unity读取灰度图生成三维地形mesh
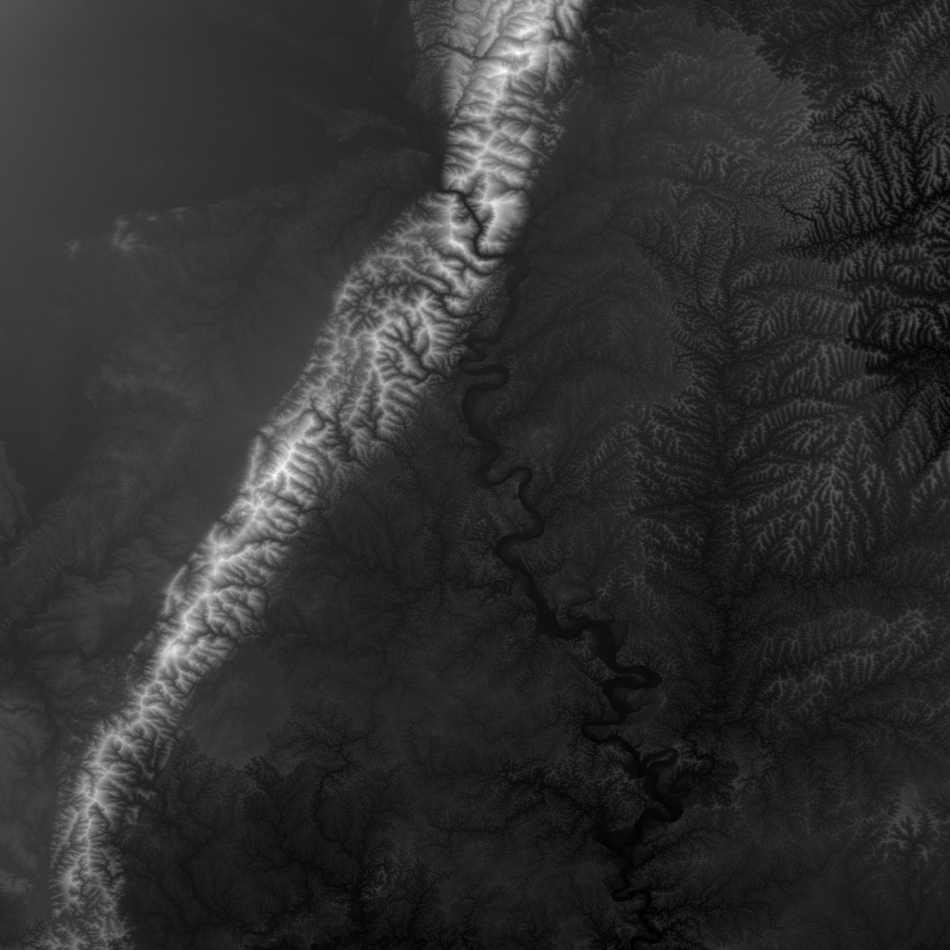
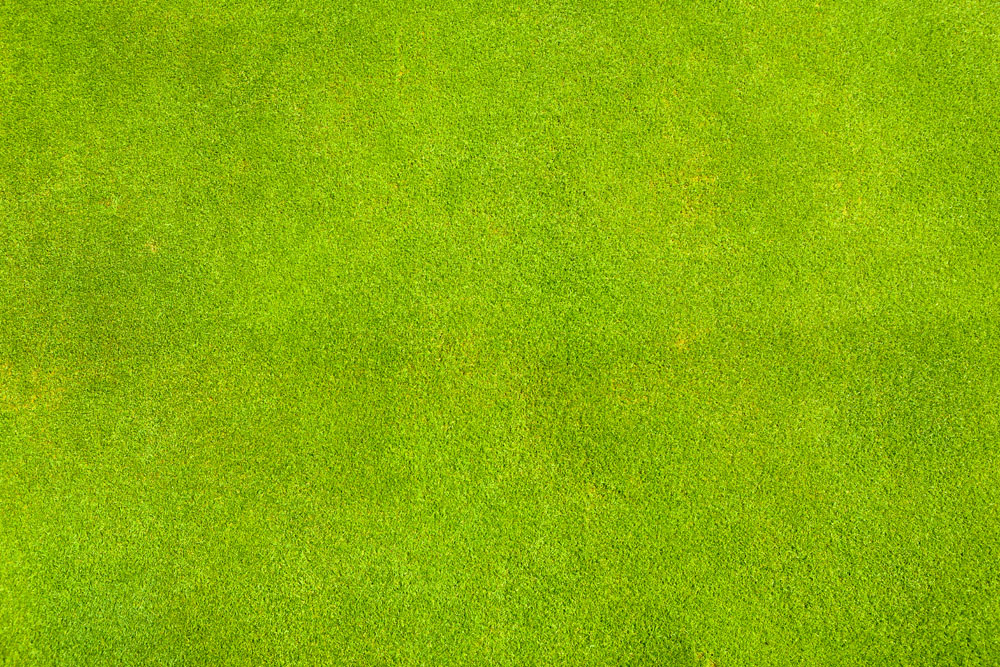
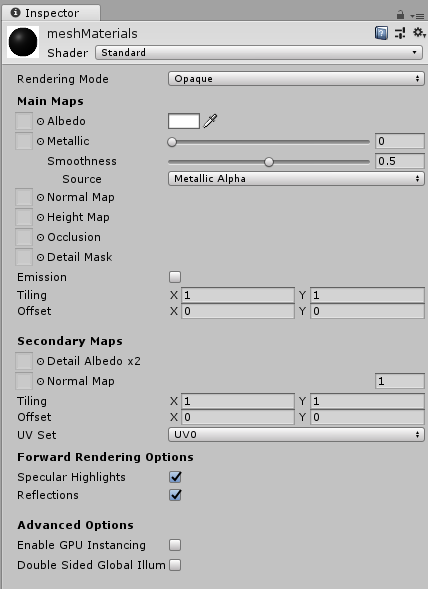
using System.Collections;
using System.Collections.Generic;
using UnityEngine; //根据灰度图创建mesh地形
public class MeshTerrainCreate : MonoBehaviour
{
private Texture textureGray;//灰度图
private Texture textureGrass;//草地贴图
private int tGrayWidth = 0, tGrayHeight = 0;//灰度图的宽和高
private bool bCreate = false;//是否完成创建
private List<GameObject> meshList;//mesh对象集合
private Texture2D texture2dGray;
public float zScale=100;//高度参数 [Tooltip("传入mesh使用的材质")]
public Material meshMaterial; void Start()
{
StartCoroutine(loadImage("IGray.png", (t) => textureGray = t));
StartCoroutine(loadImage("IGrass.jpg", (t) => textureGrass = t));
meshList = new List<GameObject>();
} void Update()
{
if (textureGray != null && textureGrass != null)
{
if (bCreate == false)
{
tGrayWidth = textureGray.width;
tGrayHeight = textureGray.height;
meshMaterial.mainTexture = textureGrass;//设置材质贴图
//mesh顶点数目最大65000,则取mesh顶点为250*250=62500
int xNum = 1+tGrayWidth / 250;//x方向mesh个数
int yNum = 1+tGrayHeight / 250; //y方向mesh个数
texture2dGray = (Texture2D)textureGray;
//根据灰度图创建mesh
for (int i = 0; i <xNum; i++)
{
for (int j = 0; j <yNum; j++)
{
if (i < xNum-1 && j < yNum-1)
{
meshList.Add(
createMesh("meshX" + i.ToString() + "Y" + j.ToString(), 251, 251,
i * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0),
(i + 1) * new Vector3(2500, 0, 0) + (j + 1) * new Vector3(0, 2500, 0)+new Vector3(10,10,0),
i * new Vector2(250, 0) + j * new Vector2(0, 250),
(i + 1) * new Vector2(250, 0) + (j + 1) * new Vector2(0, 250)+new Vector2(1,1)));
}
else if (i == xNum-1 && j < yNum-1)
{
meshList.Add(createMesh("meshX" + i.ToString() + "Y" + j.ToString(), tGrayWidth%250, 251,
i * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0),
i* new Vector3(2500, 0, 0)+new Vector3(10*(tGrayWidth % 250),10,0) + (j + 1) * new Vector3(0, 2500, 0),
i * new Vector2(250, 0) + j * new Vector2(0, 250),
i * new Vector2(250, 0)+new Vector2(tGrayWidth % 250,1) + (j + 1) * new Vector2(0, 250)));
}
else if (i < xNum-1 && j == yNum-1)
{
meshList.Add(createMesh("meshX" + i.ToString() + "Y" + j.ToString(),251, tGrayHeight%250,
i * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0),
(i + 1) * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0)+new Vector3(10,10* (tGrayHeight % 250),0),
i * new Vector2(250, 0) + j * new Vector2(0, 250),
(i + 1) * new Vector2(250, 0) + j * new Vector2(0, 150)+new Vector2(1, tGrayHeight % 250) ) );
}
else if (i == xNum-1 && j == yNum-1)
{
meshList.Add(createMesh("meshX" + i.ToString() + "Y" + j.ToString(),tGrayWidth%250,tGrayHeight%250,
i * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0),
i * new Vector3(2500, 0, 0) + j * new Vector3(0, 2500, 0)+new Vector3(10 * (tGrayWidth % 250), 10 * (tGrayHeight % 250),0),
i * new Vector2(250, 0) + j * new Vector2(0, 250),
i * new Vector2(250, 0) + j * new Vector2(0, 250) +new Vector2(tGrayWidth % 250, tGrayHeight % 250)) );
}
}
}
bCreate = true;
}
}
} //加载图片
IEnumerator loadImage(string imagePath, System.Action<Texture> action)
{
WWW www = new WWW("file://" + Application.streamingAssetsPath + "/" + imagePath);
yield return www;
if (www.error == null)
{
action(www.texture);
}
} /// <summary>
///创建mesh
/// </summary>
/// <param name="meshName">mesh名称</param>
/// <param name="row">行数</param>
/// <param name="col">列数</param>
/// <param name="minPoint">最小点位置</param>
/// <param name="maxPoint">最大点位置</param>
/// <param name="minImgPosition">最小点灰度图位置</param>
/// <param name="maxImgPosition">最大点灰度图位置</param>
/// <returns></returns>
/// private GameObject createMesh(string meshName, int row, int col, Vector3 minPoint, Vector3 maxPoint, Vector2 minImgPosition, Vector2 maxImgPosition)
{
GameObject meshObject = new GameObject(meshName); int verticeNum = row * col;
Vector3[] vertices = new Vector3[verticeNum];//顶点数组大小
int[] triangles = new int[verticeNum * 3 * 2];//三角集合数组,保存顶点索引
// Vector3[] normals = new Vector3[verticeNum];//顶点法线数组大小
Vector2[] uvs = new Vector2[verticeNum];
float rowF = (float)row;
float colF = (float)col;
Vector3 xStep = new Vector3((maxPoint.x - minPoint.x) / rowF, 0, 0);
Vector3 ySetp = new Vector3(0, (maxPoint.y - minPoint.y) / colF, 0);
int k = 0; for (int i = 0; i < row; i++)
{
for (int j = 0; j < col; j++)
{
float tempZ = texture2dGray.GetPixel((int)minImgPosition.x + i, (int)minImgPosition.y + j).grayscale;
vertices[i + j * row] = minPoint + xStep * i + ySetp * j+new Vector3(0,0,tempZ* zScale); uvs[i + j * row] = new Vector2((float)i / rowF, (float)j / colF); if (j < col - 1 && i < row - 1)
{
triangles[k++] = j * row + i;
triangles[k++] = j * row + i + 1;
triangles[k++] = j * row + i + row; triangles[k++] = j * row + i + row;
triangles[k++] = j * row + i + 1;
triangles[k++] = j * row + i + row + 1;
}
}
}
Mesh mesh = new Mesh();
mesh.vertices = vertices;
mesh.triangles = triangles;
// mesh.normals = normals;
mesh.uv = uvs;
mesh.RecalculateBounds();
mesh.RecalculateNormals();
meshObject.AddComponent<MeshFilter>();
meshObject.AddComponent<MeshRenderer>();
meshObject.GetComponent<MeshFilter>().mesh = mesh;
meshObject.GetComponent<MeshRenderer>().material = meshMaterial; return meshObject;
}
}
效果如下

unity读取灰度图生成三维地形mesh的更多相关文章
- ue4读取灰度图生成三维地形mesh
转自:https://www.cnblogs.com/gucheng/p/10116857.html 新建ue c++工程. 在Build.cs中添加"ProceduralMeshCompo ...
- unity 读取灰度图生成三维地形并贴图卫星影像
从 https://earthexplorer.usgs.gov/ 下载高程数据 从谷歌地球上保存对应地区卫星图像 从灰度图创建地形模型,并将卫星影像作为贴图 using System.Collect ...
- opengl读取灰度图生成三维地形并添加光照
转自:https://www.cnblogs.com/gucheng/p/10152889.html 准备第三方库 glew.freeglut.glm.opencv 准备一张灰度图 最终效果 代码如下 ...
- opengl读取灰度图生成三维地形
准备第三方库 glew.freeglut.glm.opencv 准备灰度图片和草地贴图 最终效果 代码包括主程序源文件mainApp.cpp.顶点着色器shader.vs.片元着色器shader.fs ...
- unity 读取灰度图生成按高程分层设色地形模型
准备灰度图 1.高程按比例对应hue色相(hsv)生成mesh效果 o.color = float4(hsv2rgb(float3(v.vertex.y/100.0, 0.5, 0.75)), 1.0 ...
- unity读取灰度图生成等值线图
准备灰度图 grayTest.png,放置于Assets下StreamingAssets文件夹中. 在场景中添加RawImage用于显示最后的等值线图. 生成等值线的过程,使用Marching ...
- blender导入灰度图生成地形模型
安装软件 在此处下载blender并安装. 添加平面 1.打开blender,右键删除初始的立方体. 2.shift+a选择平面添加进场景: 3.按下s键鼠标拖动调节平面大小确定后按下鼠标左键: 4. ...
- unity 读取excel表 生成asset资源文件
做unity 项目也有一段时间了,从unity项目开发和学习中也遇到了很多坑,并且也从中学习到了很多曾经未接触的领域.项目中的很多功能模块,从今天开始把自己的思路和代码奉上给学渣们作为一份学习的资料. ...
- (二)GameMaker:Studio ——使用等高图生成3D地形
上一篇,我们讲解了GM中导入模型的方法,这节我们来讲地形. 源文件地址:http://pan.baidu.com/share/link?shareid=685772423&uk=2466343 ...
随机推荐
- ZZNU-oj-2141:2333--【O(N)求一个数字串能整除3的连续子串的个数,前缀和数组+对3取余组合数找规律】
2141: 2333 题目描述 “别人总说我瓜,其实我一点也不瓜,大多数时候我都机智的一批“ 宝儿姐考察你一道很简单的题目.给你一个数字串,你能判断有多少个连续子串能整除3吗? 输入 多实例输入,以E ...
- java8的相关特性
1,为什么要介绍java8的相关特性? 因为现在的企业大部分已经从java7向java8开始迈进了,用java8的公司越来越多了,java8中的一些知识还是需要了解一些的; java8具有以下特点: ...
- XSS攻击(跨站攻击)
漏洞描述 跨站脚本攻击(Cross-site scripting,简称XSS攻击),通常发生在客户端,可被用于进行隐私窃取.钓鱼欺骗.密码偷取.恶意代码传播等攻击行为.XSS攻击使用到的技术主要为HT ...
- Java集合--Set架构
前面,我们已经系统的对List和Map进行了学习.接下来,我们开始可以学习Set.相信经过Map的了解之后,学习Set会容易很多.毕竟,Set的实现类都是基于Map来实现的(HashSet是通过Has ...
- 7月新的开始 - Axure学习02 - 页面属性、钢笔工具
页面属性 页面属性可以修改整个页面的效果 包含: 属性.对交互用力和事件的编辑 样式.对页面的样式操作 说明.可以对整个页面进行说明.以及样式的说明 钢笔工具:锚点.路径 锚点:钢笔点击之后的点就是锚 ...
- Ubuntu安装依赖文件
我们在安装软件的时候,有时会出现由于依赖的软件没有被安装,会导致软件安装的失败,其实我们可以用命令来安装依赖的软件,这里以Ubuntu为例进行说明. 我在安装wps-office的时候,显示安装成功了 ...
- docker换源
方案一 修改或新增 /etc/docker/daemon.json # vi /etc/docker/daemon.json { "registry-mirrors": [&quo ...
- Cannot modify resource set without a write transaction 问题
TransactionalEditingDomainImpl editingDomain = (TransactionalEditingDomainImpl) diagramEditor.getEdi ...
- DisplayModeProvider完成移动开发自动视图解析
MVC中新建视图命名:XXX.cshtml.XXX.mobile.cshtml:用手机访问会自动到xxx.mobile.cshtml 一.原理 MVC中是通过DisplayModeProvider实现 ...
- 【题解】P3069 [USACO13JAN]牛的阵容Cow Lineup-C++
题目传送门 思路这道题目可以通过尺取法来完成 (我才不管什么必须用队列)什么是尺取法呢?顾名思义,像尺子一样取一段,借用挑战书上面的话说,尺取法通常是对数组保存一对下标,即所选取的区间的左右端点,然后 ...