PHP+Gtk实例(求24点)
- 作者: Laruence(
)
- 本文地址: http://www.laruence.com/2009/05/26/871.html
- 转载请注明出处
最近要安排我为BIT提供的《PHP高级应用–关于PHP你不知道的》一门课的讲课素材, 其中有部分涉及到PHP和Gtk2开发桌面应用的部分, 于是抽空就想写一了一个demo出来.
这是一个经典的求24的算法的PHP实现, 加上了Gtk2的界面, 其实也没什么复杂的, 和MFC开发没什么太大的区别, 唯一的不爽, 就是要自己用代码来写布局。。。
有兴趣的同学可以看看.
后记: 这里有一个网页版的, 可以用来玩玩: http://www.laruence.com/stashes/compute.php
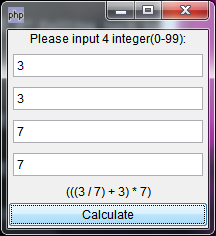
运行截图
完整源代码(PHP-Gtk example):
- <?php
- /**
- * A 24 maker
- * @version 1.0.0
- * @author laruence<laruence at yahoo.com.cn>
- * @copyright (c) 2009 http://www.laruence.com
- */
- class TwentyFourCal extends GtkWindow {
- private $chalkboard;
- private $inputs;
- public $needle = 24;
- public $precision = '1e-6';
- function TwentyFourCal() {
- parent::__construct();
- $this->draw();
- $this->show();
- }
- /**
- * 画窗体方法
- */
- public function draw() {
- $this->set_default_size(200, 200);
- $this->set_title("24计算器");
- $mesg = new GtkLabel('Please input 4 integer(0-99):');
- $this->chalkboard = new GtkLabel();
- $this->inputs = $inputs = array(
- new GtkEntry(),
- new GtkEntry(),
- new GtkEntry(),
- new GtkEntry()
- );
- /**
- * container
- */
- $table = new GtkTable(4, 1, 0);
- $layout = array(
- 'left' => 0,
- 'right' => 1,
- 'top' => 0,
- 'bottom' => 1,
- );
- $vBox = new GtkVBox(false, true);
- $vBox->pack_start($mesg);
- foreach ( $inputs as $input ) {
- $input->set_max_length(2);
- $table->attach($input, $layout['left'], $layout['right'],
- $layout['top']++, $layout['bottom']++);
- }
- $vBox->pack_start($table);
- $button = new GtkButton("Calculate");
- $button->connect("clicked", array($this, "calculate"));
- $vBox->pack_start($this->chalkboard);
- $vBox->pack_start($button, true, false);
- $this->add($vBox);
- }
- public function show() {
- $this->show_all(); // 显示窗体
- }
- private function notice($mesg) {
- $this->chalkboard->set_text($mesg);
- }
- /**
- * 取得用户输入方法
- */
- public function calculate() {
- $operants = array();
- $inputs = $this->inputs;
- foreach ($inputs as $input) {
- $number = $input->get_text();
- if (!preg_match('/^\s*\d+\s*$/', $number)) {
- $this->notice('pleas input for integer(0-99)');
- return ;
- }
- array_push($operants, $number);
- }
- $length = count($operants);
- try {
- $this->search($operants, 4);
- } catch (Exception $e) {
- $this->notice($e->getMessage());
- return;
- }
- $this->notice('can\'t compute!');
- return;
- }
- /**
- * 求24点算法PHP实现
- */
- private function search($expressions, $level) {
- if ($level == 1) {
- $result = 'return ' . $expressions[0] . ';';
- if ( abs(eval($result) - $this->needle) <= $this->precision) {
- throw new Exception($expressions[0]);
- }
- }
- for ($i=0;$i<$level;$i++) {
- for ($j=$i+1;$j<$level;$j++) {
- $expLeft = $expressions[$i];
- $expRight = $expressions[$j];
- $expressions[$j] = $expressions[$level - 1];
- $expressions[$i] = '(' . $expLeft . ' + ' . $expRight . ')';
- $this->search($expressions, $level - 1);
- $expressions[$i] = '(' . $expLeft . ' * ' . $expRight . ')';
- $this->search($expressions, $level - 1);
- $expressions[$i] = '(' . $expLeft . ' - ' . $expRight . ')';
- $this->search($expressions, $level - 1);
- $expressions[$i] = '(' . $expRight . ' - ' . $expLeft . ')';
- $this->search($expressions, $level - 1);
- if ($expLeft != 0) {
- $expressions[$i] = '(' . $expRight . ' / ' . $expLeft . ')';
- $this->search($expressions, $level - 1);
- }
- if ($expRight != 0) {
- $expressions[$i] = '(' . $expLeft . ' / ' . $expRight . ')';
- $this->search($expressions, $level - 1);
- }
- $expressions[$i] = $expLeft;
- $expressions[$j] = $expRight;
- }
- }
- return false;
- }
- function __destruct() {
- Gtk::main_quit();
- }
- }
- new TwentyFourCal();
- Gtk::main(); //进入GTK主循环
- ?>
GTK1的API Reference : http://gtk.php.net/manual/en/gtk.gtkentry.php
GTK2的API Reference: 很不完整
PHP+Gtk实例(求24点)的更多相关文章
- C 语言实例 - 求两数最小公倍数
C 语言实例 - 求两数最小公倍数 用户输入两个数,其这两个数的最小公倍数. 实例 - 使用 while 和 if #include <stdio.h> int main() { int ...
- C 语言实例 - 求两数的最大公约数
C 语言实例 - 求两数的最大公约数 用户输入两个数,求这两个数的最大公约数. 实例 - 使用 for 和 if #include <stdio.h> int main() { int n ...
- JAVA 数组实例-求学生成绩的最大成绩,获取数组中的最大值、最小值
实例: import java.util.*; //求学生最大成绩 public class Test{ public static void main(String[] args){ System. ...
- Mapreduce实例--求平均值
求平均数是MapReduce比较常见的算法,求平均数的算法也比较简单,一种思路是Map端读取数据,在数据输入到Reduce之前先经过shuffle,将map函数输出的key值相同的所有的value值形 ...
- Java文件写入与读取实例求最大子数组
出现bug的点:输入数组无限大: 输入的整数,量大: 解决方案:向文件中输入随机数组,大小范围与量都可以控制. 源代码: import java.io.BufferedReader; import j ...
- ASP.NET Core 6框架揭秘实例演示[24]:中间件的多种定义方式
ASP.NET Core的请求处理管道由一个服务器和一组中间件组成,位于 "龙头" 的服务器负责请求的监听.接收.分发和最终的响应,针对请求的处理由后续的中间件来完成.中间件最终体 ...
- C#中按指定质量保存图片的实例代码 24位深度
/// <summary> /// 按指定的压缩质量及格式保存图片(微软的Image.Save方法保存到图片压缩质量为75) /// </summary ...
- MapReduce实例——求平均值,所得结果无法写出到文件的错误原因及解决方案
1.错误原因 mapreduce按行读取文本,map需要在原有基础上增加一个控制语句,使得读到空行时不执行write操作,否则reduce不接受,也无法输出到新路径. 2.解决方案 原错误代码 pub ...
- C 语言实例 -求分数数列1/2+2/3+3/5+5/8+...的前n项和
程序分析:抓住分子与分母的变化规律:分子a:1,2,3,5,8,13,21,34,55,89,144...分母b:2,3,5,8,13,21,34,55,89,144,233...分母b把数赋给了分子 ...
随机推荐
- centos6性能监控软件
常用软件在此下载 http://rpm.pbone.net/ http://pkgs.org/ collectl 显示cpu\disk\network的实时信息http://dl.fedoraproj ...
- 77. sqlserver 锁表解决方式
select request_session_id spid, OBJECT_NAME(resource_associated_entity_id) tableName from sys.dm_tra ...
- rabbitMQ 的基本知识
参考: https://www.cnblogs.com/dwlsxj/p/RabbitMQ.html
- ajax 遍历json一维数组
$.each(data,function(index,value){}data必须是Object类型index是数组的下标value可以是一个对象 function myonclick() { var ...
- VBA 连接文本的自定义函数(可用于数组公式)
Function ConTxt(ParamArray args() As Variant) As VariantDim tmptext As Variant, i As Variant, cellv ...
- python内置函数 eval()、exec()以及complie()函数
1.eval函数 eval() 函数用来执行一个字符串表达式,并返回表达式的值. eval(expression[, globals[, locals]]) 参数 expression -- 表达式. ...
- ABAP-年月期间搜索帮助
selection-screen begin of block block1 with frame title text-. parameters:p_mon1 like s031-spmon, p_ ...
- 面试题-------SSL协议简介
SSL协议简介 SSL简介 Secure Socket Layer,为Netscape所研发,用以保障在Internet上数据传输之安全,利用数据加密(Encryption)技术,可确保数据在网络上之 ...
- 修改rabbitmq Web UI 监控页面的端口
在前几天工作中遇到一个问题,部署服务器,需要用rabbitmq自带的一个web UI监控组件,但是15672的端口没有对外映射.尝试了几种办法.开始修改rabbitmq.config,rabbitmq ...
- springMVC学习记录2-使用注解配置
前面说了一下使用xml配置springmvc,下面再说说注解配置.项目如下: 业务很简单,主页和输入用户名和密码进行登陆的页面. 看一下springmvc的配置文件: <?xml version ...