DP? (hdu3944)
DP?
Time Limit: 10000/3000 MS (Java/Others) Memory Limit: 128000/128000 K (Java/Others)
Total Submission(s): 2871 Accepted Submission(s): 894
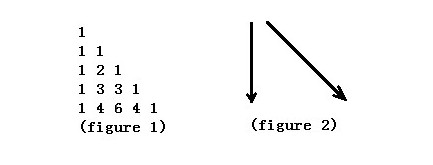
Figure
1 shows the Yang Hui Triangle. We number the row from top to bottom
0,1,2,…and the column from left to right 0,1,2,….If using C(n,k)
represents the number of row n, column k. The Yang Hui Triangle has a
regular pattern as follows.
C(n,0)=C(n,n)=1 (n ≥ 0)
C(n,k)=C(n-1,k-1)+C(n-1,k) (0<k<n)
Write
a program that calculates the minimum sum of numbers passed on a route
that starts at the top and ends at row n, column k. Each step can go
either straight down or diagonally down to the right like figure 2.
As the answer may be very large, you only need to output the answer mod p which is a prime.
to the problem will consists of series of up to 100000 data sets. For
each data there is a line contains three integers n,
k(0<=k<=n<10^9) p(p<10^4 and p is a prime) . Input is
terminated by end-of-file.
every test case, you should output "Case #C: " first, where C indicates
the case number and starts at 1.Then output the minimum sum mod p.
4 2 7
Case #2: 5
1 #include<stdio.h>
2 #include<algorithm>
3 #include<iostream>
4 #include<string.h>
5 #include<stdlib.h>
6 #include<queue>
7 #include<map>
8 #include<math.h>
9 using namespace std;
10 typedef long long LL;
11 LL a[10005];
12 bool prime[10005];
13 int id[10005];
14 int biao[2000][10005];
15 LL quick(LL n,LL m,LL mod);
16 LL lucas(LL n,LL m,LL mod);
17 int main(void)
18 {
19 int i,j,k;
20 LL n,m,mod;
21 for(i=2; i<=1005; i++)
22 if(!prime[i])
23 for(j=i; (i*j)<=10005; j++)
24 prime[i*j]=true;
25 int tk=0;
26 for(i=2; i<=10005; i++)
27 if(!prime[i])
28 {
29 id[i]=tk;
30 biao[tk][0]=1;
31 biao[tk][1]=1;
32 for(j=2; j<i; j++)
33 {
34 biao[tk][j]=biao[tk][j-1]*j%i;
35 }
36 tk++;
37 }
38 int __ca=0;
39 while(scanf("%lld %lld %lld",&n,&m,&mod)!=EOF)
40 {
41 __ca++; LL cc=n-m;
42 n+=1;
43
44 m=min(m,cc);
45 LL ask=lucas(m,n,mod);
46 ask=ask+(n-m-1)%mod;
47 ask%=mod;
48 printf("Case #%d: ",__ca);
49 printf("%lld\n",ask);
50 }
51 return 0;
52 }
53 LL lucas(LL n,LL m,LL mod)
54 {
55 if(m==0)
56 {
57 return 1;
58 }
59 else
60 {
61 LL nn=n%mod;
62 LL mm=m%mod;
63 if(mm<nn)
64 return 0;
65 else
66 {
67 LL ni=biao[id[mod]][mm-nn]*biao[id[mod]][nn]%mod;
68 ni=biao[id[mod]][mm]*quick(ni,mod-2,mod)%mod;
69 return ni*lucas(n/mod,m/mod,mod);
70 }
71 }
72 }
73 LL quick(LL n,LL m,LL mod)
74 {
75 LL ans=1;
76 while(m)
77 {
78 if(m&1)
79 {
80 ans=ans*n%mod;
81 }
82 n=n*n%mod;
83 m/=2;
84 }
85 return ans;
86 }
DP? (hdu3944)的更多相关文章
- HDU-3944 DP?(组合数求模)
一.题目链接 http://acm.hdu.edu.cn/showproblem.php?pid=3944 二.题意 给一个巨大的杨辉三角,采用类似DP入门题“数字三角形”的方式求从顶点$(0, 0) ...
- BZOJ 1911: [Apio2010]特别行动队 [斜率优化DP]
1911: [Apio2010]特别行动队 Time Limit: 4 Sec Memory Limit: 64 MBSubmit: 4142 Solved: 1964[Submit][Statu ...
- 2013 Asia Changsha Regional Contest---Josephina and RPG(DP)
题目链接 http://acm.hdu.edu.cn/showproblem.php?pid=4800 Problem Description A role-playing game (RPG and ...
- AEAI DP V3.7.0 发布,开源综合应用开发平台
1 升级说明 AEAI DP 3.7版本是AEAI DP一个里程碑版本,基于JDK1.7开发,在本版本中新增支持Rest服务开发机制(默认支持WebService服务开发机制),且支持WS服务.RS ...
- AEAI DP V3.6.0 升级说明,开源综合应用开发平台
AEAI DP综合应用开发平台是一款扩展开发工具,专门用于开发MIS类的Java Web应用,本次发版的AEAI DP_v3.6.0版本为AEAI DP _v3.5.0版本的升级版本,该产品现已开源并 ...
- BZOJ 1597: [Usaco2008 Mar]土地购买 [斜率优化DP]
1597: [Usaco2008 Mar]土地购买 Time Limit: 10 Sec Memory Limit: 162 MBSubmit: 4026 Solved: 1473[Submit] ...
- [斜率优化DP]【学习笔记】【更新中】
参考资料: 1.元旦集训的课件已经很好了 http://files.cnblogs.com/files/candy99/dp.pdf 2.http://www.cnblogs.com/MashiroS ...
- BZOJ 1010: [HNOI2008]玩具装箱toy [DP 斜率优化]
1010: [HNOI2008]玩具装箱toy Time Limit: 1 Sec Memory Limit: 162 MBSubmit: 9812 Solved: 3978[Submit][St ...
- px、dp和sp,这些单位有什么区别?
DP 这个是最常用但也最难理解的尺寸单位.它与“像素密度”密切相关,所以 首先我们解释一下什么是像素密度.假设有一部手机,屏幕的物理尺寸为1.5英寸x2英寸,屏幕分辨率为240x320,则我们可以计算 ...
随机推荐
- Oracle-一张表中增加计算某列值重复的次数列,并且把表中其他列也显示出来,或者在显示过程中做一些过滤
总结: 1.计算某列值(数值or字符串)重复的次数 select 列1,count( 列1 or *) count1 from table1 group by 列1 输出的表为:第一列是保留唯一值的 ...
- 【翻译】.NET 6 中的 dotnet monitor
原文:Announcing dotnet monitor in .NET 6 我们在 2020 年 6 月首次推出了dotnet monitor 作为实验工具,并在去年(2020年)努力将其转变为生产 ...
- 巩固javaweb的第二十五天
常用的验证 1. 非空验证 // 验证是否是空 function isNull(str) { if(str.length==0) return true; else return false; } 2 ...
- Angular @ViewChild,Angular 中的 dom 操作
Angular 中的 dom 操作(原生 js) ngAfterViewInit(){ var boxDom:any=document.getElementById('box'); boxDom.st ...
- final&static
final 1.final修饰类,那么该类不能有子类,那么也就没有子类重写父类的方法,也就没有多态 2.final修饰成员变量,那么成员变量要么显式赋值(用第一种),要么在构造方法中赋值 无论哪一种, ...
- java对象分配
1.为什么会有年轻代 我们先来屡屡,为什么需要把堆分代?不分代不能完成他所做的事情么?其实不分代完全可以,分代的唯一理由就是优化GC性能.你先想想,如果没有分代,那我们所有的对象都在一块,GC的时候我 ...
- 在Eclipse中运行OSGI工程出错的解决方案
今天学习OSGI的过程中按照书上所述搭建好第一个helloworld插件工程,运行的过程中出现下面所示的错误: !SESSION 2014-06-09 21:04:49.038 ----------- ...
- @Order注解使用
注解@Order或者接口Ordered的作用是定义Spring IOC容器中Bean的执行顺序的优先级,而不是定义Bean的加载顺序,Bean的加载顺序不受@Order或Ordered接口的影响: @ ...
- idea maven 项目 遇到 "Module not specified" 解决方法
1. 原因:我这边出现的原因是 其他同事在提交代码是 将 这个文件夹也提交了,idea 会加载 .idea 里的配置(即 他的配置),而我的 maven 配置不同,导致出错. 2. 解决方法:删除这 ...
- 【Java多线程】CompletionService
什么是CompletionService? 当我们使用ExecutorService启动多个Callable时,每个Callable返回一个Future,而当我们执行Future的get方法获取结果时 ...