Spring MVC文件上传教程
1- 介绍
2- 创建工程
- File/New/Other..
输入:
- Group ID: com.yiibai
- Artifact ID: SpringMVCFileUpload
- Package: com.yiibai.springmvcfileupload
这样,工程就被创建了,如下图所示:

不要担心项目被创建时有错误消息。原因是,你还没有声明的Servlet库。
注意:
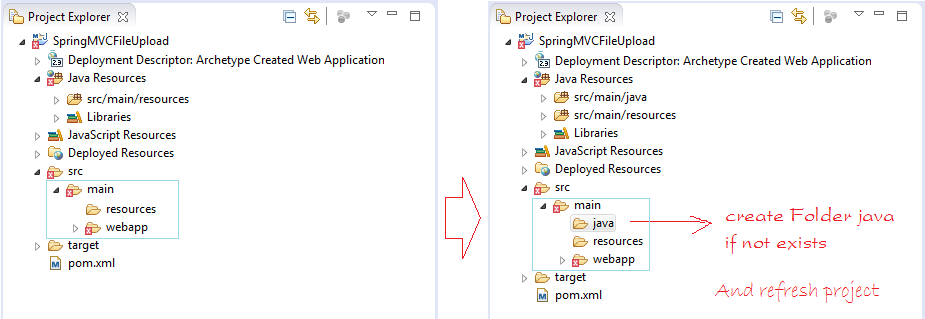
3- 配置Maven
- pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>com.yiibai</groupId>
<artifactId>SpringMVCFileUpload</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCFileUpload Maven Webapp</name>
<url>http://maven.apache.org</url> <dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency> <!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency> <!-- Jstl for jsp page -->
<!-- http://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency> <!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency> <!-- Spring dependencies -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.1.4.RELEASE</version>
</dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.1.4.RELEASE</version>
</dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.1.4.RELEASE</version>
</dependency> <!-- Apache Commons FileUpload -->
<!-- http://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency> <!-- Apache Commons IO -->
<!-- http://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
</dependencies> <build>
<finalName>SpringMVCFileUpload</finalName>
<plugins> <!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version> <!-- Config: contextPath and Port (Default - /SpringMVCFileUpload : 8080) --> <!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build> </project>
4- 配置Spring
配置 web.xml.
- WEB-INF/web.xml
<web-app xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0"> <display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>spring-mvc</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet> <servlet-mapping>
<servlet-name>spring-mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping> <!-- Other XML Configuration -->
<!-- Load by Spring ContextLoaderListener -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/root-context.xml
</param-value>
</context-param> <!-- Spring ContextLoaderListener -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener> </web-app>
- WEB-INF/spring-mvc-servlet.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.1.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd"> <!-- Package Scan -->
<context:component-scan base-package="com.yiibai.springmvcfileupload" /> <!-- Enables the Spring MVC Annotation Configuration -->
<context:annotation-config />
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix">
<value>/WEB-INF/pages/</value>
</property> <property name="suffix">
<value>.jsp</value>
</property>
</bean> <bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- Maximum file size: 1MB -->
<!-- 1MB = 125000 Byte -->
<property name="maxUploadSize" value="125000" />
</bean> </beans>
- WEB-INF/root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- Empty --> </beans>
5- Java类
- MyFileUploadController.java
package com.yiibai.springmvcfileupload; import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List; import javax.servlet.http.HttpServletRequest; import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile; @Controller
public class MyFileUploadController { // Upload One File.
@RequestMapping(value = "/uploadOneFile")
public String uploadOneFileHandler() {
// Forward to "/WEB-INF/pages/uploadOneFile.jsp".
return "uploadOneFile";
} // Upload Multi File.
@RequestMapping(value = "/uploadMultiFile")
public String uploadMultiFileHandler() {
// Forward to "/WEB-INF/pages/uploadMultiFile.jsp".
return "uploadMultiFile";
} // uploadOneFile.jsp, uploadMultiFile.jsp submit to.
@RequestMapping(value = "/doUpload", method = RequestMethod.POST)
public String uploadFileHandler(HttpServletRequest request, Model model,
@RequestParam("file") MultipartFile[] files) { // Root Directory.
String uploadRootPath = request.getServletContext().getRealPath(
"upload");
System.out.println("uploadRootPath=" + uploadRootPath); File uploadRootDir = new File(uploadRootPath);
//
// Create directory if it not exists.
if (!uploadRootDir.exists()) {
uploadRootDir.mkdirs();
}
//
List<File> uploadedFiles = new ArrayList<File>();
for (int i = 0; i < files.length; i++) {
MultipartFile file = files[i]; // Client File Name
String name = file.getOriginalFilename();
System.out.println("Client File Name = " + name); if (name != null && name.length() > 0) {
try {
byte[] bytes = file.getBytes(); // Create the file on server
File serverFile = new File(uploadRootDir.getAbsolutePath()
+ File.separator + name); // Stream to write data to file in server.
BufferedOutputStream stream = new BufferedOutputStream(
new FileOutputStream(serverFile));
stream.write(bytes);
stream.close();
//
uploadedFiles.add(serverFile);
System.out.println("Write file: " + serverFile);
} catch (Exception e) {
System.out.println("Error Write file: " + name);
}
}
}
model.addAttribute("uploadedFiles", uploadedFiles);
return "uploadResult";
} }
6- 视图(JSP)
- uploadOneFile.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Upload One File</title>
</head>
<body> <h3>Upload One File:</h3> <form method="POST" action="doUpload" enctype="multipart/form-data">
File to upload: <input type="file" name="file"><br />
<input type="submit" value="Upload">
</form> </body>
</html>
- uploadMultiFile.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Upload Multi File</title>
</head>
<body> <h3>Upload Multiple File:</h3> <form method="POST" action="doUpload" enctype="multipart/form-data"> File to upload (1): <input type="file" name="file"><br />
File to upload (2): <input type="file" name="file"><br />
File to upload (3): <input type="file" name="file"><br />
File to upload (4): <input type="file" name="file"><br />
File to upload (5): <input type="file" name="file"><br /> <input type="submit" value="Upload">
</form>
</body>
</html>
- uploadResult.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Upload Result</title>
</head>
<body>
<h3>Uploaded Files:</h3> <c:forEach items="${uploadedFiles}" var="file">
- ${file} <br>
</c:forEach> </body>
</html>
7- 运行应用程序


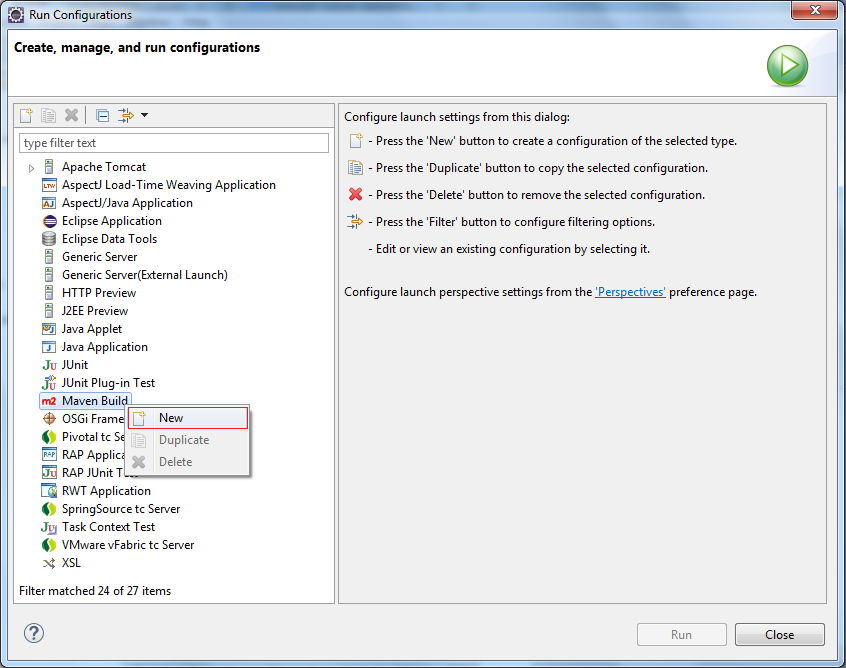
输入:
- Name: Run SpringMVCFileUpload
- Base directory: ${workspace_loc:/SpringMVCFileUpload}
- Goals: tomcat7:run
点击运行(Run):
- http://localhost:8080/SpringMVCFileUpload/uploadOneFile
- http://localhost:8080/SpringMVCFileUpload/uploadMultiFile
a
Spring MVC文件上传教程的更多相关文章
- Spring MVC文件上传教程 commons-io/commons-uploadfile
Spring MVC文件上传教程 commons-io/commons-uploadfile 用到的依赖jar包: commons-fileupload 1.3.1 commons-io 2.4 基于 ...
- Spring MVC 笔记 —— Spring MVC 文件上传
文件上传 配置MultipartResolver <bean id="multipartResolver" class="org.springframework.w ...
- 【Java Web开发学习】Spring MVC文件上传
[Java Web开发学习]Spring MVC文件上传 转载:https://www.cnblogs.com/yangchongxing/p/9290489.html 文件上传有两种实现方式,都比较 ...
- Spring mvc文件上传实现
Spring mvc文件上传实现 jsp页面客户端表单编写 三个要素: 1.表单项type="file" 2.表单的提交方式:post 3.表单的enctype属性是多部分表单形式 ...
- Spring mvc 文件上传到文件夹(转载+心得)
spring mvc(注解)上传文件的简单例子,这有几个需要注意的地方1.form的enctype=”multipart/form-data” 这个是上传文件必须的2.applicationConte ...
- spring mvc 文件上传 ajax 异步上传
异常代码: 1.the request doesn't contain a multipart/form-data or multipart/mixed stream, content type he ...
- Spring MVC文件上传出现错误:Required MultipartFile parameter 'file' is not present
1.配置文件上传的解析器 首先需要在spring mvc的配置文件中(注意是spring mvc的配置文件而不是spring的配置文件:applicationContext.xml)配置: sprin ...
- spring mvc文件上传(单个文件上传|多个文件上传)
单个文件上传spring mvc 实现文件上传需要引入两个必须的jar包 1.所需jar包: commons-fileupload-1.3.1.jar ...
- Strut2 和Spring MVC 文件上传对比
在Java领域中,有两个常用的文件上传项目:一个是Apache组织Jakarta的Common-FileUpload组件 (http://commons.apache.org/proper/commo ...
随机推荐
- 简述es6各种简单方法
1.取代var的let和const 局部变量都可以使用let 固定变量都可以使用const 2.字符串的变化 反引号的使用 3.解构赋值 let [a, b, c] = [1, 2, 3]; let ...
- t-SNE和LDA PCA的学习
t-SNE 可以看这篇文章: http://bindog.github.io/blog/2016/06/04/from-sne-to-tsne-to-largevis/ LDA可以看这篇文章: htt ...
- Yii添加验证码
添加带验证码的登陆: 1.先在模型modules下的LoginForm.php定义一个存储验证码的变量:public $verfyCode: 2.然后在rules()方法里定义:array('veri ...
- ElasticSearch 获取分词的Token
用ES建好索引,有时候需要获取索引中的Token.ES提供了两个接口,链接如下: https://www.elastic.co/guide/en/elasticsearch/reference/1.6 ...
- kubelet分析
kubelet是k8s中节点上运行的管理工具,它负责接受api-server发送的调度请求,在Node上创建管理pod,并且向api-server同步节点的状态.这篇文章主要讲讲kubelet组件如何 ...
- Required MultipartFile parameter 'file' is not present error
<input type=“file”> 中的name 与id 属性 与 addbanner(@RequestParam("file") MultipartFile ...
- 作为Java程序员应该掌握的10项技能
本文详细罗列了作为Java程序员应该掌握的10项技能.分享给大家供大家参考.具体如下: 1.语法:必须比较熟悉,在写代码的时候IDE的编辑器对某一行报错应该能够根据报错信息知道是什么样的语法错误并且知 ...
- org.hibernate.exception.ConstraintViolationException: could not delete:
转自:http://fireinwind.iteye.com/blog/848515 异常描述: org.hibernate.exception.ConstraintViolationExceptio ...
- Linux 忘记密码怎么办
Linux 忘记密码解决方法 很多朋友经常会忘记Linux系统的root密码,linux系统忘记root密码的情况该怎么办呢?重新安装系统吗?当然不用!进入单用户模式更改一下root密码即可. 步骤如 ...
- import * as obj from 'xx'
import * as obj from 'xx' 这种写法是把所有的输出包裹到obj对象里 例如: xx里中: export function hello(){ return '我是hello 内 ...