Building Particle Filters and Particle MCMC in NIMBLE
This example shows how to construct and conduct inference on a state space model using particle filtering algorithms. nimble
currently has versions of the bootstrap filter, the auxiliary particle filter, the ensemble Kalman filter, and the Liu and West filter implemented. Additionally, particle MCMC samplers are available and can be specified for both univariate and multivariate parameters.
Model Creation
## load the nimble library and set seed
library('nimble')
set.seed(1) ## define the model
stateSpaceCode <- nimbleCode({
a ~ dunif(-0.9999, 0.9999)
b ~ dnorm(0, sd = 1000)
sigPN ~ dunif(1e-04, 1)
sigOE ~ dunif(1e-04, 1)
x[1] ~ dnorm(b/(1 - a), sd = sigPN/sqrt((1-a*a)))
y[1] ~ dt(mu = x[1], sigma = sigOE, df = 5)
for (i in 2:t) {
x[i] ~ dnorm(a * x[i - 1] + b, sd = sigPN)
y[i] ~ dt(mu = x[i], sigma = sigOE, df = 5)
}
}) ## define data, constants, and initial values
data <- list(
y = c(0.213, 1.025, 0.314, 0.521, 0.895, 1.74, 0.078, 0.474, 0.656, 0.802)
)
constants <- list(
t = 10
)
inits <- list(
a = 0,
b = .5,
sigPN = .1,
sigOE = .05
) ## build the model
stateSpaceModel <- nimbleModel(stateSpaceCode,
data = data,
constants = constants,
inits = inits,
check = FALSE)
## defining model...
## building model...
## setting data and initial values...
## running calculate on model (any error reports that follow may simply
## reflect missing values in model variables) ...
##
## checking model sizes and dimensions...
## note that missing values (NAs) or non-finite values were found in model
## variables: x, lifted_a_times_x_oBi_minus_1_cB_plus_b. This is not an error,
## but some or all variables may need to be initialized for certain algorithms
## to operate properly.
##
## model building finished.
Construct and run a bootstrap filter
We next construct a bootstrap filter to conduct inference on the latent states of our state space model. Note that the bootstrap filter, along with the auxiliary particle filter and the ensemble Kalman filter, treat the top-level parameters a, b, sigPN
, and sigOE
as fixed. Therefore, the bootstrap filter below will proceed as though a = 0, b = .5, sigPN = .1
, and sigOE = .05
, which are the initial values that were assigned to the top-level parameters.
The bootstrap filter takes as arguments the name of the model and the name of the latent state variable within the model. The filter can also take a control list that can be used to fine-tune the algorithm’s configuration.
## build bootstrap filter and compile model and filter
bootstrapFilter <- buildBootstrapFilter(stateSpaceModel, nodes = 'x')
compiledList <- compileNimble(stateSpaceModel, bootstrapFilter)
## compiling... this may take a minute. Use 'showCompilerOutput = TRUE' to see C++ compiler details.
## compilation finished.
## run compiled filter with 10,000 particles.
## note that the bootstrap filter returns an estimate of the log-likelihood of the model.
compiledList$bootstrapFilter$run(10000)
## [1] -28.13009
Particle filtering algorithms in nimble
store weighted samples of the filtering distribution of the latent states in the mvSamples
modelValues object. Equally weighted samples are stored in the mvEWSamples
object. By default, nimble
only stores samples from the final time point.
## extract equally weighted posterior samples of x[10] and create a histogram
posteriorSamples <- as.matrix(compiledList$bootstrapFilter$mvEWSamples)
hist(posteriorSamples)
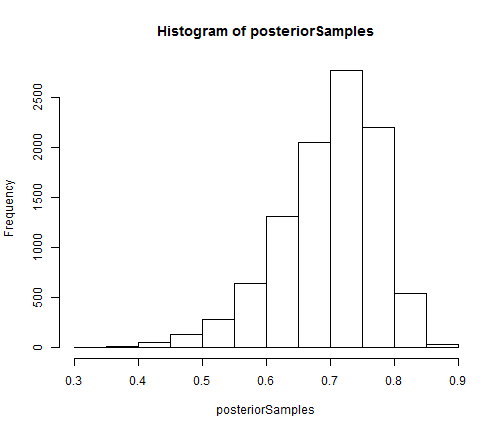
The auxiliary particle filter and ensemble Kalman filter can be constructed and run in the same manner as the bootstrap filter.
Conduct inference on top-level parameters using particle MCMC
Particle MCMC can be used to conduct inference on the posterior distribution of both the latent states and any top-level parameters of interest in a state space model. The particle marginal Metropolis-Hastings sampler can be specified to jointly sample the a, b, sigPN
, and sigOE
top level parameters within nimble
‘s MCMC framework as follows:
## create MCMC specification for the state space model
stateSpaceMCMCconf <- configureMCMC(stateSpaceModel, nodes = NULL) ## add a block pMCMC sampler for a, b, sigPN, and sigOE
stateSpaceMCMCconf$addSampler(target = c('a', 'b', 'sigPN', 'sigOE'),
type = 'RW_PF_block', control = list(latents = 'x')) ## build and compile pMCMC sampler
stateSpaceMCMC <- buildMCMC(stateSpaceMCMCconf)
compiledList <- compileNimble(stateSpaceModel, stateSpaceMCMC, resetFunctions = TRUE)
## compiling... this may take a minute. Use 'showCompilerOutput = TRUE' to see C++ compiler details.
## compilation finished.
## run compiled sampler for 5000 iterations
compiledList$stateSpaceMCMC$run(5000)
## |-------------|-------------|-------------|-------------|
## |-------------------------------------------------------|
## NULL
## create trace plots for each parameter
library('coda')
par(mfrow = c(2,2))
posteriorSamps <- as.mcmc(as.matrix(compiledList$stateSpaceMCMC$mvSamples))
traceplot(posteriorSamps[,'a'], ylab = 'a')
traceplot(posteriorSamps[,'b'], ylab = 'b')
traceplot(posteriorSamps[,'sigPN'], ylab = 'sigPN')
traceplot(posteriorSamps[,'sigOE'], ylab = 'sigOE')
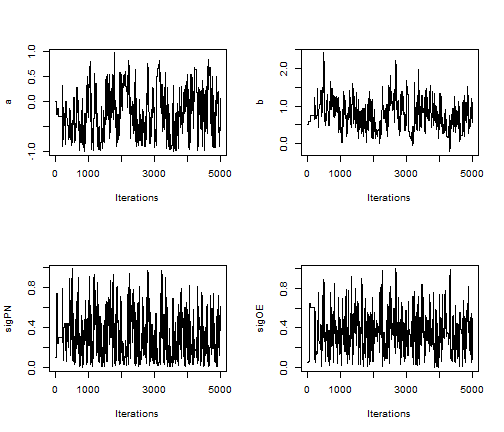
The above RW_PF_block
sampler uses a multivariate normal proposal distribution to sample vectors of top-level parameters. To sample a scalar top-level parameter, use the RW_PF
sampler instead.
转自:https://r-nimble.org/building-particle-filters-and-particle-mcmc-in-nimble-2
Building Particle Filters and Particle MCMC in NIMBLE的更多相关文章
- Particle Filters
|—粒子滤波原理 |—基础代码的建立—|—前进 | |—转弯 | |—噪音(误差 ...
- 基于粒子滤波的物体跟踪 Particle Filter Object Tracking
Video来源地址 一直都觉得粒子滤波是个挺牛的东西,每次试图看文献都被复杂的数学符号搞得看不下去.一个偶然的机会发现了Rob Hess(http://web.engr.oregonstate.edu ...
- Particle filter for visual tracking
Kalman Filter Cons: Kalman filtering is inadequate because it is based on the unimodal Gaussian dist ...
- Particle 粒子效果使用(适配微信小游戏,particle is not defined)
在微信小游戏中使用粒子效果 参考: 1. 粒子库下载地址 2. 粒子官方使用教程 3. 水友解决微信小游戏particle is not defined 一.下载第三方库 Git地址:https:// ...
- Cesium中级教程9 - Advanced Particle System Effects 高级粒子系统效应
Cesium中文网:http://cesiumcn.org/ | 国内快速访问:http://cesium.coinidea.com/ 要了解粒子系统的基础知识,请参见粒子系统入门教程. Weathe ...
- Cesium中级教程8 - Introduction to Particle Systems 粒子系统入门
Cesium中文网:http://cesiumcn.org/ | 国内快速访问:http://cesium.coinidea.com/ What is a particle system? 什么是粒子 ...
- Quick guide for converting from JAGS or BUGS to NIMBLE
Converting to NIMBLE from JAGS, OpenBUGS or WinBUGS NIMBLE is a hierarchical modeling package that u ...
- {ICIP2014}{收录论文列表}
This article come from HEREARS-L1: Learning Tuesday 10:30–12:30; Oral Session; Room: Leonard de Vinc ...
- [SLAM] GMapping SLAM源码阅读(草稿)
目前可以从很多地方得到RBPF的代码,主要看的是Cyrill Stachniss的代码,据此进行理解. Author:Giorgio Grisetti; Cyrill Stachniss http: ...
随机推荐
- PLSQL创建定时任务
在使用oracle最匹配的工具plsql的时候,如果用plsql创建定时器呢?下面我简单介绍使用工具创建定时器的方法: 1.创建任务执行的存储过程,如名称为YxtestJob,向测试表中插入数据 cr ...
- Laravel框架一:原理机制篇
Laravel作为在国内国外都颇为流行的PHP框架,风格优雅,其拥有自己的一些特点.以下是本人一点粗浅的认识,不敢奢求他人同意,更不能一一而足,仅为自己做一点总结而已. 一. 请求周期 Laravel ...
- 【2017-04-21】Ado.Nte属性扩展
通过对数据库表的封装,对该表的属性进行扩展. 1.例如:表中的性别是bool类,要实现显示给用户看的为“男.女” 2.通过表中的生日datetime类,来实现显示给用户看的年月日,自动计算年龄. 3. ...
- 【外文翻译】 为什么我要写 getters 和setters
原文作者: Shamik Mitra 原文链接:https://dzone.com/articles/why-should-i-write-getters-and-setters 当我开始我的java ...
- Android sdk配置 常见问题及处理方法
1. 下载sdk压缩包,解压后显示 2.双击SDK Manager.exe 程序进入如下界面 注:有的童鞋可能遇到如下问题 一般将一和二两种操作都完成就OK了 一. 更新sdk,遇到了更新下载失败问题 ...
- 让你的JS代码更具可读性
一.合理的添加注释 函数和方法--每个函数或方法都应该包含一个注释,描述其目的和用于完成任务所可能使用 的算法.陈述事先的假设也非常重要,如参数代表什么,函数是否有返回值(因为这不能从函 数定义中推断 ...
- SQL Server pivot 行转列遇到的问题
前段时间开发系统时,有个功能是动态加载列,于是就使用了SQL Server自带的PIVOT函数进行行转列,开始用的非常溜,效果非常好.但是提交测试后问题来了,测试添加的列名中包含空格,然后就杯具了,功 ...
- 表单提交音乐文件(php)
利用点空闲时间来写个博客,最近做的项目中需要表单提交音频的,图片的,各种类型,把它存到数据库里,这里先来说一下音乐文件的表单提交吧,后几天再来更新输入数据库的,先看一下效果 点击浏览 就会出来预览,点 ...
- 通过 bootloader 向其传输启动参数
作者:Younger Liu, 本作品采用知识共享署名-非商业性使用-相同方式共享 3.0 未本地化版本许可协议进行许可. Linux提供了一种通过bootloader向其传输启动参数的功能,内核开发 ...
- UBIFS文件系统介绍
1. 引言 UBIFS,Unsorted Block Image File System,无排序区块图像文件系统.它是用于固态硬盘存储设备上,并与LogFS相互竞争,作为JFFS2的后继文件系统之一 ...