Ajax打开三种页面的请求
xmlhttprequest对象可以打开两种方式的页面请求
<webServices >
<protocols >
<add name ="HttpGet"/>
</protocols>
</webServices>
2,在WebSerVice.asmx文件中写一个方法getResult,该方法接受两个字符型的参数,其中的WebMethod属性不可以漏掉。。。
{
[WebMethod]
public string getResult(string str1,string str2)
{
return "输入的第一个字符串是:" + str1 + "\n输入的第二个字符串是:" + str2;
}
}
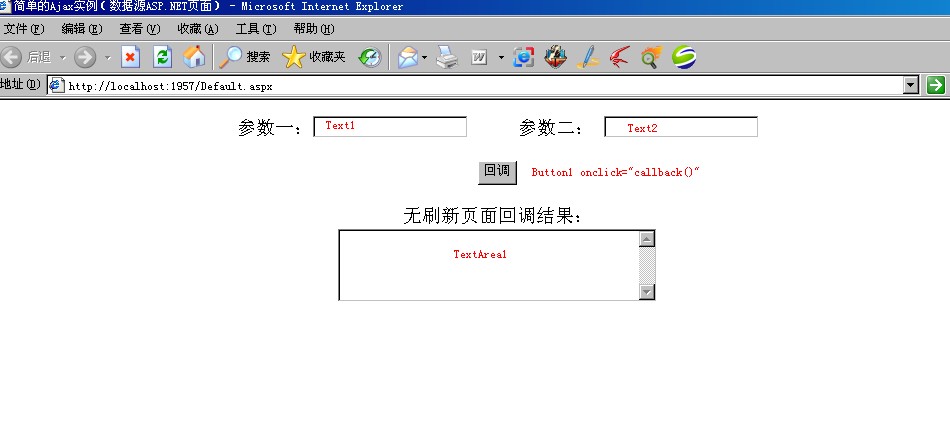
//以下为创建xmlHttpRequest对象
var xmlHttpRequest=false;
try
{
xmlHttpRequest=new XMLHttpRequest();//非IE浏览器
}
catch (microsoft)//IE浏览器
{
try
{
xmlHttpRequest=new ActiveXObject("Msxml2.XMLHTTP");
}
catch (othermicrosoft)
{
try
{
xmlHttpRequest=new ActiveXObject("Microsoft.XMLHTTP");
}
catch (failed)
{
xmlHttpRequest=false;
}
}
}
if (!xmlHttpRequest)
{
alert("不能初始化XMLHttpRequest对象!");
}
//相应button的Click事件
function callback()
{
var arg1=document.getElementById ("Text1").value;//获取Text1文本框的值
var arg2=document.getElementById ("Text2").value;//获取Text2文本框的值
var url="WebService.asmx/getResult?str1="+escape(arg1)+"&str2="+escape(arg2);//要打开的url地址,并传递两个参数,这里参数名必须同webservice提供的参数名一致
xmlHttpRequest.open("get",url,true);//以get方式打开指定的url请求,并且使用的是异步调用方式(true)
xmlHttpRequest.onreadystatechange=updatePage;//指定回调函数updatePage
xmlHttpRequest.send(null);//发送请求,由于是get方式,这里用null
}
//回调函数
function updatePage()
{
if (xmlHttpRequest.readyState==4)
{
if (xmlHttpRequest.status==200)
{
var response=xmlHttpRequest.responseXML;//以xml格式回调内容
var result;
if (response.evaluate)//XML Parsing in Mozilla
{
result=response.evaluate("//text()",response,null,XpathResult.STRING_TYPE,null).stringValue;//Mozilla中获取xml中的文本内容
}
else
{
//XML Parsing in IE
result=response.selectSingleNode("//text()").data;//IE中获取xml中的文本内容
}
document.getElementById ("TextArea1").value=result;
}
}
}
</script>
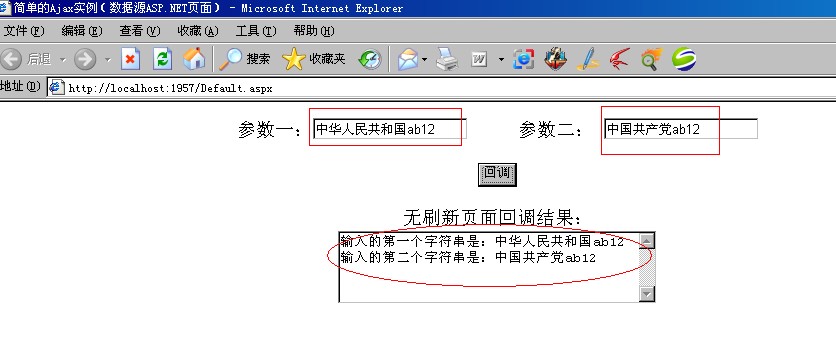
Code highlighting produced by Actipro CodeHighlighter (freeware)
http://www.CodeHighlighter.com/
-->protected void Page_Load(object sender, EventArgs e)
{
string str1 = Request["argA"].ToString();
string str2 = Request["argB"].ToString();
Response.Write("输入的第一个字符串是:" + str1 + "\n输入的第二个字符串是:" + str2);
}
//以下为创建xmlHttpRequest对象
var xmlHttpRequest=false;
try
{
xmlHttpRequest=new XMLHttpRequest();//非IE浏览器
}
catch (microsoft)//IE浏览器
{
try
{
xmlHttpRequest=new ActiveXObject("Msxml2.XMLHTTP");
}
catch (othermicrosoft)
{
try
{
xmlHttpRequest=new ActiveXObject("Microsoft.XMLHTTP");
}
catch (failed)
{
xmlHttpRequest=false;
}
}
}
if (!xmlHttpRequest)
{
alert("不能初始化XMLHttpRequest对象!");
}
//相应button的Click事件
function callback()
{
var arg1=document.getElementById ("Text1").value;//获取Text1文本框的值
var arg2=document.getElementById ("Text2").value;//获取Text2文本框的值
var url="ReturnStr.aspx?argA="+escape(arg1)+"&argB="+escape(arg2);//要打开的url地址
xmlHttpRequest.open("get",url,true);//以get方式打开指定的url请求,并且使用的是异步调用方式(true)
xmlHttpRequest.onreadystatechange=updatePage;//指定回调函数updatePage
xmlHttpRequest.send(null);//发送请求,由于是get方式,这里用null
}
//回调函数
function updatePage()
{
if (xmlHttpRequest.readyState==4)
{
if (xmlHttpRequest.status==200)
{
var response=xmlHttpRequest.responsetext;//回调的内容,以文本的方式返回,当然也可以以xml方式返回(写法为xmlHttpRequest.responseXML)
// alert(response);//这里返回的不仅有文本,还有诸如.aspx文件中的各种标签
// var result=response.split('<')[0];//所以这里要使用split来取文本内容
var res=response.split('<');
var result=res[0];
document.getElementById ("TextArea1").value=result;
}
}
}
</script>
{
if (Request["argA"]!=null && Request["argB"]!=null)//这个判断一定要加上
{
string str1 = Request["argA"].ToString();//获取客户端发送过来的参数的值
string str2 = Request["argB"].ToString();
Response.Write("输入的第一个字符串是:" + str1 + "\n输入的第二个字符串是:" + str2);
}
}
//以下为创建xmlHttpRequest对象
var xmlHttpRequest=false;
try
{
xmlHttpRequest=new XMLHttpRequest();//非IE浏览器
}
catch (microsoft)//IE浏览器
{
try
{
xmlHttpRequest=new ActiveXObject("Msxml2.XMLHTTP");
}
catch (othermicrosoft)
{
try
{
xmlHttpRequest=new ActiveXObject("Microsoft.XMLHTTP");
}
catch (failed)
{
xmlHttpRequest=false;
}
}
}
if (!xmlHttpRequest)
{
alert("不能初始化XMLHttpRequest对象!");
}
//相应button的Click事件
function callback()
{
var arg1=document.getElementById ("Text1").value;//获取Text1文本框的值
var arg2=document.getElementById ("Text2").value;//获取Text2文本框的值
var url="Default.aspx?argA="+escape(arg1)+"&argB="+escape(arg2);//要打开的url地址,并传递两个参数
// var url="ReturnStr.aspx?argA="+escape(arg1)+"&argB="+escape(arg2);
// var url="WebService.asmx/getResult?str1="+escape(arg1)+"&str2="+escape(arg2);//要打开的url地址,并传递两个参数,这里参数名必须同webservice提供的参数名一致
xmlHttpRequest.open("get",url,true);//以get方式打开指定的url请求,并且使用的是异步调用方式(true)
xmlHttpRequest.onreadystatechange=updatePage;//指定回调函数updatePage
xmlHttpRequest.send(null);//发送请求,由于是get方式,这里用null
}
//回调函数
function updatePage()
{
if (xmlHttpRequest.readyState==4)
{
if (xmlHttpRequest.status==200)
{
var response=xmlHttpRequest.responsetext;//回调的内容,以文本的方式返回,当然也可以以xml方式返回(写法为xmlHttpRequest.responseXML)
// alert(response);//这里返回的不仅有文本,还有诸如.aspx文件中的各种标签
// var result=response.split('<')[0];//所以这里要使用split来取文本内容
var res=response.split('<');
var result=res[0];
document.getElementById ("TextArea1").value=result;
// var response=xmlHttpRequest.responseXML;//以xml格式回调内容
// var result;
// if (response.evaluate)//XML Parsing in Mozilla
// {
// result=response.evaluate("//text()",response,null,XpathResult.STRING_TYPE,null).stringValue;//Mozilla中获取xml中的文本内容
// }
// else
// {
// //XML Parsing in IE
// result=response.selectSingleNode("//text()").data;//IE中获取xml中的文本内容
// }
// document.getElementById ("TextArea1").value=result;
}
}
}
</script>
Ajax打开三种页面的请求的更多相关文章
- Ajax的三种实现及JSON解析
本文为学习笔记,属新手文章,欢迎指教!! 本文主要是比较三种实现Ajax的方式,为以后的学习开个头. 准备: 1. prototype.js 2. jquery1.3.2.min.js 3. j ...
- MVC异步AJAX的三种方法(JQuery的Get方法、JQuery的Post方法和微软自带的异步方法)
异步是我们在网站开发过程中必不可少的方法,MVC框架的异步方法也有很多,这里介绍三种方法: 一.JQuery的Get方法 view @{ Layout = null; } <!DOCTYPE h ...
- ajax 使用 三种方法 设置csrf_token的装饰器
1. CSRF中间件 CSRF跨站请求伪造 2. 补充两个装饰器 from django.views.decorators.csrf import csrf_exempt, csrf_prote ...
- [Web 前端] 006 css 三种页面引入的方法
1. 外链式 用法 step 1: 在 html 文档的 head 头部分写入下方这句话 <link rel="stylesheet" href="./xxx.cs ...
- Jquery Ajax处理,服务端三种页面aspx,ashx,asmx的比较
常规的Jquery Ajax 验证登录,主要有3种服务端页面相应 ,也就是 aspx,ashx,asmx即webserivice . 下面分别用3种方式来(aspx,ashx,asmx)做服务端来处理 ...
- web三种跨域请求数据方法
以下测试代码使用php,浏览器测试使用IE9,chrome,firefox,safari <!DOCTYPE HTML> <html> <head> < ...
- ajax 的三种使用方法
第一种 也是最古老的一种方法之一 from 表单直接提交数据到php文件里 action为路径 <form method="post" action="./inde ...
- AJax的三种响应
AJax的响应 1.普通文本方式(字符串) resp.getWriter().print("你好"); 2.JSON格式当要给前台页面传输 集合或者对象时 使用普通文本传输的时St ...
- CSS——三种页面引入方法
目的:为了把样式和内容分开,并且使网页元素更加丰富,引入了CSS CSS页面引入有三种方式: 1)内联式:比较不常用,因为内容和样式仍然在一起,不方便.示例: <!DOCTYPE html> ...
随机推荐
- HDU 1425 sort hash+加速输入
http://acm.hdu.edu.cn/showproblem.php?pid=1425 题目大意: 给你n个整数,请按从大到小的顺序输出其中前m大的数. 其中n和m都是位于[-500000,50 ...
- log4cxx入门篇
log4cxx入门篇 先看官网:http://logging.apache.org/log4cxx/index.html 转载自:http://wenku.baidu.com/view/d88 ...
- 【55.70%】【codeforces 557A】Ilya and Diplomas
time limit per test1 second memory limit per test256 megabytes inputstandard input outputstandard ou ...
- java数组10大技巧
0. 声明一个数组(Declare an array) String[] aArray = new String[5]; String[] bArray = {"a"," ...
- ConcurrentLinkedQueue使用方法
它是一个基于链接节点的无界线程安全队列.该队列的元素遵循先进先出的原则.头是最先加入的,尾是最近加入的. 插入元素是追加到尾上.提取一个元素是从头提取.当多个线程共享访问一个公共 collection ...
- pandas 学习(五)—— datetime(日期)
date range pd.date_range('2014-11-19', '2014-11-21', freq='D') # 起始时间,终止时间,时间间隔,也即步长,D ⇒ Day,5H:以 5 ...
- Android 节日短信送祝福(功能篇:2-短信历史记录Fragment的编写)
因为用于展示短信记录的是一个ListView,但是为了方便,可以直接继承自ListFragment,就可以免去写ListView对应的布局了,只需要写其item对应的布局即可. item_sended ...
- 小雷FansUnion:我有了第一个付费客户(第一个徒弟)
很高兴地告诉大家一个振奋人心的消息,我刚刚拥有了第一个付费客户. 第一个付费客户是山东青岛的一个上班族,有2年.Net经验,今年转Java开发.对我比较信任,在我的建议下,选择了"拜师学艺& ...
- 以Graphicslayer为管理组来管理Element.
转自原文 以Graphicslayer为管理组来管理Element. 前言 在AE开发过程中,我们经常使用Element(元素).它的出现让地图与用户之间的交互增加了不少的效果.在地图上,可以通过各种 ...
- [React Native] Installing and Linking Modules with Native Code in React Native
Learn to install JavaScript modules that include native code. Some React Native modules include nati ...