22.Generate Parentheses[M]括号生成
题目
Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.
For example, given n=3, a solution set is:
[
"( ( ( ) ) )",
"( ( ) ( ) )",
"( ( ) ) ( )",
"( ) ( ( ) )",
"( ) ( ) ( )"
]
思路
回溯法
对于\(n\)对有效括号的生成,我们可以将其看成以下的方式:
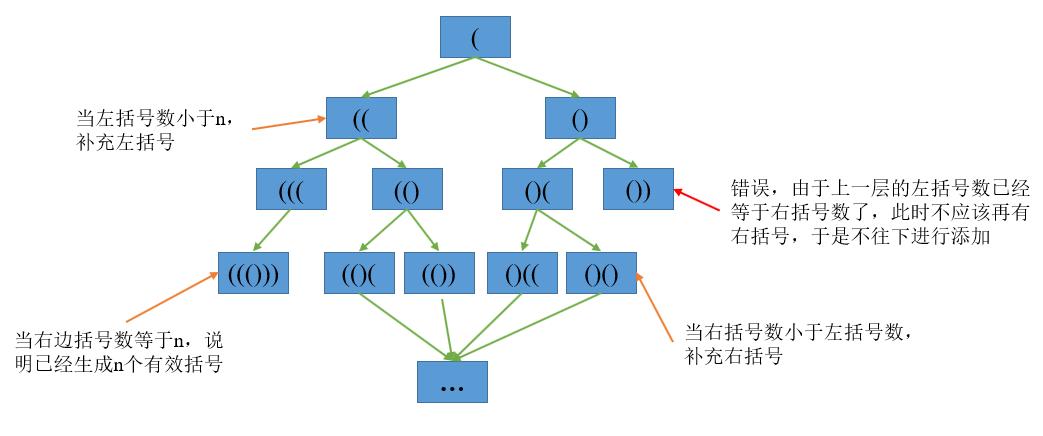
图1:回溯法生成括号示意图
在上图中,由于一对有效括号总是从"("开始,所以树的根节点是"("。将左括号的个数记为$l$,右括号的个数记为$r$,给定个数$n$,在生成新括号的过程中,分为三种情况
* $l r$,说明右括号的个数不够多,应该生成右括号
* $r = n$,说明完成$n$对有效括号的生成。
注意在此过程中,右括号的个数不能超过左括号,如果超过,则不往下进行递归。由此完成了一个回溯法的过程:递归生成括号,但是在生成括号的同时,检查左右括号是否匹配。如果匹配,则继续递归;如果不匹配,则不往下递归。在具体实现中,通过保证右边括号的个数\(r\)始终小于等于左边括号的个数来实现匹配的检查。
Tips
回溯法
基本思想
将问题的解空间转化为图或者树的结构表示,然后利用深度优先搜索策略进行遍历,遍历过程中记录和寻找可行解和最优解。
基本行为
回溯法的基本行为是搜索,在搜索过程中利用两种方法来避免无效的搜索
- 1.使用约束函数,剪去不满足约束条件的路径
- 2.使用限定条件,剪去不能得到最优解的路径
回溯法是一种思想方法,在具体实现中是通过递归或者迭代实现。
C++
vector<string> generateParenthesis(int n) {
vector<string> result;
if(n==0)
return result;
backTrack(result, "", 0, 0, n);
return result;
}
void backTrack(vector<string> &res,string curStr,int l, int r, int n){
if(r == n)
res.push_back(curStr);
//如果左括号没有达到给定的n
if(l < n)
backTrack(res, curStr+"(", l+1, r, n);
//如果右括号数目不够
if(r < l)
backTrack(res, curStr+")", l, r+1, n);
}
Python
参考
[1]
[2] https://blog.csdn.net/zjc_game_coder/article/details/78520742
22.Generate Parentheses[M]括号生成的更多相关文章
- LeetCode OJ:Generate Parentheses(括号生成)
Given n pairs of parentheses, write a function to generate all combinations of well-formed parenthes ...
- [Leetcode][Python]22: Generate Parentheses
# -*- coding: utf8 -*-'''__author__ = 'dabay.wang@gmail.com' 22: Generate Parentheseshttps://oj.leet ...
- 刷题22. Generate Parentheses
一.题目说明 这个题目是22. Generate Parentheses,简单来说,输入一个数字n,输出n对匹配的小括号. 简单考虑了一下,n=0,输出"";n=1,输出" ...
- 22. Generate Parentheses(ML)
22. Generate Parentheses . Generate Parentheses Given n pairs of parentheses, write a function to ge ...
- 【LeetCode】22. Generate Parentheses 括号生成
作者: 负雪明烛 id: fuxuemingzhu 个人博客:http://fuxuemingzhu.cn/ 个人公众号:负雪明烛 本文关键词:括号, 括号生成,题解,leetcode, 力扣,Pyt ...
- 【LeetCode】22. Generate Parentheses (2 solutions)
Generate Parentheses Given n pairs of parentheses, write a function to generate all combinations of ...
- 22. Generate Parentheses (recursion algorithm)
Given n pairs of parentheses, write a function to generate all combinations of well-formed parenthes ...
- [LeetCode] 22. Generate Parentheses 生成括号
Given n pairs of parentheses, write a function to generate all combinations of well-formed parenthes ...
- [leetcode]22. Generate Parentheses生成括号
Given n pairs of parentheses, write a function to generate all combinations of well-formed parenthes ...
随机推荐
- NET MVC FileResult 导出/下载 文件/Excel
参考http://www.cnblogs.com/ldp615/archive/2010/09/17/asp-net-mvc-file-result.html 1.引入NPOI 2.代码 using ...
- springboot 多数据源的实现
相关的依赖 yml配置 java配置类: DataSourceConfigurerjava /** * Created by zhiqi.shao on 2017/11/20. */ @Configu ...
- Bootstrap 模态框(Modal)带参数传值实例
模态框(Modal)是覆盖在父窗体上的子窗体.通常,目的是显示来自一个单独的源的内容,可以在不离开父窗体的情况下有一些互动.子窗体可提供信息.交互等. 为了实现父窗体与其的交互,通常需要向其传值,实现 ...
- Python学习教程(Python学习视频_Python学些路线):Day06 函数和模块的使用
Python学习教程(Python学习视频_Python学些路线):函数和模块的使用 在讲解本章节的内容之前,我们先来研究一道数学题,请说出下面的方程有多少组正整数解. $$x_1 + x_2 + x ...
- luogu P1856 [USACO5.5]矩形周长Picture 扫描线 + 线段树
Code: #include<bits/stdc++.h> #define maxn 200007 #define inf 100005 using namespace std; void ...
- ZooKeeper 运维经验
转自:http://www.juvenxu.com/2015/03/20/experiences-on-zookeeper-ops/ ZooKeeper 运维经验 ZooKeeper 是分布式环境下非 ...
- proc程序中使用PLSQL、Exception 、 动态SQL(day08)
. proc中如何使用plsql 1.1 使用plsql的语法 exec sql execute begin /* 相当于plsql的匿名块 */ end; end-exec; 在预编译时,需要加如下 ...
- JDK环境变量设置(linux)
1.下载jdk1.8版本软件包 2.解压 tar -zxvf jdk1.8.tar.gz mv jdk1.8 /usr/local/ 3.添加环境变量 vim /etc/profile 在文件底部加入 ...
- mysql查询表里的重复数据
先贴个简单的SQL语句 select username,count(*) as count from hk_test group by username having count>1; 使用详情 ...
- C#关键字的个人理解与注释
C#关键字注释:abstract:抽象as:类型转换(返回转换结果)base:基类bool:布尔类型break:条件中断语句byte:字节case:条件语句catch:异常捕获后执行char:16 位 ...