Ultra-QuickSort (poj 2002)
Description
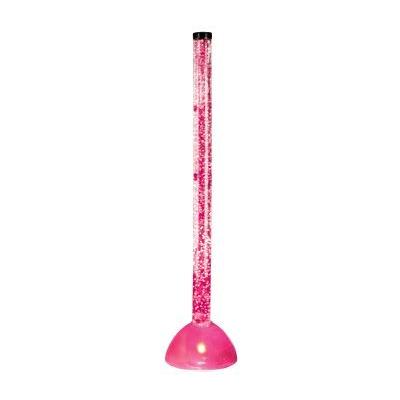
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
这题很简单的样子,就是求冒泡排序的交换次数,but 超时
#include<iostream>
#include<cstring>
#include<cstdio>
using namespace std;
int s[],temp[];
long long cut;//这里害我WA了一次
void merge_sort(int* A,int x,int y,int* T )
{
if(y-x>)
{
int m=x+(y-x)/;//划分
int p=x,q=m,i=x;
merge_sort(A,x,m,T);//递归求解
merge_sort(A,m,y,T);
while(p<m||q<y)
{
if(q>=y||(p<m&&A[p]<=A[q]))
T[i++]=A[p++];//从左半数组复制到临时空间
else
{
T[i++]=A[q++];//从右半数组复制到临时空间
cut+= (m-p);//统计逆序数
}
}
for(i=x; i<y; i++)
A[i]=T[i];//从辅助数组复制回原数组
}
}
int main()
{
int n,i;
while(scanf("%d",&n)&&n)
{
cut=;
for(i=; i<n; i++)
scanf("%d",&s[i]);
merge_sort(s,,n,temp);
printf("%lld\n",cut);
}
return ;
}
#include<iostream>
using namespace std;
long long cnt;
void merge(int array[],int left,int mid,int right)
{
int* temp=new int[right-left+];
int i,j,p;
for(i=left,j=mid+,p=; i<=mid&&j<=right; p++)
{
if(array[i]<=array[j])temp[p]=array[i++];
else temp[p]=array[j++],cnt+=(mid-i+);
}
while(i<=mid)temp[p++]=array[i++];
while(j<=right)temp[p++]=array[j++];
for(i=left,p=; i<=right; i++)array[i]=temp[p++];
delete temp;
}
void mergesort(int array[],int left,int right)
{
if(left==right)array[left]=array[right];
else
{
int mid=(left+right)/;
mergesort(array,left,mid);
mergesort(array,mid+,right);
merge(array,left,mid,right);
}
}
int main()
{
int n,array[];
while(cin>>n&&n)
{
cnt=;
for(int i=; i<n; i++)
cin>>array[i];
mergesort(array,,n-);
cout<<cnt<<endl;
}
return ;
}
下面这个是网上找的还算好懂得,耗时是我敲得那个的10倍左右
Hint
Ultra-QuickSort (poj 2002)的更多相关文章
- 雷达装置 (POJ 1328/ codevs 2625)题解
[问题描述] 假定海岸线是一条无限延伸的直线,陆地在海岸线的一边,大海在另一侧.海中有许多岛屿,每一个小岛我们可以认为是一个点.现在要在海岸线上安装雷达,雷达的覆盖范围是d,也就是说大海中一个小岛能被 ...
- 最后一个非零数字(POJ 1604、POJ 1150、POJ 3406)
POJ中有些问题给出了一个长数字序列(即序列中的数字非常多),这个长数字序列的生成有一定的规律,要求求出这个长数字序列中某个位上的数字是多少.这种问题通过分析,找出规律就容易解决. 例如,N!是一个非 ...
- POJ中和质数相关的三个例题(POJ 2262、POJ 2739、POJ 3006)
质数(prime number)又称素数,有无限个.一个大于1的自然数,除了1和它本身外,不能被其他自然数整除,换句话说就是该数除了1和它本身以外不再有其他的因数:否则称为合数. 最小的质数 ...
- POJ 2002 Squares 解题报告(哈希 开放寻址 & 链式)
经典好题. 题意是要我们找出所有的正方形.1000点,只有枚举咯. 如图,如果我们知道了正方形A,B的坐标,便可以推测出C,D两点的坐标.反之,遍历所有点作为A,B点,看C,D点是否存在.存在的话正方 ...
- (多重背包+记录路径)Charlie's Change (poj 1787)
http://poj.org/problem?id=1787 描述 Charlie is a driver of Advanced Cargo Movement, Ltd. Charlie dri ...
- POJ 2002 统计正方形 HASH
题目链接:http://poj.org/problem?id=2002 题意:给定n个点,问有多少种方法可以组成正方形. 思路:我们可以根据两个点求出对应正方形[有2个一个在两点左边,一个在两点右边] ...
- 种类并查集(POJ 1703)
1703 -- Find them, Catch them http://poj.org/problem?id=1703 题目大意:有2个敌对帮派,输入D a b表示a,b在不同帮派,输入A a b表 ...
- 01背包问题:Charm Bracelet (POJ 3624)(外加一个常数的优化)
Charm Bracelet POJ 3624 就是一道典型的01背包问题: #include<iostream> #include<stdio.h> #include& ...
- Scout YYF I(POJ 3744)
Scout YYF I Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 5565 Accepted: 1553 Descr ...
随机推荐
- 使用dom4j解析xml文件,并封装为javabean对象
dom4j是一个java的XML api,性能优异.功能强大.易于使用.这里使用dom4j对xml文件进行解析,并完成对文件的封装. 实现对xml文件的解析,主要使用到的是dom4j中的SAXRead ...
- 基于Flume的美团日志收集系统(一)架构和设计【转】
美团的日志收集系统负责美团的所有业务日志的收集,并分别给Hadoop平台提供离线数据和Storm平台提供实时数据流.美团的日志收集系统基于Flume设计和搭建而成. <基于Flume的美团日志收 ...
- Linq中Union与Contact方法用法对比
文章一开始,我们来看看下面这个简单的实例. 代码片段1: int[] ints1 = { 2, 4, 9, 3, 0, 5, 1, 7 }; int[] ints2 = { 1, 3, 6, 4, 4 ...
- URAL 1062 - Triathlon(半平面交)
这个题乍眼一看好像很简单,然后我就认为u.v.w只要有全部比另外一个人小的就不能win,否则就能win,但是这个思路只对了一半 不能win的结论是正确的,但是win的结论不止排除这一个条件 将这个人与 ...
- java中for循环的6种写法
有些写法上的说明写的过于武断,可能有很多不当之处,仅供参考. package ForLoop; import java.util.ArrayList; import java.util.Itera ...
- Java异常之try,catch,finally,throw,throws
Java异常之try,catch,finally,throw,throws 你能区分异常和错误吗? 我们每天上班,正常情况下可能30分钟就能到达.但是由于车多,人多,道路拥挤,致使我们要花费更多地时间 ...
- JS实现一键复制功能
var copyClick = function (d) { var Url2 = $(d).parent().parent().find("#copy_value"); Url2 ...
- SQL语句优化(分享)
一.操作符优化 1.IN 操作符 用IN写出来的SQL的优点是比较容易写及清晰易懂,这比较适合现代软件开发的风格.但是用IN的SQL性能总是比较低的,从Oracle执行的步骤来分析用IN的SQL与不用 ...
- 使用strut2要注意的问题
- 单例模式,多种实现方式JAVA
转载请注明出处:http://cantellow.iteye.com/blog/838473 第一种(懒汉,线程不安全): public class Singleton { private stati ...