poj3162(树形dp+优先队列)
Time Limit: 10000MS | Memory Limit: 131072K | |
Total Submissions: 5409 | Accepted: 1371 | |
Case Time Limit: 3000MS |
Description
flymouse’s sister wc is very capable at sports and her favorite event is walking race. Chasing after the championship in an important competition, she comes to a training center to attend a training course. The center has N check-points numbered 1 through N. Some pairs of check-points are directly connected by two-way paths. The check-points and the paths form exactly a tree-like structure. The course lasts N days. On the i-th day, wc picks check-point i as the starting point and chooses another check-point as the finishing point and walks along the only simple path between the two points for the day’s training. Her choice of finishing point will make it that the resulting path will be the longest among those of all possible choices.
After every day’s training, flymouse will do a physical examination from which data will obtained and analyzed to help wc’s future training be better instructed. In order to make the results reliable, flymouse is not using data all from N days for analysis. flymouse’s model for analysis requires data from a series of consecutive days during which the difference between the longest and the shortest distances wc walks cannot exceed a bound M. The longer the series is, the more accurate the results are. flymouse wants to know the number of days in such a longest series. Can you do the job for him?
Input
The input contains a single test case. The test case starts with a line containing the integers N (N ≤ 106) and M (M < 109). Then follow N − 1 lines, each containing two integers fi and di (i = 1, 2, …, N − 1), meaning the check-points i + 1 and fi are connected by a path of length di.
Output
Output one line with only the desired number of days in the longest series.
Sample Input
3 2
1 1
1 3
Sample Output
3
Hint
Explanation for the sample:
There are three check-points. Two paths of lengths 1 and 3 connect check-points 2 and 3 to check-point 1. The three paths along with wc walks are 1-3, 2-1-3 and 3-1-2. And their lengths are 3, 4 and 4. Therefore data from all three days can be used for analysis.
Source
1 2
2 4
2 2
1 6
1 5
6 1
6 1
7 2
7 3
10 1
11 4
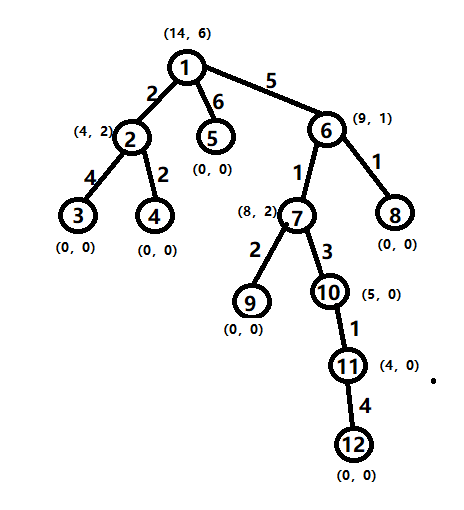
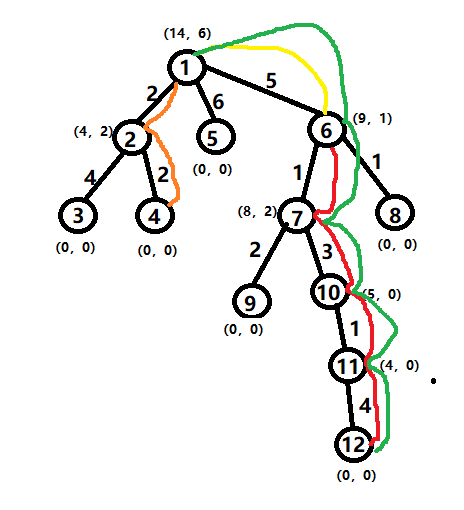
#include<cstdio>
#include<algorithm>
#include<queue>
#define ll long long
using namespace std;
struct{
int v,w,next;
}edge[2000010];//前向星存树
int head[1000010];
int cnt;
deque<ll> mx,mn;//双向队列求连续天数
struct{
ll lfst;//最长距离
ll lsnd;//次长距离
}dp[1000010];
ll ans[1000010];//存实际最长距离
void add(int u,int v,int w){//加边
edge[cnt].v=v;
edge[cnt].w=w;
edge[cnt].next=head[u];
head[u]=cnt++;
} void dfs1(int k,int fz){//(当前节点,父亲节点)
//printf("%d\n",k);
for(int i=head[k];i!=-1;i=edge[i].next){
int v=edge[i].v;
if(v==fz)
continue;
dfs1(v,k); //先往下递归
ll a=dp[v].lfst+edge[i].w;
if(a>dp[k].lfst){//更新最长,次长距离
dp[k].lsnd=dp[k].lfst;
dp[k].lfst=a;
}
else if(a>dp[k].lsnd)
dp[k].lsnd=a;
}
}
void dfs2(int k,int fz,int w){//(儿子节点,根节点,儿子节点到根节点的距离)
//printf("wwww%d %d %d %d\n",k,fz,dp[fz].lfst,dp[fz].lsnd);
if(dp[k].lfst+w==dp[fz].lfst){//如果当前节点在其父亲节点的最长距离线路上
ll a=w+dp[fz].lsnd;//尝试走父亲节点的次长距离
if(a>dp[k].lfst){
dp[k].lsnd=dp[k].lfst;
dp[k].lfst=a;
}
else if(a>dp[k].lsnd)
dp[k].lsnd=a;
ans[k]=dp[k].lfst;
}
else{
ll a=w+dp[fz].lfst;//尝试走父亲节点的最长距离
if(a>dp[k].lfst){//比较并更新
dp[k].lsnd=dp[k].lfst;
dp[k].lfst=a;
}
else if(a>dp[k].lsnd)
dp[k].lsnd=a;
ans[k]=dp[k].lfst;//求出实际最长距离
}
for(int i=head[k];i!=-1;i=edge[i].next){
int v=edge[i].v;
if(v==fz)
continue;
dfs2(v,k,edge[i].w);
}
}
void addmn(int k){//加入递增队列
while(mn.size()&&(ans[mn.back()]>ans[k])){//保持加入节点后队列的单调性
mn.pop_back();
}
mn.push_back(k);
}
void addmx(int k){//加入递减队列
while(mx.size()&&(ans[mx.back()]<ans[k])){
mx.pop_back();
}
mx.push_back(k);
}
int main(){
int n,m;
int v,w;
cnt=0;
scanf("%d%d",&n,&m);
fill(head,head+n+2,-1);//初始化
dp[0].lfst=dp[0].lsnd=0;
dp[1].lfst=dp[1].lsnd=0;
for(int i=2;i<=n;i++){
scanf("%d%d",&v,&w);
dp[i].lfst=dp[i].lsnd=0;//初始化
add(i,v,w);
add(v,i,w);
}
dfs1(1,0);
ans[1]=dp[1].lfst;//我是以1根,那么1的实际最长距离就等于它的最长距离
for(int i=head[1];i!=-1;i=edge[i].next){//往它的儿子节点遍历
dfs2(edge[i].v,1,edge[i].w);//(儿子节点,根节点,儿子节点到根节点的距离)
}
mx.clear();
mn.clear();
mx.push_back(1);//先放入第一天
mn.push_back(1);
int len=0;
int len1=1;
int lf=1;//左标记,表示以第几天开始来计算
for(int i=2;i<=n;i++){
addmx(i);//将该天加入到队列里
addmn(i);
len1++;//长度加1
while(lf<i&&len1&&abs(ans[mn.front()]-ans[mx.front()])>m){//如果绝对差大于m,那么开始天数要往右移
len1--;//当前绝对差大于m,我们要尝试右移开始天数来使得绝对差变小
if(mn.front()==lf)//如果当前的开始天数是最小的距离
mn.pop_front();
if(mx.front()==lf)//如果当前的开始天数是最大距离
mx.pop_front();
lf++;
}
len=max(len,len1);//更新答案
//printf("wwwwwww%d %lld %lld %d\n",i,mn.front(),mx.front(),lf);
}
printf("%d\n",len);
return 0;
}
poj3162(树形dp+优先队列)的更多相关文章
- poj3162 树形dp|树的直径 + 双单调队列|线段树,好题啊
题解链接:https://blog.csdn.net/shiqi_614/article/details/8105149 用树形dp是超时的,, /* 先求出每个点可以跑的最长距离dp[i][0|1] ...
- POJ 3162 Walking Race 树形dp 优先队列
http://poj.org/problem?id=3162 题意 : 一棵n个节点的树.wc爱跑步,跑n天,第i天从第i个节点开始跑步,每次跑到距第i个节点最远的那个节点(产生了n个距离),现在要 ...
- Codeforces 1118 F2. Tree Cutting (Hard Version) 优先队列+树形dp
题目要求将树分为k个部分,并且每种颜色恰好在同一个部分内,问有多少种方案. 第一步显然我们需要知道哪些点一定是要在一个部分内的,也就是说要求每一个最小的将所有颜色i的点连通的子树. 这一步我们可以将所 ...
- poj3162(树形dp+线段树求最大最小值)
题目链接:https://vjudge.net/problem/POJ-3162 题意:给一棵树,求每个结点的树上最远距离,记为a[i],然后求最大区间[l,r]满足区间内的max(a[i])-min ...
- POJ3162 Walking Race(树形DP+尺取法+单调队列)
题目大概是给一棵n个结点边带权的树,记结点i到其他结点最远距离为d[i],问d数组构成的这个序列中满足其中最大值与最小值的差不超过m的连续子序列最长是多长. 各个结点到其他结点的最远距离可以用树形DP ...
- HDU 4123 Bob’s Race 树形dp+单调队列
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=4123 Time Limit: 5000/2000 MS (Java/Others) Memory L ...
- 【树形DP】JSOI BZOJ4472 salesman
题目内容 vjudge链接 某售货员小T要到若干城镇去推销商品,由于该地区是交通不便的山区,任意两个城镇 之间都只有唯一的可能经过其它城镇的路线. 小T 可以准确地估计出在每个城镇停留的净收 益.这些 ...
- poj3417 LCA + 树形dp
Network Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 4478 Accepted: 1292 Descripti ...
- COGS 2532. [HZOI 2016]树之美 树形dp
可以发现这道题的数据范围有些奇怪,为毛n辣么大,而k只有10 我们从树形dp的角度来考虑这个问题. 如果我们设f[x][k]表示与x距离为k的点的数量,那么我们可以O(1)回答一个询问 可是这样的话d ...
随机推荐
- Error: EACCES: permission denied, mkdir '......node-sass/build'错误解决方案
安装node-sass时出现一下错误: gyp ERR! configure error gyp ERR! stack Error: EACCES: permission denied, mkdir ...
- 使用RStudio调试(debug)基础学习(二)和fGarch包中的garchFit函数估计GARCH模型的原理和源码
一.garchFit函数的参数--------------------------------------------- algorithm a string parameter that deter ...
- centos7 基础命令
一: linux基础 (1) 查看服务器的IP信息 ip add showifconfig (2) 操作网卡命令(重启网络和启用网卡) systemctl restart networksystemc ...
- Kafka集群安装部署、Kafka生产者、Kafka消费者
Storm上游数据源之Kakfa 目标: 理解Storm消费的数据来源.理解JMS规范.理解Kafka核心组件.掌握Kakfa生产者API.掌握Kafka消费者API.对流式计算的生态环境有深入的了解 ...
- Redis(二)--Jedis使用
1.Jedis是连接java和redis的jar,这里用maven来添加jar包 # 在eclipse中新建maven project,填写GroupID.Artifactid # 在百度搜索mave ...
- LoRa无线技术介绍
什么是LoRa LoRa是semtech公司创建的低功耗局域网无线标准,低功耗一般很难覆盖远距离,远距离一般功耗高,要想马儿不吃草还要跑得远,好像难以办到.LoRa的名字就是远距离无线电(Long R ...
- vue 简单实现父组件向子组件传值,简单来说就是子组件肆意妄为的调用父组件里后台返回的值
首先在于父子组件传值的方法很多,本人在这里只是简单描述一下一个组件里面引用了子组件,那么子组件如何才能获取父组件中后台返回的值呢? 首先调用组件相信大家都应该明白了(不明白的自己撸撸文档), < ...
- sqlalchemy基础教程
一.基本配置 连接数据库 外部连接数据库时,用于表名数据库身份的一般是一个URL.在sqlalchemy中将该URL包装到一个引擎中,利用这个引擎可以扩展出很多ORM中的对象. from sqlalc ...
- JSON.stringify()方法是将一个javascript值(对象或者数组)转换成为一个JSON字符串;JSON.parse()解析JSON字符串,构造由字符串描述的javascript值或对象
JSON.stringify()方法是将一个javascript值(对象或者数组)转换成为一个JSON字符串:JSON.parse()解析JSON字符串,构造由字符串描述的javascript值或对象
- (03) spring Boot 的配置
1. spring boot 的核心配置 spring boot 项目建立之后,已经创建好了application.properties 配置文件 其实, 配置文件还支持*.yml 格式的: 2. 多 ...