【Qt开发】Qt在QLabel(QWidget)鼠标绘制直线和矩形框
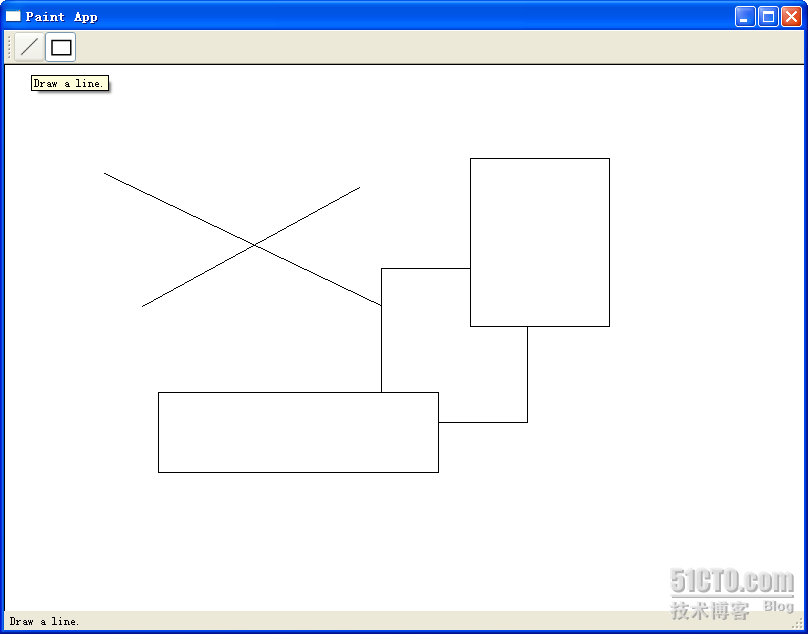


































bar);









bar);


























































































*event)































*event)








































& painter) = 0;





























































【Qt开发】Qt在QLabel(QWidget)鼠标绘制直线和矩形框的更多相关文章
- Qt 为QPushButton、QLabel添加鼠标移入移出事件
QT 为QPushButton.QLabel添加鼠标移入移出事件**要实现的效果:**鼠标移入QPushButton时与移出时按钮变换字体颜色,鼠标移入QLabel时显示上面的文字,移出时不显示.** ...
- c#在pictureBox控件上绘制多个矩形框及删除绘制的矩形框
在pictureBox上每次只绘制一个矩形框,绘制下一个矩形框时上次绘制的矩形框取消,代码如链接:https://www.cnblogs.com/luxiao/p/5625196.html 在绘制矩形 ...
- 【Qt开发】在QLabel已经显示背景图片后绘制图形注意事项
主要是要解决图形覆盖的问题,通常的办法就是对QLabel进行子类化,并重载函数: void myLabel::paintEvent(QPaintEvent *event) { QLab ...
- QT笔记 -- (3) 为QLabel添加鼠标响应方法1
参考 http://qt-project.org/wiki/Make-a-QLabel-Clickable 1.首先重载QLabel的mousePressEvent,这样点击QLabel时就能发出cl ...
- QT笔记 -- (4) 为QLabel添加鼠标响应方法2
1.实现 bool eventFilter(QObject *target,QEvent *event) 函数内容如下: bool eventFilter(QObject *target,QEvent ...
- opencv鼠标绘制直线 C++版
因为需要在图片上标记直线,所以从网上找了相应的参考资料.但大多都是c风格的,于是自己就写了一个c++风格的. opencv2.4.11,win8.1,vs2013 #include <cv.h& ...
- OpenCV绘制直线,矩形和园
首先导入我们所需要的库: import numpy as np import cv2 import matplotlib.pyplot as plt 自定义显示图像的函数: def show(imag ...
- Java基础之在窗口中绘图——绘制直线和矩形(Sketcher 2 drawing lines and rectangles)
控制台程序. import javax.swing.JComponent; import java.util.*; import java.awt.*; import java.awt.geom.*; ...
- Qt开发Activex笔记(一):环境搭建、基础开发流程和演示Demo
前言 使用C#开发动画,绘图性能跟不上,更换方案使用Qt开发Qt的控件制作成OCX以供C#调用,而activex则是ocx的更高级形式. QtCreator是没有Active控件项目的,所有需要 ...
随机推荐
- 【每日一包0003】kind-of
github地址:https://github.com/ABCDdouyae... kind-of 判断数据类型用法:kind-of(date)返回:string 数据类型 January undef ...
- CAP 与 注册中心
https://blog.csdn.net/fly910905/article/details/100023415 http://www.ruanyifeng.com/blog/2018/07/cap ...
- std::setw(size)与std::setfill(char)
头文件:#include <iostream>#include <iomanip>using namespace std; 功能: std::setw :需要填充多少个字符, ...
- C# 匿名对象 增加属性
dynamic data = new System.Dynamic.ExpandoObject(); IDictionary<string, object> dictionary = (I ...
- linux 免密码 使用sudo 直接使用root权限执行命令
1.切换到root用户下,怎么切换就不用说了吧,不会的自己百度去. 2.添加sudo文件的写权限,命令是: chmod u+w /etc/sudoers 3.编辑sudoers文件 vi /etc/s ...
- 深度学习笔记(十二)车道线检测 LaneNet
论文:Towards End-to-End Lane Detection: an Instance Segmentation Approach 代码:https://github.com/MaybeS ...
- php curl方法 支持 http https get post cookie
//请求方式curl封装 @author Geyaru QQ 534208139 参数1:访问的URL,参数2:post数据(不填则为GET),参数3:提交的$cookies,参数4:是否返回$coo ...
- Python3.X Selenium 自动化测试中如何截图并保存成功
在selenium for python中主要有三个截图方法,我们挑选其中最常用的一种. 挑最常用的:get_screenshot_as_file() 相关代码如下:(下面的代码可直接复制) # co ...
- 自动化部署脚本--linux执行sh脚本
自动化部署脚本文件目录: 运行主程序:./install.sh #!/bin/bash SCRIPTPATH=$(cd "$(dirname "$0")"; p ...
- LeetCode 120. 三角形最小路径和(Triangle)
题目描述 给定一个三角形,找出自顶向下的最小路径和.每一步只能移动到下一行中相邻的结点上. 例如,给定三角形: [ [2], [3,4], [6,5,7], [4,1,8,3] ] 自顶向下的最小路径 ...