K - Ancient Messages(dfs求联通块)
Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu
Description
In order to understand early civilizations, archaeologists often study texts written in ancient languages. One such language, used in Egypt more than 3000 years ago, is based on characters called hieroglyphs. Figure C.1 shows six hieroglyphs and their names. In this problem, you will write a program to recognize these six characters.
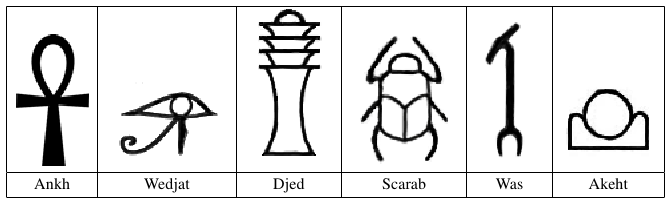
Input
The input consists of several test cases, each of which describes an image containing one or more hieroglyphs chosen from among those shown in Figure C.1. The image is given in the form of a series of horizontal scan lines consisting of black pixels (represented by 1) and white pixels (represented by 0). In the input data, each scan line is encoded in hexadecimal notation. For example, the sequence of eight pixels 10011100 (one black pixel, followed by two white pixels, and so on) would be represented in hexadecimal notation as 9c. Only digits and lowercase letters a through f are used in the hexadecimal encoding. The first line of each test case contains two integers, H and W. H(0 < H200) is the number of scan lines in the image. W(0 < W
50) is the number of hexadecimal characters in each line. The next H lines contain the hexadecimal characters of the image, working from top to bottom. Input images conform to the following rules:
- The image contains only hieroglyphs shown in Figure C.1.
- Each image contains at least one valid hieroglyph.
- Each black pixel in the image is part of a valid hieroglyph.
- Each hieroglyph consists of a connected set of black pixels and each black pixel has at least one other black pixel on its top, bottom, left, or right side.
- The hieroglyphs do not touch and no hieroglyph is inside another hieroglyph.
- Two black pixels that touch diagonally will always have a common touching black pixel.
- The hieroglyphs may be distorted but each has a shape that is topologically equivalent to one of the symbols in Figure C.1. (Two figures are topologically equivalent if each can be transformed into the other by stretching without tearing.)
The last test case is followed by a line containing two zeros.
Output
For each test case, display its case number followed by a string
containing one character for each hieroglyph recognized in the image,
using the following code:
Ankh: A
Wedjat: J
Djed: D
Scarab: S
Was: W
Akhet: K
In each output string, print the codes in alphabetic order. Follow the format of the sample output.
The sample input contains descriptions of test cases shown in Figures
C.2 and C.3. Due to space constraints not all of the sample input can be
shown on this page.
Sample Input
100 25
0000000000000000000000000
0000000000000000000000000
...(50 lines omitted)...
00001fe0000000000007c0000
00003fe0000000000007c0000
...(44 lines omitted)...
0000000000000000000000000
0000000000000000000000000
150 38
00000000000000000000000000000000000000
00000000000000000000000000000000000000
...(75 lines omitted)...
0000000003fffffffffffffffff00000000000
0000000003fffffffffffffffff00000000000
...(69 lines omitted)...
00000000000000000000000000000000000000
00000000000000000000000000000000000000
0 0
Sample Output
Case 1: AKW
Case 2: AAAAA
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cmath>
#include <algorithm>
#include <string>
#include <vector>
#include <stack>
#include <queue>
#include <set>
#include <map>
#include <list>
#include <iomanip>
#include <cstdlib>
#include <sstream>
using namespace std;
const int INF=0x5fffffff;
const double EXP=1e-;
const int maxh=;
const int maxw=*+;
int H,W;
int pic[maxh][maxw],color[maxh][maxw];
int dir[][]={{,},{,},{,-},{-,}};
char bin[][];
char line[maxw];
const char* code = "WAKJSD"; void decode(int row,int col,char c)
{
for(int i=;i<;i++)
{
pic[row][col+i]=bin[(int)c][i]-'';
}
} void dfs(int row,int col,int c)
{
color[row][col]=c;
for(int i=;i<;i++)
{
int x=row+dir[i][];
int y=col+dir[i][];
if(x>=&&x<H&&y>=&&y<W&&pic[x][y]==pic[row][col]&&color[x][y]==)
dfs(x,y,c);
}
} vector<set<int> > neighbor; void check(int row,int col) //check white
{
for(int i=;i<;i++)
{
int x=row+dir[i][];
int y=col+dir[i][];
if(x>=&&x<H&&y>=&&y<W&&pic[x][y]==&&color[x][y]!=)
neighbor[color[row][col]].insert(color[x][y]);
}
} char recognize(int c)
{
int cnt=neighbor[c].size();
return code[cnt];
} int main()
{
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin[''], "");
strcpy(bin['a'], "");
strcpy(bin['b'], "");
strcpy(bin['c'], "");
strcpy(bin['d'], "");
strcpy(bin['e'], "");
strcpy(bin['f'], "");
int kase=;
while(scanf("%d%d",&H,&W)==&&(H+W))
{
memset(pic,,sizeof(pic));
memset(color,,sizeof(color));
for(int i=;i<H;i++)
{
scanf("%s",line);
for(int j=;j<W;j++)
decode(i+,j*+,line[j]); //注意起始位置
}
H+=;
W=W*+;
int cnt=;
vector<int > c;
for(int i=;i<H;i++)
for(int j=;j<W;j++)
{
if(color[i][j]==)
{
dfs(i,j,++cnt);
if(pic[i][j]==)
c.push_back(cnt);
}
}
neighbor.clear();
neighbor.resize(cnt+);
for(int i=;i<H;i++)
for(int j=;j<W;j++)
{
if(pic[i][j]==)
check(i,j);
}
vector<char> ans;
int t=c.size();
for(int i=;i<t;i++)
ans.push_back(recognize(c[i]));
sort(ans.begin(),ans.end());
printf("Case %d: ",kase++);
for(int i=;i<t;i++)
printf("%c",ans[i]);
printf("\n");
}
return ;
}
K - Ancient Messages(dfs求联通块)的更多相关文章
- 利用DFS求联通块个数
/*572 - Oil Deposits ---DFS求联通块个数:从每个@出发遍历它周围的@.每次访问一个格子就给它一个联通编号,在访问之前,先检查他是否 ---已有编号,从而避免了一个格子重复访问 ...
- 【紫书】Oil Deposits UVA - 572 dfs求联通块
题意:给你一个地图,求联通块的数量. 题解: for(所有还未标记的‘@’点) 边dfs边在vis数组标记id,直到不能继续dfs. 输出id及可: ac代码: #define _CRT_SECURE ...
- 用dfs求联通块(UVa572)
一.题目 输入一个m行n列的字符矩阵,统计字符“@”组成多少个八连块.如果两个字符所在的格子相邻(横.竖.或者对角线方向),就说它们属于同一个八连块. 二.解题思路 和前面的二叉树遍历类似,图也有DF ...
- HDU - 1213 dfs求联通块or并查集
思路:给定一个无向图,判断有几个联通块. AC代码 #include <cstdio> #include <cmath> #include <algorithm> ...
- 中矿新生赛 H 璐神看岛屿【BFS/DFS求联通块/连通块区域在边界则此连通块无效】
时间限制:C/C++ 1秒,其他语言2秒空间限制:C/C++ 32768K,其他语言65536K64bit IO Format: %lld 题目描述 璐神现在有张n*m大小的地图,地图上标明了陆地(用 ...
- Educational Codeforces Round 1D 【DFS求联通块】
http://blog.csdn.net/snowy_smile/article/details/49924965 D. Igor In the Museum time limit per test ...
- POJ 1562 Oil Deposits (并查集 OR DFS求联通块)
Oil Deposits Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 14628 Accepted: 7972 Des ...
- HDU1241 Oil Deposits —— DFS求连通块
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1241 Oil Deposits Time Limit: 2000/1000 MS (Java/Othe ...
- C. Learning Languages 求联通块的个数
C. Learning Languages 1 #include <iostream> 2 #include <cstdio> 3 #include <cstring&g ...
随机推荐
- hdu 1045 Fire Net(最小覆盖点+构图(缩点))
http://acm.hdu.edu.cn/showproblem.php?pid=1045 Fire Net Time Limit:1000MS Memory Limit:32768KB ...
- XML的特殊字符处理
XML中共有5个特殊的字符,分别是:&<>“’.如果配置文件中的注入值包括这些特殊字符,就需要进行特别处理.有两种解决方法:其一,采用本例中的<![CDATA[ ]]> ...
- 咏南WEB开发框架
和咏南CS开发框架共享同一个咏南中间件.
- JavaScript 核心参考教程 内置对象
这个标准基于 JavaScript (Netscape) 和 JScript (Microsoft).Netscape (Navigator 2.0) 的 Brendan Eich 发明了这门语言,从 ...
- CodeForces 710E Generate a String (DP)
题意:给定 n,x,y,表示你要建立一个长度为 n的字符串,如果你加一个字符要花费 x时间,如果你复制前面的字符要花费y时间,问你最小时间. 析:这个题,很明显的DP,dp[i]表示长度为 i 的字符 ...
- SetTimer函数的用法
什么时候我们需要用到SetTimer函数呢?当你需要每个一段时间执行一件事的的时候就需要使用SetTimer函数 了.使用定时器的方法比较简单,通常告诉WINDOWS一个时间间隔,然后WINDOWS以 ...
- thinkphp框架 查询语言
thinkphp框架 查询语言 EQ 等于 (=) NEQ 不等于 (!=) GT 大于 (>) EGT 大于等于 (>=) LT ...
- C#泛型在unity3D中的运用...
泛型是什么? 这是摘自百度百科中对泛型的介绍: 泛型是c#2.0的一个新增加的特性,它为使用c#语言编写面向对象程序增加了极大的效力和灵活性.不会强行对值类型进行装箱和拆箱,或对引用类型进行向下强制类 ...
- android 动画属性(一)之Animation
Animation 在android 程序当中很多时候要用到动画效果,而动画效果主要是Animation来实现的,API给出的解释: 其中包含4种动画效果 AlphaAnimation 渐变透明度 R ...
- 异常:exception和error的区别
Throwable 是所有 Java 程序中错误处理的父类 ,有两种子类: Error 和 Exception . Error :表示由 JVM 所侦测到的无法预期的错误,由于这是属于 JVM ...