golang的interface剖析
type MyInt int
var i int
var j MyInt
var r io.Reader
var r io.Reader
tty, err := os.OpenFile("/dev/tty", os.O_RDWR, 0)
if err != nil {
return nil, err
}
r = tty
到这里,r的类型是什么?r的类型仍然是interface io.Reader,只是r = tty这一句,隐含了一个类型转换,将tty转成了io.Reader。
type Binary uint64
func (i Binary) String() string {
return strconv.Uitob64(i.Get(), 2)
} func (i Binary) Get() uint64 {
return uint64(i)
}
.png)
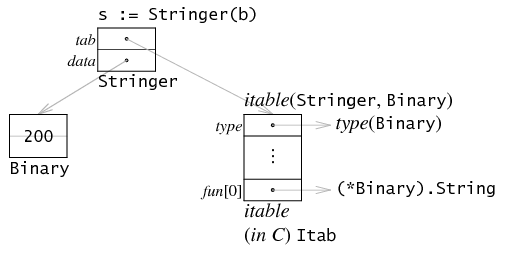
var w io.Writer
switch v := any.(type) {
case int:
return strconv.Itoa(v)
case float:
return strconv.Ftoa(v, 'g', -1)
}
1 var any interface{} // initialized elsewhere
2 s := any.(Stringer) // dynamic conversion
3 for i := 0; i < 100; i++ {
4 fmt.Println(s.String())
5 }
143 type iface struct {
144 tab *itab // 指南itable
145 data unsafe.Pointer // 指向真实数据
146 }
617 type itab struct {
618 inter *interfacetype
619 _type *_type
620 link *itab
621 bad int32
622 unused int32
623 fun [1]uintptr // variable sized
624 }
310 type imethod struct {
311 name nameOff
312 ityp typeOff
313 }
314
315 type interfacetype struct {
316 typ _type
317 pkgpath name
318 mhdr []imethod
319 }
320
28 type _type struct {
29 size uintptr
30 ptrdata uintptr // size of memory prefix holding all pointers
31 hash uint32
32 tflag tflag
33 align uint8
34 fieldalign uint8
35 kind uint8
36 alg *typeAlg
37 // gcdata stores the GC type data for the garbage collector.
38 // If the KindGCProg bit is set in kind, gcdata is a GC program.
39 // Otherwise it is a ptrmask bitmap. See mbitmap.go for details.
40 gcdata *byte
41 str nameOff
42 ptrToThis typeOff
43 }
22 func itabhash(inter *interfacetype, typ *_type) uint32 {
23 // compiler has provided some good hash codes for us.
24 h := inter.typ.hash
25 h += 17 * typ.hash
26 // TODO(rsc): h += 23 * x.mhash ?
27 return h % hashSize
28 }
44 h := itabhash(inter, typ)
45
46 // look twice - once without lock, once with.
47 // common case will be no lock contention.
48 var m *itab
49 var locked int
50 for locked = 0; locked < 2; locked++ {
51 if locked != 0 {
52 lock(&ifaceLock)
53 }
54 for m = (*itab)(atomic.Loadp(unsafe.Pointer(&hash[h]))); m != nil; m = m.link {
55 if m.inter == inter && m._type == typ {
71 return m // 找到了前面计算过的itab
72 }
73 }
74 }
75 // 没有找到,生成一个,并加入到itab的hash中。
76 m = (*itab)(persistentalloc(unsafe.Sizeof(itab{})+uintptr(len(inter.mhdr)-1)*sys.PtrSize, 0, &memstats.other_sys))
77 m.inter = inter
78 m._type = typ
79 additab(m, true, canfail)
13 const (
14 hashSize = 1009
15 )
16
17 var (
18 ifaceLock mutex // lock for accessing hash
19 hash [hashSize]*itab
20 )
92 // both inter and typ have method sorted by name,
93 // and interface names are unique,
94 // so can iterate over both in lock step;
95 // the loop is O(ni+nt) not O(ni*nt). // 按name排序过的,因此这里的匹配只需要O(ni+nt)
99 j := 0
100 for k := 0; k < ni; k++ {
101 i := &inter.mhdr[k]
102 itype := inter.typ.typeOff(i.ityp)
103 name := inter.typ.nameOff(i.name)
104 iname := name.name()
109 for ; j < nt; j++ {
110 t := &xmhdr[j]
111 tname := typ.nameOff(t.name)
112 if typ.typeOff(t.mtyp) == itype && tname.name() == iname {
118 if m != nil {
119 ifn := typ.textOff(t.ifn)
120 *(*unsafe.Pointer)(add(unsafe.Pointer(&m.fun[0]), uintptr(k)*sys.PtrSize)) = ifn // 找到匹配,将实际类型的方法填入itab的fun
121 }
122 goto nextimethod
123 }
124 }
125 }
135 nextimethod:
136 }
140 h := itabhash(inter, typ) //插入上面的全局hash
141 m.link = hash[h]
142 atomicstorep(unsafe.Pointer(&hash[h]), unsafe.Pointer(m))
143 }
到这里,interface的数据结构的框架。
golang的interface剖析的更多相关文章
- Golang 源码剖析:log 标准库
Golang 源码剖析:log 标准库 原文地址:Golang 源码剖析:log 标准库 日志 输出 2018/09/28 20:03:08 EDDYCJY Blog... 构成 [日期]<空格 ...
- Golang的Interface是个什么鬼
问题概述 Golang的interface,和别的语言是不同的.它不需要显式的implements,只要某个struct实现了interface里的所有函数,编译器会自动认为它实现了这个interfa ...
- golang 关于 interface 的学习整理
Golang-interface(四 反射) go语言学习-reflect反射理解和简单使用 为什么在Go语言中要慎用interface{} golang将interface{}转换为struct g ...
- Golang 的 `[]interface{}` 类型
Golang 的 []interface{} 类型 我其实不太喜欢使用 Go 语言的 interface{} 类型,一般情况下我宁愿多写几个函数:XxxInt, XxxFloat, XxxString ...
- Golang接口(interface)三个特性(译文)
The Laws of Reflection 原文地址 第一次翻译文章,请各路人士多多指教! 类型和接口 因为映射建设在类型的基础之上,首先我们对类型进行全新的介绍. go是一个静态性语言,每个变量都 ...
- Golang-interface(四 反射)
github:https://github.com/ZhangzheBJUT/blog/blob/master/reflect.md 一 反射的规则 反射是程序执行时检查其所拥有的结构.尤其是类型的一 ...
- golang之interface
一.概述 接口类型是对 "其他类型行为" 的抽象和概况:因为接口类型不会和特定的实现细节绑定在一起:很多面向对象都有类似接口概念,但Golang语言中interface的独特之处在 ...
- golang将interface{}转换为struct
项目中需要用到golang的队列,container/list,需要放入的元素是struct,但是因为golang中list的设计,从list中取出时的类型为interface{},所以需要想办法把i ...
- Golang-interface(二 接口与nil)
github: https://github.com/ZhangzheBJUT/blog/blob/master/nil.md 一 接口与nil 前面解说了go语言中接口的基本用法,以下将说一说nil ...
随机推荐
- 从angularjs传递参数至Web API
昨天分享的博文<angularjs呼叫Web API>http://www.cnblogs.com/insus/p/7772022.html,只是从Entity获取数据,没有进行参数POS ...
- 2018 Multi-University Training Contest 2 部分简单题解析
Preface 多校第二场,依靠罚时优势打到了校内的Rank 2 暴力分块碾标算系列 T4 Game 题目大意:在一个数集\([1,n]\)中两个人轮流选择其中的一个数,并从数集中删去这个数所有约数. ...
- [Spark][Hive]外部文件导入到Hive的例子
外部文件导入到Hive的例子: [training@localhost ~]$ cd ~[training@localhost ~]$ pwd/home/training[training@local ...
- Git常用命令梳理
在日常的Git版本库管理工作中用到了很多操作命令,以下做一梳理: 查看分支列表,带有*的分支表示是当前所在分支 [root@115~~]#git branch 查看分支详细情况 (推荐这种方式) [r ...
- sigar开发(java)
下载sigar,地址:https://yunpan.cn/cBEWbEfdAm98f (提取码:f765) 可以收集的信息 CPU信息:包括基本信息(vendor.model.mhz.cacheSiz ...
- SQL基础语句总结
前言: SQL 是用于访问和处理数据库的标准的计算机语言. 什么是 SQL? SQL 指结构化查询语言SQL 使我们有能力访问数据库SQL 是一种 ANSI 的标准计算机语言编者注:ANSI,美国国家 ...
- Linux实践一:问题及解决
安装ubuntu出现的问题 : 打开镜像.iso文件,v-box好像是不识别这种格式的,它识别的好像是.vdi等格式,所以要用vm虚拟机打开镜像安装 打开镜像,按照步骤安装后,安装很久后,出现问题.初 ...
- git体会
刘仙臣个人github链接:http://www.github.com/liuxianchen 这次作业学会了关于git的一些基本操作,学习了到了许多东西,为以后的学习奠定了基础,激发了学习的兴趣.具 ...
- TCP报文格式详解
TCP报文是TCP层传输的数据单元,也叫报文段. 1.端口号:用来标识同一台计算机的不同的应用进程. 1)源端口:源端口和IP地址的作用是标识报文的返回地址. 2)目的端口:端口指明接收方计算机上的应 ...
- windows、ubuntu、centos7下mysql 的安装与使用
一.windows 及ubuntu下安装 windows可以傻瓜式安装,另一种空闲了下来写,也不麻烦 ubuntu: apt-get install mysql 强烈推荐使用ubuntu从这儿就很方便 ...