users_csv, name='export_users_csv'),
]
template.html
<a href="{% url 'export_users_csv' %}">Export all users</a>
In the example above I used the values_list
in the QuerySet for two reasons: First to query only the fields I needed to export, and second because it will return the data in a tuple instead of model instances. The writerow
method expects a list/tuple.
Another way to do it would be:
writer.writerow([user.username, user.first_name, user.last_name, user.email, ])
Export Data to XLS File
Use it if you really need to export to a .xls file. You will be able to add formating as bold font, font size, define column size, etc.
First of all, install the xlwt module. The easiest way is to use pip.
pip install xlwt
views.py
import xlwt
from django.http import HttpResponse
from django.contrib.auth.models import User
def export_users_xls(request):
response = HttpResponse(content_type='application/ms-excel')
response['Content-Disposition'] = 'attachment; filename="users.xls"'
wb = xlwt.Workbook(encoding='utf-8')
ws = wb.add_sheet('Users')
# Sheet header, first row
row_num = 0
font_style = xlwt.XFStyle()
font_style.font.bold = True
columns = ['Username', 'First name', 'Last name', 'Email address', ]
for col_num in range(len(columns)):
ws.write(row_num, col_num, columns[col_num], font_style)
# Sheet body, remaining rows
font_style = xlwt.XFStyle()
rows = User.objects.all().values_list('username', 'first_name', 'last_name', 'email')
for row in rows:
row_num += 1
for col_num in range(len(row)):
ws.write(row_num, col_num, row[col_num], font_style)
wb.save(response)
return response
urls.py
import views
urlpatterns = [
...
url(r'^export/xls/$', views.export_users_xls, name='export_users_xls'),
]
template.html
<a href="{% url 'export_users_xls' %}">Export all users</a>
Learn more about the xlwt module reading its official documentation.
How to Export to Excel的更多相关文章
- export to excel
using NPOI.HSSF.UserModel; using NPOI.SS.UserModel; using NPOI.XSSF.UserModel;npoi是重点. 定义一个exporttoe ...
- [Stephen]Export from Excel to ALM
1.根据当前安装的ALM版本和Excel版本到https://hpln.hp.com/page/alm-excel-addin-page中对应的插件进行下载安装,安装时Excel需要关闭.安装成功后, ...
- 进阶-案例九: WD中实现export 到Excel,Doc,Txt.
1.导出excel 文件代码 导出事件代码: METHOD onactionimport . *导出excel: DATA: lo_node TYPE REF TO if_wd_context_nod ...
- 笔记11 export to excel
参考两篇博客:http://blog.csdn.net/zyming0815/article/details/5939104 http://blog.csdn.net/g710710/article/ ...
- We refined export to Excel for SharePoint
http://sysmagazine.com/posts/208948/ http://sharepointwikipedia.blogspot.kr/2013/05/export-to-spread ...
- csharp: DataTable export to excel,word,csv etc
http://code.msdn.microsoft.com/office/Export-GridView-to-07c9f836 https://exporter.codeplex.com/ htt ...
- 笔记12 export to excel (NPOI)
1:filestream 熟悉关于文件操作 ==>fs.Seek(0, SeekOrigin.Begin);//每次打开文件, ==>若果重写覆盖的话,必须先清空 fs.SetLength ...
- javascript export excel
<input type="button" onclick="tableToExcel('tablename', 'name')" value=" ...
- [Rodbourn's Blog]How to export Excel plots to a vector image (EPS, EMF, SVG, etc.)
This is a bit of a workaround, but it's the only way I know of to export an Excel plot into a vector ...
随机推荐
- 预期结果 参数化parametrize
1.pytest.mark.parametrize装饰器可以实现测试用例参数化. 2.实例: import pytest @pytest.mark.parametrize("req,expe ...
- leetcode第15题:三数之和
给定一个包含 n 个整数的数组 nums,判断 nums 中是否存在三个元素 a,b,c ,使得 a + b + c = 0 ?找出所有满足条件且不重复的三元组. 注意:答案中不可以包含重复的三元组. ...
- 团队-爬取豆瓣电影TOP250-需求分析
需求: 1.搜集相关电影网址 2.实现相关逻辑的代码 项目步骤: 1.通过豆瓣网搜索关键字,获取相关地址 2.根据第三方包实现相关逻辑
- django中scrf的实现机制
第一步:django第一次响应来自某个客户端的请求时,后端随机产生一个token值,把这个token保存在SESSION状态中,后端把这个token放到cookie中交给前端页面. 第二步:下次前端需 ...
- Tomcat jdk 项目搭建问题
Tomcat 出现log4j未找到 是因为缺少servlet包 出现版本1.5及更高错误 是Java Compiler的版本错误 需重新导包Installed JRES.
- Java学习笔记13(equals()方法;toString()方法)
equals()方法: equals方法是Object类中的方法:Object是所有类的祖宗,所以所有类都有equals()方法: boolean equals(Object obj); equals ...
- Python 验证进程之间是空间隔离的
from multiprocessing import Process num = 100 def f1(): global num num = 3 print("子进程中的num" ...
- 部署php的正确姿势
1. 更新源 apt-get update 2.安装apache apt-get install apache2 ubuntu下apache2虚拟主机配置 cd /etc/apache2/sites- ...
- file类和io流
一.file类 file类是一个可以用其对象表示目录或文件的一个Java.io包中的类 import java.io.File; import java.io.IOException; public ...
- Kafka高可用实现原理
数据存储格式 Kafka的高可靠性的保障来源于其健壮的副本(replication)策略.一个Topic可以分成多个Partition,而一个Partition物理上由多个Segment组成. Seg ...