Why you should use async tasks in .NET 4.5 and Entity Framework 6
Improve response times and handle more users with parallel processing
Building a web application using non blocking calls to the data layer is a great way to increase the scalability of your system. Performing a task asynchronously frees up the worker thread to accept another request while work is being done in the background. Until recently, designing your system this way was significantly more complicated. With the .NET framework 4.5 and Entity Framework 6, the task has become trivial.

These talks will help you reshape how you approach work and see your career in a new light.
If you’re starting out building a new ASP .NET MVC 5 application, the new best practice is to use Async tasks for basically everything, unless you have a good reason to do otherwise. Your view controllers can be implemented as async methods, your generic collections have async functions, and with EF6, your data layer can take advantage of the technique as well. This functionality has existed in different forms in the past (PLINQ, Task Parallel Library, etc.) but the coding overhead and added thread management caused many to opt out from implementing it. Today, the System.Threading.Tasks library greatly simplifies the process.
In a .NET web application running on an IIS server, the application pool for the app only has so many threads it can spin up to use for request handling. When a user makes a request for a relatively long running task, the synchronous thread will begin to execute and will be blocked while it’s waiting for data to return, let’s say from an IO operation. If a new request comes in during that time, a new thread will be required to fulfill that request as the existing thread is waiting on IO. Spinning up these new threads requires additional memory and processing, and if your application is heavily used the app pool can quickly run out of available threads and result in an unresponsive application or even a timeout error for the user.
With asynchronous tasks, once the thread reaches the long running task that you’ve called asynchronously, the thread will be released and become available to process further requests while the task is being executed. This will keep your application responsive to the user and it will be able to accommodate significantly more simultaneous requests. In terms of benchmarked performance, I’ve seen load tests show 300% improvement in response times and concurrent connections boost almost 8x [I can’t find the link, but I promise I saw it] over the synchronous counterparts.
It’s not a silver bullet however. There is a time and place for async tasks, knowing when not to use them is just as important as knowing when you should. For a low level guide on how it works, have a look at this MSDN article detailing the process. Basically, the rule of thumb is to use an async task when your operation is accessing a slow medium like the network, disk, or database. If your operation is using data that you know is already in memory (memory stream, cached object, etc.), you’re better off using a synchronous task. Another vital consideration is that async tasks are not thread safe and they are not compatible with lazy loading. To keep your code from imploding, you need to ensure that you never execute two async tasks in parallel using the same data context. You also need to make sure you eager load / include any relational data your object might have to fetch from your async call.
There are some caveats, and even though it’s suggested that you use async tasks as the de facto going forward, you need to consider your application thoroughly before diving in. The performance benefits are too great to ignore, so while it may add a bit of complexity to your software, it should pay off in spades when a few thousand people decide to use it at the same time. Pay close attention to the functions you use it on, structure your data layer in a way that promotes compartmentalization of the context, and know when to use a synchronous call instead. With those things in mind you should be on your way to providing a snappy user experience and software your server administrators will love.
3 reasons to use code first design with Entity Framework
Does relying on an IDE for development make you a bad programmer?
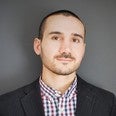
Matthew Mombrea is a software engineer, founder ofCypress North, and a technology enthusiast.
The opinions expressed in this blog are those of the author and do not necessarily represent those of ITworld, its parent, subsidiary or affiliated companies.
Why you should use async tasks in .NET 4.5 and Entity Framework 6的更多相关文章
- [转]Upgrading to Async with Entity Framework, MVC, OData AsyncEntitySetController, Kendo UI, Glimpse & Generic Unit of Work Repository Framework v2.0
本文转自:http://www.tuicool.com/articles/BBVr6z Thanks to everyone for allowing us to give back to the . ...
- Entity Framework 6.0 Tutorials(2):Async query and Save
Async query and Save: You can take advantage of asynchronous execution of .Net 4.5 with Entity Frame ...
- Async/Await - Best Practices in Asynchronous Programming z
These days there’s a wealth of information about the new async and await support in the Microsoft .N ...
- 编程概念--使用async和await的异步编程
Asynchronous Programming with Async and Await You can avoid performance bottlenecks and enhance the ...
- 《C#并发编程经典实例》学习笔记—异步编程关键字 Async和Await
C# 5.0 推出async和await,最早是.NET Framework 4.5引入,可以在Visual Studio 2012使用.在此之前的异步编程实现难度较高,async使异步编程的实现变得 ...
- C#的多线程——使用async和await来完成异步编程(Asynchronous Programming with async and await)
https://msdn.microsoft.com/zh-cn/library/mt674882.aspx 侵删 更新于:2015年6月20日 欲获得最新的Visual Studio 2017 RC ...
- Ext JS学习第十六天 事件机制event(一) DotNet进阶系列(持续更新) 第一节:.Net版基于WebSocket的聊天室样例 第十五节:深入理解async和await的作用及各种适用场景和用法 第十五节:深入理解async和await的作用及各种适用场景和用法 前端自动化准备和详细配置(NVM、NPM/CNPM、NodeJs、NRM、WebPack、Gulp/Grunt、G
code&monkey Ext JS学习第十六天 事件机制event(一) 此文用来记录学习笔记: 休息了好几天,从今天开始继续保持更新,鞭策自己学习 今天我们来说一说什么是事件,对于事件 ...
- C#扫盲篇(四):.NET Core 的异步编程-只讲干货(async,await,Task)
关于async,await,task的用法和解释这里就不要说明了,网上一查一大堆.至于为啥还要写这篇文章,主要是其他文章水分太多,不适合新手学习和理解.以下内容纯属个人理解,如果有误,请高手指正.本文 ...
- ASP.NET 中的 Async/Await 简介
本文转载自MSDN 作者:Stephen Cleary 原文地址:https://msdn.microsoft.com/en-us/magazine/dn802603.aspx 大多数有关 async ...
随机推荐
- webpack的版本进化史
一.概述2015,webpack1支持CMD和AMD,同时拥有丰富的plugin和loader,webpack逐渐得到广泛应用. 2016.12,webpack2相对于webpack1最大的改进就是支 ...
- Shiro笔记(五)JSP标签
Shiro笔记(五)JSP标签 导入标签库 <%@taglib prefix="shiro" uri="http://shiro.apache.org/tags&q ...
- Wannafly挑战赛25游记
Wannafly挑战赛25游记 A - 因子 题目大意: 令\(x=n!(n\le10^{12})\),给定一大于\(1\)的正整数\(p(p\le10000)\)求一个\(k\)使得\(p^k|x\ ...
- BZOJ2759一个动态树好题 LCT
题如其名啊 昨天晚上写了一发忘保存 只好今天又码一遍了 将题目中怕$p[i]$看做$i$的$father$ 可以发现每个联通块都是一个基环树 我们对每个基环删掉环上一条边 就可以得到一个森林了 可以用 ...
- db2报错信息
sqlcode sqlstate 说明000 00000 SQL语句成功完成01xxx SQL语句成功完成,但是有警告+012 01545 未限定的列名被解释为一个有相互关系的引用+098 01568 ...
- android:制作 Nine-Patch 图片
它是一种被特殊处理过的 png 图片,能够指定哪些区域可以被拉伸而 哪些区域不可以. 那么 Nine-Patch 图片到底有什么实际作用呢?我们还是通过一个例子来看一下吧.比如 说项目中有一张气泡样式 ...
- Deploying JAR Package & JSP Page in EBS R12.2.4 WLS
https://pan.baidu.com/s/1OomyeLdbGWxTtCKVcweo0w # Uninstall JAR JSP QRCODE 1.# 查找QRCODE相关文件位置 [root@ ...
- java中关于AtomicInteger的使用
在Java语言中,++i和i++操作并不是线程安全的,在使用的时候,不可避免的会用到synchronized关键字.而AtomicInteger则通过一种线程安全的加减操作接口.咳哟参考我之前写的一篇 ...
- Django Web开发学习笔记(4)
第四章 模板篇 上一章的内容,我们将HTML的代码和Python代码都混合在了在view.py的文件下.但是这样做的坏处无疑是明显的,引用DjangoBook的说法: 对页面设计进行的任何改变都必须对 ...
- Nginx 限制访问速率
本文测试的nginx版本为nginx version: nginx/1.12.2 Nginx 提供了 limit_rate 和limit_rate_after,举个例子来说明一下在需要限速的站点 se ...