[iOS基础控件 - 6.9] 聊天界面Demo
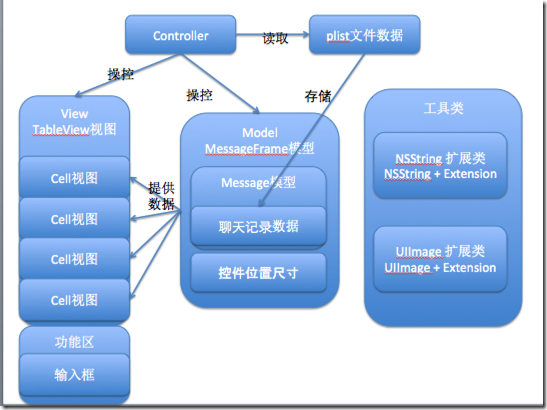
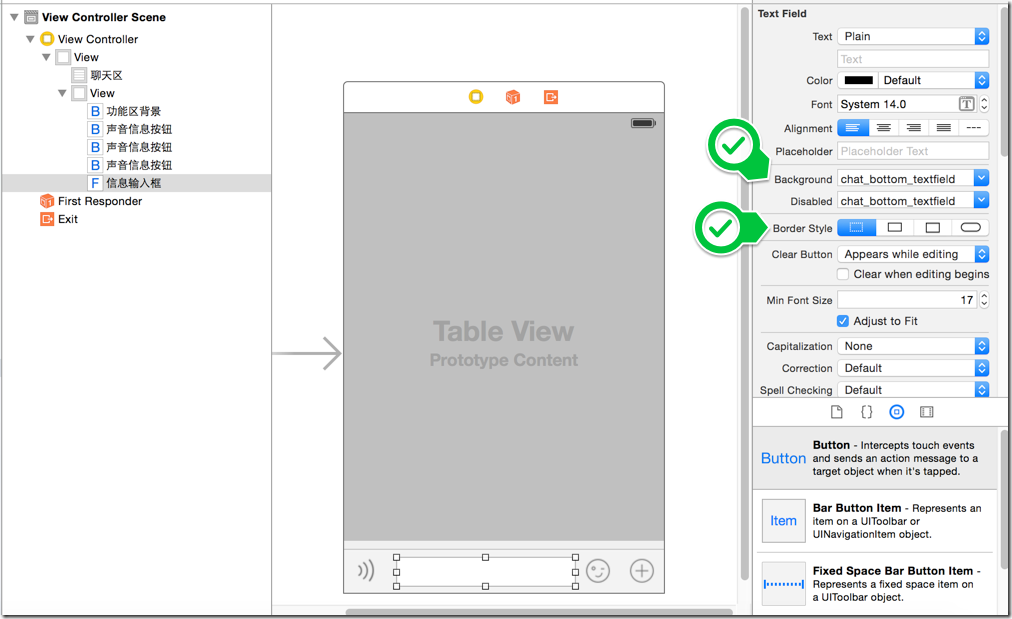
- 自定义message模型
- 自定义cell
- 装载了message模型和cell子控件位置尺寸的frame

@implementation NSString (Extension) /** 测量文本的尺寸 */
- (CGSize)sizeWithFont:(UIFont *)font maxSize:(CGSize)maxSize {
NSDictionary *attrs = @{NSFontAttributeName: font};
CGSize size = [self boundingRectWithSize:maxSize options:NSStringDrawingUsesLineFragmentOrigin attributes:attrs context:nil].size; return size;
} @end
// 3.信息,尺寸可变
CGFloat screenWidth = [UIScreen mainScreen].bounds.size.width;
// 3.1 设置最大尺寸
CGSize textMaxSize = CGSizeMake(screenWidth - iconWidth - padding * , MAXFLOAT);
// 3.2 计算真实尺寸
CGSize textRealSize = [message.text sizeWithFont:MESSAGE_TEXT_FONT maxSize:textMaxSize]; // 3.3 调整信息的位置
CGFloat textX;
if (MessageTypeMe == message.type) {
// 我方,放在靠右
textX = CGRectGetMinX(_iconFrame) - textRealSize.width - padding;
} else {
// 对方,放在靠左
textX = CGRectGetMaxX(_iconFrame) + padding;
} CGFloat textY = iconY;
_textFrame = CGRectMake(textX, textY, textRealSize.width, textRealSize.height);
// 2.头像
CGFloat iconWidth = ;
CGFloat iconHeight = ; // 2.1 根据信息的发送方调整头像位置
CGFloat iconX;
if (MessageTypeMe == message.type) {
// 我方,放在右边
iconX = [UIScreen mainScreen].bounds.size.width - padding - iconWidth;
} else {
// 对方,放在左边
iconX = padding;
} CGFloat iconY = CGRectGetMaxY(_timeFrame) + padding;
_iconFrame = CGRectMake(iconX, iconY, iconWidth, iconHeight);
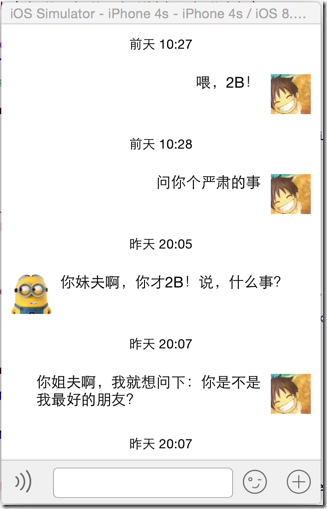
@implementation UIImage (Extension) + (UIImage *) resizableImage:(NSString *) imageName {
UIImage *image = [UIImage imageNamed:imageName];
// 取图片中部的1 x 1进行拉伸
UIEdgeInsets insets = UIEdgeInsetsMake(image.size.height/, image.size.width/, image.size.height/ + , image.size.width/ + );
return [image resizableImageWithCapInsets:insets];
} @end
// 3.1 设置聊天框
NSString *chatImageNormalName;
NSString *chatImageHighlightedName;
if (MessageTypeMe == messageFrame.message.type) {
chatImageNormalName = @"chat_send_nor";
chatImageHighlightedName = @"chat_send_press_pic";
} else {
chatImageNormalName = @"chat_receive_nor";
chatImageHighlightedName = @"chat_receive_press_pic";
} UIImage *chatImageNormal = [UIImage resizableImage:chatImageNormalName];
UIImage *chatImageHighlighted = [UIImage resizableImage:chatImageHighlightedName];
[self.textView setBackgroundImage:chatImageNormal forState:UIControlStateNormal];
[self.textView setBackgroundImage:chatImageHighlighted forState:UIControlStateHighlighted];
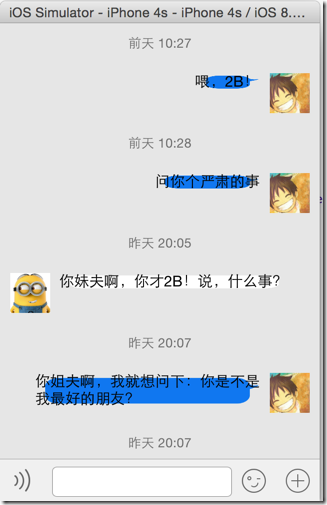
// 3.2 调整文字的内边距
textView.contentEdgeInsets = UIEdgeInsetsMake(TEXT_INSET, TEXT_INSET, TEXT_INSET, TEXT_INSET);
// 3.信息,尺寸可变
CGFloat screenWidth = [UIScreen mainScreen].bounds.size.width;
// 3.1 设置文本最大尺寸
CGSize textMaxSize = CGSizeMake(screenWidth - iconWidth - padding * , MAXFLOAT);
// 3.2 计算文本真实尺寸
CGSize textRealSize = [message.text sizeWithFont:MESSAGE_TEXT_FONT maxSize:textMaxSize]; // 3.3 按钮尺寸
CGSize btnSize = CGSizeMake(textRealSize.width + TEXT_INSET*, textRealSize.height + TEXT_INSET*); // 3.4 调整信息的位置
CGFloat textX;
if (MessageTypeMe == message.type) {
// 我方,放在靠右
textX = CGRectGetMinX(_iconFrame) - btnSize.width - padding;
} else {
// 对方,放在靠左
textX = CGRectGetMaxX(_iconFrame) + padding;
} CGFloat textY = iconY;
_textFrame = CGRectMake(textX, textY, btnSize.width, btnSize.height);

/** 是否隐藏发送时间 */
@property(nonatomic, assign) BOOL hideTime;
// 判断是否发送时间与上一条信息的发送时间相同,若是则不用显示了
MessageFrame *lastMessageFrame = [mdictArray lastObject];
if (lastMessageFrame && [message.time isEqualToString:lastMessageFrame.message.time]) {
message.hideTime = YES;
}
// 1.发送时间
if (NO == message.hideTime) {
CGFloat timeWidth = [UIScreen mainScreen].bounds.size.width;
CGFloat timeHeight = ;
CGFloat timeX = ;
CGFloat timeY = ;
_timeFrame = CGRectMake(timeX, timeY, timeWidth, timeHeight);
}
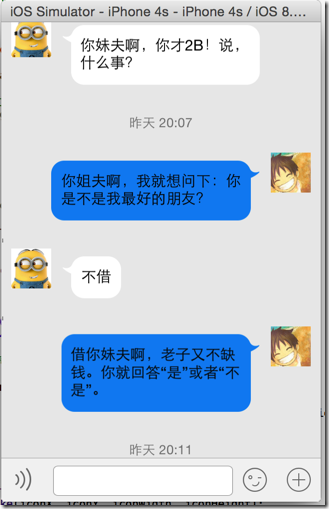
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillChangeFrame:) name:UIKeyboardWillChangeFrameNotification object:nil];
/** 点击拖曳聊天区的时候,缩回键盘 */
- (void)scrollViewWillBeginDragging:(UIScrollView *)scrollView {
// 1.缩回键盘
[self.view endEditing:YES];
} #pragma mark - 监听事件
- (void) keyboardWillChangeFrame:(NSNotification *) note {
// 1.取得弹出后的键盘frame
CGRect keyboardFrame = [note.userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue]; // 2.键盘弹出的耗时时间
CGFloat duration = [note.userInfo[UIKeyboardAnimationDurationUserInfoKey] floatValue]; // 3.键盘变化时,view的位移,包括了上移/恢复下移
CGFloat transformY = keyboardFrame.origin.y - self.view.frame.size.height; [UIView animateWithDuration:duration animations:^{
self.view.transform = CGAffineTransformMakeTranslation(, transformY);
}]; }
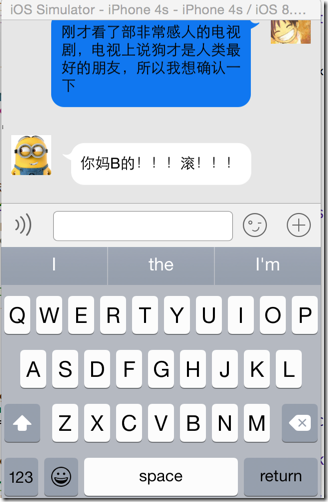
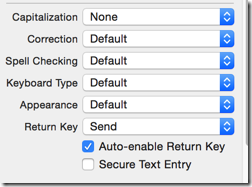
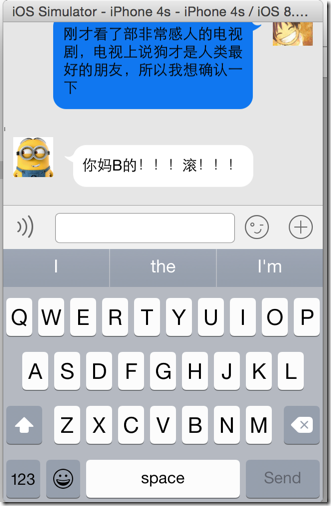
// 设置信息输入框的代理
self.inputView.delegate = self;
#pragma mark - TextField 代理方法
/** 回车响应事件 */
- (BOOL)textFieldShouldReturn:(UITextField *)textField {
// 我方发出信息
[self sendMessageWithContent:textField.text andType:MessageTypeMe]; // 自动回复
[self sendMessageWithContent:[NSString stringWithFormat:@"%@\n%@", textField.text, @"你妹!!!"] andType:MessageTypeOhter]; // 消除消息框内容
self.inputView.text = nil; [self.tableView reloadData]; // 滚动到最新的消息
NSIndexPath *lastIndexPath = [NSIndexPath indexPathForRow:self.messages.count - inSection:];
[self.tableView scrollToRowAtIndexPath:lastIndexPath atScrollPosition:UITableViewScrollPositionBottom animated:YES]; return YES; // 返回值意义不明
} // 发送消息
- (void) sendMessageWithContent:(NSString *) text andType:(MessageType) type {
// 获取当前时间
NSDate *date = [NSDate date];
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
formatter.dateFormat = @"yyyy-MMM-dd hh:mm:ss";
NSString *dateStr = [formatter stringFromDate:date]; // 我方发出信息
NSDictionary *dict = @{@"text":text,
@"time":dateStr,
@"type":[NSString stringWithFormat:@"%d", type]}; Message *message = [[Message alloc] init];
[message setValuesForKeysWithDictionary:dict];
MessageFrame *messageFrame = [[MessageFrame alloc] init];
messageFrame.message = message; [self.messages addObject:messageFrame];
}
// 滚动到最新的消息
NSIndexPath *lastIndexPath = [NSIndexPath indexPathForRow:self.messages.count - inSection:];
[self.tableView scrollToRowAtIndexPath:lastIndexPath atScrollPosition:UITableViewScrollPositionBottom animated:YES];
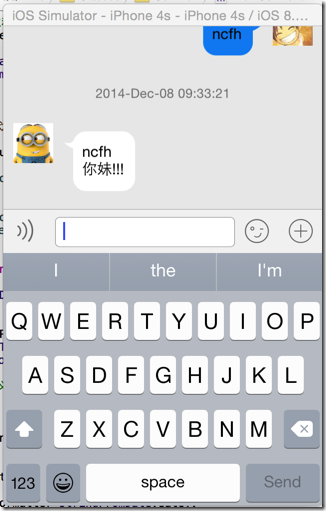
[iOS基础控件 - 6.9] 聊天界面Demo的更多相关文章
- [iOS基础控件 - 5.5] 代理设计模式 (基于”APP列表"练习)
A.概述 在"[iOS基础控件 - 4.4] APP列表 进一步封装,初见MVC模式”上进一步改进,给“下载”按钮加上效果.功能 1.按钮点击后,显示为“已下载”,并且不 ...
- [iOS基础控件 - 6.9.1] 聊天界面Demo 代码
框架: 所有代码文件: Model: // // Message.h // QQChatDemo // // Created by hellovoidworld on 14/12/8. // ...
- [iOS基础控件 - 3.1] QQ登陆界面
A.storyboard 控件版 1.label 2.textfield a.Keyboard Type 账号:Number Pad 密码:Num ...
- iOS 基础控件(下)
上篇介绍了UIButton.UILabel.UIImageView和UITextField,这篇就简短一点介绍UIScrollView和UIAlertView. UIScrollView 顾名思义也知 ...
- [iOS基础控件 - 6.11.3] 私人通讯录Demo 控制器的数据传递、存储
A.需求 1.搭建一个"私人通讯录"Demo 2.模拟登陆界面 账号 密码 记住密码开关 自动登陆开关 登陆按钮 3.退出注销 4.增删改查 5.恢复数据(取消修改) 这个代码 ...
- [iOS基础控件 - 6.10.2] PickerView 自定义row内容 国家选择Demo
A.需求 1.自定义一个UIView和xib,包含国家名和国旗显示 2.学习row的重用 B.实现步骤 1.准备plist文件和国旗图片 2.创建模型 // // Flag.h // Co ...
- [iOS基础控件 - 7.0] UIWebView
A.基本使用 1.概念 iOS内置的浏览器控件 Safari浏览器就是通过UIWebView实现的 2.用途:制作简易浏览器 (1)基本请求 创建请求 加载请求 (2)代理监听webView加载, ...
- [iOS基础控件 - 6.9.3] QQ好友列表Demo TableView
A.需求 1.使用plist数据,展示类似QQ好友列表的分组.组内成员显示缩进功能 2.组名使用Header,展示箭头图标.组名.组内人数和上线人数 3.点击组名,伸展.缩回好友组 code so ...
- [iOS基础控件 - 6.7] 微博展示 使用代码自定义TableCell(动态尺寸)
A.需求 1.类似于微博内容的展示 2.头像 3.名字 4.会员标志 5.内容 6.分割线 7.配图(可选,可有可无) code source: https://github.com/hellov ...
随机推荐
- [HDOJ4022]Bombing(离散化+stl)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4022 一个图上有n个点,之后m个操作,每次操作一行或者一列.使得这一行或者这一列的点全部消除.每次操作 ...
- 通俗易懂的讲解iphone视图控制器的生命周期
IOS 视图控制器的生命周期非常非常重要,所以我有必要写个文章来和大家一起探讨问题. 今天在学习视图控制器的生命周期,也看了一下网上的一些资料,但总觉得不是那么好理解,首先我们来看一张图: 先粗略讲一 ...
- 函数fsp_try_extend_data_file
扩展表空间 /***********************************************************************//** Tries to extend t ...
- RecyclerView 结合 CardView 使用(二)
上一篇的基础上,修改了,CardView的布局和点击效果 总结: CardView的奇葩属性 :app:cardPreventCornerOverlap="false" 和园角边框 ...
- NavieBayes中的多项式与伯努力模型
1文本分类过程 例如文档:Good good study Day day up可以用一个文本特征向量来表示,x=(Good, good, study, Day, day , up).在文本分类中,假设 ...
- LA 3708 Graveyard 墓地雕塑 NEERC 2006
在一个周长为 10000 的圆上等距分布着 n 个雕塑.现在又有 m 个新雕塑加入(位置可以随意摆放),希望所有 n + m 个雕塑能在圆周上均匀分布.这就需要移动一些原有的雕塑.要求 n 个雕塑移动 ...
- mysql互为主从复制配置笔记
MySQL-master1:192.168.72.128 MySQL-master2:192.168.72.129 OS版本:CentOS 5.4MySQL版本:5.5.9(主从复制的master和s ...
- jsp防盗链代码
// 禁止缓存 response.setHeader("Cache-Control", "no-store"); response.setHeader( ...
- MySQL基础之第5章 操作数据库
假设已经登录 mysql-h localhost -uroot -proot 5.1.显示.创建.删除数据库 show databases; 显示所有的数据库 create database ...
- 深入浅出 iOS 之生命周期
转:http://blog.csdn.net/kesalin/article/details/6691766 iOS应用程序的生命周期相比 Android 应用程序的生命周期来说,没那么简明易懂,但是 ...