hdu 5078 Osu!(鞍山现场赛)
Osu!
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 262144/262144 K (Java/Others)
Total Submission(s): 20 Accepted Submission(s): 15
Special Judge
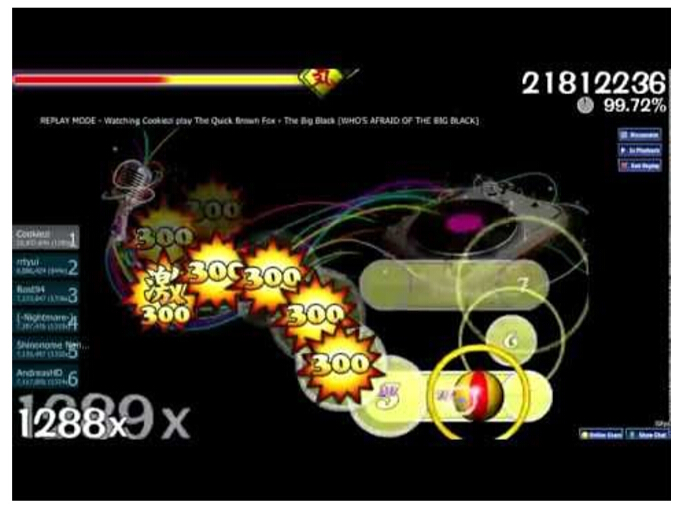
Now, you want to write an algorithm to estimate how diffecult a game is.
To simplify the things, in a game consisting of N points, point i will occur at time ti at place (xi, yi), and you should click it exactly at ti at (xi, yi). That means you should move your cursor
from point i to point i+1. This movement is called a jump, and the difficulty of a jump is just the distance between point i and point i+1 divided by the time between ti and ti+1. And the difficulty of a game is simply the difficulty
of the most difficult jump in the game.
Now, given a description of a game, please calculate its difficulty.
For each test case, the first line contains an integer N (2 ≤ N ≤ 1000) denoting the number of the points in the game. Then N lines follow, the i-th line consisting of 3 space-separated integers, ti(0 ≤ ti < ti+1 ≤ 106),
xi, and yi (0 ≤ xi, yi ≤ 106) as mentioned above.
Your answer will be considered correct if and only if its absolute or relative error is less than 1e-9.
2
5
2 1 9
3 7 2
5 9 0
6 6 3
7 6 0
10
11 35 67
23 2 29
29 58 22
30 67 69
36 56 93
62 42 11
67 73 29
68 19 21
72 37 84
82 24 98
9.2195444573
54.5893762558HintIn memory of the best osu! player ever Cookiezi.
求最大难度,难度为相邻两点的距离除以时间差。
代码:
#include <iostream>
#include <cstdio>
#include <algorithm>
#include <math.h>
using namespace std;
double a[10000];
double b[10000];
int ti[10000];
double dis(int i,int j)
{
return sqrt((a[i]-a[j])*(a[i]-a[j])+(b[i]-b[j])*(b[i]-b[j]));
}
int main()
{
int t,n;
scanf("%d",&t);
while(t--)
{
scanf("%d",&n);
double ans=0; for(int i=0;i<n;i++)
{
scanf("%d%lf%lf",&ti[i],&a[i],&b[i]);
}
for(int i=1;i<n;i++)
{
ans=max(ans,(dis(i-1,i)/(ti[i]-ti[i-1])));
}
printf("%.10f\n",ans);
}
return 0;
}
hdu 5078 Osu!(鞍山现场赛)的更多相关文章
- hdu 5078 2014鞍山现场赛 水题
http://acm.hdu.edu.cn/showproblem.php?pid=5078 现场最水的一道题 连排序都不用,由于说了ti<ti+1 //#pragma comment(link ...
- hdu 5078(2014鞍山现场赛 I题)
数据 表示每次到达某个位置的坐标和时间 计算出每对相邻点之间转移的速度(两点间距离距离/相隔时间) 输出最大值 Sample Input252 1 9//t x y3 7 25 9 06 6 37 6 ...
- hdu 5071(2014鞍山现场赛B题,大模拟)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=5071 思路:模拟题,没啥可说的,移动的时候需要注意top的变化. #include <iostr ...
- 2014 acm鞍山现场赛总结
好像大家都习惯打完比赛写总结,我也来水一发好了.. 记一下流水账,那么多第一次献给了acm,不记一下就白去那么远的地方了.. 首先比赛前网上买了机票跟火车票了.比赛前一天早上6点钟起来收拾东西6点半坐 ...
- hdu 5078 Osu! (2014 acm 亚洲区域赛鞍山 I)
题目链接:http://acm.hdu.edu.cn/showproblem.php? pid=5078 Osu! Time Limit: 2000/1000 MS (Java/Others) ...
- 2014 Asia AnShan Regional Contest --- HDU 5078 Osu!
Osu! Problem's Link: http://acm.hdu.edu.cn/showproblem.php?pid=5078 Mean: 略. analyse: 签到题,直接扫一遍就得答 ...
- ACM/ICPM2014鞍山现场赛D Galaxy (HDU 5073)
题目链接:pid=5073">http://acm.hdu.edu.cn/showproblem.php?pid=5073 题意:给定一条线上的点,然后能够去掉当中的m个,使剩下的到重 ...
- hdu 5073 Galaxy(2014 鞍山现场赛)
Galaxy Time Limit: 2000/1000 MS (J ...
- ACM/ICPC2014鞍山现场赛E hdu5074Hatsune Miku
题目链接:pid=5074">http://acm.hdu.edu.cn/showproblem.php?pid=5074 题意: 给定一个m*m的矩阵mp.然后给定一个长度为n的序列 ...
随机推荐
- 61.C++文件操作实现硬盘检索
#include <iostream> #include <fstream> #include <memory> #include <cstdlib> ...
- 14.字符串hash寻找第一个只出现一次的字符
//char 0-255一共256个 char getonebyhash(char *str) { if (str == NULL) { return '\0'; } char ch = '\0'; ...
- POJ 2227 FloodFill (priority_queue)
题意: 思路: 搞一个priority_queue 先把边界加进去 不断取最小的 向中间扩散 //By SiriusRen #include <queue> #include <cs ...
- java好文章链接
❀Java内存分配全面浅析:http://blog.csdn.net/yangyuankp/article/details/7651251 ❀自定义控件进阶篇1:http://mp.weixin.qq ...
- Linux硬件信息查看
more /proc/cpuinfo more /proc/meminfo more /proc/*info lspci 查看主板信息等cat /proc/cpuinfo CPU信息cat /proc ...
- SQL优化工具SQLAdvisor使用(转)
一.简介 在数据库运维过程中,优化SQL是业务团队与DBA团队的日常任务.例行SQL优化,不仅可以提升程序性能,还能够降低线上故障的概率. 目前常用的SQL优化方式包括但不限于:业务层优化.SQL逻辑 ...
- 【Cocos2d-x 017】 多分辨率适配全然解析
转:http://blog.csdn.net/w18767104183/article/details/22668739 文件夹从Cocos2d-x 2.0.4開始,Cocos2d-x提出了自己的多分 ...
- BZOJ2142: 礼物(拓展lucas)
Description 一年一度的圣诞节快要来到了.每年的圣诞节小E都会收到许多礼物,当然他也会送出许多礼物.不同的人物在小E 心目中的重要性不同,在小E心中分量越重的人,收到的礼物会越多.小E从商店 ...
- PYTHON学习第五天课后总结:
今日重点: 数字类型 字符串类型 列表类型 元组类型 1,数字类型 数字类型为不可变类型 也只能将纯数字类型的字符串转换成int包括: 整型: int() int() 为内置函数,可 ...
- Spider_scrapy
多线程爬虫 进程线程回顾 进程 系统中正在运行的一个应用程序 1个CPU核心1次只能执行1个进程,其他进程处于非运行状态 N个CPU核心可同时执行N个任务 线程 进程中包含的执行单元,1个进程可包含多 ...