poj 1981(单位圆覆盖最多点问题模板)
Time Limit: 5000MS | Memory Limit: 30000K | |
Total Submissions: 7327 | Accepted: 2651 | |
Case Time Limit: 2000MS |
Description
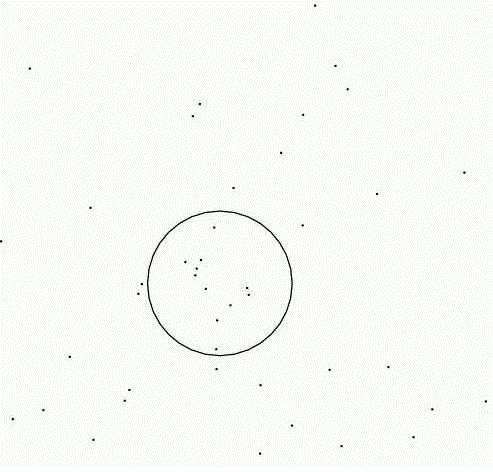
Fig 1. Circle and Points
Input
input consists of a series of data sets, followed by a single line only
containing a single character '0', which indicates the end of the input.
Each data set begins with a line containing an integer N, which
indicates the number of points in the data set. It is followed by N
lines describing the coordinates of the points. Each of the N lines has
two decimal fractions X and Y, describing the x- and y-coordinates of a
point, respectively. They are given with five digits after the decimal
point.
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0
<= Y <= 10.0. No two points are closer than 0.0001. No two points
in a data set are approximately at a distance of 2.0. More precisely,
for any two points in a data set, the distance d between the two never
satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a
data set are simultaneously very close to a single circle of radius one.
More precisely, let P1, P2, and P3 be any three points in a data set,
and d1, d2, and d3 the distances from an arbitrarily selected point in
the xy-plane to each of them respectively. Then it never simultaneously
holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
Output
each data set, print a single line containing the maximum number of
points in the data set that can be simultaneously enclosed by a circle
of radius one. No other characters including leading and trailing spaces
should be printed.
Sample Input
3
6.47634 7.69628
5.16828 4.79915
6.69533 6.20378
6
7.15296 4.08328
6.50827 2.69466
5.91219 3.86661
5.29853 4.16097
6.10838 3.46039
6.34060 2.41599
8
7.90650 4.01746
4.10998 4.18354
4.67289 4.01887
6.33885 4.28388
4.98106 3.82728
5.12379 5.16473
7.84664 4.67693
4.02776 3.87990
20
6.65128 5.47490
6.42743 6.26189
6.35864 4.61611
6.59020 4.54228
4.43967 5.70059
4.38226 5.70536
5.50755 6.18163
7.41971 6.13668
6.71936 3.04496
5.61832 4.23857
5.99424 4.29328
5.60961 4.32998
6.82242 5.79683
5.44693 3.82724
6.70906 3.65736
7.89087 5.68000
6.23300 4.59530
5.92401 4.92329
6.24168 3.81389
6.22671 3.62210
0
Sample Output
2
5
5
11
代码转自,不想去弄了。。以后就做模板用好了 http://www.cnblogs.com/-sunshine/archive/2012/10/11/2719859.html
贴个模板:
#include<stdio.h>
#include<iostream>
#include<string.h>
#include <stdlib.h>
#include<math.h>
#include<algorithm>
using namespace std;
const int N = ;
struct Point{
double x,y;
}p[N];
struct Node{
double angle;
bool in;
}arc[];
int n,cnt;
double R;
double dist(Point p1,Point p2){
return sqrt((p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y));
}
bool cmp(Node n1,Node n2){
return n1.angle!=n2.angle?n1.angle<n2.angle:n1.in>n2.in;
}
void MaxCircleCover(){
int ans=;
for(int i=;i<n;i++){
int cnt=;
for(int j=;j<n;j++){
if(i==j) continue;
if(dist(p[i],p[j])>R*) continue;
double angle=atan2(p[i].y-p[j].y,p[i].x-p[j].x);
double phi=acos(dist(p[i],p[j])/);
arc[cnt].angle=angle-phi;arc[cnt++].in=true;
arc[cnt].angle=angle+phi;arc[cnt++].in=false;
}
sort(arc,arc+cnt,cmp);
int tmp=;
for(int i=;i<cnt;i++){
if(arc[i].in) tmp++;
else tmp--;
ans=max(ans,tmp);
}
}
printf("%d\n",ans);
}
int main(){
while(scanf("%d",&n)!=EOF&&n){
//scanf("%lf",&R);
R = ; //此题R为1
for(int i=;i<n;i++)
scanf("%lf%lf",&p[i].x,&p[i].y);
MaxCircleCover();
}
return ;
}
poj 1981(单位圆覆盖最多点问题模板)的更多相关文章
- bzoj1338: Pku1981 Circle and Points单位圆覆盖
题目链接:http://www.lydsy.com/JudgeOnline/problem.php?id=1338 1338: Pku1981 Circle and Points单位圆覆盖 Time ...
- POJ 2914 - Minimum Cut - [stoer-wagner算法讲解/模板]
首先是当年stoer和wagner两位大佬发表的关于这个算法的论文:A Simple Min-Cut Algorithm 直接上算法部分: 分割线 begin 在这整篇论文中,我们假设一个普通无向图G ...
- poj 1981 Circle and Points
Circle and Points Time Limit: 5000MS Memory Limit: 30000K Total Submissions: 8131 Accepted: 2899 ...
- 【[Offer收割]编程练习赛14 D】剑刃风暴(半径为R的圆能够覆盖的平面上最多点数目模板)
[题目链接]:http://hihocoder.com/problemset/problem/1508 [题意] [题解] 求一个半径为R的圆能够覆盖的平面上的n个点中最多的点数; O(N2log2N ...
- POJ 3468 A Simple Problem with Integers (线段树多点更新模板)
题意: 给定一个区间, 每个区间有一个初值, 然后给出Q个操作, C a b c是给[a,b]中每个数加上c, Q a b 是查询[a,b]的和 代码: #include <cstdio> ...
- POJ-1981 Circle and Points 单位圆覆盖
题目链接:http://poj.org/problem?id=1981 容易想到直接枚举两个点,然后确定一个圆来枚举,算法复杂度O(n^3). 这题还有O(n^2*lg n)的算法.将每个点扩展为单位 ...
- POJ 1981 Circle and Points (扫描线)
[题目链接] http://poj.org/problem?id=1981 [题目大意] 给出平面上一些点,问一个半径为1的圆最多可以覆盖几个点 [题解] 我们对于每个点画半径为1的圆,那么在两圆交弧 ...
- 【POJ 1981】Circle and Points(已知圆上两点求圆心坐标)
[题目链接]:http://poj.org/problem?id=1981 [题意] 给你n个点(n<=300); 然后给你一个半径R: 让你在平面上找一个半径为R的圆; 这里R=1 使得这个圆 ...
- 【POJ 1981 】Circle and Points
当两个点距离小于直径时,由它们为弦确定的一个单位圆(虽然有两个圆,但是想一想知道只算一个就可以)来计算覆盖多少点. #include <cstdio> #include <cmath ...
随机推荐
- 真是shi
降雨量那题,真踏马shi. 调到还有五个RE不调了. 开始以为map可水后来发现一定要二分查找一下. 这种题没啥营养,不过我发现了我ST表一处错误并打了个板子.就这点用处吧. 这几天做题太少了,每天不 ...
- echo shell commands as they are executed
http://stackoverflow.com/questions/2853803/in-a-shell-script-echo-shell-commands-as-they-are-execute ...
- Java重写与重载
重写的规则: 参数列表必须完全与被重写方法的相同: 返回类型必须完全与被重写方法的返回类型相同: 访问权限不能比父类中被重写的方法的访问权限更低.例如:如果父类的一个方法被声明为public,那么在子 ...
- 一个python的文件对比脚本
脚本主要用来给游戏客户端做热更的. 处理方式就是针对每个文件求其MD5值,再根据文件的目录和名字对比两个版本的MD5值,如果不一样,则这次热更就需要更新这个文件. 用法很简单. 1,生成MD5码列表 ...
- 【APUE】Chapter7 Process Environment
这一章内容是Process的基础准备篇章.这一章的内容都是基于C Programm为例子. (一)进程开始: kernel → C start-up rountine → main function ...
- 【Search in Rotated Sorted Array II 】cpp
题目: Follow up for "Search in Rotated Sorted Array":What if duplicates are allowed? Would t ...
- Jmeter测试SOAP协议(Jmeter 3.3)
公司协议都是SOAP协议的,最初在网上看到Jmeter测试soap协议需要插件,但是Jmeter3.2开始就不在支持该插件,后来又查了些资料,找到了解决办法,Jmeter提供专门创建针对soap协议的 ...
- 关于windows10设置环境变量的问题
在设置环境变量的时候往往在网上能找到这样的文章: 1:新建环境变量 2:将新增的环境变量 加到path 变量中: 3.由于有的小伙伴的 系统是 windows10 在点击 编辑path 环境变量的时候 ...
- 孤荷凌寒自学python第三天 初识序列
孤荷凌寒自学python第三天 初识序列 (完整学习过程屏幕记录视频地址在文末,手写笔记在文末) Python的序列非常让我着迷,之前学习的其它编程语言中没有非常特别关注过序列这种类型的对象,而pyt ...
- [0] OpenCV_Notes - 琐碎
CV_8UC1,CV_8UC2,CV_8UC3等意思 一般的图像文件格式使用的是 Unsigned 8bits,CvMat矩阵对应的参数类型就是CV_8UC1,CV_8UC2,CV_8UC3.最后的C ...