hdu 3345 War Chess
War Chess
Time Limit : 2000/1000ms (Java/Other) Memory Limit : 32768/32768K (Java/Other)
Total Submission(s) : 5 Accepted Submission(s) : 3
Font: Times New Roman | Verdana | Georgia
Font Size: ← →
Problem Description
In this game, there is an N * M battle map, and every player has his own Moving Val (MV). In each round, every player can move in four directions as long as he has enough MV. To simplify the problem, you are given your position and asked to output which grids you can arrive.
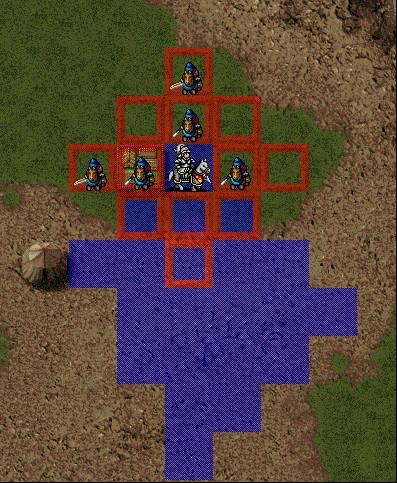
In the map:
'Y' is your current position (there is one and only one Y in the given map).
'.' is a normal grid. It costs you 1 MV to enter in this gird.
'T' is a tree. It costs you 2 MV to enter in this gird.
'R' is a river. It costs you 3 MV to enter in this gird.
'#' is an obstacle. You can never enter in this gird.
'E's are your enemies. You cannot move across your enemy, because once you enter the grids which are adjacent with 'E', you will lose all your MV. Here “adjacent” means two grids share a common edge.
'P's are your partners. You can move across your partner, but you cannot stay in the same grid with him final, because there can only be one person in one grid.You can assume the Ps must stand on '.' . so ,it also costs you 1 MV to enter this grid.
Input
Then T cases follow:
Each test case starts with a line contains three numbers N,M and MV (2<= N , M <=100,0<=MV<= 65536) which indicate the size of the map and Y's MV.Then a N*M two-dimensional array follows, which describe the whole map.
Output
Sample Input
5
3 3 100
...
.E.
..Y 5 6 4
......
....PR
..E.PY
...ETT
....TT 2 2 100
.E
EY 5 5 2
.....
..P..
.PYP.
..P..
..... 3 3 1
.E.
EYE
...
Sample Output
...
.E*
.*Y ...***
..**P*
..E*PY
...E**
....T* .E
EY ..*..
.*P*.
*PYP*
.*P*.
..*.. .E.
EYE
.*. 自己写的spfa代码:
注意输出的每行后面都有一个空行,我就错了presentation error。。。
#include <iostream>
#include<cstdio>
#include<cstring>
#include<queue>
using namespace std; struct node
{
int x,y;
node(int a,int b){x=a; y=b;}
};
int dr[][]={{,},{,},{-,},{,-}};
int n,m,mv,t,sx,sy;
int dis[][];
bool vis[][];
char mp[][]; bool work(int x,int y)
{
if(mp[x][y]=='Y') return ;
for(int i=;i<;i++)
{
int xx=x+dr[i][];
int yy=y+dr[i][];
if(xx< || xx>=n || yy< || yy>=m) continue;
if (mp[xx][yy]=='E') return ;
}
return ;
}
void spfa()
{
queue<node> Q;
memset(dis,-,sizeof(dis));
memset(vis,,sizeof(vis));
Q.push(node(sx,sy));
dis[sx][sy]=mv;
vis[sx][sy]=;
while(!Q.empty())
{
node p=Q.front();
vis[p.x][p.y]=;
Q.pop();
if (work(p.x,p.y)) dis[p.x][p.y]=;
if (dis[p.x][p.y]>)
for(int i=;i<;i++)
{
int xx=p.x+dr[i][];
int yy=p.y+dr[i][];
char ch=mp[xx][yy];
if(xx< || xx>=n || yy< || yy>=m) continue;
if(ch=='#') continue;
if(ch=='.'|| ch=='T' || ch=='R')
{
int k;
if (ch=='.') k=; else
if (ch=='T') k=; else
if (ch=='R') k=;
if (dis[xx][yy]>=dis[p.x][p.y]-k) continue;
dis[xx][yy]=dis[p.x][p.y]-k;
if(!vis[xx][yy])
{
Q.push(node(xx,yy));
vis[xx][yy]=;
}
}
if(ch=='P' && dis[p.x][p.y]>)
{
if (dis[xx][yy]>=dis[p.x][p.y]-) continue;
dis[xx][yy]=dis[p.x][p.y]-;
if(!vis[xx][yy])
{
Q.push(node(xx,yy));
vis[xx][yy]=;
}
}
}
}
}
int main()
{
scanf("%d",&t);
for(;t>;t--)
{
scanf("%d%d%d",&n,&m,&mv);
for(int i=;i<n;i++)
{
scanf("%s",&mp[i]);
for(int j=;j<m;j++)
if (mp[i][j]=='Y') sx=i,sy=j;
} spfa();
for(int i=;i<n;i++)
{
for(int j=;j<m;j++)
{
if (dis[i][j]<) printf("%c",mp[i][j]);
else
{
if (mp[i][j]=='E' || mp[i][j]=='P' || mp[i][j]=='Y') printf("%c",mp[i][j]);
else printf("*");
}
}
printf("\n");
}
printf("\n");
}
return ;
}
转自:http://www.bkjia.com/cjjc/857812.html
/*
bfs+优先队列,刚开始没有优化,果断超时,第二次竟然因为优先级符号TLE!!(该记得的东西真得记牢) 使用mark数组记录该点MV值大小,初始化为零,搜索时只有当从某个点到达当前点使MV变大时才把该点值更新;入队时判断该点MV值是否大于零,大于则入队。 具体看代码:
*/
#include"stdio.h"
#include"string.h"
#include"queue"
#include"vector"
#include"algorithm"
using namespace std;
#define N 105
#define max(a,b) (a>b?a:b)
int mark[N][N],n,m,v;
int dir[][]={,,,-,-,,,};
char str[N][N];
struct node
{
int x,y,d;
friend bool operator<(node a,node b)
{
return a.d=&&x=&&yq;
node cur,next;
cur.x=x;cur.y=y;cur.d=v;
q.push(cur);
memset(mark,-,sizeof(mark));
mark[x][y]=v;
while(!q.empty())
{
cur=q.top();
q.pop();
for(i=;i<;i++)
{
next.x=x=dir[i][]+cur.x;
next.y=y=dir[i][]+cur.y;
if(judge(x,y))
{
if(str[x][y]=='.'||str[x][y]=='P')
t=cur.d-;
else if(str[x][y]=='T')
t=cur.d-;
else if(str[x][y]=='R')
t=cur.d-;
else
t=-;
if(ok(x,y)&&t>)
t=; //战斗力减为0
if(t>&&t>mark[x][y])
{
next.d=t;
q.push(next);
}
mark[x][y]=max(mark[x][y],t);
}
}
}
}
int main()
{
int T,i,j;
scanf("%d",&T);
while(T--)
{
scanf("%d%d%d",&n,&m,&v);
for(i=;i=)
{
if(str[i][j]!='P'&&str[i][j]!='Y')
printf("*");
else
printf("%c",str[i][j]);
}
else
printf("%c",str[i][j]);
}
puts("");
}
puts("");
}
return ;
}
hdu 3345 War Chess的更多相关文章
- HDU - 3345 War Chess 广搜+优先队列
War chess is hh's favorite game: In this game, there is an N * M battle map, and every player has hi ...
- 【HDOJ】3345 War Chess
简单BFS.注意最后一组数据,每个初始点不考虑周围是否有敌人. /* 3345 */ #include <iostream> #include <cstdio> #includ ...
- War Chess (hdu 3345)
http://acm.hdu.edu.cn/showproblem.php?pid=3345 Problem Description War chess is hh's favorite game:I ...
- hihoCoder 1392 War Chess 【模拟】 (ACM-ICPC国际大学生程序设计竞赛北京赛区(2016)网络赛)
#1392 : War Chess 时间限制:1000ms 单点时限:1000ms 内存限制:256MB 描述 Rainbow loves to play kinds of War Chess gam ...
- War Chess bfs+优先队列
War chess is hh's favorite game: In this game, there is an N * M battle map, and every player has hi ...
- HDU 5724:Chess(博弈 + 状压)
http://acm.hdu.edu.cn/showproblem.php?pid=5724 Chess Problem Description Alice and Bob are playing ...
- HDU 4405 Aeroplane chess 期望dp
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=4405 Aeroplane chess Time Limit: 2000/1000 MS (Java/ ...
- HDU 3345
http://acm.hdu.edu.cn/showproblem.php?pid=3345 最近重写usaco压力好大,每天写的都想吐.. 水一道bfs 注意的是开始旁边有敌人可以随便走,但是一旦开 ...
- HDU 4405 Aeroplane chess 概率DP 难度:0
http://acm.hdu.edu.cn/showproblem.php?pid=4405 明显,有飞机的时候不需要考虑骰子,一定是乘飞机更优 设E[i]为分数为i时还需要走的步数期望,j为某个可能 ...
随机推荐
- webapi中的扩展点
Extension Points Web API provides extension points for some parts of the routing process. Interface ...
- 我所使用的Linux软件集合
自从2003-2004春节之际初次尝试使用Linux以来,至今已十年有余了.尤其是整个博士研究期间,坚持在Linux下开展学习与研究工作,前前后后试用了不少桌面环境.窗口管理器.终端程序以及其他应用软 ...
- LeetCode OJ 59. Spiral Matrix II
Given an integer n, generate a square matrix filled with elements from 1 to n2 in spiral order. For ...
- xampp 搭建 web mac上
1.安装 2.修改数据库密码,删除phpmyadmin ,用navicat 控制数据库 3.修改/Applications/XAMPP/xamppfiles/etc/extra/httpd-vhos ...
- Ecstore1.2启用mongodb添加索引
配置config(连接mongo) mongo define('KVSTORE_STORAGE', 'base_kvstore_mongodb'); define('MONGODB_SERVER_CO ...
- 28.按要求编写一个Java应用程序: (1)定义一个类,描述一个矩形,包含有长、宽两种属性,和计算面积方法。 (2)编写一个类,继承自矩形类,同时该类描述长方体,具有长、宽、高属性, 和计算体积的方法。 (3)编写一个测试类,对以上两个类进行测试,创建一个长方体,定义其长、 宽、高,输出其底面积和体积。
//矩形父类 package d922A; public class Rect { private double l,w; Rect(double c,double k) { l=c; w=k; } ...
- 【开发笔记】Spring的配置文件
factory-method竟然写成了destroy-method,害得我运行报错,找原因找了几分钟. 原来我按alt+/来提示代码的时候,有许多可选项,可能一时眼花选错了
- COM与.NET程序集导出和部署COM组件
为了分布式和多客户端调用我们还需要将写好的COM组件发布到一台服务器上.这里我们将组件部署到操作系统的COM+应用程序中去.如果没此需要就可以导出后,在C++环境中调用COM了. 第一步:导出COM组 ...
- 【java学习】Servlet简单的表单程序(一)
此文用于java学习,在此小记. 在此小Demo中使用到了Servlet,所以有必要了解一下Servlet的相关知识.(Servlet的相关知识摘抄自http://blog.csdn.net/jiuq ...
- Centos6.6升级python版本
centos原生python为2.6.6,可以通过下面的命令查看 #python -V Python 注:在安装新版本前,请先安装zlib\openssl组件,如果你确认你用不到这个,也可以不装 需要 ...