[ACM] hdu 4418 Time travel (高斯消元求期望)
Time travel
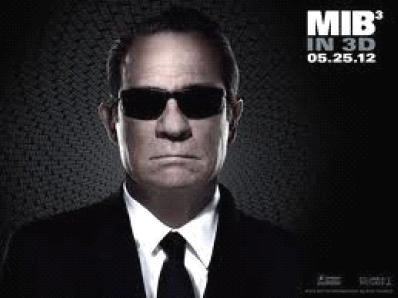
Agent K is one of the greatest agents in a secret organization called Men in Black. Once he needs to finish a mission by traveling through time with the Time machine. The Time machine can take agent K to some point (0 to n-1) on the timeline and when he gets
to the end of the time line he will come back (For example, there are 4 time points, agent K will go in this way 0, 1, 2, 3, 2, 1, 0, 1, 2, 3, 2, 1, ...). But when agent K gets into the Time machine he finds it has broken, which make the Time machine can't
stop (Damn it!). Fortunately, the time machine may get recovery and stop for a few minutes when agent K arrives at a time point, if the time point he just arrive is his destination, he'll go and finish his mission, or the Time machine will break again. The
Time machine has probability Pk% to recover after passing k time points and k can be no more than M. We guarantee the sum of Pk is 100 (Sum(Pk) (1 <= k <= M)==100). Now we know agent K will appear at the point X(D is the direction of the Time machine: 0 represents
going from the start of the timeline to the end, on the contrary 1 represents going from the end. If x is the start or the end point of the time line D will be -1. Agent K want to know the expectation of the amount of the time point he need to pass before
he arrive at the point Y to finish his mission.
If finishing his mission is impossible output "Impossible !" (no quotes )instead.
If finishing his mission is impossible output one line "Impossible !"
(no quotes )instead.
2
4 2 0 1 0
50 50
4 1 0 2 1
100
8.14
2.00
解题思路:
转自:http://972169909-qq-com.iteye.com/blog/1689107
题意:一个人在数轴上来回走。以pi的概率走i步i∈[1, m]。给定n(数轴长度)。m。e(终点),s(起点),d(方向)。求从s走到e经过的点数期望
解析:设E[x]是人从x走到e经过点数的期望值,显然对于终点有:E[e] = 0
一般的:E[x] = sum((E[x+i]+i) * p[i])(i∈[1, m])
(走i步经过i个点,所以是E[x+i]+i)
建立模型:高斯消元每一个变量都是一个互不同样的独立的状态,因为人站在一个点,另一个状态是方向!比如人站在x点。有两种状态向前、向后,不能都当成一种状态建立方程,所以要把两个方向化为一个方向从而使状态不受方向的影响
实现:
n个点翻过去(除了头尾两个点~~~)变为2*(n-1)个点,比如:
6个点:012345 ---> 0123454321
那么显然,从5開始向右走事实上就是相当于往回走
然后方向就由两个状态转化成一个状态的,然后每一个点就是仅仅有一种状态了,对每一个点建立方程高斯消元就可以
状态转移有E[x]=sum((E[x+i]+i)*p[i])
即 E[x]=sum(E[x+i]*p[i]) + sum(i*p[i])
即E[x]-E[x+1]*p[1]-E[x+2]*p[2]-.........-E[x+m]*p[m]=sum(i*p[i])
代码:
#include <iostream>
#include <string.h>
#include <stdio.h>
#include <algorithm>
#include <iomanip>
#include <queue>
#include <cmath>
using namespace std;
const double eps=1e-9;
double p[110];
bool vis[220];
int n,m,x,y,d;
double a[220][220];
int equ,var;//equ个方程,var个变量
double xi[220];//解集
bool free_x[220]; int sgn(double x)
{
return (x>eps)-(x<-eps);
} bool bfs()
{
memset(vis,0,sizeof(vis));
vis[x]=true;
queue<int>q;
q.push(x);
while(!q.empty())
{
int first=q.front();
q.pop();
for(int i=1;i<=m;i++)
{
int temp=(first+i)%n;
if(p[i]>=eps&&!vis[temp])
{
vis[temp]=true;
q.push(temp);
}
} }
if(vis[y]||vis[(n-y)%n])
return true;
return false;
} void create()
{
double sum=0;
for(int i=1;i<=m;i++)
sum+=p[i]*i;
memset(a,0,sizeof(a));
for(int i=0;i<n;i++)
{
a[i][i]=1;
if(!vis[i])
continue;
if(i==y||i==(n-y)%n)
{
a[i][n]=0;
continue;
}
a[i][n]=sum;
for(int j=1;j<=m;++j)
{
a[i][(i+j)%n]-=p[j];//一定不能写成a[i][(i+j)%n]=-p[j];//由于同一个点可能到达两次或多次。系数要叠加,切记! !!
}
}
} int gauss()
{
equ=n,var=n;
int i,j,k;
int max_r; // 当前这列绝对值最大的行.
int col; // 当前处理的列.
double temp;
int free_x_num;
int free_index;
// 转换为阶梯阵.
col=0; // 当前处理的列.
memset(free_x,true,sizeof(free_x));
for(k=0;k<equ&&col<var;k++,col++)
{
max_r=k;
for(i=k+1;i<equ;i++)
{
if(sgn(fabs(a[i][col])-fabs(a[max_r][col]))>0)
max_r=i;
}
if(max_r!=k)
{ // 与第k行交换.
for(j=k;j<var+1;j++)
swap(a[k][j],a[max_r][j]);
}
if(sgn(a[k][col])==0)
{ // 说明该col列第k行下面全是0了,则处理当前行的下一列.
k--; continue;
}
for(i=k+1;i<equ;i++)
{ // 枚举要删去的行.
if (sgn(a[i][col])!=0)
{
temp=a[i][col]/a[k][col];
for(j=col;j<var+1;j++)
{
a[i][j]=a[i][j]-a[k][j]*temp;
}
}
}
} for(i=k;i<equ;i++)
{
if (sgn(a[i][col])!=0)
return 0;
}
if(k<var)
{
for(i=k-1;i>=0;i--)
{
free_x_num=0;
for(j=0;j<var;j++)
{
if (sgn(a[i][j])!=0&&free_x[j])
free_x_num++,free_index=j;
}
if(free_x_num>1) continue;
temp=a[i][var];
for(j=0;j<var;j++)
{
if(sgn(a[i][j])!=0&&j!=free_index)
temp-=a[i][j]*xi[j];
}
xi[free_index]=temp/a[i][free_index];
free_x[free_index]=0;
}
return var-k;
} for (i=var-1;i>=0;i--)
{
temp=a[i][var];
for(j=i+1;j<var;j++)
{
if(sgn(a[i][j])!=0)
temp-=a[i][j]*xi[j];
}
xi[i]=temp/a[i][i];
}
return 1;
} int main()
{
int t;cin>>t;
while(t--)
{
cin>>n>>m>>y>>x>>d;
double temp;
for(int i=1;i<=m;i++)
{
cin>>temp;
p[i]=temp/100;
}
if(x==y)
{
cout<<"0.00"<<endl;
continue;
}
n=(n-1)*2;
if(d>0)
x=(n-x)%n;
if(!bfs())
{
cout<<"Impossible !"<<endl;
continue;
}
create();
if(!gauss())
cout<<"Impossible !"<<endl;
else
cout<<setiosflags(ios::fixed)<<setprecision(2)<<xi[x]<<endl;
}
return 0;
}
[ACM] hdu 4418 Time travel (高斯消元求期望)的更多相关文章
- hdu 4870 rating(高斯消元求期望)
Rating Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Sub ...
- HDU4870_Rating_双号从零单排_高斯消元求期望
原题链接:http://acm.hdu.edu.cn/showproblem.php?pid=4870 原题: Rating Time Limit: 10000/5000 MS (Java/Other ...
- hdu 4418 高斯消元求期望
Time travel Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total ...
- hdu 3992 AC自动机上的高斯消元求期望
Crazy Typewriter Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) ...
- hdu 2262 高斯消元求期望
Where is the canteen Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Ot ...
- HDU 5833 (2016大学生网络预选赛) Zhu and 772002(高斯消元求齐次方程的秩)
网络预选赛的题目……比赛的时候没有做上,确实是没啥思路,只知道肯定是整数分解,然后乘起来素数的幂肯定是偶数,然后就不知道该怎么办了… 最后题目要求输出方案数,首先根据题目应该能写出如下齐次方程(从别人 ...
- 高斯消元与期望DP
高斯消元可以解决一系列DP序混乱的无向图上(期望)DP DP序 DP序是一道DP的所有状态的一个排列,使状态x所需的所有前置状态都位于状态x前: (通俗的说,在一个状态转移方程中‘=’左侧的状态应该在 ...
- 【BZOJ2137】submultiple 高斯消元求伯努利数
[BZOJ2137]submultiple Description 设函数g(N)表示N的约数个数.现在给出一个数M,求出所有M的约数x的g(x)的K次方和. Input 第一行输入N,K.N表示M由 ...
- SPOJ HIGH(生成树计数,高斯消元求行列式)
HIGH - Highways no tags In some countries building highways takes a lot of time... Maybe that's bec ...
随机推荐
- UVA 10718 Bit Mask 贪心+位运算
题意:给出一个数N,下限L上限U,在[L,U]里面找一个整数,使得N|M最大,且让M最小. 很明显用贪心,用位运算搞了半天,样例过了后还是WA,没考虑清楚... 然后网上翻到了一个人家位运算一句话解决 ...
- Mac下MAMP初试体验
原创文章,转载请注明出处! 近期小学习了一下Mac下的Apache,Mysql,php.这里记录一下,以备忘 1 php 1.1 php返回值的測试 在MAMP下測试成功,直接echo返回所数据 1. ...
- NLP | 自然语言处理 - 标注问题与隐马尔科夫模型(Tagging Problems, and Hidden Markov Models)
什么是标注? 在自然语言处理中有一个常见的任务,即标注.常见的有:1)词性标注(Part-Of-Speech Tagging),将句子中的每一个词标注词性,比如名词.动词等:2)实体标注(Name E ...
- git使用说明
1,git clone git://github.com/schacon/simplegit.git git工作目录,暂存目录,本地代码仓库都有代码了. 2,git pull git://github ...
- C#判断文件是否正在被使用
生成文件的时候,如果该文件夹下的同名文件被打开(或者被使用),如果这时再生成一个同名文件,则会提示文件正在被占用. 解决方法有两个,一个是保存的文件名改成该文件夹下不存在的(随机数之类的XXOO都行, ...
- WM_DRAWITEM与DrawItem()的讨论(自绘)
http://blog.csdn.net/FlowShell/archive/2009/10/10/4648800.aspx 我在学习中经常遇到要重写DrawItem()的情况,但又有一个WM_DRA ...
- 发掘ListBox的潜力(一):自动调整横向滚动条宽度
<自绘ListBox的两种效果>一文帖出之后,从反馈信息来看,大家对这种小技巧还是很认同.接下来我将继续围绕ListBox写一系列的文章,进一步发掘ListBox的潜力,其中包括:自动调整 ...
- js获取手机型号和手机操作系统版本号
1.js 判断IOS版本号 先来观察 iOS 的 User-Agent 串: iPhone 4.3.2 系统:Mozilla/5.0 (iPhone; U; CPU iPhone OS 4_3_2 l ...
- SQL查询数据封装JavaBean对象
public static List getListBySql(String sql, Class cls){ List list = new ArrayList(); Connection ...
- NET5
ASP.NET5(RC1) - 翻译 前言 ASP.NET 5 是一次令人惊叹的对于ASP.NET的创新革命. 他将构建目标瞄准了 .NET Core CLR, 同时ASP.NET又是对于云服务进行优 ...