POJ 3522 Slim Span 最小差值生成树
Slim Span
Time Limit: 20 Sec
Memory Limit: 256 MB
题目连接
http://poj.org/problem?id=3522
Description
Given an undirected weighted graph G, you should find one of spanning trees specified as follows.
The graph G is an ordered pair (V, E), where V is a set of vertices {v1, v2, …, vn} and E is a set of undirected edges {e1, e2, …, em}. Each edge e ∈ E has its weight w(e).
A spanning tree T is a tree (a connected subgraph without cycles) which connects all the n vertices with n − 1 edges. The slimness of a spanning tree T is defined as the difference between the largest weight and the smallest weight among the n − 1 edges of T.
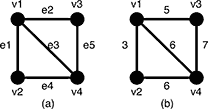
Figure 5: A graph G and the weights of the edges
For example, a graph G in Figure 5(a) has four vertices {v1, v2, v3, v4} and five undirected edges {e1, e2, e3, e4, e5}. The weights of the edges are w(e1) = 3, w(e2) = 5, w(e3) = 6, w(e4) = 6, w(e5) = 7 as shown in Figure 5(b).
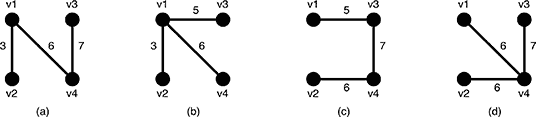
Figure 6: Examples of the spanning trees of G
There are several spanning trees for G. Four of them are depicted in Figure 6(a)~(d). The spanning tree Ta in Figure 6(a) has three edges whose weights are 3, 6 and 7. The largest weight is 7 and the smallest weight is 3 so that the slimness of the tree Ta is 4. The slimnesses of spanning trees Tb, Tc and Td shown in Figure 6(b), (c) and (d) are 3, 2 and 1, respectively. You can easily see the slimness of any other spanning tree is greater than or equal to 1, thus the spanning tree Td in Figure 6(d) is one of the slimmest spanning trees whose slimness is 1.
Your job is to write a program that computes the smallest slimness.
Input
The input consists of multiple datasets, followed by a line containing two zeros separated by a space. Each dataset has the following format.
n | m | |
a1 | b1 | w1 |
⋮ | ||
am | bm | wm |
Every input item in a dataset is a non-negative integer. Items in a line are separated by a space. n is the number of the vertices and m the number of the edges. You can assume 2 ≤ n ≤ 100 and 0 ≤ m ≤ n(n − 1)/2. ak and bk (k = 1, …, m) are positive integers less than or equal to n, which represent the two vertices vak and vbk connected by the kth edge ek. wk is a positive integer less than or equal to 10000, which indicates the weight of ek. You can assume that the graph G = (V, E) is simple, that is, there are no self-loops (that connect the same vertex) nor parallel edges (that are two or more edges whose both ends are the same two vertices).
Output
For each dataset, if the graph has spanning trees, the smallest slimness among them should be printed. Otherwise, −1 should be printed. An output should not contain extra characters.
Sample Input
4 5
1 2 3
1 3 5
1 4 6
2 4 6
3 4 7
4 6
1 2 10
1 3 100
1 4 90
2 3 20
2 4 80
3 4 40
2 1
1 2 1
3 0
3 1
1 2 1
3 3
1 2 2
2 3 5
1 3 6
5 10
1 2 110
1 3 120
1 4 130
1 5 120
2 3 110
2 4 120
2 5 130
3 4 120
3 5 110
4 5 120
5 10
1 2 9384
1 3 887
1 4 2778
1 5 6916
2 3 7794
2 4 8336
2 5 5387
3 4 493
3 5 6650
4 5 1422
5 8
1 2 1
2 3 100
3 4 100
4 5 100
1 5 50
2 5 50
3 5 50
4 1 150
0 0
Sample Output
1
20
0
-1
-1
1
0
1686
50
HINT
题意
给你一个无向图,然后让你找到一个生成树,使得这棵树最大边减去最小边的差值最小
题解:
跑kruskal,我们枚举最小边之后,我们就可以跑kruskal
由于kruskal是排序之后,贪心去拿的,那么最后加入的边一定是最大边
然后我们注意更新答案就好了
代码
#include<iostream>
#include<stdio.h>
#include<cstring>
#include<algorithm>
using namespace std; #define maxn 100005
struct edge
{
int u,v,w;
};
edge E[maxn];
int fa[maxn];
int n,m;
int ans;
bool cmp(edge a,edge b)
{
return a.w<b.w;
}
int fi(int x)
{
if(x!=fa[x])fa[x]=fi(fa[x]);
return fa[x];
}
int uni(int x,int y)
{
int p = fi(x),q = fi(y);
if(p==q)return ;
fa[q] = p;
return ;
}
void solve()
{
ans = ;
sort(E+,E++m,cmp);
int flag = ;
for(int i=;i<=m;i++)
{
for(int j=;j<=n;j++)
fa[j]=j;
int low = E[i].w,high = E[i].w;
int cnt = ;
uni(E[i].u,E[i].v);
cnt++;
for(int j=i+;j<=m;j++)
{
if(uni(E[j].u,E[j].v))
{
cnt++;
high = max(high,E[j].w);
}
}
if(cnt == n-)
{
flag = ;
ans = min(ans,high - low);
}
}
if(flag == )
ans = -;
}
int main()
{
while(scanf("%d%d",&n,&m)!=EOF)
{
if(n==&&m==)break;
memset(E,,sizeof(E));
for(int i=;i<=n;i++)
fa[i]=i;
for(int i=;i<=m;i++)
scanf("%d%d%d",&E[i].u,&E[i].v,&E[i].w);
solve();
printf("%d\n",ans);
}
}
POJ 3522 Slim Span 最小差值生成树的更多相关文章
- poj 3522 Slim Span (最小生成树kruskal)
http://poj.org/problem?id=3522 Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions ...
- POJ 3522 Slim Span(极差最小生成树)
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 9546 Accepted: 5076 Descrip ...
- POJ 3522 ——Slim Span——————【最小生成树、最大边与最小边最小】
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 7102 Accepted: 3761 Descrip ...
- POJ 3522 - Slim Span - [kruskal求MST]
题目链接:http://poj.org/problem?id=3522 Time Limit: 5000MS Memory Limit: 65536K Description Given an und ...
- POJ 3522 Slim Span
题目链接http://poj.org/problem?id=3522 kruskal+并查集,注意特殊情况比如1,0 .0,1.1,1 #include<cstdio> #include& ...
- POJ 3522 Slim Span 暴力枚举 + 并查集
http://poj.org/problem?id=3522 一开始做这个题的时候,以为复杂度最多是O(m)左右,然后一直不会.最后居然用了一个近似O(m^2)的62ms过了. 一开始想到排序,然后扫 ...
- POJ 3522 Slim Span (Kruskal枚举最小边)
题意: 求出最小生成树中最大边与最小边差距的最小值. 分析: 排序,枚举最小边, 用最小边构造最小生成树, 没法构造了就退出 #include <stdio.h> #include < ...
- POJ 3522 Slim Span 最小生成树,暴力 难度:0
kruskal思想,排序后暴力枚举从任意边开始能够组成的最小生成树 #include <cstdio> #include <algorithm> using namespace ...
- Poj(3522),UVa(1395),枚举生成树
题目链接:http://poj.org/problem?id=3522 Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submis ...
随机推荐
- HDU 3695-Computer Virus on Planet Pandora(ac自动机)
题意: 给一个母串和多个模式串,求模式串在母串后翻转后的母串出现次数的的总和. 分析: 模板题 /*#include <cstdio> #include <cstring> # ...
- HDU5780 gcd (BestCoder Round #85 E) 欧拉函数预处理——分块优化
分析(官方题解): 一点感想: 首先上面那个等式成立,然后就是求枚举gcd算贡献就好了,枚举gcd当时赛场上写了一发O(nlogn)的反演,写完过了样例,想交发现结束了 吐槽自己手速慢,但是发了题解后 ...
- KVO KVC
@interface FoodData : NSObject { NSString * foodName; float foodPrice; } @end ////////////////////// ...
- HTML 表单提交 的简单代码
<form action="check.php" method="post"> 用户名:<input type="text" ...
- ASP.NET开发在JavaScript有中文汉字时出现乱码时简单有效的解决
一般情况在使用ASP.NET开发使用JavaScript有中文汉字时不会出现乱码情况,比如:alert('您看到我了吗?');这样直接输入中文汉字的代码中是不会出现乱码的,如果出现了,一是检查Web. ...
- 在/proc文件系统中增加一个目录hello,并在这个目录中增加一个文件world,文件的内容为hello world
一.题目 编写一个内核模块,在/proc文件系统中增加一个目录hello,并在这个目录中增加一个文件world,文件的内容为hello world.内核版本要求2.6.18 二.实验环境 物理主机:w ...
- .net中的"异步"-手把手带你体验
周二刚过,离5.1小长假还有那么一阵,北京的天气已经开始热起来了.洗完澡,突然想起博客园一位大哥暂称呼元哥吧,当时我写了一篇windows服务的安装教程(http://www.cnblogs.com/ ...
- [Hive - LanguageManual] Create/Drop/Alter -View、 Index 、 Function
Create/Drop/Alter View Create View Drop View Alter View Properties Alter View As Select Version info ...
- openstack 云平台API
aaarticlea/png;base64,iVBORw0KGgoAAAANSUhEUgAABVYAAAKrCAIAAACV8EEMAAAgAElEQVR4nOydeVgUaZ7n/W9299nd7n
- JNI调用测试
有需求使用JNI调用,籍着这个机会按照<Linux下测试Java的JNI(Java Native Interface)>上进行了下测试. 这篇文章记录得很清楚了,对原理未做深入的分析,希望 ...