hdu 4119 Isabella's Message
Isabella's Message
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1365 Accepted Submission(s): 400
The encrypted message is an N * N matrix, and each grid contains a character.
Steve uses a special mask to work as a key. The mask is N * N(where N is an even number) matrix with N*N/4 holes of size 1 * 1 on it.
The decrypt process consist of the following steps:
1. Put the mask on the encrypted message matrix
2. Write down the characters you can see through the holes, from top to down, then from left to right.
3. Rotate the mask by 90 degrees clockwise.
4. Go to step 2, unless you have wrote down all the N*N characters in the message matrix.
5. Erase all the redundant white spaces in the message.
For example, you got a message shown in figure 1, and you have a mask looks like figure 2. The decryption process is shown in figure 3, and finally you may get a message "good morning".
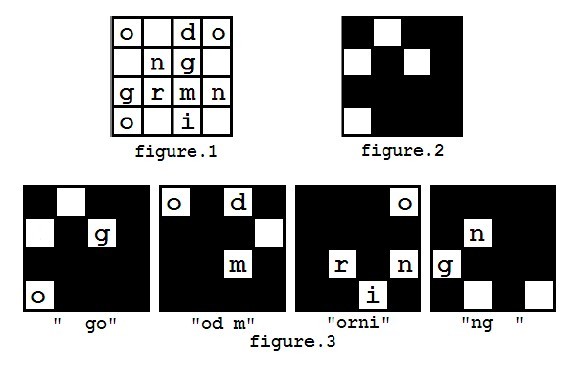
You can assume that the mask is always carefully chosen that each character in the encrypted message will appear exactly once during decryption.
However, in the first step of decryption, there are several ways to put the mask on the message matrix, because the mask can be rotated (but not flipped). So you may get different results such as "od morning go" (as showed in figure 4), and you may also get other messages like "orning good m", "ng good morni".
Steve didn't know which direction of the mask should be chosen at the beginning, but after he tried all possibilities, he found that the message "good morning" is the only one he wanted because he couldn't recognize some words in the other messages. So he will always consider the message he can understand the correct one. Whether he can understand a message depends whether he knows all the words in the message. If there are more than one ways to decrypt the message into an understandable one, he will choose the lexicographically smallest one. The way to compare two messages is to compare the words of two messages one by one, and the first pair of different words in the two messages will determine the lexicographic order of them.
Isabella sends letters to Steve almost every day. As decrypting Isabella's message takes a lot of time, and Steve can wait no longer to know the content of the message, he asked you for help. Now you are given the message he received, the mask, and the list of words he already knew, can you write a program to help him decrypt it?
Each test case contains several lines.
The first line contains an even integer N(2 <= N <= 50), indicating the size of the matrix.
The following N lines each contains exactly N characters, reresenting the message matrix. The message only contains lowercase letters and periods('.'), where periods represent the white spaces.
You can assume the matrix contains at least one letter.
The followingN lines each containsN characters, representing the mask matrix. The asterisk('*') represents a hole, and period('.') otherwise. The next line contains an integer M(1 <= M <= 100), the number of words he knew.
Then the following M lines each contains a string represents a word. The words only contain lowercase letters, and its length will not exceed 20.
If Steve cannot understand the message, just print the Y as "FAIL TO DECRYPT".
4
o.do
.ng.
grmn
o.i.
.*..
*.*.
....
*...
2
good
morning
4
..lf
eoyv
oeou
vrer
..*.
.*..
....
*.*.
5
i
you
the
love
forever
4
.sle
s.c.
e.fs
..uu
*...
.*..
...*
..*.
1
successful
Case #2: love you forever
Case #3: FAIL TO DECRYPT
#include <iostream>
#include <stdio.h>
#include <string.h>
using namespace std;
#define MAXN 55
struct node {
char map[MAXN][MAXN];
};
int n,kk;char str[4][10000],lib[10000][25],anss[10000],stemp[10000];int strnum[4];
bool strflag[4];
node change(node a)
{
int i,j,ii,k;node b;
for(i=0,ii=0;i<n;i++,ii++)
{
for(j=n-1,k=0;j>=0;j--,k++)
{
b.map[ii][k]=a.map[j][i];
}
}
return b;
}
bool find(char str[])
{
int i;
for(i=0;i<kk;i++)
{
if(strcmp(str,lib[i])==0)
return true;
}
return false;
}
int main()
{
int tcase,i,j,i2,space,tt=1;
node a,b;
scanf("%d",&tcase);
while(tcase--)
{
memset(strnum,0,sizeof(strnum));
memset(strflag,true,sizeof(strflag));
for(i=0;i<4;i++)
{
memset(str[i],0,sizeof(str[i]));
}
scanf("%d",&n);
gets(stemp);
for(i=0;i<n;i++)
{
for(j=0;j<n;j++)
{
char c=getchar();
a.map[i][j]=c;
}
gets(stemp);
}
for(i=0;i<n;i++)
{
for(j=0;j<n;j++)
{
char c=getchar();
b.map[i][j]=c;
}
gets(stemp);
}
scanf("%d",&kk);
gets(stemp);
for(i=0;i<kk;i++)
{
scanf("%s",lib[i]);
}
memset(stemp,0,sizeof(stemp));
int space=0;node primeb=b;
for(i=0;i<4;i++)
{
bool flag=true;space=0;b=primeb;
for(j=0;j<4&&flag;j++)
{
for(int ii=0;ii<n&&flag;ii++)
for(int jj=0;jj<n&&flag;jj++)
{
if(b.map[ii][jj]=='*')
{
if(a.map[ii][jj]=='.'&&space>0)
{
stemp[space++]='\0';
if(!find(stemp))
{
flag=false;
strflag[i]=false;
}
else
{
str[i][strnum[i]++]=' ';
for(i2=0;i2<=space-2;i2++)
{
str[i][strnum[i]++]=stemp[i2];
}
}
space=0;
}
if(a.map[ii][jj]!='.')
{
stemp[space++]=a.map[ii][jj];
}
}
} b=change(b);
}
if(space>0&&flag)
{
stemp[space++]='\0';
if(!find(stemp))
{
flag=false;
strflag[i]=false;
}
else
{
str[i][strnum[i]++]=' ';
for(i2=0;i2<=space-2;i2++)
{
str[i][strnum[i]++]=stemp[i2];
}
}
space=0;
}
primeb=change(primeb);
}
bool flag=true;
for(i=0;i<4;i++)
{
if(strflag[i])
{
str[i][strnum[i]++]='\0';
if(flag)
{
strcpy(anss,str[i]);
flag=false;
}
if(strcmp(anss,str[i])>0)
strcpy(anss,str[i]);
}
}
if(!flag)
printf("Case #%d:%s\n",tt++,anss);
else
printf("Case #%d: FAIL TO DECRYPT\n",tt++);
}
return 0;
}
hdu 4119 Isabella's Message的更多相关文章
- hdu 4119 Isabella's Message 模拟题
Isabella's Message Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://acm.hdu.edu.cn/showproblem.p ...
- hdu 3410 Passing the Message(单调队列)
题目链接:hdu 3410 Passing the Message 题意: 说那么多,其实就是对于每个a[i],让你找他的从左边(右边)开始找a[j]<a[i]并且a[j]=max(a[j])( ...
- hdu 4300 Clairewd’s message KMP应用
Clairewd’s message 题意:先一个转换表S,表示第i个拉丁字母转换为s[i],即a -> s[1];(a为明文,s[i]为密文).之后给你一串长度为n<= 100000的前 ...
- hdu 4300 Clairewd’s message(具体解释,扩展KMP)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4300 Problem Description Clairewd is a member of FBI. ...
- hdu 4300 Clairewd’s message(扩展kmp)
Problem Description Clairewd is a member of FBI. After several years concealing in BUPT, she interce ...
- HDU 4300 Clairewd’s message(KMP+思维)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4300 题目大意:题目大意就是给以一段字符xxxxzzz前面x部分是密文z部分是明文,但是我们不知道是从 ...
- 【HDU 4300 Clairewd’s message】
Clairewd is a member of FBI. After several years concealing in BUPT, she intercepted some important ...
- HDU - 3410 Passing the Message 单调递减栈
Passing the Message What a sunny day! Let’s go picnic and have barbecue! Today, all kids in “Sun Flo ...
- hdu 4300 Clairewd’s message 字符串哈希
Clairewd’s message Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Other ...
随机推荐
- 有关android源码编译的几个问题
项目用到编译环境,与源码有些差异不能照搬,关键是连源码都没编译过,下面基本上是行网上照的各种自学成才的分享,病急乱投医了,都记在下面作为参照吧. 1.验证是否编译正确,在终端执行 emulator & ...
- SQL Server中 sysobjects、syscolumns、systypes
1.sysobjects 系统对象表. 保存当前数据库的对象,如约束.默认值.日志.规则.存储过程等 在大多数情况下,对你最有用的两个列是Sysobjects.name和Sysobjects.x ...
- python打包成exe
目前有三种方法可以实现python打包成exe,分别为 py2exe Pyinstaller cx_Freeze 其中没有一个是完美的 1.py2exe的话不支持egg类型的python库 2.Pyi ...
- day2_python学习笔记_chapter4_标准类型和内建函数
1. 标准类型 Integer,Boolean, Long integer, Floating point real number, Complex number, String, List, Tup ...
- 以Android环境为例的多线程学习笔记(二)-----------------锁和条件机制
现在的绝大多数应用程序都是多线程的程序,而当有两个或两个以上的线程需要对同一数据进行存取时,就会出现条件竞争,也即 是这几个线程中都会有一段修改该数据状态的代码.但是如果这些线程的运行顺序推行不当的话 ...
- 网络技术教程笔记(20)ISDN
广域网与接入网技术 广域网与接入网技术 常见接入技术--ISDN 综合业务数字网(Integrated Services Digital Network,ISDN)由电话综合数字网IDN演化而成,能够 ...
- JQuery的Select操作集合
jQuery获取Select选择的Text和Value: 语法解释: $("#select_id").change(function(){//code...}); //为Sel ...
- Auto login to your computer
in run dialog, type in following words and enter Rundll32 netplwiz.dll,UsersRunDll On user account d ...
- 重拾javascript动态客户端网页脚本
笔记一: 一.DOM 作用: · DOM(Doument Object Model) 1.document文档 HTML 文件 (标记语言) <html> < ...
- oracle 两表数据对比---minus
1 引言 在程序设计的过程中,往往会遇到两个记录集的比较.如华东电网PMS接口中实现传递一天中变更(新增.修改.删除)的数据.实现的方式有多种,如编程存储过程返回游标,在存储过程中对两批数据进 ...