ACM学习历程——UVA11234 Expressions(栈,队列,树的遍历,后序遍历,bfs)
Description
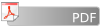
Problem E: Expressions2007/2008 ACM International Collegiate Programming Contest University of Ulm Local Contest
Problem E: Expressions
Arithmetic expressions are usually written with the operators in between the two operands (which is called infix notation). For example, (x+y)*(z-w) is an arithmetic expression in infix notation. However, it is easier to write a program to evaluate an expression if the expression is written in postfix notation (also known as reverse polish notation). In postfix notation, an operator is written behind its two operands, which may be expressions themselves. For example, x y + z w - * is a postfix notation of the arithmetic expression given above. Note that in this case parentheses are not required.
To evaluate an expression written in postfix notation, an algorithm operating on a stack can be used. A stack is a data structure which supports two operations:
- push: a number is inserted at the top of the stack.
- pop: the number from the top of the stack is taken out.
During the evaluation, we process the expression from left to right. If we encounter a number, we push it onto the stack. If we encounter an operator, we pop the first two numbers from the stack, apply the operator on them, and push the result back onto the stack. More specifically, the following pseudocode shows how to handle the case when we encounter an operator O:
a := pop();
b := pop();
push(b O a);
The result of the expression will be left as the only number on the stack.
Now imagine that we use a queue instead of the stack. A queue also has a push and pop operation, but their meaning is different:
- push: a number is inserted at the end of the queue.
- pop: the number from the front of the queue is taken out of the queue.
Can you rewrite the given expression such that the result of the algorithm using the queue is the same as the result of the original expression evaluated using the algorithm with the stack?
Input Specification
The first line of the input contains a number T (T ≤ 200). The following T lines each contain one expression in postfix notation. Arithmetic operators are represented by uppercase letters, numbers are represented by lowercase letters. You may assume that the length of each expression is less than 10000 characters.
Output Specification
For each given expression, print the expression with the equivalent result when using the algorithm with the queue instead of the stack. To make the solution unique, you are not allowed to assume that the operators are associative or commutative.
Sample Input
2
xyPzwIM
abcABdefgCDEF
Sample Output
wzyxIPM
gfCecbDdAaEBF 根据题目意思,运算符是二元的,故想到使用二叉树结构来存放所有元素。
根据题目意思,读入二叉树的过程是一个后序遍历的过程,故使用题目描述中的栈结构进行建树。
根据题目意思,输出过程是从树的最下层网上,一层层将树输出。
考虑到此处使用的是指针,故没有使用STL里面的队列,构造了Queue类,使用了循环队列。 代码:
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <cmath>
#include <algorithm>
#include <set>
#include <map>
#include <vector>
#include <queue>
#include <string>
#define inf 0x3fffffff
#define eps 1e-10 using namespace std; struct node
{
char val;
node *left;
node *right;
}; struct Queue
{
node *v[10005];
int top;
int rear;
const int N = 10005;
void Init()
{
top = 0;
rear = 0;
}
void Pop()
{
top = (top+1) % N;
}
node *Front()
{
return v[top];
}
void Push(node * a)
{
v[rear] = a;
rear = (rear+1) % N;
}
bool Empty()
{
if (top != rear)
return 0;
else
return 1;
}
}q; node *head, *Stack[10005];
int top; node *Create()
{
top = 0;
char ch;
for (;;)
{
ch = getchar();
if (ch == '\n')
{
return Stack[0];
}
if (ch >= 'a' && ch <= 'z')
{
node *k;
k = (node *)malloc(sizeof(node));
k->val = ch;
k->left = NULL;
k->right = NULL;
Stack[top++] = k;
}
else
{
node *k;
k = (node *)malloc(sizeof(node));
k->val = ch;
k->left = Stack[top-2];
k->right = Stack[top-1];
top -= 2;
Stack[top++] = k;
}
}
} void bfs()
{
q.Init();
q.Push(head);
top = 0;
while (!q.Empty())
{
Stack[top] = q.Front();
q.Pop();
if (Stack[top]->left != NULL)
{
q.Push(Stack[top]->left);
q.Push(Stack[top]->right);
}
top++;
}
} void Output()
{
for (int i = top-1; i >= 0; --i)
printf("%c", Stack[i]->val);
printf("\n");
} int main()
{
//freopen ("test.txt", "r", stdin);
int T;
scanf("%d", &T);
getchar();
for (int times = 1; times <= T; ++times)
{
head = Create();
bfs();
Output();
}
return 0;
}
ACM学习历程——UVA11234 Expressions(栈,队列,树的遍历,后序遍历,bfs)的更多相关文章
- 二叉树 Java 实现 前序遍历 中序遍历 后序遍历 层级遍历 获取叶节点 宽度 ,高度,队列实现二叉树遍历 求二叉树的最大距离
数据结构中一直对二叉树不是很了解,今天趁着这个时间整理一下 许多实际问题抽象出来的数据结构往往是二叉树的形式,即使是一般的树也能简单地转换为二叉树,而且二叉树的存储结构及其算法都较为简单,因此二叉树显 ...
- 剑指Offer的学习笔记(C#篇)-- 平衡二叉树(二叉树后序遍历递归详解版)
题目描述 输入一棵二叉树,判断该二叉树是否是平衡二叉树. 一 . 题目分析 首先要理解一个概念:什么是平衡二叉树,如果某二叉树中任意的左右子树深度相差不超过1,那么他就是一颗平衡二叉树.如下图: 所以 ...
- ACM学习历程—HDU 5289 Assignment(线段树 || RMQ || 单调队列)
Problem Description Tom owns a company and he is the boss. There are n staffs which are numbered fro ...
- ACM学习历程—HDU 2795 Billboard(线段树)
Description At the entrance to the university, there is a huge rectangular billboard of size h*w (h ...
- 利用树的先序和后序遍历打印 os 中的目录树
[0]README 0.1)本代码均为原创,旨在将树的遍历应用一下下以加深印象而已:(回答了学习树的遍历到底有什么用的问题?)你对比下linux 中的文件树 和我的打印结果就明理了: 0.2)我们采用 ...
- 二叉树中序遍历,先序遍历,后序遍历(递归栈,非递归栈,Morris Traversal)
例题 中序遍历94. Binary Tree Inorder Traversal 先序遍历144. Binary Tree Preorder Traversal 后序遍历145. Binary Tre ...
- ACM学习历程—SNNUOJ 1110 传输网络((并查集 && 离线) || (线段树 && 时间戳))(2015陕西省大学生程序设计竞赛D题)
Description Byteland国家的网络单向传输系统可以被看成是以首都 Bytetown为中心的有向树,一开始只有Bytetown建有基站,所有其他城市的信号都是从Bytetown传输过来的 ...
- 前、中、后序遍历随意两种是否能确定一个二叉树?理由? && 栈和队列的特点和区别
前序和后序不能确定二叉树理由:前序和后序在本质上都是将父节点与子结点进行分离,但并没有指明左子树和右子树的能力,因此得到这两个序列只能明确父子关系,而不能确定一个二叉树. 由二叉树的中序和前序遍历序列 ...
- python实现二叉树的建立以及遍历(递归前序、中序、后序遍历,队栈前序、中序、后序、层次遍历)
#-*- coding:utf-8 -*- class Node: def __init__(self,data): self.data=data self.lchild=None self.rchi ...
随机推荐
- java变参
java变参是通过数组来实现的 Object[] addAll(Object[] array1, Object... array2)和Object[] addAll(Object[] array1, ...
- (webstorm的css编写插件)Emmet:HTML/CSS代码快速编写神器
Emmet的前身是大名鼎鼎的Zen coding,如果你从事Web前端开发的话,对该插件一定不会陌生.它使用仿CSS选择器的语法来生成代码,大大提高了HTML/CSS代码编写的速度,比如下面的演示: ...
- 49 个jquery代码经典片段
49 个jquery代码经典片段,这些代码能够给你的javascript项目提供帮助.其中的一些代码段是从jQuery1.4.2才开始支持的做法,另一 些则是真正有用的函数或方法,他们能够帮助你又快又 ...
- warning: mysql-community-libs-5.7.11-1.el7.x86_64.rpm: Header V3 DSA/SHA1 Signature, key ID 5072e1f5
1.错误描写叙述 [root@ mysql]# rpm -ivh mysql-community-libs-5.7.11-1.el7.x86_64.rpm warning: mysql-communi ...
- HDFS源码分析心跳汇报之整体结构
我们知道,HDFS全称是Hadoop Distribute FileSystem,即Hadoop分布式文件系统.既然它是一个分布式文件系统,那么肯定存在很多物理节点,而这其中,就会有主从节点之分.在H ...
- 仿易讯clientloading效果
以下来实现一个loading效果.详细效果例如以下: 首先对这个效果进行拆分,它由以下部分组成: 1 一个"闪电"样式的图案. 2 "闪电"图案背后是一个圆角矩 ...
- spring mvc 及NUI前端框架学习笔记
spring mvc 及NUI前端框架学习笔记 页面传值 一.同一页面 直接通过$J.getbyName("id").setValue(id); Set值即可 二.跳转页面(bus ...
- cakephp 基本的环境
这里是在 window下的环境搭建,我假设php,apache,mysql,的基本环境都ok,如果没有的话,偷个懒,用集成环境吧,我用的wampserver.1:去官网(http://cakephp. ...
- MySql 三大知识点——索引、锁、事务(转)
1. 索引 索引,类似书籍的目录,可以根据目录的某个页码立即找到对应的内容. 索引的优点:1. 天生排序.2. 快速查找.索引的缺点:1. 占用空间.2. 降低更新表的速度. 注意点:小表使用全表扫描 ...
- 一起来学linux:例行性任务之at和crontab
对于我们日常生活来说,有很多例行需要进行的工作,比如每天早上起床一杯水,例如家人的生日,每天的起床时间等.这性例行的工作有可能被遗忘,但是如果我们用计算机来进行提醒的话,则方便很多.这里就要介绍到Li ...