vue 图片放大镜效果
插件名称:vue-photo-zoom-pro
https://github.com/Mater1996/vue-photo-zoom-pro
效果图
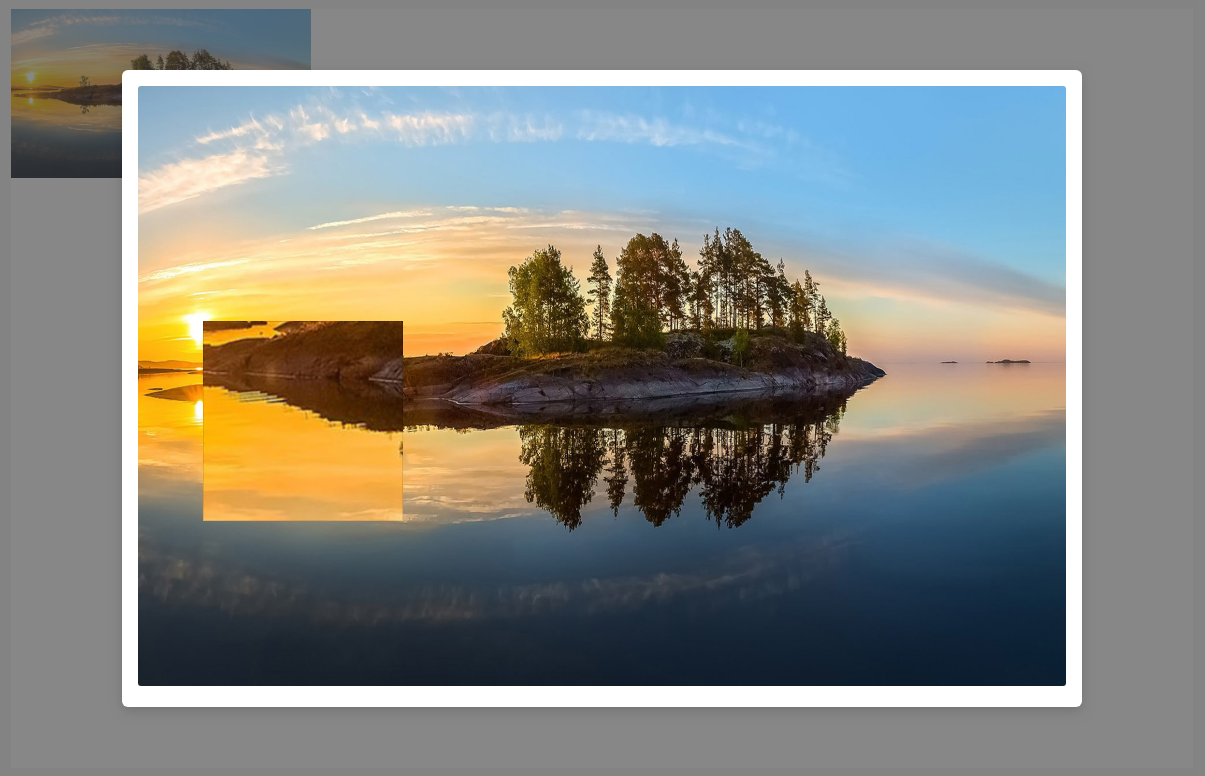
使用:
<template>
<div class="images">
<img style="width: 300px;" @click.stop="showBigPicture(url)" :src="url" alt=""> <!-- 显示大图 -->
<Modal v-model="pictureModal" width="960" footer-hide :closable="closable" class-name="vertical-center-modal">
<photo-zoom :url="bigPictureUrl" :bigWidth="200" :scale="2"
overlayStyle="width: 100%;height: 600px;border-radius: 3px;"></photo-zoom>
</Modal>
</div>
</template>
<script>
import PhotoZoom from '@/components/photo-zoom'
export default {
components: { PhotoZoom },
data() {
return {
url:'http://pic1.win4000.com/wallpaper/2019-01-28/5c4ebd381fc8b.jpg',
bigPictureUrl:'',
pictureModal: false,
closable: false,
}
},
methods: {
showBigPicture(url) {
this.pictureModal = true;
this.bigPictureUrl = url;
},
},
}
</script>
代码中使用了iviewUI的Modal,
组件内容:
index.vue:
<template>
<div style="width: 100%;height: 100%">
<vue-photo-zoom-pro :width="bigWidth" :url="url" :type="type" :scale="scale" :out-show="showType"
:overlayStyle="overlayStyle">
</vue-photo-zoom-pro>
</div>
</template> <script>
import vuePhotoZoomPro from '@/components/photo-zoom/vue-photo-zoom-pro'
export default {
name: 'PicZoom',
components: { vuePhotoZoomPro },
data() {
return {
type: "square",
showType: false,
}
},
props: {
url: {
type: String,
required: true,
// default: require('@/assets/vehicle_img/blank_vehicle.jpg')
},
bigWidth: {
type: Number,
required: true,
default: 168
},
scale: {
type: Number,
required: true,
default: 2
},
overlayStyle: {
type: String,
default: 'width:100%;height:100%'
}
},
}
</script>
vue-photo-zoom-pro.vue:
<template>
<div class="pic-img">
<div class="img-container">
<img ref="img" @load="imgLoaded" :src="url" :style="overlayStyle" @error="imgerrorfun" />
<div class="overlay" @mousemove.stop="!moveEvent && mouseMove($event)"
@mouseout.stop="!leaveEvent && mouseLeave($event)" :style="overlayStyle">
</div>
<div v-if="!hideZoom && imgLoadedFlag &&!hideSelector" :class="['img-selector', {'circle': type === 'circle'}]"
:style="[imgSelectorStyle, imgSelectorSize, imgSelectorPosition, !outShow && imgBg, !outShow && imgBgSize, !outShow && imgBgPosition]">
<slot></slot>
</div>
<div v-if="outShow" v-show="!hideOutShow" :class="['img-out-show', {'base-line': baseline}]"
:style="[imgOutShowSize, imgOutShowPosition, imgBg, imgBgSize, imgBgPosition]">
<div v-if="pointer" class="img-selector-point"></div>
</div>
</div>
</div> </template> <script>
export default {
name: "vue-photo-zoom-pro",
props: {
url: {
type: String,
},
highUrl: String,
width: {
type: Number,
default: 168
},
type: {
type: String,
default: "square",
validator: function (value) {
return ["circle", "square"].indexOf(value) !== -1;
}
},
selectorStyle: {
type: Object,
default() {
return {};
}
},
outShowStyle: {},
scale: {
type: Number,
default: 3
},
moveEvent: {
type: [Object, MouseEvent],
default: null
},
leaveEvent: {
type: [Object, MouseEvent],
default: null
},
hideZoom: {
type: Boolean,
default: false
},
outShow: {
type: Boolean,
default: false
},
pointer: {
type: Boolean,
default: false
},
baseline: {
type: Boolean,
default: false
},
overlayStyle: {
type: String,
default: 'width:100%;height:100%'
},
},
data() {
return {
selector: {
width: this.width,
top: 0,
left: 0,
bgTop: 0,
bgLeft: 0,
rightBound: 0,
bottomBound: 0,
absoluteLeft: 0,
absoluteTop: 0
},
imgInfo: {},
$img: null,
screenWidth: document.body.clientWidth,
outShowInitTop: 0,
outShowTop: 0,
hideOutShow: true,
imgLoadedFlag: false,
hideSelector: false,
timer: null
};
},
watch: {
moveEvent(e) {
this.mouseMove(e);
},
leaveEvent(e) {
this.mouseLeave(e);
},
url(n) {
this.imgLoadedFlag = false;
// let img = require('@/assets/vehicle_img/blank_vehicle.jpg')
// if(n == img){
// this.outShow = false
// }
},
width(n) {
this.initSelectorProperty(n);
},
screenWidth(val) {
if (!this.timer) {
this.screenWidth = val;
this.timer = setTimeout(() => {
this.imgLoaded();
clearTimeout(this.timer);
this.timer = null;
}, 400);
}
}
},
computed: {
addWidth() {
return !this.outShow ? (this.width / 2) * (1 - this.scale) : 0;
},
imgSelectorPosition() {
let { top, left } = this.selector;
return {
top: `${top}px`,
left: `${left}px`
};
},
imgSelectorSize() {
let width = this.selector.width;
return {
width: `${width}px`,
height: `${width}px`
};
},
imgSelectorStyle() {
return this.selectorStyle;
},
imgOutShowSize() {
let {
scale,
selector: { width }
} = this;
return {
width: `${width * scale}px`,
height: `${width * scale}px`
};
},
imgOutShowPosition() {
return {
top: `${this.outShowTop}px`,
right: `${-8}px`
};
},
imgBg() {
return {
backgroundImage: `url(${this.highUrl || this.url})`
};
},
imgBgSize() {
let {
scale,
imgInfo: { height, width }
} = this;
return {
backgroundSize: `${width * scale}px ${height * scale}px`
};
},
imgBgPosition() {
let { bgLeft, bgTop } = this.selector;
return {
backgroundPosition: `${bgLeft}px ${bgTop}px`
};
},
},
mounted() {
this.$img = this.$refs["img"];
},
methods: {
imgLoaded() {
let imgInfo = this.$img.getBoundingClientRect();
if (JSON.stringify(this.imgInfo) != JSON.stringify(imgInfo)) {
this.imgInfo = imgInfo;
this.initSelectorProperty(this.width);
this.resetOutShowInitPosition();
}
if (!this.imgLoadedFlag) {
this.imgLoadedFlag = true;
this.$emit("created", imgInfo);
}
},
mouseMove(e) {
if (!this.hideZoom && this.imgLoadedFlag) {
this.imgLoaded();
const { pageX, pageY, clientY } = e;
const { scale, selector, outShow, addWidth, outShowAutoScroll } = this;
let { outShowInitTop } = this;
const scrollTop = pageY - clientY;
const { absoluteLeft, absoluteTop, rightBound, bottomBound } = selector;
const x = pageX - absoluteLeft; // 选择器的x坐标 相对于图片
const y = pageY - absoluteTop; // 选择器的y坐标
if (outShow) {
if (!outShowInitTop) {
outShowInitTop = this.outShowInitTop = scrollTop + this.imgInfo.top;
}
this.hideOutShow && (this.hideOutShow = false);
this.outShowTop =
scrollTop > outShowInitTop ? scrollTop - outShowInitTop : 0;
}
this.hideSelector && (this.hideSelector = false);
selector.top = y > 0 ? (y < bottomBound ? y : bottomBound) : 0;
selector.left = x > 0 ? (x < rightBound ? x : rightBound) : 0;
selector.bgLeft = addWidth - x * scale; // 选择器图片的坐标位置
selector.bgTop = addWidth - y * scale;
}
},
initSelectorProperty(selectorWidth) {
const selectorHalfWidth = selectorWidth / 2;
const selector = this.selector;
const { width, height, left, top } = this.imgInfo;
const { scrollLeft, scrollTop } = document.documentElement;
selector.width = selectorWidth;
selector.rightBound = width - selectorWidth;
selector.bottomBound = height - selectorWidth;
selector.absoluteLeft = left + selectorHalfWidth + scrollLeft;
selector.absoluteTop = top + selectorHalfWidth + scrollTop;
},
mouseLeave() {
this.hideSelector = true;
if (this.outShow) {
this.hideOutShow = true;
}
},
reset() {
Object.assign(this.selector, {
top: 0,
left: 0,
bgLeft: 0,
bgTop: 0
});
this.resetOutShowInitPosition();
},
resetOutShowInitPosition() {
this.outShowInitTop = 0;
},
imgerrorfun(e) {
// let img = require('@/assets/vehicle_img/blank_vehicle.jpg')
// this.url = img
// e.target.src = img
// e.target.onerror= null
}
}
};
</script> <style scoped>
.img-container { position: relative; }
.overlay { cursor: crosshair; position: absolute; top: 0; left: 0; opacity: 0.5; z-index: 3; }
.img-selector { position: absolute; cursor: crosshair; border: 1px solid rgba(0, 0, 0, 0.1); background-repeat: no-repeat; background-color: rgba(64, 64, 64, 0.6);}
.img-selector.circle {border-radius: 50%;}
.img-out-show {z-index: 5000;position: absolute;background-repeat: no-repeat;-webkit-background-size: cover;background-color: white;transform: translate(100%, 0);}
.img-selector-point {position: absolute;width: 4px;height: 4px;top: 50%;left: 50%;transform: translate(-50%, -50%);background-color: black;}
.img-out-show.base-line::after {position: absolute;box-sizing: border-box;content: "";width: 1px;top: 0;bottom: 0;left: 50%;transform: translateX(-50%);}
.img-out-show.base-line::before {position: absolute;box-sizing: border-box;content: "";height: 1px;border: 1px dashed rgba(0, 0, 0, 0.36);left: 0;right: 0;top: 50%;transform: translateY(-50%);}
</style>
vue 图片放大镜效果的更多相关文章
- vue图片放大镜效果
原作者地址:https://github.com/lemontree2000/vue-magnify 经测试,原插件在使用时有bug,即在预览时进行鼠标滚动,导致遮罩层计算错误.我已修复该bug,特分 ...
- Magnifier.js - 支持鼠标滚轮缩放的图片放大镜效果
Magnifier.js 是一个 JavaScript 库,能够帮助你在图像上实现放大镜效果,支持使用鼠标滚轮放大/缩小功能.放大的图像可以显示在镜头本身或它的外部容器中.Magnifier.js 使 ...
- WPF设置VistualBrush的Visual属性制作图片放大镜效果
原文:WPF设置VistualBrush的Visual属性制作图片放大镜效果 效果图片:原理:设置VistualBrush的Visual属性,利用它的Viewbox属性进行缩放. XAML代码:// ...
- 原生javascript实现图片放大镜效果
当我们在电商网站上购买商品时,经常会看到这样一种效果,当我们把鼠标放到我们浏览的商品图片上时,会出现类似放大镜一样的一定区域的放大效果,方便消费者观察商品.今天我对这一技术,进行简单实现,实现图片放大 ...
- 【Demo】jQuery 图片放大镜效果——模仿淘宝图片放大效果
实现功能: 模仿淘宝图片放大效果,鼠标移动到小图片的某一处,放大镜对应显示大图片的相应位置. 实现效果: 实现代码: <!DOCTYPE html> <html> <he ...
- javascript图片放大镜效果展示
javascript图片放大镜效果展示 <!DOCTYPE html> <html> <head lang="en"> <meta cha ...
- canvas知识02:图片放大镜效果
效果截图: JS代码: <script> // 初始化canvas01和上下文环境 var cav01 = document.getElementById('cav01'); var cx ...
- jQuery实现图片放大镜效果
实现图片放大镜的原理: 给放大镜元素一个对应的html元素为<div class='right'> 设置这个div的宽高固定为某个值(350px,350px) 设置div的css为超出部分 ...
- 用css3的cursor:zoom-in/zoom-out实现微博看图片放大镜效果
1.前言 CSS3的出现解决了很多让人头疼的问题,至少我想很多童鞋都这样认为.css3的cursor属性大家用的应该是非常的多的,我想用的比较多的像cursor:pointer;cursor:help ...
随机推荐
- Jvisualvm简单使用教程
本博客介绍一下jvisualvm的简单使用教程,jvisualvm功能还是挺多的,不过本博客之简单介绍一下 1.拿线程快照信息 在jdk安装目录找到jvisualvm.exe,${JDK_HOME}\ ...
- WIN7快速打开hosts方法
WIN7快速打开hosts方法 1直接运行C:\Windows\System32\drivers\etc\hosts 浏览选择notepad++打开即可 2打开notepad++打开 C:\Windo ...
- CSS3倒影效果
比较简单的倒影效果 <pre><div class="box-reflect"><img src="https://www.baidu.co ...
- JVM的监控工具之jconsole
JConsole(Java Monitoring and Management Console)是一种基于JMX的可视化监视.管理工具.管理的是什么?管理的是监控信息.永久代的使用信息.类加载等等 如 ...
- 进程间通信的信道与控制(io机制)
进程间通信 = 信道 + 控制(状态) + io 信道: 1.流式信道: 2.队列信道: 3.共享内存信道: 控制机制: 数据就绪状态的通知与数据获取机制. 1.信号: 2.循环: 3.io机制
- 【spring】spring aop
Aspect-Oriented Programming (AOP) 一.官方介绍 通过提供另一种考虑程序结构的方式,面向方面编程(AOP)补充了面向对象编程(OOP).OOP中模块化的关键单元是类,而 ...
- 记录vue用 html5+做移动APP 用barcode做扫一扫功能时安卓 的bug(黑屏、错位等等)和解决方法
最近做项目时,要用到扫一扫二维码的功能,在html5+里面有提供barcode功能,于是照过来用了, 写的代码如下 : 扫码页面: <style lang="less" sc ...
- python关于 微型微服务框架bottle实践
代码实践 资源接口类MyWeb.py,定义了资源接口,代码时python2的代码,和3语法略有不同! # coding: utf-8 import json import logging import ...
- maven 学习---使用“mvn site-deploy”部署站点
这里有一个指南,向您展示如何使用“mvn site:deploy”来自动部署生成的文档站点到服务器,这里通过WebDAV机制说明. P.S 在这篇文章中,我们使用的是Apache服务器2.x的WebD ...
- Unity导出Gradle工程给Android Studio使用
1 Unity导出Gradle项目 Unity打包时Build System选择Gradle,勾选Export Project 2 Android Studio导入Unity导出的Gradle项目 打 ...