poj 1113 wall(凸包裸题)(记住求线段距离的时候是点积,点积是cos)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 43274 | Accepted: 14716 |
Description
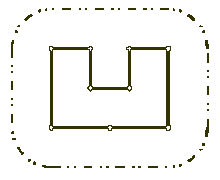
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
Input
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Output
Sample Input
9 100
200 400
300 400
300 300
400 300
400 400
500 400
500 200
350 200
200 200
Sample Output
1628
Hint
#include<iostream>
#include<cmath>
#include<algorithm>
#include<cstdio>
using namespace std; const int MAXN =1010;
const double PI= acos(-1.0);
//精度
double eps=1e-8;
//避免出现-0.00情况,可以在最后加eps
//精度比较
int sgn(double x)
{
if(fabs(x)<=eps)return 0;
if(x<0)return -1;
return 1;
} //点的封装
struct Point
{
double x,y;
Point (){}
//赋值
Point (double _x,double _y)
{
x=_x;
y=_y;
}
//点相减
Point operator -(const Point &b)const
{
return Point (x-b.x,y-b.y);
}
//点积
double operator *(const Point &b)const
{
return x*b.x+y*b.y;
}
//叉积
double operator ^(const Point &b)const
{
return x*b.y-y*b.x;
}
} ; //线的封装
struct Line
{
Point s,e;
Line (){}
Line (Point _s,Point _e)
{
s=_s;
e=_e;
}
//平行和重合判断 相交输出交点
//直线相交和重合判断,不是线段,
Point operator &(const Line &b)const{
Point res=b.s;
if(sgn((e-s)^(b.e-b.s))==0)
{
if(sgn((e-s)^(e-b.e))==0)
{
//重合
return Point(0,0);
}
else
{
//平行
return Point(0,0);
}
}
double t=((e-s)^(s-b.s))/((e-s)^(b.e-b.s));
res.x+=(b.e.x-b.s.x)*t;
res.y+=(b.e.y-b.s.y)*t;
return res;
}
}; //向量叉积
double xmult(Point p0,Point p1,Point p2)
{
return (p0-p1)^(p2-p1);
} //线段和线段非严格相交,相交时true
//此处是线段
bool seg_seg(Line l1,Line l2)
{
return sgn(xmult(l1.s,l2.s,l2.e)*xmult(l1.e,l2.s,l2.e))<=0&&sgn(xmult(l2.s,l1.s,l1.e)*xmult(l2.e,l1.s,l1.e))<=0;
} //两点之间的距离
double dist(Point a,Point b)
{
return sqrt((a-b)*(a-b));
} //极角排序;对100个点进行极角排序
int pos;//极点下标
Point p[MAXN];
int Stack[MAXN],top;
bool cmp(Point a,Point b)
{
double tmp=sgn((a-p[pos])^(b-p[pos]));//按照逆时针方向进行排序
if(tmp==0)return dist(a,p[pos])<dist(b,p[pos]);
if(tmp<0)return false ;
return true;
}
void Graham(int n)
{
Point p0;
int k=0;
p0=p[0];
for(int i=1;i<n;i++)//找到最左下边的点
{
if(p0.y>p[i].y||(sgn(p0.y-p[i].y))==0&&p0.x>p[i].x)
{
p0=p[i];
k=i;
}
}
swap(p[k],p[0]);
sort(p+1,p+n,cmp);
if(n==1)
{
top=2;
Stack[0]=0;
return ;
}
if(n==2)
{
top=2;
Stack[0]=0;
Stack[1]=1;
return ;
}
Stack[0]=0;Stack[1]=1;
top=2;
for(int i=2;i<n;i++)
{
while(top>1&&sgn((p[Stack[top-1]]-p[Stack[top-2]])^(p[i]-p[Stack[top-2]]))<=0)
top--;
Stack[top++]=i;
}
} int main ()
{
int n,l;
cin>>n>>l;
for(int i=0;i<n;i++)
cin>>p[i].x>>p[i].y;
Graham(n);
double sum=0;
for(int i=0;i<top-1;i++)
sum+=dist(p[Stack[i]],p[Stack[i+1]]);
sum+=dist(p[Stack[top-1]],p[Stack[0]]);
sum+=PI*2*l;
sum=(sum)*10/10;
printf("%.f\n",sum);
return 0;
}
poj 1113 wall(凸包裸题)(记住求线段距离的时候是点积,点积是cos)的更多相关文章
- POJ 1113 Wall 凸包 裸
LINK 题意:给出一个简单几何,问与其边距离长为L的几何图形的周长. 思路:求一个几何图形的最小外接几何,就是求凸包,距离为L相当于再多增加上一个圆的周长(因为只有四个角).看了黑书使用graham ...
- poj 1113 Wall 凸包的应用
题目链接:poj 1113 单调链凸包小结 题解:本题用到的依然是凸包来求,最短的周长,只是多加了一个圆的长度而已,套用模板,就能搞定: AC代码: #include<iostream> ...
- POJ 1113 Wall 凸包求周长
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 26286 Accepted: 8760 Description ...
- POJ 1113 - Wall 凸包
此题为凸包问题模板题,题目中所给点均为整点,考虑到数据范围问题求norm()时先转换成double了,把norm()那句改成<vector>压栈即可求得凸包. 初次提交被坑得很惨,在GDB ...
- POJ 1113 Wall(思维 计算几何 数学)
题意 题目链接 给出平面上n个点的坐标.你需要建一个围墙,把所有的点围在里面,且围墙距所有点的距离不小于l.求围墙的最小长度. \(n \leqslant 10^5\) Sol 首先考虑如果没有l的限 ...
- POJ 1087 最大流裸题 + map
A Plug for UNIX Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 15597 Accepted: 5308 ...
- poj 1113:Wall(计算几何,求凸包周长)
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 28462 Accepted: 9498 Description ...
- POJ 1113 Wall 求凸包的两种方法
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 31199 Accepted: 10521 Descriptio ...
- POJ 1113 Wall 求凸包
http://poj.org/problem?id=1113 不多说...凸包网上解法很多,这个是用graham的极角排序,也就是算导上的那个解法 其实其他方法随便乱搞都行...我只是测一下模板... ...
随机推荐
- JVM-03
目录 1.1 新生代垃圾收集器 1.1.1 Serial 垃圾收集器(单线程) 1.1.2 ParNew 垃圾收集器(多线程) 1.1.3 Parallel Scavenge 垃圾收集器(多线程) 2 ...
- Openstack dashboard 仪表盘服务 (八)
Openstack dashboard 仪表盘服务 (八) # 说明: 这个部分将描述如何在控制节点上安装和配置仪表板.dashboard仅在核心服务中要求认证服务.你可以将dashboard与其他服 ...
- wpf 在不同DPI下如何在DrawingVisual中画出清晰的图形
环境Win10 VS2017 .Net Framework4.7.1 本文仅讨论在DrawingVisual中进行的画图. WPF单位,系统DPI,显示器DPI三者的定义及关系 WPF单位:一 ...
- git revert 回退已经push的内容
如题,在日常的开发过程中,可能有组员不小心一下子吧文件修改,需要进行回退 回退主要涉及到2种命令,一种是git reset 一种是 git revert git reset 会修改git log提交历 ...
- 一次snapshot迁移引发的Hbase RIT(hbase2.1.0-cdh6.3.0)
1. 问题起因 通过snapshot做跨集群数据同步时,在执行拷贝脚本里没有指定所有者及所有组,导致clone时没有权限,客户端卡死.master一直报错,经过一系列操作后,导致RIT异常. 2. 异 ...
- ctfhub技能树—文件上传—MIME绕过
什么是MIME MIME(Multipurpose Internet Mail Extensions)多用途互联网邮件扩展类型.是设定某种扩展名的文件用一种应用程序来打开的方式类型,当该扩展名文件被访 ...
- USB充电限流IC,可调到4.8A,输入 6V关闭
随着手机充电电流的提升,和设备的多样化,USB限流芯片就随着需求的增加而越来越多,同时为了更好的保护电子设备,需要进行一路或者多路的负载进行限流. 一般说明 PW1503,PW1502是超低RDS(O ...
- Redis 实战 —— 07. 复制、处理故障、事务及性能优化
复制简介 P61 关系型数据库通常会使用一个主服务器 (master) 向多个从服务器 (slave) 发送更新,并使用从服务器来处理所有读请求. Redis 也采用了同样的方法实现自己的复制特性,并 ...
- JavaScript中的Object类型!
3.4.8 Object 类型 ECMAScript 中的对象其实就是一组数据和功能的集合.对象通过 new 操作符后跟对象类型的名称来创建.开发者可以通过创建 Object 类型的实例来创建自己的对 ...
- LOJ10128. 花神游历各国
花神喜欢步行游历各国,顺便虐爆各地竞赛.花神有一条游览路线,它是线型的,也就是说,所有游历国家呈一条线的形状排列,花神对每个国家都有一个喜欢程度(当然花神并不一定喜欢所有国家). 每一次旅行中,花神会 ...