hdu5531 Rebuild
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 262144/262144 K (Java/Others)
Total Submission(s): 181 Accepted Submission(s): 42
The ruins form a closed path on an x-y plane, which has n endpoints.
The endpoints locate on (x1,y1), (x2,y2), …,(xn,yn) respectively.
Endpoint i and
endpointi−1 are
adjacent for 1<i≤n,
also endpoint 1 and
endpoint n are
adjacent. Distances between any two adjacent endpoints are positive integers.
To rebuild, they need to build one cylindrical pillar at each endpoint, the radius of the pillar of endpoint i is ri.
All the pillars perpendicular to the x-y plane, and the corresponding endpoint is on the centerline of it. We call two pillars are adjacent if and only if two corresponding endpoints are adjacent. For any two adjacent pillars, one must be tangent externally
to another, otherwise it will violate the aesthetics of Ancient ACM Civilization. If two pillars are not adjacent, then there are no constraints, even if they overlap each other.
Note that ri must
not be less than 0 since
we cannot build a pillar with negative radius and pillars with zero radius are acceptable since those kind of pillars still exist in their neighbors.
You are given the coordinates of n endpoints.
Your task is to find r1,r2,…,rn which
makes sum of base area of all pillars as minimum as possible.
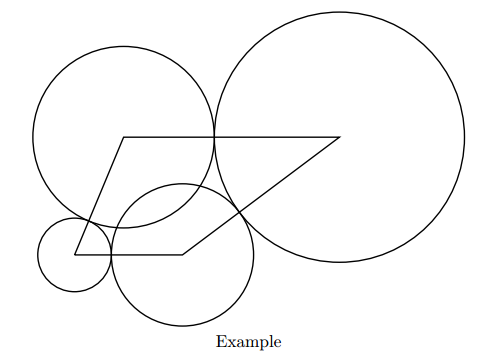
For example, if the endpoints are at (0,0), (11,0), (27,12), (5,12),
we can choose (r1, r2, r3, r4)=(3.75, 7.25, 12.75, 9.25).
The sum of base area equals to 3.752π+7.252π+12.752π+9.252π=988.816….
Note that we count the area of the overlapping parts multiple times.
If there are several possible to produce the minimum sum of base area, you may output any of them.
the total number of test cases. The following lines describe a test case.
The first line of each case contains one positive integer n,
the size of the closed path. Next n lines,
each line consists of two integers (xi,yi) indicate
the coordinate of the i-th
endpoint.
1≤t≤100
3≤n≤104
|xi|,|yi|≤104
Distances between any two adjacent endpoints are positive integers.
the i-th
of them should contain a number ri,
rounded to 2 digits after the decimal point.
If there are several possible ways to produce the minimum sum of base area, you may output any of them.
4
0 0
11 0
27 12
5 12
5
0 0
7 0
7 3
3 6
0 6
5
0 0
1 0
6 12
3 16
0 12
3.75
7.25
12.75
9.25
157.08
6.00
1.00
2.00
3.00
0.00
IMPOSSIBLE
#include<stdio.h>
#include<string.h>
#include<algorithm>
#include<math.h>
#include<iostream>
#include<stdlib.h>
#include<set>
#include<map>
#include<queue>
#include<vector>
#include<bitset>
#pragma comment(linker, "/STACK:1024000000,1024000000")
template <class T>
bool scanff(T &ret){ //Faster Input
char c; int sgn; T bit=0.1;
if(c=getchar(),c==EOF) return 0;
while(c!='-'&&c!='.'&&(c<'0'||c>'9')) c=getchar();
sgn=(c=='-')?-1:1;
ret=(c=='-')?0:(c-'0');
while(c=getchar(),c>='0'&&c<='9') ret=ret*10+(c-'0');
if(c==' '||c=='\n'){ ret*=sgn; return 1; }
while(c=getchar(),c>='0'&&c<='9') ret+=(c-'0')*bit,bit/=10;
ret*=sgn;
return 1;
}
#define inf 1073741823
#define llinf 4611686018427387903LL
#define PI acos(-1.0)
#define lth (th<<1)
#define rth (th<<1|1)
#define rep(i,a,b) for(int i=int(a);i<=int(b);i++)
#define drep(i,a,b) for(int i=int(a);i>=int(b);i--)
#define gson(i,root) for(int i=ptx[root];~i;i=ed[i].next)
#define tdata int testnum;scanff(testnum);for(int cas=1;cas<=testnum;cas++)
#define mem(x,val) memset(x,val,sizeof(x))
#define mkp(a,b) make_pair(a,b)
#define findx(x) lower_bound(b+1,b+1+bn,x)-b
#define pb(x) push_back(x)
using namespace std;
typedef long long ll;
typedef pair<int,int> pii;
#define NN 100100
struct node{
double x,y;
}a[NN];
int n;
double len[NN];
double r[NN];
double caldis(int x,int y){
return sqrt((a[x].x-a[y].x)*(a[x].x-a[y].x)+(a[x].y-a[y].y)*(a[x].y-a[y].y));
}
double cal(int idx,double lx){
double temp=lx;
double s=0.0;
rep(i,1,n){
s+=lx*lx;
r[idx]=lx;
if(lx<0.0||lx>len[idx]||lx>len[idx==1?n:idx-1])return -1.0;
lx=len[idx]-lx;
idx++;
if(idx>n)idx=1;
}
if(fabs(temp-lx)>1e-7)return -1.0;
return s;
}
int main(){
tdata{
scanff(n);
rep(i,1,n){
scanf("%lf%lf",&a[i].x,&a[i].y);
}
rep(i,1,n){
if(i!=n)len[i]=caldis(i,i+1);
else len[i]=caldis(i,1);
}
int idx;
if(n&1){
double lenx=0.0;
idx=1;
rep(i,1,n){
if(i&1)lenx+=len[idx];
else lenx-=len[idx];
idx++;
if(idx>n)idx=1;
}
lenx/=2.0;
double ans=cal(1,lenx);
if(ans>=0.0){
printf("%.2f\n",ans*PI);
rep(i,1,n){
printf("%.2f\n",r[i]);
}
}
else printf("IMPOSSIBLE\n");
}
else{
double lx=0.0,rx=len[1],t=0.0;
idx=1;
rep(i,1,n){
t=len[idx]-t;
if(i&1)rx=min(rx,t);
else lx=max(lx,-t);
idx++;
if(idx>n)idx=1;
}
if(lx>rx){
printf("IMPOSSIBLE\n");
continue;
}
rep(i,1,250){
/*
double d=(rx-lx)/3.0;
double d1=lx+d;
double d2=rx-d;
*/
double d1=(lx*2+rx)/3.0;
double d2=(lx+rx*2)/3.0;
if(cal(1,d1)<cal(1,d2))rx=d2;
else lx=d1;
}
double ans=cal(1,lx);
if(ans<0.0){
printf("IMPOSSIBLE\n");
continue;
}
printf("%.2f\n",ans*PI);
rep(i,1,n){
printf("%.2f\n",r[i]);
}
}
}
return 0;
}
hdu5531 Rebuild的更多相关文章
- Visual Studio 中 Build 和 Rebuild 的区别
因为之前写的程序比较小,编译起来比较快,所以一直都没有太在意 Build 和 Rebuild 之间的区别,后来发现两个还是有很大不同. Build 只针对在上次编译之后更改过的文件进行编译,在项目比较 ...
- 解决 node-gyp rebuild 卡住 的问题
node-gyp在编译前会首先尝试下载node的headers文件,像这样: gyp http GET https://nodejs.org/download/release/v6.8.1/node- ...
- AndroidStudio中make Project、clean Project、Rebuild Project的区别
1.Make Project:编译Project下所有Module,一般是自上次编译后Project下有更新的文件,不生成apk. 2.Make Selected Modules:编译指定的Modul ...
- Rebuild Instance 操作详解 - 每天5分钟玩转 OpenStack(37)
上一节我们讨论了 snapshot,snapshot 的一个重要作用是对 instance 做备份. 如果 instance 损坏了,可以通过 snapshot 恢复,这个恢复的操作就是 Rebuil ...
- Xcode7 *** does not contain bitcode. You must rebuild it with bitcode enabled (Xcode setting ENABLE_BITCODE)
*** does not contain bitcode. You must rebuild it with bitcode enabled (Xcode setting ENABLE_BITCODE ...
- rebuild new environment for DW step
Steps to rebuild PPE environment: (CTS) 1, Disable both CTS Daily Job (Daily) and CTS Daily Job (Sta ...
- node-gyp rebuild 卡住?
最近 npm install 时候经常遇到在 node-gyp rebuild 那里卡很久的情况(大于十分钟),于是研究了一下输出的错误日志解决了这个问题,在这里分享一下. 首先,请检查 node-g ...
- Andriod Studio Clear Project或Rebuild Project出错
以前在Eclipse中出现过类似的错误:在编译工程时,提示无法删除bin目录下的某个jar. 想不到Android Studio中也会有. Clear Project或Rebuild Project, ...
- 2015ACM/ICPC亚洲区长春站 E hdu 5531 Rebuild
Rebuild Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 262144/262144 K (Java/Others)Total S ...
随机推荐
- Hystrix-服务降级-服务熔断-服务限流
Hystrix简介 Hystrix是一个用于处理分布式系统的延迟和容错的开源库,在分布式系统里,许多依赖不可避免的会调用失败,比如超时.异常等,Hystrix能够保证在一个依赖出问题的情况下,不会导致 ...
- Python实验6--网络编程
题目1 1.编写程序实现基于多线程的TCP客户机/服务器程序. (1)创建服务器端套接字Socket,监听客户端的连接请求: (2)创建客户端套接字Socket,向服务器端发起连接: 服务器端套接字 ...
- Git软件安装过程
Git程序安装过程 官网: https://git-scm.com/ 下载: https://git-scm.com/downloads 我的操作系统是 Windows + 64位的 https:// ...
- C语言流程图画法(C语言学习笔记)
常用符号及其含义 图片来自百度文库 https://wenku.baidu.com/view/beb410dea216147916112853.html 常用结构 N-S图
- ctfhub技能树—信息泄露—git泄露—Stash
打开靶机环境 查看页面内容 使用dirsearch进行扫描 使用Githack工具处理git泄露情况 进入.git/refs目录 发现stash文件,使用notepad++打开文件 使用git dif ...
- 未使用绑定变量对share_pool的影响
oracle SGA中包含数据高速缓冲,重做日志缓冲,以及共享池(share_pool).共享池中包含库高速缓冲(所有的SQL,执行计划等)和数据字典缓冲(对象的定义,权限等). 所以,如果SQL中没 ...
- 干货!上古神器 sed 教程详解,小白也能看的懂
目录: 介绍工作原理正则表达式基本语法数字定址和正则定址基本子命令实战练习 介绍 熟悉 Linux 的同学一定知道大名鼎鼎的 Linux 三剑客,它们是 grep.awk.sed,我们今天要聊的主角就 ...
- ELK (elasticsearch+kibana+logstash+elasticsearch-head) 华为云下载地址
https://mirrors.huaweicloud.com/elasticsearch https://mirrors.huaweicloud.com/kibana https://mirrors ...
- SQL函数知识点
SQL函数知识点 SQL题目(一) 1.查询部门编号为10的员工信息 select*from emp where empno=10; 2.查询年薪大于3万的人员的姓名与部门编号 select enam ...
- 对于两个输入文件,即文件A 和文件B ,请编写MapReduce程序,对两个文件进行合并排除其中重复的内容,得到一个新的输出文件C。
package org.apache.hadoop.examples; import java.util.HashMap; import java.io.IOException; import jav ...