POJ 1099 Square Ice
Description
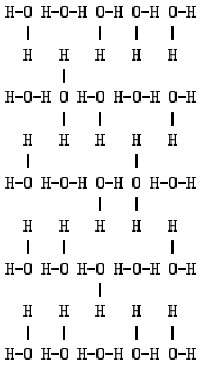
Note that each hydrogen atom is attached to exactly one of its neighboring oxygen atoms and each oxygen atom is attached to two of its neighboring hydrogen atoms. (Recall that one water molecule is a unit of one O linked to two H's.)
It turns out we can encode a square ice pattern with what is known as an alternating sign matrix (ASM): horizontal molecules are encoded as 1, vertical molecules are encoded as -1 and all other molecules are encoded as 0. So, the above pattern would be encoded as:
An ASM is a square matrix with entries 0, 1 and -1, where the sum of each row and column is 1 and the non-zero entries in each row and in each column must alternate in sign. (It turns out there is a one-to-one correspondence between ASM's and square ice patterns!)
Your job is to display the square ice pattern, in the same format as the example above, for a given ASM. Use dashes (-) for horizontal attachments and vertical bars (|) for vertical attachments. The pattern should be surrounded with a border of asterisks (*), be left justified and there should be exactly one character between neighboring hydrogen atoms (H) and oxygen atoms (O): either a space, a dash or a vertical bar.
Input
Output
Sample Input
2
0 1
1 0
4
0 1 0 0
1 -1 0 1
0 0 1 0
0 1 0 0
0
Sample Output
Case 1: ***********
*H-O H-O-H*
* | *
* H H *
* | *
*H-O-H O-H*
*********** Case 2: *******************
*H-O H-O-H O-H O-H*
* | | | *
* H H H H *
* | *
*H-O-H O H-O H-O-H*
* | | *
* H H H H *
* | | *
*H-O H-O H-O-H O-H*
* | *
* H H H H *
* | | | *
*H-O H-O-H O-H O-H*
*******************
题目大意:模拟
分析: 1.1个'O'连接2个'H',1个'H'只连接1个'O'
2.初始化不可以用memset(map,0,sizeof(map)) 应该初始化为空格
3.1对应两个'-',-1对应两个'|',0必须对应一个'-'和一个'|'
4.模拟题目尽量少开flag标记,容易出错又不好检查出来,尽量按照正常思维模拟。
代码如下:
# include <iostream>
# include<cstdio>
# include<cstring>
# include<cmath>
using namespace std;
char map[][];
int s[][]; bool judge(int i,int j)
{
if(map[i-][j]=='|'||map[i+][j]=='|'||map[i][j-]=='-'||map[i][j+]=='-')
return false;
return true;
} int main()
{
int T,cas=,i,j,n,a,b;
while(scanf("%d",&n) && n)
{
for(i=; i<n; i++)
for(j=; j<n; j++)
scanf("%d",&s[i][j]);
int y = *n+;
int x = *n-;
for(i=; i<=x; i++)
for(j=; j<=y; j++)
map[i][j] = ' '; for(i=; i<x; i++)
map[i][] = map[i][y-] = '*';
for(j=; j<y; j++)
map[][j] = map[x-][j] = '*'; for(i=; i<=x-; i+=)
{
for(j=; j<=y-; j+=)
map[i][j] = 'H';
for(j=; j<=y-; j+=)
map[i][j] = 'O';
}
for(i=; i<=x-; i+=)
{
for(j=; j<=y-; j+=)
map[i][j] = 'H';
} for(i=; i<n; i++)
{
a = i*+;
for(j=; j<n; j++)
{
b= j*+;
if(s[i][j] == )
{
map[a][b-] = '-';
map[a][b+] = '-';
}
else if(s[i][j] == -)
{
map[a+][b] = '|';
map[a-][b] = '|';
}
}
} for(i=; i<n; i++)
{
for(j=; j<n; j++)
{
if(s[i][j]==)
{
a = i*+;
b= j*+;
if(judge(a,b-))
map[a][b-] = '-';
else
map[a][b+] = '-';
if(a->&&judge(a-,b))
map[a-][b] = '|';
else
map[a+][b] = '|';
}
}
} printf("Case %d:\n\n",cas++);
for(i=; i<x; i++)
{
for(j=; j<y; j++)
{
printf("%c",map[i][j]);
}
printf("\n");
}
printf("\n");
}
return ;
}
POJ 1099 Square Ice的更多相关文章
- POJ 1099 Square Ice 连蒙带猜+根据样例找规律
目录 题面 思路 思路 AC代码 题面 Square Ice Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 4526 A ...
- [ACM_其他] Square Ice (poj1099 规律)
Description Square Ice is a two-dimensional arrangement of water molecules H2O, with oxygen at the v ...
- DFS POJ 2362 Square
题目传送门 /* DFS:问能否用小棍子组成一个正方形 剪枝有3:长的不灵活,先考虑:若根本构不成正方形,直接no:若第一根比边长长,no 这题是POJ_1011的精简版:) */ #include ...
- poj 1099
http://poj.org/problem?id=1099 #include<stdio.h> #include<string.h> #include <iostrea ...
- (中等) POJ 1084 Square Destroyer , DLX+可重复覆盖。
Description The left figure below shows a complete 3*3 grid made with 2*(3*4) (=24) matchsticks. The ...
- POJ 2362 Square DFS
传送门:http://poj.org/problem?id=2362 题目大意: 给一些不同长度的棍棒,问是否可能组成正方形. 学习了写得很好的dfs 赶紧去玩博饼了.....晚上三个地方有约.... ...
- POJ 2362 Square
题意:给n个木棍,问能不能正好拼成一个正方形. 解法:POJ1011的简单版……不需要太多剪枝……随便剪一剪就好了……但是各种写屎来着QAQ 代码: #include<stdio.h> # ...
- [DLX反复覆盖] poj 1084 Square Destroyer
题意: n*n的矩形阵(n<=5),由2*n*(n+1)根火柴构成,那么当中会有非常多诸如边长为1,为2...为n的正方形,如今能够拿走一些火柴,那么就会有一些正方形被破坏掉. 求在已经拿走一些 ...
- POJ题目排序的Java程序
POJ 排序的思想就是根据选取范围的题目的totalSubmittedNumber和totalAcceptedNumber计算一个avgAcceptRate. 每一道题都有一个value,value ...
随机推荐
- 转(HP大中华区总裁孙振耀退休感言)
开篇转发一篇好文,苦闷,消沉,寂寞,堕落的时候看看. 发现这篇文章是09年之前就有人转发到自己博客了.放到自己的地盘,容易记起有这么个心灵鸡汤. 一.关于工作与生活 我有个有趣的观察,外企公司多的 ...
- 将某个组中的账户移动到新的OU下
将某个组中的账户移动到新的OU下 #定义组名 $groupname = "testg" #定义新的OU名称 $newou = "OU=oo,OU=Admins,dc=dd ...
- PS自定义对象二_PSCustomObject
创建自定义对象 $obj = [pscustomobject]@{a=1;b="";c=$null} % 选择属性列 $obj | gm | % definition ( $ob ...
- 从零开始学android开发-IDE空间不够报错
E:\ProSoft\adt-bundle-windows-x86-20140321\eclipse目录下 右键eclipse用记事本打开 可以设置运行的最大的运行空间
- 从零开始学android开发-获取TextView的值
昨日写一个Android Demo,逻辑大概是从TextView获取其中的值,然后处理后再放回TextView中.整个处理过程是由一个Button的OnClick触发的. 可是在调试的过程中,一点击B ...
- PHP strlen() 函数
定义和用法 strlen() 函数返回字符串的长度. 语法 strlen(string) 参数 描述 string 必需.规定要检查的字符串. 例子 <?php echo strlen(&quo ...
- android图片处理方法(不断收集中)
//压缩图片大小 public static Bitmap compressImage(Bitmap image) { ByteArrayOutputStream baos = new ByteArr ...
- windows MySQL 5+ 服务手动安装
一.手动安装mysql 1.准备一个mysql免安装版本(把原来安装好的版本复制一份即可.一次安装多次使用^_^),将mysql复制到指定目录. 2.配置my.ini文件(本例使用的是5.0.22版本 ...
- 王帅:深入PHP内核
[问底]王帅:深入PHP内核(三)——内核利器哈希表与哈希碰撞攻击 [问底]王帅:深入PHP内核(二)——SAPI探究 [问底]王帅:深入PHP内核(一)——弱类型变量原理探究
- Visual Studio 2012 Web一键式发布
按照保哥的介绍,尝试“ Web一键式发布”,但总是出错,主要就是404错误,不知道是什么原因.默认的 Web一键式发布是在C:\inetpub\wwwroot目录下,难道是权限问题?折腾N久无果.好吧 ...