puk1251 最小生成树
Description
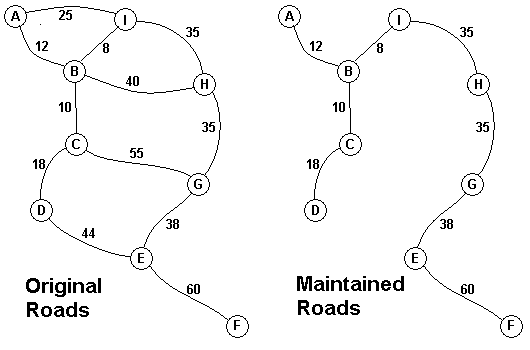
The Head Elder of the tropical island of Lagrishan has a problem. A burst of foreign aid money was spent on extra roads between villages some years ago. But the jungle overtakes roads relentlessly, so the large road network is too expensive to maintain. The Council of Elders must choose to stop maintaining some roads. The map above on the left shows all the roads in use now and the cost in aacms per month to maintain them. Of course there needs to be some way to get between all the villages on maintained roads, even if the route is not as short as before. The Chief Elder would like to tell the Council of Elders what would be the smallest amount they could spend in aacms per month to maintain roads that would connect all the villages. The villages are labeled A through I in the maps above. The map on the right shows the roads that could be maintained most cheaply, for 216 aacms per month. Your task is to write a program that will solve such problems.
Input
Output
Sample Input
9
A 2 B 12 I 25
B 3 C 10 H 40 I 8
C 2 D 18 G 55
D 1 E 44
E 2 F 60 G 38
F 0
G 1 H 35
H 1 I 35
3
A 2 B 10 C 40
B 1 C 20
0
Sample Output
216
30
#include<stdio.h>
#define A 30
int a[A][A]; //邻接矩阵
int lowcost[A]; //保存已在生成树中的顶点到未在生成树中顶点的最短长度
// 找出权值最小的两个顶点的位置 ,并返回其中一个顶点的位置
int First(int n){
int i,j,min,flag;
min=100;
for(i=1;i<=n;i++){
for(j=1;j<=n;j++){
if(min>a[i][j]&&a[i][j]!=0){
min=a[i][j];
flag=i;
}
}
}
return flag;
}
//找出lowcost[]中最短的长度
int minimum(int n){
int i,min,flag=1;
min=100;
for(i=1;i<=n;i++){
if(min>lowcost[i]&&lowcost[i]!=0){
flag=i;
min=lowcost[i];
}
}
return flag;
}
void Tree(int n){
int i,j,k,sum=0;
k = First(n); //得到位置
for(i=1;i<=n;i++){
if(i!=k){
lowcost[i]=a[k][i]; //初始化lowcost[]
}
}
lowcost[k]=0; //每次加入生成树中的顶点对应的位置的权值更新为0
for(i=1;i<n;i++){
k=minimum(n); //获取locost[]中最小的位置
sum=sum+lowcost[k]; //求路径长度值和
lowcost[k]=0; //每次加入生成树中的顶点对应的位置的权值更新为0
//更新lowcost[],如果找到的最小位置到其他顶点的权值小于在lowcost[]中的,就更新为较小的
for(j=1;j<=n;j++){
if(a[k][j]<lowcost[j]&&j!=k){
lowcost[j]=a[k][j];
}
}
}
printf("%d\n",sum);
}
int main()
{
int N,i,j,k,x,y,z;
char ch1[2],ch2[2];
for(i=1;i<=A;i++)
for(j=1;j<=A;j++)
a[i][j]= 100;
scanf("%d",&N);
while(N!=0){
for(i=1;i<N;i++){
scanf("%s %d",ch1,&j);
y=ch1[0]-'A'+1;
for(k=1;k<=j;k++){
scanf("%s %d",ch2,&x); //接收字符
z=ch2[0]-'A'+1; //保存两点间的权值
a[y][z]=x;
a[z][y]=x;
}
}
for(i=1;i<=N;i++){
for(j=1;j<=N;j++) printf(" %d ",a[i][j]);
printf("\n");
} Tree(N);
//执行一次后初始化邻接矩阵
for(i=1;i<=N;i++)
for(j=1;j<=N;j++)
a[i][j]= 100;
scanf("%d",&N);
}
return 0;
}
puk1251 最小生成树的更多相关文章
- 最小生成树(Kruskal算法-边集数组)
以此图为例: package com.datastruct; import java.util.Scanner; public class TestKruskal { private static c ...
- 最小生成树计数 bzoj 1016
最小生成树计数 (1s 128M) award [问题描述] 现在给出了一个简单无向加权图.你不满足于求出这个图的最小生成树,而希望知道这个图中有多少个不同的最小生成树.(如果两颗最小生成树中至少有一 ...
- poj 1251 Jungle Roads (最小生成树)
poj 1251 Jungle Roads (最小生成树) Link: http://poj.org/problem?id=1251 Jungle Roads Time Limit: 1000 ...
- 【BZOJ 1016】【JSOI 2008】最小生成树计数
http://www.lydsy.com/JudgeOnline/problem.php?id=1016 统计每一个边权在最小生成树中使用的次数,这个次数在任何一个最小生成树中都是固定的(归纳证明). ...
- 最小生成树---Prim算法和Kruskal算法
Prim算法 1.概览 普里姆算法(Prim算法),图论中的一种算法,可在加权连通图里搜索最小生成树.意即由此算法搜索到的边子集所构成的树中,不但包括了连通图里的所有顶点(英语:Vertex (gra ...
- Delaunay剖分与平面欧几里得距离最小生成树
这个东西代码我是对着Trinkle的写的,所以就不放代码了.. Delaunay剖分的定义: 一个三角剖分是Delaunay的当且仅当其中的每个三角形的外接圆内部(不包括边界)都没有点. 它的存在性是 ...
- 最小生成树(prim&kruskal)
最近都是图,为了防止几次记不住,先把自己理解的写下来,有问题继续改.先把算法过程记下来: prime算法: 原始的加权连通图——————D被选作起点,选与之相连的权值 ...
- 最小生成树 prime poj1258
题意:给你一个矩阵M[i][j]表示i到j的距离 求最小生成树 思路:裸最小生成树 prime就可以了 最小生成树专题 AC代码: #include "iostream" #inc ...
- 最小生成树 prime + 队列优化
存图方式 最小生成树prime+队列优化 优化后时间复杂度是O(m*lgm) m为边数 优化后简直神速,应该说对于绝大多数的题目来说都够用了 具体有多快呢 请参照这篇博客:堆排序 Heapsort / ...
随机推荐
- xss小游戏通关
xss url:http://test.ctf8.com/level1.php?name=test 小游戏payload: <script>alert("'test'" ...
- python安装和首次使用
安装: 1.安装python环境: 首先打开python官网,下载配置环境:www.python.org 点击上方downloads, 根据系统选择python环境下载 找到 windows x86- ...
- java安全编码指南之:异常处理
目录 简介 异常简介 不要忽略checked exceptions 不要在异常中暴露敏感信息 在处理捕获的异常时,需要恢复对象的初始状态 不要手动完成finally block 不要捕获NullPoi ...
- Python 3 入门,看这篇就够了(超全整理)
史上最全Python资料汇总(长期更新).隔壁小孩都馋哭了 --- 点击领取 今天和大家分享的内容是Python入门干货,文章很长. 简介 Python 是一种高层次的结合了解释性.编译性.互动性和面 ...
- mysql-18-function
#函数 /* 存储过程:可以有0个或多个返回,适合批量插入.批量更新 函数:有且仅有一个返回,适合处理数据后返回一个结果 */ #一.创建语法 /* create function 函数名(参数列表) ...
- The Python Tutorial 和 documentation和安装库lib步骤
链接: The Python Tutorial : https://docs.python.org/3.6/tutorial/index.html Documentation: https://doc ...
- Informatica报错“表或视图不存在”的某种原因
软件版本:9.6.1 背景:测试将OLTP数据库的用户信息表(CUST_INFO)抽取到DW库(DW_CUST_INFO) 问题:工作流启动后,报错RR_4035,并告知表或视图不存在 分析:在导入源 ...
- 如何在Windows7安装U盘中加入USB3.0驱动的支持
安装前请务必备份好您硬盘中的重要数据. 一.在Windows7安装U盘中加入USB3.0驱动的支持 故障现象: 原生Win7系统不包含USB3.0的驱动,所以无法使用USB3.0的U盘在US ...
- Spring IOC 容器预启动流程源码探析
Spring IOC 容器预启动流程源码探析 在应用程序中,一般是通过创建ClassPathXmlApplicationContext或AnnotationConfigApplicationConte ...
- redis哨兵搭建
redis哨兵搭建 1.复制配置文件到conf #单机安装以后[root@t3 redis-5.0.8]# pwd/app/redis-5.0.8[root@t3 redis-5.0.8]# cp s ...