EventLoop介绍
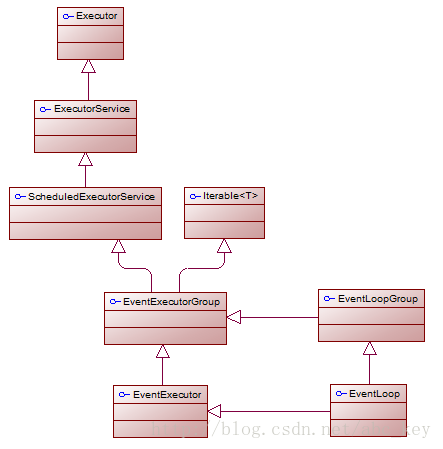
可以进行各种骚操作
//nio
java.nio.channels.SocketChannel mySocket = java.nio.channels.SocketChannel.open();
//netty
SocketChannel ch = new NioSocketChannel(mySocket);
EventLoopGroup group = new NioEventLoopGroup();
//register channel
ChannelFuture registerFuture = group.register(ch);
//de-register channel
ChannelFuture deregisterFuture = ch.deregister();
EventLoop.register(...)和Channel.deregister(...)都是非阻塞异步的,也就是说它们可能不会理解执行完成,可能稍后完成。它们返回ChannelFuture,我们在需要进一步操作或确认完成操作时可以添加一个ChannelFutureLister或在ChannelFuture上同步等待至完成;选择哪一种方式看实际需求,一般建议使用ChannelFutureLister,应避免阻塞。
挂起IO处理
EventLoopGroup group = new NioEventLoopGroup();
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(group).channel(NioSocketChannel.class)
.handler(new SimpleChannelInboundHandler<ByteBuf>() {
@Override
protected void channelRead0(ChannelHandlerContext ctx,
ByteBuf msg) throws Exception {
//remove this ChannelHandler and de-register
ctx.pipeline().remove(this);
ctx.deregister();
}
});
ChannelFuture future = bootstrap.connect(
new InetSocketAddress("www.baidu.com", 80)).sync();
//....
Channel channel = future.channel();
//re-register channel and add ChannelFutureLister
group.register(channel).addListener(new ChannelFutureListener() {
@Override
public void operationComplete(ChannelFuture future) throws Exception {
if(future.isSuccess()){
System.out.println("Channel registered");
}else{
System.out.println("register channel on EventLoop fail");
future.cause().printStackTrace();
}
}
});
迁移通道到另一个事件循环
- 当前EventLoop太忙碌,需要将Channel移到一个不是很忙碌的EventLoop;
- 终止EventLoop释放资源同时保持活跃Channel可以继续使用;
- 迁移Channel到一个执行级别较低的非关键业务的EventLoop中。
EventLoopGroup group = new NioEventLoopGroup();
final EventLoopGroup group2 = new NioEventLoopGroup();
Bootstrap b = new Bootstrap();
b.group(group).channel(NioSocketChannel.class)
.handler(new SimpleChannelInboundHandler<ByteBuf>() {
@Override
protected void channelRead0(ChannelHandlerContext ctx,
ByteBuf msg) throws Exception {
// remove this channel handler and de-register
ctx.pipeline().remove(this);
ChannelFuture f = ctx.deregister();
// add ChannelFutureListener
f.addListener(new ChannelFutureListener() {
@Override
public void operationComplete(ChannelFuture future)
throws Exception {
// migrate this handler register to group2
group2.register(future.channel());
}
});
}
});
ChannelFuture future = b.connect("www.baidu.com", 80);
future.addListener(new ChannelFutureListener() {
@Override
public void operationComplete(ChannelFuture future)
throws Exception {
if (future.isSuccess()) {
System.out.println("connection established");
} else {
System.out.println("connection attempt failed");
future.cause().printStackTrace();
}
}
});
EventLoop介绍的更多相关文章
- Netty 核心组件 EventLoop 源码解析
前言 在前文 Netty 启动过程源码分析 (本文超长慎读)(基于4.1.23) 中,我们分析了整个服务器端的启动过程.在那篇文章中,我们重点关注了启动过程,而在启动过程中对核心组件并没有进行详细介绍 ...
- 【Netty】Netty核心组件介绍
一.前言 前篇博文体验了Netty的第一个示例,下面接着学习Netty的组件和其设计. 二.核心组件 2.1. Channel.EventLoop和ChannelFuture Netty中的核心组件包 ...
- 深入理解javascript中的事件循环event-loop
前面的话 本文将详细介绍javascript中的事件循环event-loop 线程 javascript是单线程的语言,也就是说,同一个时间只能做一件事.而这个单线程的特性,与它的用途有关,作为浏览器 ...
- Netty源码分析(五):EventLoop
上一篇主要介绍了一下EventLoopGroup,本篇详细看下它的成员EventLoop. 类结构 NioEventLoop继承自SingleThreadEventLoop,而SingleThread ...
- Netty重要概念介绍
Netty重要概念介绍 Bootstrap Netty应用程序通过设置bootstrap(引导)类开始,该类提供了一个用于网络成配置的容器. 一种是用于客户端的Bootstrap 一种是用于服务端的S ...
- Qt 之 模态、非模态、半模态窗口的介绍及 实现QDialog的exec()方法
一.简述 先简单介绍一下模态与非模态对话框. 模态对话框 简单一点讲就是在弹出模态对话框时,除了该对话框整个应用程序窗口都无法接受用户响应,处于等待状态,直到模态对话框被关闭.这时一般需要点击对话框中 ...
- swoole的EventLoop学习
我们先使用php来写一个socket的服务端.先从最开始的模型开始将起逐步引申到为何要使用eventloop 1.最简单的socket服务端,直接按照官方文档来执行 <?php $sock = ...
- Netty源码细节IO线程(EventLoop)(转)
原文:http://budairenqin.iteye.com/blog/2215896 源码来自Netty5.x版本, 本系列文章不打算从架构的角度去讨论netty, 只想从源码细节展开, 又不想通 ...
- EventLoop(netty源码死磕4)
精进篇:netty源码 死磕4-EventLoop的鬼斧神工 目录 1. EventLoop的鬼斧神工 2. 初识 EventLoop 3. Reactor模式回顾 3.1. Reactor模式的组 ...
随机推荐
- (2)打造简单OS-开机BIOS初始化与MBR操作系统引导详解
================大概了解即可=============== 1.BIOS的工作: 我们的计算机在开机之前,它是一个纯硬件的机器,但是从按下开机按钮的那一刻起,ROM上的固化程序就开始为 ...
- 多项式求逆/分治FFT 学习笔记
一.多项式求逆 给定一个多项式 \(F(x)\),请求出一个多项式 \(G(x)\), 满足 \(F(x) * G(x) \equiv 1 ( \mathrm{mod\:} x^n )\).系数对 \ ...
- 图灵学院java架构课程
1.wps文档地址 https://docs.qq.com/doc/DRVNLUndvTmFSdEhO 2.百度网盘地址 https://pan.baidu.com/s/1uxaTzJZHKrsw_H ...
- Hadoop(3)如何构建HDFS--HA,YARN---HA
什么是HA? HA的意思是High Availability高可用,指当当前工作中的机器宕机后,会自动处理这个异常,并将工作无缝地转移到其他备用机器上去,以来保证服务的高可用. HA方式安装部署才是最 ...
- python3编程基础之一:操作
基本操作有:读数据.写数据.运算.控制.输入.输出.语句块 1.读取数据: num1 = 50 num2 = num1 //通过num2取得num1的值,这就是逻辑上的读取 测试数据:print(nu ...
- [WEB安全]Weblogic漏洞总结
0x01 Weblogic简介 1.1 叙述 Weblogic是美国Oracle公司出品的一个应用服务器(application server),确切的说是一个基于Java EE架构的中间件,是用于开 ...
- 网页表格导入导出Excel
用JS实现网页表格数据导入导出excel. 首先是JS文件中的代码 (function($){ function getRows(target){ var state = $(target).data ...
- C# 将文本写入到文件
将字符串数组写入到文件,每个元素为一行 string[] lines = { "First line", "Second line", "Third ...
- gevent学习
gevent gevent基于协程的网络库,基于libev的快速的事件循环,基于greenlet的轻量级执行单元,重用了Python标准库中的概念,支持socket.ssl.三方库通过打猴子补丁的形式 ...
- log4j:ERROR setFile(null,true) call failed.错误解决
首先说明,我是用hive执行bin/hiveserver2时出现的这个错误.如下图所示,红框中的内容也清晰的告诉我们出错的原因和文件路径. 之后,我查看了一下该路径.发现我用的是beifeng的用户, ...