(转)java并发编程--Executor框架
本文转自https://www.cnblogs.com/MOBIN/p/5436482.html
java并发编程--Executor框架
只要用到线程,就可以使用executor.,在开发中如果需要创建线程可优先考虑使用Executor,并非只有线程池可以使用executor,单线程也可以使用executor,因为executor提供了很多其他功能,包括线程状态,生命周期的管理。故,只要用到线程,就可以使用executor.
只要用到线程,就可以使用executor.,在开发中如果需要创建线程可优先考虑使用Executor
只要用到线程,就可以使用executor.在开发中如果需要创建线程可优先考虑使用Executor
public ThreadPoolExecutor(int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue,
ThreadFactory threadFactory,
RejectedExecutionHandler handler)
摘要:
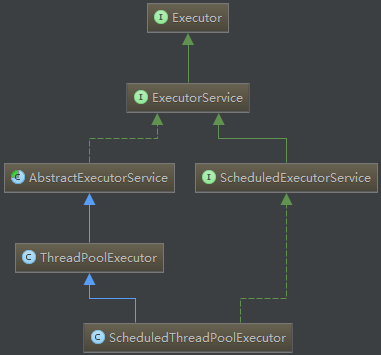


public ThreadPoolExecutor(int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue,
ThreadFactory threadFactory,
RejectedExecutionHandler handler) //后两个参数为可选参数

public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
//使用一个基于FIFO排序的阻塞队列,在所有corePoolSize线程都忙时新任务将在队列中等待
new LinkedBlockingQueue<Runnable>());
}

public static ExecutorService newSingleThreadExecutor() {
return new FinalizableDelegatedExecutorService
//corePoolSize和maximumPoolSize都等于,表示固定线程池大小为1
(new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>()));
}




1 public class HeartBeat {
2 public static void main(String[] args) {
3 ScheduledExecutorService executor = Executors.newScheduledThreadPool(5);
4 Runnable task = new Runnable() {
5 public void run() {
6 System.out.println("HeartBeat.........................");
7 }
8 };
9 executor.scheduleAtFixedRate(task,5,3, TimeUnit.SECONDS); //5秒后第一次执行,之后每隔3秒执行一次
10 }
11 }

HeartBeat....................... //5秒后第一次输出
HeartBeat....................... //每隔3秒输出一个
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
//使用同步队列,将任务直接提交给线程
new SynchronousQueue<Runnable>());
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
public class ThreadPoolTest { public static void main(String[] args) throws InterruptedException { ExecutorService threadPool = Executors.newCachedThreadPool(); //线程池里面的线程数会动态变化,并可在线程线被移除前重用 for ( int i = 1 ; i <= 3 ; i ++) { final int task = i; //10个任务 //TimeUnit.SECONDS.sleep(1); threadPool.execute( new Runnable() { //接受一个Runnable实例 public void run() { System.out.println( "线程名字: " + Thread.currentThread().getName() + " 任务名为: " +task); } }); } } } |
线程名字: pool-1-thread-1 任务名为: 1
线程名字: pool-1-thread-2 任务名为: 2
线程名字: pool-1-thread-3 任务名为: 3
线程名字: pool-1-thread-1 任务名为: 1
线程名字: pool-1-thread-1 任务名为: 2
线程名字: pool-1-thread-1 任务名为: 3
1
2
3
4
5
6
7
8
9
10
11
|
public class CallableAndFuture { public static void main(String[] args) throws ExecutionException, InterruptedException { ExecutorService executor = Executors.newSingleThreadExecutor(); Future<String> future = executor.submit( new Callable<String>() { //接受一上callable实例 public String call() throws Exception { return "MOBIN" ; } }); System.out.println( "任务的执行结果:" +future.get()); } } |
任务的执行结果:MOBIN
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
public class CompletionServiceTest { public static void main(String[] args) throws InterruptedException, ExecutionException { ExecutorService executor = Executors.newFixedThreadPool( 10 ); //创建含10.条线程的线程池 CompletionService completionService = new ExecutorCompletionService(executor); for ( int i = 1 ; i <= 10 ; i ++) { final int result = i; completionService.submit( new Callable() { public Object call() throws Exception { Thread.sleep( new Random().nextInt( 5000 )); //让当前线程随机休眠一段时间 return result; } }); } System.out.println(completionService.take().get()); //获取执行结果 } } |
3
(转)java并发编程--Executor框架的更多相关文章
- Java 并发编程——Executor框架和线程池原理
Eexecutor作为灵活且强大的异步执行框架,其支持多种不同类型的任务执行策略,提供了一种标准的方法将任务的提交过程和执行过程解耦开发,基于生产者-消费者模式,其提交任务的线程相当于生产者,执行任务 ...
- Java 并发编程——Executor框架和线程池原理
Java 并发编程系列文章 Java 并发基础——线程安全性 Java 并发编程——Callable+Future+FutureTask java 并发编程——Thread 源码重新学习 java并发 ...
- java并发编程-Executor框架
Executor框架是指java 5中引入的一系列并发库中与executor相关的一些功能类,其中包括线程池,Executor,Executors,ExecutorService,Completion ...
- Java 并发编程 Executor 框架
本文部分摘自<Java 并发编程的艺术> Excutor 框架 1. 两级调度模型 在 HotSpot VM 的线程模型中,Java 线程被一对一映射为本地操作系统线程.在上层,Java ...
- Java并发编程-Executor框架(转)
本文转自http://blog.csdn.net/chenchaofuck1/article/details/51606224 感谢作者 我们在传统多线程编程创建线程时,常常是创建一些Runnable ...
- Java并发编程-Executor框架集
Executor框架集对线程调度进行了封装,将任务提交和任务执行解耦. 它提供了线程生命周期调度的所有方法,大大简化了线程调度和同步的门槛. Executor框架集的核心类图如下: 从上往下,可以很清 ...
- java并发编程--Executor框架(一)
摘要: Eexecutor作为灵活且强大的异步执行框架,其支持多种不同类型的任务执行策略,提供了一种标准的方法将任务的提交过程和执行过程解耦开发,基于生产者-消费者模式,其提交任务的线程 ...
- java并发编程-Executor框架 + Callable + Future
from: https://www.cnblogs.com/shipengzhi/articles/2067154.html import java.util.concurrent.*; public ...
- java 并发编程 Executor框架
http://blog.csdn.net/chenchaofuck1/article/details/51606224 demo package executor; import java.util. ...
随机推荐
- Apache+Tomcat+mod_jk实现负载均衡
最近公司提出了负载均衡的新需求,以减轻网站的高峰期的服务器负担.趁空闲时间我就准备了一下这方面的知识,都说有备无患嘛.网上相关资料很多,但是太散.我希望可以通过这篇随笔,系统的总结. 一.Tomcat ...
- 006使用Grafana展示时间序列数据
简介 Grafana是一个独立运行的系统,内置了Web服务器.它可以基于仪表盘的方式来展示.分析时间序列数据. Grafana支持多种数据源,例如:Graphite.OpenTSDB.InfluxDB ...
- (转载)ibatis:解决sql注入问题
原文地址:http://blog.csdn.net/scorpio3k/article/details/7610973 对于ibaits参数引用可以使用#和$两种写法,其中#写法会采用预编译方式,将转 ...
- 文字小于12px时,设置line-height不居中问题
设置了文字了小于12px时,会存在设置了line-height的不生效的问题,主要是由于基线的问题,这篇文章解释的很清楚,有兴趣的可以看下https://blog.csdn.net/q12151634 ...
- css-实现图标在输入框中显示
一:JavaScript 是脚本语言 JavaScript 是一种轻量级的编程语言. JavaScript 是可插入 HTML 页面的编程代码. JavaScript 插入 HTML 页面后,可由所有 ...
- Android方法引用数超过65535优雅解决
随着应用不断迭代更新,业务线的扩展,应用越来越大(比如:集成了各种第三方SDK或者公共开源的Library文件.jar文件)这样一来,项目耦合性就很高,重复作用的类就越来越多了,SO:问题就来了.相信 ...
- ajax调用WebService 不能跨域
http://www.cnblogs.com/dojo-lzz/p/4265637.html "Access-Control-Allow-Origin":'http://local ...
- window10 显示QQ图标
- vs2010 快捷键
我自己的快捷键: visual studio 2010快捷键: visual studio 2010快捷键: 强迫智能感知:Ctrl+J撤销:Ctrl+Z强迫显示参数信息:Ctrl+Shift+空格重 ...
- input onchange事件
//输入帐号,默认赋值给员工电话和后台登录帐号$('#mobile_phone_').bind('input propertychange', function() { var phone = $(' ...