POJ-2415 Hike on a Graph (BFS)
Description
In the sixties ("make love not war") a one-person variant of the
game emerged. In this variant one person moves all the three pieces, not
necessarily one after the other, but of course only one at a time. Goal
of this game is to get all pieces onto the same location, using as few
moves as possible. Find out the smallest number of moves that is
necessary to get all three pieces onto the same location, for a given
board layout and starting positions.
Input
input contains several test cases. Each test case starts with the
number n. Input is terminated by n=0. Otherwise, 1<=n<=50. Then
follow three integers p1, p2, p3 with 1<=pi<=n denoting the
starting locations of the game pieces. The colours of the arrows are
given next as a m×m matrix of whitespace-separated lower-case letters.
The element mij denotes the colour of the arrow between the locations i
and j. Since the graph is undirected, you can assume the matrix to be
symmetrical.
Output
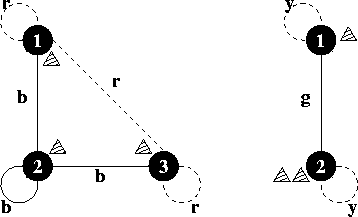
each test case output on a single line the minimum number of moves
required to get all three pieces onto the same location, or the word
"impossible" if that is not possible for the given board and starting
locations.
Sample Input
3 1 2 3
r b r
b b b
r b r
2 1 2 2
y g
g y
0
Sample Output
2
impossible 题目大意:一张完全图,三个人,图的边上有颜色,三个人的位置已知,每次只能让一个人移动一次,求总共最少移动多少次,三个人能碰面。移动的规则是:当前人要走的边的颜色与其他两个人之间的边的颜色相同时,才能移动。
题目分析:大水题!!!wa了几次都是因为没有搞懂移动规则,把这道题记下来给自己涨个记性!!! 代码如下:
# include<iostream>
# include<cstdio>
# include<queue>
# include<cstring>
# include<algorithm>
using namespace std;
struct node
{
int a,b,c,t;
node(int _a,int _b,int _c,int _t):a(_a),b(_b),c(_c),t(_t){}
bool operator < (const node &a) const {
return t>a.t;
}
};
int n,vis[][][];
char p[][];
void bfs(int a,int b,int c)
{
priority_queue<node>q;
memset(vis,,sizeof(vis));
vis[a][b][c]=;
q.push(node(a,b,c,));
while(!q.empty())
{
node u=q.top();
q.pop();
if(u.a==u.b&&u.b==u.c&&u.a==u.c){
printf("%d\n",u.t);
return ;
}
for(int i=;i<=n;++i){
if(p[u.a][i]==p[u.b][u.c]&&!vis[i][u.b][u.c]){
vis[i][u.b][u.c]=;
q.push(node(i,u.b,u.c,u.t+));
}
}
for(int i=;i<=n;++i){
if(p[u.b][i]==p[u.a][u.c]&&!vis[u.a][i][u.c]){
vis[u.a][i][u.c]=;
q.push(node(u.a,i,u.c,u.t+));
}
}
for(int i=;i<=n;++i){
if(p[u.c][i]==p[u.a][u.b]&&!vis[u.a][u.b][i]){
vis[u.a][u.b][i]=;
q.push(node(u.a,u.b,i,u.t+));
}
}
}
printf("impossible\n");
}
int main()
{
int a,b,c;
while(scanf("%d",&n)&&n)
{
scanf("%d%d%d",&a,&b,&c);
for(int i=;i<=n;++i)
for(int j=;j<=n;++j)
cin>>p[i][j];
bfs(a,b,c);
}
return ;
}
POJ-2415 Hike on a Graph (BFS)的更多相关文章
- [ACM_搜索] ZOJ 1103 || POJ 2415 Hike on a Graph (带条件移动3盘子到同一位置的最少步数 广搜)
Description "Hike on a Graph" is a game that is played on a board on which an undirected g ...
- HDU 5876:Sparse Graph(BFS)
http://acm.hdu.edu.cn/showproblem.php?pid=5876 Sparse Graph Problem Description In graph theory, t ...
- 2017ICPC南宁赛区网络赛 Minimum Distance in a Star Graph (bfs)
In this problem, we will define a graph called star graph, and the question is to find the minimum d ...
- POJ.1426 Find The Multiple (BFS)
POJ.1426 Find The Multiple (BFS) 题意分析 给出一个数字n,求出一个由01组成的十进制数,并且是n的倍数. 思路就是从1开始,枚举下一位,因为下一位只能是0或1,故这个 ...
- Leetcode之广度优先搜索(BFS)专题-133. 克隆图(Clone Graph)
Leetcode之广度优先搜索(BFS)专题-133. 克隆图(Clone Graph) BFS入门详解:Leetcode之广度优先搜索(BFS)专题-429. N叉树的层序遍历(N-ary Tree ...
- 【算法导论】图的广度优先搜索遍历(BFS)
图的存储方法:邻接矩阵.邻接表 例如:有一个图如下所示(该图也作为程序的实例): 则上图用邻接矩阵可以表示为: 用邻接表可以表示如下: 邻接矩阵可以很容易的用二维数组表示,下面主要看看怎样构成邻接表: ...
- 深度优先搜索(DFS)与广度优先搜索(BFS)的Java实现
1.基础部分 在图中实现最基本的操作之一就是搜索从一个指定顶点可以到达哪些顶点,比如从武汉出发的高铁可以到达哪些城市,一些城市可以直达,一些城市不能直达.现在有一份全国高铁模拟图,要从某个城市(顶点) ...
- 图的 储存 深度优先(DFS)广度优先(BFS)遍历
图遍历的概念: 从图中某顶点出发访遍图中每个顶点,且每个顶点仅访问一次,此过程称为图的遍历(Traversing Graph).图的遍历算法是求解图的连通性问题.拓扑排序和求关键路径等算法的基础.图的 ...
- 数据结构与算法之PHP用邻接表、邻接矩阵实现图的广度优先遍历(BFS)
一.基本思想 1)从图中的某个顶点V出发访问并记录: 2)依次访问V的所有邻接顶点: 3)分别从这些邻接点出发,依次访问它们的未被访问过的邻接点,直到图中所有已被访问过的顶点的邻接点都被访问到. 4) ...
随机推荐
- (七)git分支的操作
1.git branch——显示分支一览表 2.git checkout -b——创建.切换分支 往feature-A中不断add.commit叫培育分支 git checkout - 切回上一个分支 ...
- Linux启动vi编辑器时提示E325: ATTENTION解决方案
Linux启动vi编辑器时提示E325: ATTENTION解决方案 Vi编辑器是Linux的文本编辑器,在Linux系统的运用非常广泛,不少朋友在打开Vi编辑器的时候提示E325: ATTENTIO ...
- 安装webpack出现警告: fsevents@^1.0.0 (node_modules\chokidar\node_modules\fsevents):
警告如下: npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@^1.0.0 (node_modules\chokidar\node_mo ...
- 重写(override)与重载(overload)的区别
一.重写(override) override是重写(覆盖)了一个方法,以实现不同的功能.一般是用于子类在继承父类时,重写(重新实现)父类中的方法. 重写(覆盖)的规则: 1.重写方法的参数列表必须完 ...
- PyQt5 - 01 使用qt creator创建第一个pyqt5界面程序
1. 安装Qt Creator qt creator下载点我 2. 利用Qt Creator创建界面 点击文件 -> 新建文件或项目 选择Qt -> Qt设计师界面类 选择一个模版,创建一 ...
- linux性能分析工具之火焰图
一.环境 1.1 jello@jello:~$ uname -a Linux jello 4.4.0-98-generic #121-Ubuntu SMP Tue Oct 10 14:24:03 UT ...
- babun安装,整合到cmder
babun Babun的特性: 预装了Cygwin以及许多的插件 默认的命令行安装工具,没有管理员权限要求. 预装了 pact工具,一个高级的包管理器,类似 apt-get或yum xTerm-256 ...
- 史丰收速算|2014年蓝桥杯B组题解析第四题-fishers
史丰收速算 史丰收速算法的革命性贡献是:从高位算起,预测进位.不需要九九表,彻底颠覆了传统手算! 速算的核心基础是:1位数乘以多位数的乘法. 其中,乘以7是最复杂的,就以它为例. 因为,1/7 是个循 ...
- The way to Go(7): 变量
参考: Github: Go Github: The way to Go 变量 一般格式:var identifier type. Go在声明变量时将变量的类型放在变量的名称之后: 避免像 C 语言中 ...
- UVa 225 黄金图形(回溯+剪枝)
https://vjudge.net/problem/UVA-225 题意:平面上有k个障碍点,从(0,0)出发,第一次走1个单位,第二次走2个单位,...第n次走n个单位,最后恰好回到(n,n).每 ...