poj1151==codevs 3044 矩形面积求并
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 21511 | Accepted: 8110 |
Description
Input
The input file is terminated by a line containing a single 0. Don't process it.
Output
Output a blank line after each test case.
Sample Input
- 2
- 10 10 20 20
- 15 15 25 25.5
- 0
Sample Output
- Test case #1
- Total explored area: 180.00
Source
注意到要表示一个矩形,只需要知道其2个顶点的坐标就可以了(最左下,最右上)。可以用2个数组x[0...2n-1],y[0...2n-1]记录下矩形Ri的2个坐标(x1,y1),(x2,y2),然后将数组x[0...xn-1],y[0...2n-1]排序,为下一步的扫描线作准备,这就是离散化的思想。这题还可以用线段树做进一步优化,但是这里只介绍离散化的思想。
看下面这个例子:有2个矩形(1,1),(3,3)和(2,2),(4,4)。如图:
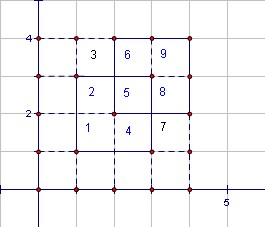
图中虚线表示扫描线,下一步工作只需要将这2个矩形覆盖过的部分的bool数组的对应位置更新为true,接下去用扫描线从左到右,从上到下扫描一遍,就可以求出矩形覆盖的总面积。
这个图对应的bool数组的值如下:
1 1 0 1 2 3
1 1 1 <----> 4 5 6
0 1 1 7 8 9
- #include<cstdio>
- #include<cstring>
- #include<cmath>
- #include<iostream>
- #include<algorithm>
- using namespace std;
- const int N=;
- const double eps=1e-;
- double ans=,x[N<<],y[N<<],pos[N][];
- bool hash[N<<][N<<];
- int cmp(const void *a,const void *b){
- double *aa=(double *)a;
- double *bb=(double *)b;
- if(fabs(*aa-*bb)<=eps) return ;
- else if(*aa-*bb>) return ;
- return -;
- }
- int main(){int i,j,k,n,x1,y1,x2,y2,cas=;
- while(scanf("%d",&n)==){
- if(!n) break;
- for(ans=k=i=;i<n;i++,k+=){
- scanf("%lf%lf%lf%lf",&pos[i][],&pos[i][],&pos[i][],&pos[i][]);
- x[k]=pos[i][];y[k]=pos[i][];x[k+]=pos[i][];y[k+]=pos[i][];
- }
- memset(hash,,sizeof hash);
- qsort(x,n<<,sizeof x[],cmp);
- qsort(y,n<<,sizeof y[],cmp);
- for(i=;i<n;i++){
- for(k=;fabs(x[k]-pos[i][])>eps;k++); x1=k;
- for(k=;fabs(y[k]-pos[i][])>eps;k++); y1=k;
- for(k=;fabs(x[k]-pos[i][])>eps;k++); x2=k;
- for(k=;fabs(y[k]-pos[i][])>eps;k++); y2=k;
- for(j=x1;j<x2;j++){
- for(k=y1;k<y2;k++){
- hash[j][k]=;
- }
- }
- }
- for(i=;i<*n-;i++){
- for(j=;j<*n-;j++){
- ans+=hash[i][j]*(x[i+]-x[i])*(y[j+]-y[j]);
- }
- }
- printf("Test case #%d\n",++cas);
- printf("Total explored area: %.2lf\n\n",ans);
- }
- return ;
- }
poj1151==codevs 3044 矩形面积求并的更多相关文章
- codevs 3044 矩形面积求并
3044 矩形面积求并 题目描述 Description 输入n个矩形,求他们总共占地面积(也就是求一下面积的并) 输入描述 Input Description 可能有多组数据,读到n=0为止(不 ...
- codevs 3044 矩形面积求并 (扫描线)
/* 之前一直偷懒离散化+暴力做着题 今天搞一下扫描线 自己按照线段树的一般写法写的有些问题 因为不用于以前的区间sum so 题解搬运者23333 Orz~ 去掉了打标记的过程 同时更新区间的时候先 ...
- codevs 3044 矩形面积求并 || hdu 1542
这个线段树的作用其实是维护一组(1维 平面(?) 上的)线段覆盖的区域的总长度,支持加入/删除一条线段. 线段树只能维护整数下标,因此要离散化. 也可以理解为将每一条处理的线段分解为一些小线段,要求每 ...
- codves 3044 矩形面积求并
codves 3044 矩形面积求并 题目等级 : 钻石 Diamond 题目描述 Description 输入n个矩形,求他们总共占地面积(也就是求一下面积的并) 输入描述 Input Desc ...
- 【题解】codevs 3044 矩形面积合并
传送门 3044 矩形面积求并 时间限制: 1 s 空间限制: 256000 KB 题目等级 : 钻石 Diamond 题目描述 Description 输入n个矩形,求他们总共占地面积(也就是求一下 ...
- 3044 矩形面积求并 - Wikioi
题目描述 Description 输入n个矩形,求他们总共占地面积(也就是求一下面积的并) 输入描述 Input Description 可能有多组数据,读到n=0为止(不超过15组) 每组数据第一行 ...
- [Codevs] 矩形面积求并
http://codevs.cn/problem/3044/ 线段树扫描线矩形面积求并 基本思路就是将每个矩形的长(平行于x轴的边)投影到线段树上 下边+1,上边-1: 然后根据线段树的权值和与相邻两 ...
- [codevs3044][POJ1151]矩形面积求并
[codevs3044][POJ1151]矩形面积求并 试题描述 输入n个矩形,求他们总共占地面积(也就是求一下面积的并) 输入 可能有多组数据,读到n=0为止(不超过15组) 每组数据第一行一个数n ...
- 矩形面积求并(codevs 3044)
题目描述 Description 输入n个矩形,求他们总共占地面积(也就是求一下面积的并) 输入描述 Input Description 可能有多组数据,读到n=0为止(不超过15组) 每组数据第一行 ...
随机推荐
- [PyTorch] rnn,lstm,gru中输入输出维度
本文中的RNN泛指LSTM,GRU等等 CNN中和RNN中batchSize的默认位置是不同的. CNN中:batchsize的位置是position 0. RNN中:batchsize的位置是pos ...
- ajax 传参数 数组 会加上中括号
解决办法 1,由于版本过高导致 我用的是1.9版本 2, 有三种选择. 一种是JSON.stringify([1,2,3]),到后端再解析. 另外一种是后端的接受的contentType改成appli ...
- 启发式合并CSU - 1811
F - Tree Intersection CSU - 1811 Bobo has a tree with n vertices numbered by 1,2,…,n and (n-1) edges ...
- MySQL和Oracle的比较
可以从以下几个方面来进行比较: (1) 对事务的提交 MySQL默认是自动提交,而Oracle默认不自动提交,需要用户手动提交,需要在写commit;指令或者点击commit按钮(2) 分页查询 ...
- java使用ant.jar解压缩文件
ant.jar下载地址http://ant.apache.org/bindownload.cgi 压缩文件代码: import org.apache.tools.ant.Project; import ...
- ubuntu 安装 navicat
下载navicat解压到opt目录 创建桌面快捷方式sudo vim /usr/share/applications/navicat.desktop [Desktop Entry] Encoding= ...
- bzoj3262陌上花开 cdq分治入门题
Description 有n朵花,每朵花有三个属性:花形(s).颜色(c).气味(m),又三个整数表示.现要对每朵花评级,一朵花的级别是它拥有的美丽能超过的花的数量.定义一朵花A比另一朵花B要美丽,当 ...
- mysql和Oracle 备份表
1.SQL Server中,如果目标表存在: insert into 目标表 select * from 原表; 2.SQL Server中,,如果目标表不存在: select * into 目标表 ...
- hash存储结构【六】
一.概述: 我们可以将Redis中的Hashes类型看成具有String Key和String Value的map容器.所以该类型非常适合于存储值对象的信息.如Username.Password和Ag ...
- Gym 100792 King's Rout 拓扑排序
K. King's Rout time limit per test 4.0 s memory limit per test 512 MB input standard input output st ...