Spring boot官方文档学习(一)
个人说明:本文内容都是从为知笔记上复制过来的,样式难免走样,以后再修改吧。另外,本文可以看作官方文档的选择性的翻译(大部分),以及个人使用经验及问题。
- 内置Servlet Container
- 使用Spring Boot
- 安装Spring Boot CLI
- 开发一个简单的Spring Boot应用--使用最原始的方式
- Dependency Management
- Starters
- 自动配置
- Spring Beans 和 依赖注入(略)
- @SpringBootApplication
- 运行Spring Boot Application
- Developer tools
- 生产打包
Name Servlet Version Java Version Tomcat 8
3.1
Java 7+
Tomcat 7
3.0
Java 6+
Jetty 9.3
3.1
Java 8+
Jetty 9.2
3.1
Java 7+
Jetty 8
3.0
Java 6+
Undertow 1.3
3.1
Java 7+
此外,你仍然可以部署Spring Boot项目到任何兼容Servlet3.0+的容器。
org.springframework.boot
groupId
。
- <?xml version="1.0" encoding="UTF-8"?>
- <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>com.example</groupId>
- <artifactId>myproject</artifactId>
- <version>0.0.1-SNAPSHOT</version>
- <!-- Inherit defaults from Spring Boot -->
- <parent>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-parent</artifactId>
- <version>1.4.0.RELEASE</version>
- </parent>
- <!-- Add typical dependencies for a web application -->
- <dependencies>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-web</artifactId>
- </dependency>
- </dependencies>
- <!-- Package as an executable jar -->
- <build>
- <plugins>
- <plugin>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-maven-plugin</artifactId>
- </plugin>
- </plugins>
- </build>
- </project>


- <?xml version="1.0" encoding="UTF-8"?>
- <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>com.example</groupId>
- <artifactId>myproject</artifactId>
- <version>0.0.1-SNAPSHOT</version>
- <parent>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-parent</artifactId>
- <version>1.4.0.RELEASE</version>
- </parent>
- <!-- Additional lines to be added here... -->
- </project>

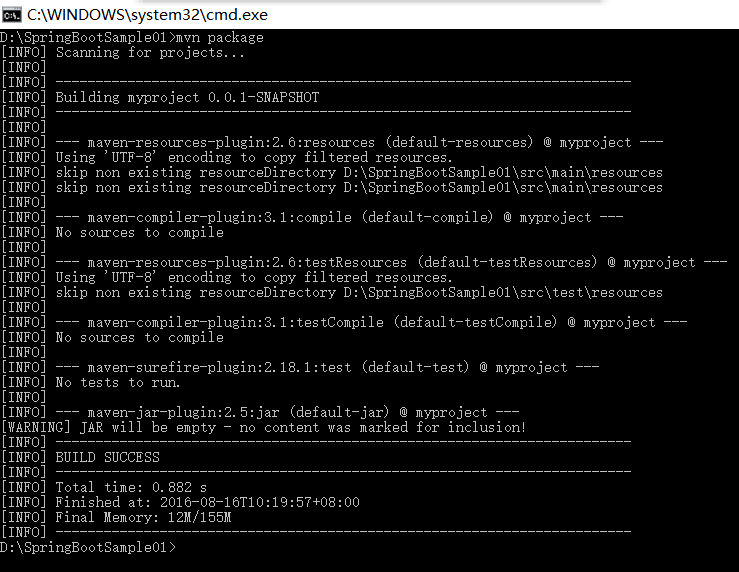
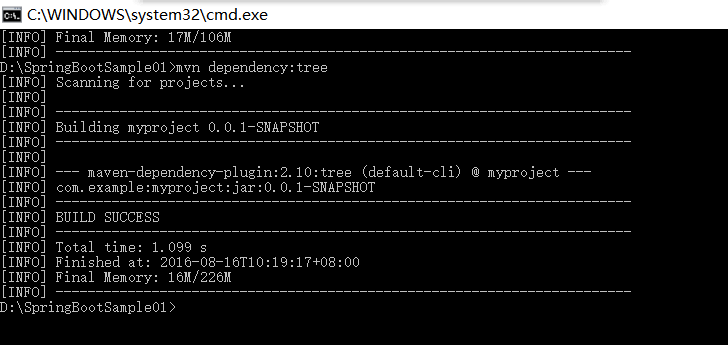

- <dependencies>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-starter-web</artifactId>
- </dependency>
- </dependencies>

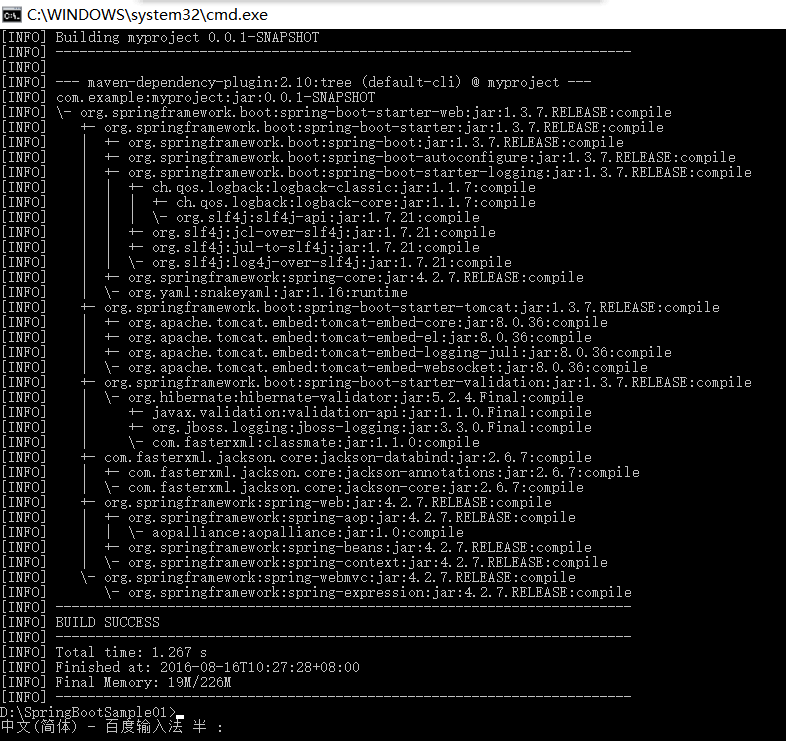

- import org.springframework.boot.*;
- import org.springframework.boot.autoconfigure.*;
- import org.springframework.stereotype.*;
- import org.springframework.web.bind.annotation.*;
- @RestController
- @EnableAutoConfiguration
- public class Example {
- @RequestMapping("/")
- String home() {
- return "Hello World!";
- }
- public static void main(String[] args) throws Exception {
- SpringApplication.run(Example.class, args);
- }
- }


- <build>
- <plugins>
- <plugin>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-maven-plugin</artifactId>
- </plugin>
- </plugins>
- </build>

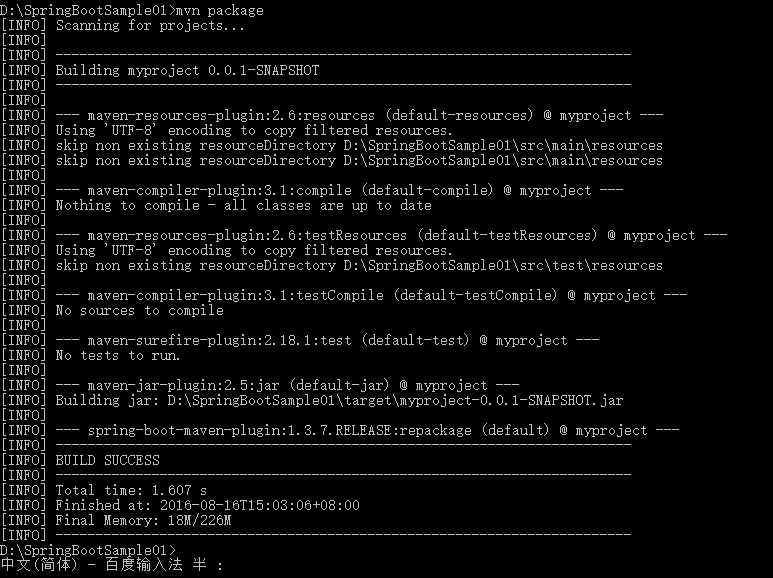
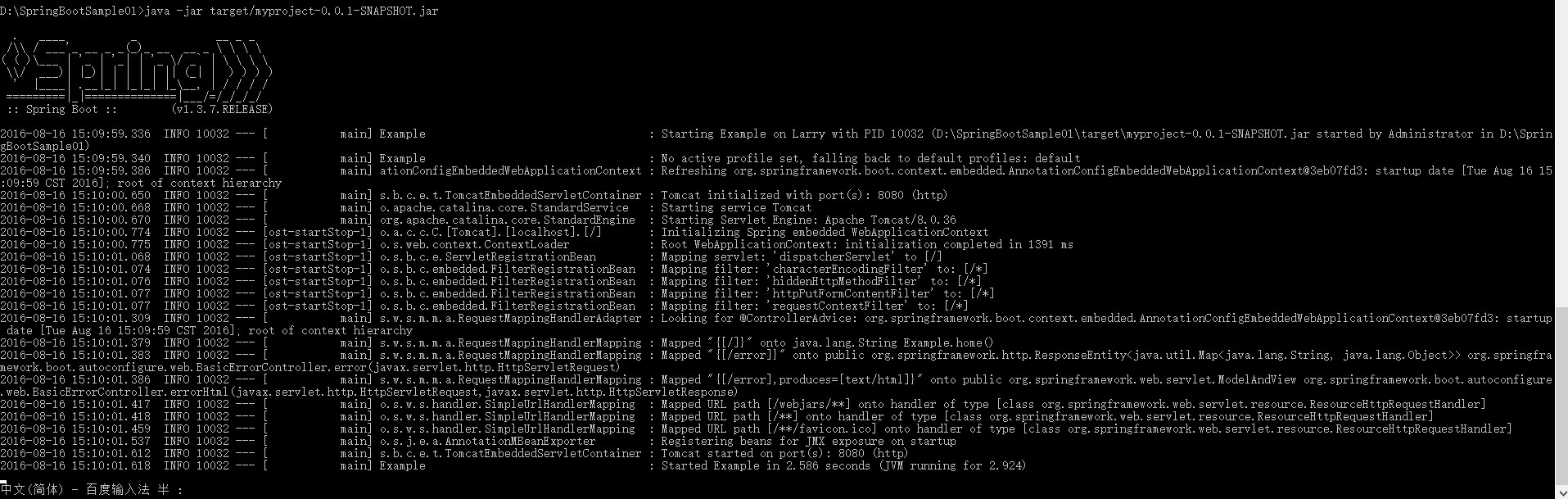
- <properties>
- <spring-data-releasetrain.version>Fowler-SR2</spring-data-releasetrain.version>
- </properties>
- <!-- 使用 java 1.8 -->
- <java.version>1.8</java.version>

- <dependencyManagement>
- <dependencies>
- <dependency>
- <!-- Import dependency management from Spring Boot -->
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-dependencies</artifactId>
- <version>1.4.0.RELEASE</version>
- <type>pom</type>
- <scope>import</scope>
- </dependency>
- </dependencies>
- </dependencyManagement>


- <dependencyManagement>
- <dependencies>
- <!-- Override Spring Data release train provided by Spring Boot -->
- <dependency>
- <groupId>org.springframework.data</groupId>
- <artifactId>spring-data-releasetrain</artifactId>
- <version>Fowler-SR2</version>
- <scope>import</scope>
- <type>pom</type>
- </dependency>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-dependencies</artifactId>
- <version>1.4.0.RELEASE</version>
- <type>pom</type>
- <scope>import</scope>
- </dependency>
- </dependencies>
- </dependencyManagement>


- <build>
- <plugins>
- <plugin>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-maven-plugin</artifactId>
- </plugin>
- </plugins>
- </build>


- import org.springframework.boot.autoconfigure.*;
- import org.springframework.boot.autoconfigure.jdbc.*;
- import org.springframework.context.annotation.*;
- @Configuration
- @EnableAutoConfiguration(exclude={DataSourceAutoConfiguration.class})
- public class MyConfiguration {
- }

@Configuration
, @EnableAutoConfiguration
and @ComponentScan
。
- java -Xdebug -Xrunjdwp:server=y,transport=dt_socket,address=8000,suspend=n -jar target/myproject-0.0.1-SNAPSHOT.jar
mvn spring-boot:run

- <dependencies>
- <dependency>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-devtools</artifactId>
- <optional>true</optional>
- </dependency>
- </dependencies>


- <build>
- <plugins>
- <plugin>
- <groupId>org.springframework.boot</groupId>
- <artifactId>spring-boot-maven-plugin</artifactId>
- <configuration>
- <fork>true</fork>
- </configuration>
- </plugin>
- </plugins>
- </build>

- spring.devtools.restart.exclude=static/**,public/**
- public static void main(String[] args) {
- System.setProperty("spring.devtools.restart.enabled", "false");
- SpringApplication.run(MyApp.class, args);
- }
- restart.include.companycommonlibs=/mycorp-common-[\\w-]+\.jar
- restart.include.projectcommon=/mycorp-myproj-[\\w-]+\.jar
- spring.devtools.reload.trigger-file=.reloadtrigger
- spring.devtools.remote.secret=mysecret
Select Run -> Run Configurations...
Create a new Java Application "launch configuration"
Browse for the my-app project
Use org.springframework.boot.devtools.RemoteSpringApplication as the main class.
Add https://myapp.cfapps.io to the Program arguments (or whatever your remote URL is).
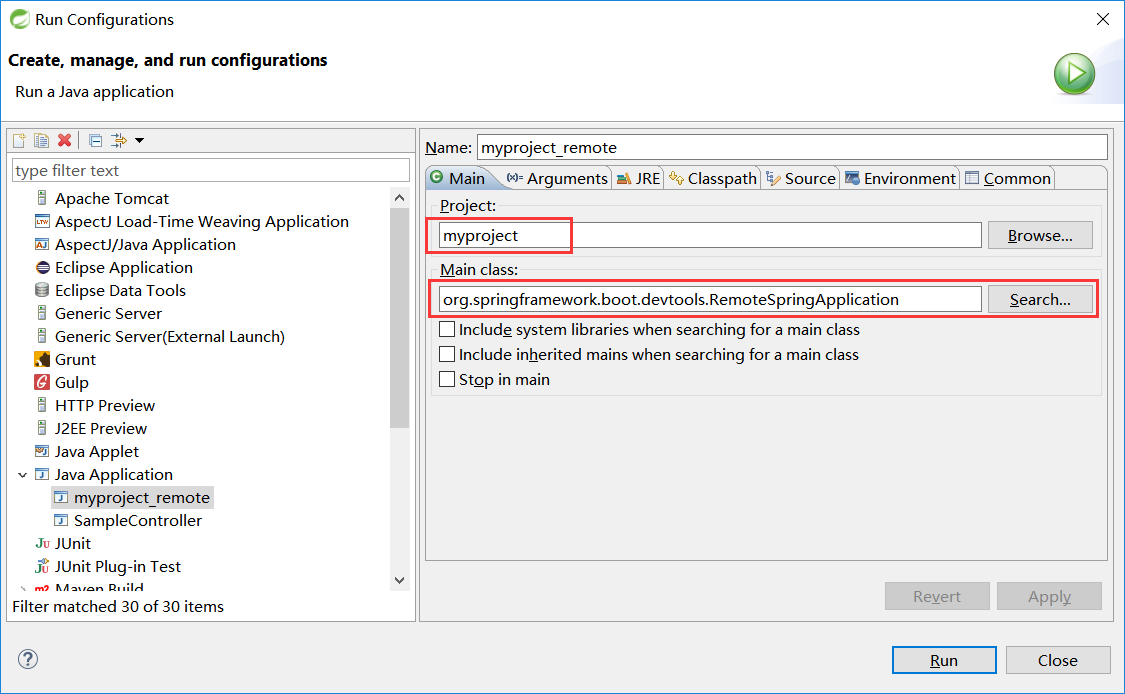
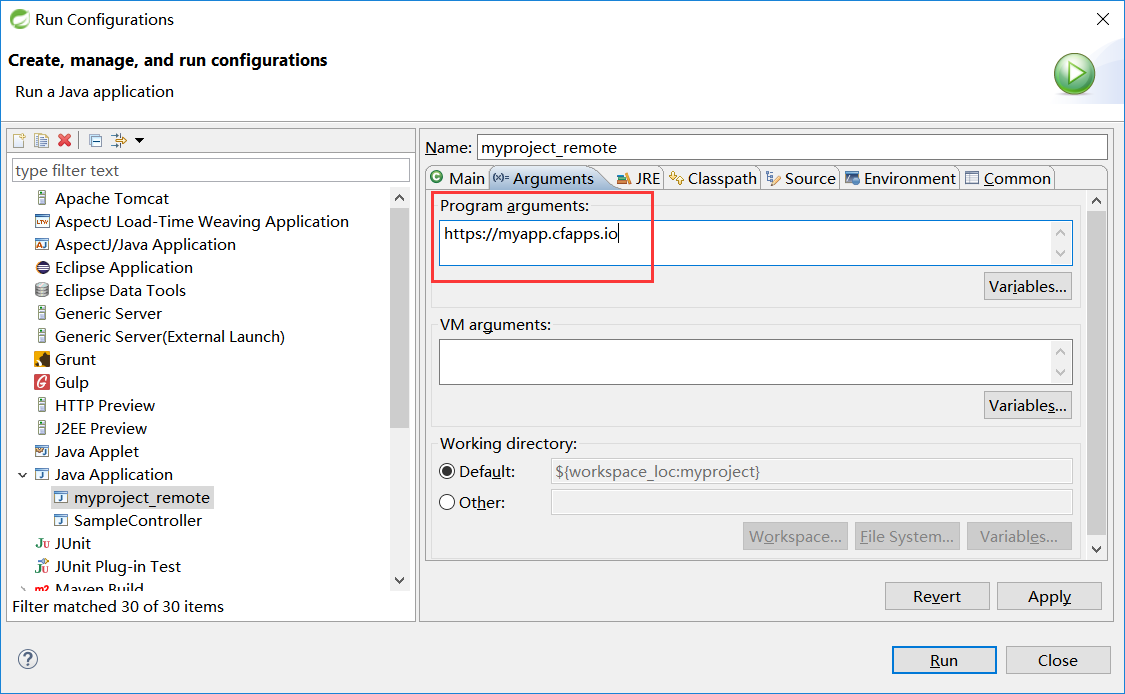
- ---
- env:
- JAVA_OPTS: "-Xdebug -Xrunjdwp:server=y,transport=dt_socket,suspend=n"
Spring boot官方文档学习(一)的更多相关文章
- Spring Boot 官方文档学习(一)入门及使用
个人说明:本文内容都是从为知笔记上复制过来的,样式难免走样,以后再修改吧.另外,本文可以看作官方文档的选择性的翻译(大部分),以及个人使用经验及问题. 其他说明:如果对Spring Boot没有概念, ...
- 20191106 Spring Boot官方文档学习(1-2)
学习内容相关信息 最新版本:2.2.0 CURRENT GA 官网地址 官方文档地址 单页版文档地址 代码生成网址 2.入门 Spring Boot的主要目标是: 为所有Spring开发提供更快且入门 ...
- 20191127 Spring Boot官方文档学习(9.1-9.3)
9."使用方法"指南 9.1.Spring Boot应用程序 9.1.1.创建自己的FailureAnalyzer FailureAnalyzer被包装在FailureAnalys ...
- 20191110 Spring Boot官方文档学习(3)
3.使用Spring Boot 3.1.构建系统 建议选择Maven或Gradle作为构建工具 每个Spring Boot版本都提供了它所支持的依赖关系的精选列表.实际上,您不需要为构建配置中的所有这 ...
- 20191128 Spring Boot官方文档学习(9.4-9.8)
9.4.Spring MVC Spring Boot有许多启动器包含Spring MVC.请注意,一些启动器包括对Spring MVC的依赖,而不是直接包含它. 9.4.1.编写JSON REST服务 ...
- 20191128 Spring Boot官方文档学习【目录】
Spring Boot文档 入门 使用Spring Boot 3.1. 构建系统 3.2. 结构化代码 3.3. 配置类 3.4. 自动配置 3.5. Spring beans和依赖注入 3.6. 使 ...
- 20191128 Spring Boot官方文档学习(9.11-9.17)
9.11.消息传递 Spring Boot提供了许多包含消息传递的启动器.本部分回答了将消息与Spring Boot一起使用所引起的问题. 9.11.1.禁用事务JMS会话 如果您的JMS代理不支持事 ...
- 20191110 Spring Boot官方文档学习(4.2)
4.2.外部化配置 Spring Boot使您可以外部化配置,以便可以在不同环境中使用相同的应用程序代码.您可以使用Properties文件,YAML文件,环境变量和命令行参数来外部化配置.属性值可以 ...
- 20191114 Spring Boot官方文档学习(4.7)
4.7.开发Web应用程序 Spring Boot非常适合于Web应用程序开发.您可以使用嵌入式Tomcat,Jetty,Undertow或Netty创建独立的HTTP服务器.大多数Web应用程序都使 ...
随机推荐
- 第二百八十四节,MySQL数据库-MySQL触发器
MySQL数据库-MySQL触发器 对某个表进行[增/删/改]操作的前后如果希望触发某个特定的行为时,可以使用触发器,触发器用于定制用户对表的行进行[增/删/改]前后的行为. 1.创建触发器基本语法 ...
- map用法小例子
一. Map< Key , Value > m_Eg; 一般赋值表示成: TypeElem value; m_Eg[key] = value; 或 m_Eg.insert(make_ ...
- db2 over()
说起 DB2 在线分析处理,可以用很好很强大来形容.这项功能特别适用于各种统计查询,这些查询用通常的SQL很难实现,或者根本就无发实现.首先,我们从一个简单的例子开始,来一步一步揭开它神秘的面纱,请看 ...
- delphi中设置listview行高的方法
第一步.在form中放置一个ImageList: 第二步.将ListView的SmallImages设置为第一步中放置的ImageList: 第三部.将imageList的height设置成自己需要的 ...
- [转]五分钟看懂UML类图与类的关系详解
在画类图的时候,理清类和类之间的关系是重点.类的关系有泛化(Generalization).实现(Realization).依赖(Dependency)和关联(Association).其中关联又分为 ...
- mysql数据库中,查看数据库的字符集(所有库的字符集或者某个特定库的字符集)
需求描述: mysql中,想要查看某个数据库的字符集.通过information_schma模式下的schemata表来查询 环境描述: mysql版本:5.7.21-log 操作过程: 1.查看in ...
- java 正则表达式验证
package com.fsti.icop.util.regexp; import java.util.regex.Matcher; import java.util.regex.Pattern; p ...
- mybatis由浅入深day02_3一对多查询
3 一对多查询 3.1 需求(查询订单及订单明细的信息) 查询订单及订单明细的信息. 3.2 sql语句 确定主查询表:订单表 确定关联查询表:订单明细表 在一对一查询基础上添加订单明细表关联即可. ...
- 【RF库Collections测试】Get Dictionary Items
Name:Get Dictionary ItemsSource:Collections <test library>Arguments:[ dictionary ]Returns item ...
- C语言各种存储模式的区别?最常用的存储模式有哪些?
DOS用一种段地址结构来编址计算机的内存,每一个物理内存位置都有一个可通过段地址一偏移量的方式来访问的相关地址.为了支持这种段地址结构,大多数C编译程序都允许你用以下6种存储模式来创建程序: ---- ...