POJ 1463 Strategic game(二分图最大匹配)
Description
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
For example for the tree:
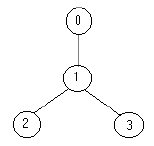
Input
- the number of nodes
- the description of each node in the following format node_identifier:(number_of_roads) node_identifier1 node_identifier2 ... node_identifiernumber_of_roads or node_identifier:(0)
The node identifiers are integer numbers between 0 and n-1, for n nodes (0 < n <= 1500);the number_of_roads in each line of input will no more than 10. Every edge appears only once in the input data.
Output
题目大意:给一棵树,求最小顶点覆盖(即用最小的点覆盖所有的边)。
思路:DP靠边。树肯定是二分图无误,在二分图中,最小顶点覆盖=最大匹配,直接匈牙利跑出答案。
代码(375MS):
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
using namespace std; const int MAXN = ;
const int MAXE = MAXN << ; int head[MAXN];
int to[MAXE], next[MAXE];
int n, ecnt; void init() {
memset(head, , sizeof(head));
ecnt = ;
} void add_edge(int u, int v) {
to[ecnt] = v; next[ecnt] = head[u]; head[u] = ecnt++;
to[ecnt] = u; next[ecnt] = head[v]; head[v] = ecnt++;
} int link[MAXN], dep[MAXN];
bool vis[MAXN]; bool dfs(int u) {
for(int p = head[u]; p; p = next[p]) {
int &v = to[p];
if(vis[v]) continue;
vis[v] = true;
if(!~link[v] || dfs(link[v])) {
link[v] = u;
return true;
}
}
return false;
} int main() {
while(scanf("%d", &n) != EOF) {
init();
memset(dep, , sizeof(dep));
for(int i = ; i < n; ++i) {
int a, b, c;
scanf("%d:(%d)", &a, &b);
while(b--) {
scanf("%d", &c);
dep[c] = dep[a] + ;
add_edge(a, c);
}
}
int ans = ;
memset(link, , sizeof(link));
for(int i = ; i < n; ++i) {
if(dep[i] & ) continue;
memset(vis, , sizeof(vis));
if(dfs(i)) ++ans;
}
printf("%d\n", ans);
}
}
POJ 1463 Strategic game(二分图最大匹配)的更多相关文章
- POJ - 1422 Air Raid 二分图最大匹配
题目大意:有n个点,m条单向线段.如今问要从几个点出发才干遍历到全部的点 解题思路:二分图最大匹配,仅仅要一条匹配,就表示两个点联通,两个点联通仅仅须要选取当中一个点就可以,所以有多少条匹配.就能够减 ...
- POJ 3041 Asteroids(二分图最大匹配)
###题目链接### 题目大意: 给你 N 和 K ,在一个 N * N 个图上有 K 个 小行星.有一个可以横着切或竖着切的武器,问最少切多少次,所有行星都会被毁灭. 分析: 将 1~n 行数加入左 ...
- POJ 2446 Chessboard(二分图最大匹配)
题意: M*N的棋盘,规定其中有K个格子不能放任何东西.(即不能被覆盖) 每一张牌的形状都是1*2,问这个棋盘能否被牌完全覆盖(K个格子除外) 思路: M.N很小,把每一个可以覆盖的格子都离散成一个个 ...
- poj 1463 Strategic game DP
题目地址:http://poj.org/problem?id=1463 题目: Strategic game Time Limit: 2000MS Memory Limit: 10000K Tot ...
- [POJ] 3020 Antenna Placement(二分图最大匹配)
题目地址:http://poj.org/problem?id=3020 输入一个字符矩阵,'*'可行,'o'不可行.因为一个点可以和上下左右四个方向的一个可行点组成一个集合,所以对图进行黑白染色(每个 ...
- [POJ] 2239 Selecting Courses(二分图最大匹配)
题目地址:http://poj.org/problem?id=2239 Li Ming大学选课,每天12节课,每周7天,每种同样的课可能有多节分布在不同天的不同节.问Li Ming最多可以选多少节课. ...
- POJ - 3020 Antenna Placement 二分图最大匹配
http://poj.org/problem?id=3020 首先注意到,答案的最大值是'*'的个数,也就是相当于我每用一次那个技能,我只套一个'*',是等价的. 所以,每结合一对**,则可以减少一次 ...
- poj 1463 Strategic game
题目链接:http://poj.org/problem?id=1463 题意:给出一个无向图,每个节点只有一个父亲节点,可以有多个孩子节点,在一个节点上如果有一位战士守着,那么他可以守住和此节点相连的 ...
- poj 3894 System Engineer (二分图最大匹配--匈牙利算法)
System Engineer Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 507 Accepted: 217 Des ...
随机推荐
- Python基础—15-正则表达式
正则表达式 应用场景 特定规律字符串的查找替换切割等 邮箱格式.URL.IP等的校验 爬虫项目中,特定内容的提取 使用原则 只要是能够使用字符串函数解决的问题,就不要使用正则 正则的效率较低,还会降低 ...
- iOS | XIB简单应用和注意点
2018开篇第一篇文章,本文分享一点关于XIB的小知识,对于iOS开发新人来说或许有用. XIB 是 Interface Builder 的图形界面设计文档. 从Xcode 3.0 开始,苹果提供Xi ...
- JavaScript 基础(二)数组
字符串, JavaScript 字符串就是用'' 和""括起来的字符表示. 字符字面量, \n 换行, \t 制表, \b 退格, \r 回车, \f 进纸, \\ 斜杠,\' 单 ...
- ABAP术语-BW (Business Information Warehouse)
BW (Business Information Warehouse) 原文:http://www.cnblogs.com/qiangsheng/archive/2008/01/14/1037761. ...
- CASE WHEN 小结
1.简单的一个case when 例子: CASE sex ' THEN '男' ' THEN '女' ELSE '其他' END 2. case when 在一整个表为空强行让其显示出一个值,在其后 ...
- Linux之MariaDB
MariaDB数据库的起源 MariaDB数据库管理系统是MySQL的一个分支,主要由开源社区在维护,采用GPL授权许可.开发这个分支的原因之一是:甲骨文公司收购了MySQL后,有将MySQL闭源的潜 ...
- hadoop生态搭建(3节点)-07.hive配置
# http://archive.apache.org/dist/hive/hive-2.1.1/ # ================================================ ...
- Excel VBA表格自行开发计划
Excel VBA表格自行开发计划 要求功能 1. 批量删除 2. [X] 批量填充 3. [X] 批量重命名 4. [ ] 按颜色求和 5. [ ] 按底纹色选中单元格 6. [ ] 统计底纹颜色个 ...
- spring-boot整合ehcache实现缓存机制
EhCache 是一个纯Java的进程内缓存框架,具有快速.精干等特点,是Hibernate中默认的CacheProvider. ehcache提供了多种缓存策略,主要分为内存和磁盘两级,所以无需担心 ...
- 批量安装Python第三方库
1.首先在python程序的文件夹内,新建一个文本文档,名字自定义,在文档中输入需要安装的第三方库,并用英文半角逗号隔开. import os def getTxt(): txt = open(&qu ...