UVA - 297Quadtrees(四分图)
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
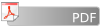
A quadtree is a representation format used to encode images. The fundamental idea behind the quadtree is that any image can be split into four quadrants. Each quadrant may again be split in four sub quadrants, etc. In the quadtree, the image is represented by a parent node, while the four quadrants are represented by four child nodes, in a predetermined order.
Of course, if the whole image is a single color, it can be represented by a quadtree consisting of a single node. In general, a quadrant needs only to be subdivided if it consists of pixels of different colors. As a result, the quadtree need not be of uniform depth.
A modern computer artist works with black-and-white images of units, for a total of 1024 pixels per image. One of the operations he performs is adding two images together, to form a new image. In the resulting image a pixel is black if it was black in at least one of the component images, otherwise it is white.
This particular artist believes in what he calls the preferred fullness: for an image to be interesting (i.e. to sell for big bucks) the most important property is the number of filled (black) pixels in the image. So, before adding two images together, he would like to know how many pixels will be black in the resulting image. Your job is to write a program that, given the quadtree representation of two images, calculates the number of pixels that are black in the image, which is the result of adding the two images together.
In the figure, the first example is shown (from top to bottom) as image, quadtree, pre-order string (defined below) and number of pixels. The quadrant numbering is shown at the top of the figure.
Input Specification
The first line of input specifies the number of test cases (N) your program has to process.
The input for each test case is two strings, each string on its own line. The string is the pre-order representation of a quadtree, in which the letter 'p' indicates a parent node, the letter 'f' (full) a black quadrant and the letter 'e' (empty) a white quadrant. It is guaranteed that each string represents a valid quadtree, while the depth of the tree is not more than 5 (because each pixel has only one color).
Output Specification
For each test case, print on one line the text 'There are X black pixels.', where X is the number of black pixels in the resulting image.
Example Input
3
ppeeefpffeefe
pefepeefe
peeef
peefe
peeef
peepefefe
Example Output
There are 640 black pixels.
There are 512 black pixels.
There are 384 black pixels.
题解:
给你两个像素,让求两个像素合并起来的黑色像素的大小;
由于给的是四分图的先序遍历,那么这个图也就可以画出来了,于是可以边建图,边画图,如果遇到黑色像素f就画图,如果遇到p就递归建图;
其中x,y表示坐上点的位置,len代表当前图的面积;
代码:
#include<cstdio>
#include<cstring>
#include<cmath>
#include<algorithm>
#include<queue>
using namespace std;
#define SI(x) scanf("%d",&x)
#define mem(x,y) memset(x,y,sizeof(x))
#define PI(x) printf("%d",x)
#define P_ printf(" ")
const int INF=0x3f3f3f3f;
typedef long long LL;
const int MAXN=40;
int buf[MAXN][MAXN];
char s[2020];
int cnt;
void draw(int &k,int x,int y,int len){
char ch=s[k++];
if(ch=='p'){
draw(k,x,y,len/2);
draw(k,x,y+len/2,len/2);
draw(k,x+len/2,y,len/2);
draw(k,x+len/2,y+len/2,len/2);
}
else if(ch=='f'){
for(int i=x;i<x+len;i++)
for(int j=y;j<y+len;j++)
if(!buf[i][j])buf[i][j]=1,cnt++;
}
}
int main(){
int T;
SI(T);
while(T--){
cnt=0;
mem(buf,0);
for(int i=0;i<2;i++){
mem(s,0);
scanf("%s",s);
int k=0;
draw(k,0,0,32);
}
printf("There are %d black pixels.\n",cnt);
}
return 0;
}
UVA - 297Quadtrees(四分图)的更多相关文章
- UVaLive 7375 Hilbert Sort (递归,四分图,模拟)
题意:告诉你一条希尔伯特曲线的大小,然后给你n 个人,及n 个人的坐标,你的起点是左下角,终点是右下角,按照希尔伯特的曲线去走,按照这个顺序给n个人排序, 按顺序输出每个人的名字! 析:这就是一个四分 ...
- 7.26T1四分图匹配
四分图匹配 题目描述 一天晚上,zzh 在做梦,忽然梦见了她. 见到她,zzh 也不去看她,只顾低头自语…… “噫,OI 这个东西,真是无奇不有.” “嘿,你又学了什么?” “嗯,学到了一种算法,”z ...
- UVA 11552 四 Fewest Flops
Fewest Flops Time Limit:2000MS Memory Limit:0KB 64bit IO Format:%lld & %llu Submit Statu ...
- Python可视化库Matplotlib的使用
一.导入数据 import pandas as pd unrate = pd.read_csv('unrate.csv') unrate['DATE'] = pd.to_datetime(unrate ...
- bzoj usaco 金组水题题解(2)
续.....TAT这回不到50题编辑器就崩了.. 这里塞40道吧= = bzoj 1585: [Usaco2009 Mar]Earthquake Damage 2 地震伤害 比较经典的最小割?..然而 ...
- Python的Matplotlib库简述
Matplotlib 库是 python 的数据可视化库import matplotlib.pyplot as plt 1.字符串转化为日期 unrate = pd.read_csv("un ...
- bzoj 4823 [Cqoi2017]老C的方块——网络流
题目:https://www.lydsy.com/JudgeOnline/problem.php?id=4823 一个不合法方案其实就是蓝线的两边格子一定选.剩下两部分四相邻格子里各选一个. 所以这个 ...
- unity3d之游戏优化
=============================================================================== 美术规格: 1.单个蒙皮网格渲染器2.一 ...
- python数据处理与机器学习
提纲 numpy: #genformtxt import numpy as np #genformtxtdata=np.genfromtxt("genfromtxtdata") # ...
随机推荐
- silverlight依赖属性
依赖属性(Dependency Property)和附加属性(Attached Property) 参考 http://www.cnblogs.com/KevinYang/archive/2010/0 ...
- English interview!
Q1:Why are you interested in working for our company?为什么有兴趣在我们公司工作?A1:Because your company has a goo ...
- 源码学习之ASP.NET MVC Application Using Entity Framework
源码学习的重要性,再一次让人信服. ASP.NET MVC Application Using Entity Framework Code First 做MVC已经有段时间了,但看了一些CodePle ...
- MVC自学第一课
了解传统的ASP.NET WebForm ASP.NET 在02年问世,给Web开发领域带来了巨大转变.下图描述了当时微软的技术堆栈. ASP.NET WebForm 技术堆栈 (注:此图的含义为,W ...
- Android XListView实现原理讲解及分析
XListview是一个非常受欢迎的下拉刷新控件,但是已经停止维护了.之前写过一篇XListview的使用介绍,用起来非常简单,这两天放假无聊,研究了下XListview的实现原理,学到了很多,今天分 ...
- Android消息机制不完全解析(下)
接着上一篇文章Android消息机制不完全解析(上),接着看C++部分的实现. 首先,看看在/frameworks/base/core/jni/android_os_MessageQueue.cpp文 ...
- iOS6和iOS7代码的适配(4)——tableView
iOS7上不少控件的样子有了变化(毕竟要扁平化嘛),不过感觉变化最大的肯定非tableView莫属.因为这个控件的高度可定制性,原先是使用及其广泛的,这样的一个改变自然也影响颇大. 1.accesso ...
- 解决Node.js调用fs.renameSync报错的问题(Error: EXDEV, cross-device link not permitted)
2014-08-23 今天开始学习Node.js,在写一个文件上传的功能时候,调用fs.renameSync方法错误 出错代码所在如下: function upload(response,reques ...
- ubuntu FTP服务安装
//安装vsftp apt-get install vsftpd -y //增加账号 //1 查找 nologin位置 /usr/sbin/nologin useradd -d /alidata/ww ...
- 关于HTTP 协议的特点,以及网络请求GET 和 POST 的区别?
HTTP协议有何特点: HTTP 超文本的传输协议,是短连接,是客户端主动发送请求,服务器做出响应,服务器响应之后连接断开 HTTP是属于应用层面向对象的协议,HTTP 有2类报文:请求报文和响应报文 ...