POJ 2553 The Bottom of Graph 强连通图题解
Description
Then G=(V,E) is called a directed graph.
Let n be a positive integer, and let p=(e1,...,en) be a sequence of length n of edges ei∈E such that ei=(vi,vi+1) for a sequence of vertices(v1,...,vn+1).
Then p is called a path from vertex v1 to vertex vn+1 in G and we say that vn+1 is reachable from v1, writing (v1→vn+1).
Here are some new definitions. A node v in a graph G=(V,E) is called a sink, if for every node w in G that is reachable from v, v is also reachable from w. The bottom of a graph is the subset of
all nodes that are sinks, i.e., bottom(G)={v∈V|∀w∈V:(v→w)⇒(w→v)}. You have to calculate the bottom of certain graphs.
Input
numbers in the set V={1,...,v}. You may assume that 1<=v<=5000. That is followed by a non-negative integer e and, thereafter, e pairs of vertex identifiers v1,w1,...,ve,we with
the meaning that (vi,wi)∈E. There are no edges other than specified by these pairs. The last test case is followed by a zero.
Output
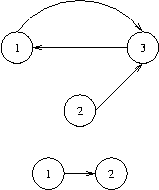
Sample Input
3 3
1 3 2 3 3 1
2 1
1 2
0
Sample Output
1 3
2
题意的本质是查找没有出度的强连通子图,没有出度就是sink。the bottom of graph了。
就是利用Tarjan算法求强连通子图,并要用标识号标识各个强连通子图,然后记录好各个顶点属于哪强连通子图。
程序带具体的注解:
#include <stdio.h>
#include <stdlib.h>
#include <vector>
#include <algorithm>
#include <stack>
using namespace std; const int MAX_V = 5001;
vector<int> graAdj[MAX_V];//vector表示的邻接图
int conNo, vToCon[MAX_V];//强连通子图标号及顶点相应强连通子图号的数组
int low[MAX_V];//标识最低标识号。假设都属于这个标识号的顶点都属于同一连通子图
int stk[MAX_V], top;//数组表示栈
bool vis[MAX_V];//记录是否訪问过的顶点
int out[MAX_V];//强连通子图的出度。假设出度为零。那么改强连通子图为sink template<typename T>
inline bool equ(T t1, T t2) { return t1 == t2; } void dfsTar(int u, int no = 1)
{
low[u] = no;//每递归进一个顶点。初始表示low[]
stk[++top] = u;//每一个顶点记录入栈
vis[u] = true;//标志好是否訪问过了 int n = (int)graAdj[u].size();
for (int i = 0; i < n; i++)
{
int v = graAdj[u][i];
if (!vis[v])
{
dfsTar(v, no+1);//这里递归
if (low[u] > low[v]) low[u] = low[v];//更新最低标识号
}
else if (!vToCon[v] && low[u] > low[v]) low[u] = low[v];//更新
}
if (equ(low[u], no))//最低标识号和递归进的初始号同样就找到一个子图了
{
++conNo;
int v;
do
{
v = stk[top--];//出栈
vToCon[v] = conNo;//顶点相应到子图号
} while (v != u);//出栈到本顶点,那么改子图全部顶点出栈完成
}
} void Tarjan(int n)
{
conNo = 0;//记得前期的清零工作
fill(vToCon, vToCon+n+1, 0);
fill(low, low+n+1, 0);
fill(vis, vis+n+1, false);
top = -1; for (int u = 1; u <= n; u++) if (!vis[u]) dfsTar(u);
} int main()
{
int V, E, u, v;
while(~scanf("%d %d", &V, &E) && V)
{
for (int i = 1; i <= V; i++)
{
graAdj[i].clear();//清零
}
for (int i = 0; i < E; i++)
{
scanf("%d %d", &u, &v);
graAdj[u].push_back(v);//建立vector表示的邻接表
}
Tarjan(V);
fill(out, out+conNo+1, 0);
for (int u = 1; u <= V; u++)
{
int n = graAdj[u].size();
for (int i = 0; i < n; i++)
{
int v = graAdj[u][i];
if (vToCon[u] != vToCon[v])
{
out[vToCon[u]]++;//记录强连通子图号的出度数
}
}
}
for (int u = 1; u <= V; u++)//出度为零,即为答案:Graph Bottom
{
if (!out[vToCon[u]]) printf("%d ", u);
}
putchar('\n');
}
return 0;
}
POJ 2553 The Bottom of Graph 强连通图题解的更多相关文章
- POJ 2553 The Bottom of a Graph(强连通分量)
POJ 2553 The Bottom of a Graph 题目链接 题意:给定一个有向图,求出度为0的强连通分量 思路:缩点搞就可以 代码: #include <cstdio> #in ...
- poj 2553 The Bottom of a Graph(强连通分量+缩点)
题目地址:http://poj.org/problem?id=2553 The Bottom of a Graph Time Limit: 3000MS Memory Limit: 65536K ...
- POJ 2553 The Bottom of a Graph (强连通分量)
题目地址:POJ 2553 题目意思不好理解.题意是:G图中从v可达的全部点w,也都能够达到v,这种v称为sink.然后升序输出全部的sink. 对于一个强连通分量来说,全部的点都符合这一条件,可是假 ...
- POJ 2553 The Bottom of a Graph Tarjan找环缩点(题解解释输入)
Description We will use the following (standard) definitions from graph theory. Let V be a nonempty ...
- POJ 2553 The Bottom of a Graph TarJan算法题解
本题分两步: 1 使用Tarjan算法求全部最大子强连通图.而且标志出来 2 然后遍历这些节点看是否有出射的边,没有的顶点所在的子强连通图的全部点,都是解集. Tarjan算法就是模板算法了. 这里使 ...
- POJ 2553 The Bottom of a Graph (Tarjan)
The Bottom of a Graph Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 11981 Accepted: ...
- poj 2553 The Bottom of a Graph : tarjan O(n) 存环中的点
/** problem: http://poj.org/problem?id=2553 将所有出度为0环中的点排序输出即可. **/ #include<stdio.h> #include& ...
- poj 2553 The Bottom of a Graph【强连通分量求汇点个数】
The Bottom of a Graph Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 9641 Accepted: ...
- poj - 2186 Popular Cows && poj - 2553 The Bottom of a Graph (强连通)
http://poj.org/problem?id=2186 给定n头牛,m个关系,每个关系a,b表示a认为b是受欢迎的,但是不代表b认为a是受欢迎的,关系之间还有传递性,假如a->b,b-&g ...
随机推荐
- svn log笔记
背景: svn,版本 1.7.8 以下所有命令直接和svn服务器进行交互并没有checkout代码到本地 1.查询分支从拉出来到现在的变更: svn log branche_url --stop-on ...
- 修复eclipse build-helper-maven-plugin 异常
安装 help --> install new http://repo1.maven.org/maven2/.m2e/connectors/m2eclipse-buildhelper/0.15. ...
- IOS版微信小视频导出方法
1.在电脑上连接手机,打开iTools 选择 应用-应用-文件共享. 2.依次打开/Library/WechatPrivate/6e2809aac61608de6a6cc55d9570d25b/Sig ...
- 关闭ubuntu终端的BELL声音
在shell提示符下面操作时有时会用到Tab来自动补全,这个时候系统就会发出BELL的声音,听了让人挺烦的. 有个方法能解决:编辑 /etc/inputrc,找到#set bell-style non ...
- Swift教程_swift常见问题(0005)_完美解决Cannot override 'dealloc'异常
Swift教程_swift常见问题(0001)_CoreData: warning: Unable to load class named 'xxx' for entity 'xxx' Swift教程 ...
- Oracle-client支持exp|imp|rman
官方精简版的驱动,不支持持exp/imp/rman,故需要安装oracle_client客户端. 实验环境: Centos6.5 x64 Oracle 11.2.0.4.0 Oracle_clie ...
- Linux ssh服务开启秘钥和密码认证
问题描述: 实现Linux秘钥和密码同时认证 解决方案: vim /etc/ssh/sshd_config 基本参数: PermitRootLogin yes #允许root认证登录 Password ...
- Linux 禁止用户或 IP通过 SSH 登录
一切都是为了安全,做到来着可知! 限制用户 SSH 登录 1.只允许指定用户进行登录(白名单): 在 /etc/ssh/sshd_config 配置文件中设置 AllowUsers ...
- 使用c:forEach 控制5个换行
今天做项目的时候碰到一个问题,我须要显示不确定数目的图片在网页上(图片是从数据库查出来的),用的是<c:forEach>循环取值的.就须要做成一行显示固定个数的图片.代码例如以下(我这里是 ...
- Android网络开发之基本介绍
Android平台浏览器采用WebKit引擎,名为ChormeLite,拥有强大扩展特性,每个开发者都可以编写自己的插件. 目前,Android平台有3种网络接口可以使用,分别是:java.net, ...