python走起之第三话
一. SET集合
set是一个无序且不重复的元素集
class set(object):
"""
set() -> new empty set object
set(iterable) -> new set object Build an unordered collection of unique elements.
"""
def add(self, *args, **kwargs): # real signature unknown
""" 添加 """
"""
Add an element to a set. This has no effect if the element is already present.
"""
pass def clear(self, *args, **kwargs): # real signature unknown
""" Remove all elements from this set. """
pass def copy(self, *args, **kwargs): # real signature unknown
""" Return a shallow copy of a set. """
pass def difference(self, *args, **kwargs): # real signature unknown
"""
Return the difference of two or more sets as a new set. (i.e. all elements that are in this set but not the others.)
"""
pass def difference_update(self, *args, **kwargs): # real signature unknown
""" 删除当前set中的所有包含在 new set 里的元素 """
""" Remove all elements of another set from this set. """
pass def discard(self, *args, **kwargs): # real signature unknown
""" 移除元素 """
"""
Remove an element from a set if it is a member. If the element is not a member, do nothing.
"""
pass def intersection(self, *args, **kwargs): # real signature unknown
""" 取交集,新创建一个set """
"""
Return the intersection of two or more sets as a new set. (i.e. elements that are common to all of the sets.)
"""
pass def intersection_update(self, *args, **kwargs): # real signature unknown
""" 取交集,修改原来set """
""" Update a set with the intersection of itself and another. """
pass def isdisjoint(self, *args, **kwargs): # real signature unknown
""" 如果没有交集,返回true """
""" Return True if two sets have a null intersection. """
pass def issubset(self, *args, **kwargs): # real signature unknown
""" 是否是子集 """
""" Report whether another set contains this set. """
pass def issuperset(self, *args, **kwargs): # real signature unknown
""" 是否是父集 """
""" Report whether this set contains another set. """
pass def pop(self, *args, **kwargs): # real signature unknown
""" 移除 """
"""
Remove and return an arbitrary set element.
Raises KeyError if the set is empty.
"""
pass def remove(self, *args, **kwargs): # real signature unknown
""" 移除 """
"""
Remove an element from a set; it must be a member. If the element is not a member, raise a KeyError.
"""
pass def symmetric_difference(self, *args, **kwargs): # real signature unknown
""" 差集,创建新对象"""
"""
Return the symmetric difference of two sets as a new set. (i.e. all elements that are in exactly one of the sets.)
"""
pass def symmetric_difference_update(self, *args, **kwargs): # real signature unknown
""" 差集,改变原来 """
""" Update a set with the symmetric difference of itself and another. """
pass def union(self, *args, **kwargs): # real signature unknown
""" 并集 """
"""
Return the union of sets as a new set. (i.e. all elements that are in either set.)
"""
pass def update(self, *args, **kwargs): # real signature unknown
""" 更新 """
""" Update a set with the union of itself and others. """
pass def __and__(self, y): # real signature unknown; restored from __doc__
""" x.__and__(y) <==> x&y """
pass def __cmp__(self, y): # real signature unknown; restored from __doc__
""" x.__cmp__(y) <==> cmp(x,y) """
pass def __contains__(self, y): # real signature unknown; restored from __doc__
""" x.__contains__(y) <==> y in x. """
pass def __eq__(self, y): # real signature unknown; restored from __doc__
""" x.__eq__(y) <==> x==y """
pass def __getattribute__(self, name): # real signature unknown; restored from __doc__
""" x.__getattribute__('name') <==> x.name """
pass def __ge__(self, y): # real signature unknown; restored from __doc__
""" x.__ge__(y) <==> x>=y """
pass def __gt__(self, y): # real signature unknown; restored from __doc__
""" x.__gt__(y) <==> x>y """
pass def __iand__(self, y): # real signature unknown; restored from __doc__
""" x.__iand__(y) <==> x&=y """
pass def __init__(self, seq=()): # known special case of set.__init__
"""
set() -> new empty set object
set(iterable) -> new set object Build an unordered collection of unique elements.
# (copied from class doc)
"""
pass def __ior__(self, y): # real signature unknown; restored from __doc__
""" x.__ior__(y) <==> x|=y """
pass def __isub__(self, y): # real signature unknown; restored from __doc__
""" x.__isub__(y) <==> x-=y """
pass def __iter__(self): # real signature unknown; restored from __doc__
""" x.__iter__() <==> iter(x) """
pass def __ixor__(self, y): # real signature unknown; restored from __doc__
""" x.__ixor__(y) <==> x^=y """
pass def __len__(self): # real signature unknown; restored from __doc__
""" x.__len__() <==> len(x) """
pass def __le__(self, y): # real signature unknown; restored from __doc__
""" x.__le__(y) <==> x<=y """
pass def __lt__(self, y): # real signature unknown; restored from __doc__
""" x.__lt__(y) <==> x<y """
pass @staticmethod # known case of __new__
def __new__(S, *more): # real signature unknown; restored from __doc__
""" T.__new__(S, ...) -> a new object with type S, a subtype of T """
pass def __ne__(self, y): # real signature unknown; restored from __doc__
""" x.__ne__(y) <==> x!=y """
pass def __or__(self, y): # real signature unknown; restored from __doc__
""" x.__or__(y) <==> x|y """
pass def __rand__(self, y): # real signature unknown; restored from __doc__
""" x.__rand__(y) <==> y&x """
pass def __reduce__(self, *args, **kwargs): # real signature unknown
""" Return state information for pickling. """
pass def __repr__(self): # real signature unknown; restored from __doc__
""" x.__repr__() <==> repr(x) """
pass def __ror__(self, y): # real signature unknown; restored from __doc__
""" x.__ror__(y) <==> y|x """
pass def __rsub__(self, y): # real signature unknown; restored from __doc__
""" x.__rsub__(y) <==> y-x """
pass def __rxor__(self, y): # real signature unknown; restored from __doc__
""" x.__rxor__(y) <==> y^x """
pass def __sizeof__(self): # real signature unknown; restored from __doc__
""" S.__sizeof__() -> size of S in memory, in bytes """
pass def __sub__(self, y): # real signature unknown; restored from __doc__
""" x.__sub__(y) <==> x-y """
pass def __xor__(self, y): # real signature unknown; restored from __doc__
""" x.__xor__(y) <==> x^y """
pass __hash__ = None set Set
练习:寻找差异,打印出需要更新的、新增的、删除的
# 数据库中原有
old_dict
=
{
"#1"
:{
'hostname'
:c1,
'cpu_count'
:
2
,
'mem_capicity'
:
80
},
"#2"
:{
'hostname'
:c1,
'cpu_count'
:
2
,
'mem_capicity'
:
80
}
"#3"
:{
'hostname'
:c1,
'cpu_count'
:
2
,
'mem_capicity'
:
80
}
}
# cmdb 新汇报的数据
new_dict
=
{
"#1"
:{
'hostname'
:c1,
'cpu_count'
:
2
,
'mem_capicity'
:
800
},
"#3"
:{
'hostname'
:c1,
'cpu_count'
:
2
,
'mem_capicity'
:
80
}
"#4"
:{
'hostname'
:c2,
'cpu_count'
:
2
,
'mem_capicity'
:
80
}
}
# 数据库中原有
old_dict = {
"#1":{ 'hostname':'1.1.1.1', 'cpu_count': 2, 'mem_capicity': 80 },
"#2":{ 'hostname':'1.1.1.1', 'cpu_count': 2, 'mem_capicity': 80 },
"#3":{ 'hostname':'1.1.1.1', 'cpu_count': 2, 'mem_capicity': 80 }
} # cmdb 新汇报的数据
new_dict = {
"#1":{ 'hostname':'1.1.1.1', 'cpu_count': 2, 'mem_capicity': 800 },
"#3":{ 'hostname':'1.1.1.1', 'cpu_count': 2, 'mem_capicity': 80 },
"#4":{ 'hostname':'2.2.2.2', 'cpu_count': 2, 'mem_capicity': 80 }
} old_set = set(old_dict.keys())
update_list = list(old_set.intersection(new_dict.keys())) new_list = []
del_list = [] for i in new_dict.keys():
if i not in update_list:
new_list.append(i) for i in old_dict.keys():
if i not in update_list:
del_list.append(i) print(update_list,new_list,del_list) demo
二、深浅拷贝
对于 数字 和 字符串 而言,赋值、浅拷贝和深拷贝无意义,因为其永远指向同一个内存地址。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
import copy # ######### 数字、字符串 ######### n1 = 123 # n1 = "i am alex age 10" print ( id (n1)) # ## 赋值 ## n2 = n1 print ( id (n2)) # ## 浅拷贝 ## n2 = copy.copy(n1) print ( id (n2)) # ## 深拷贝 ## n3 = copy.deepcopy(n1) print ( id (n3)) |
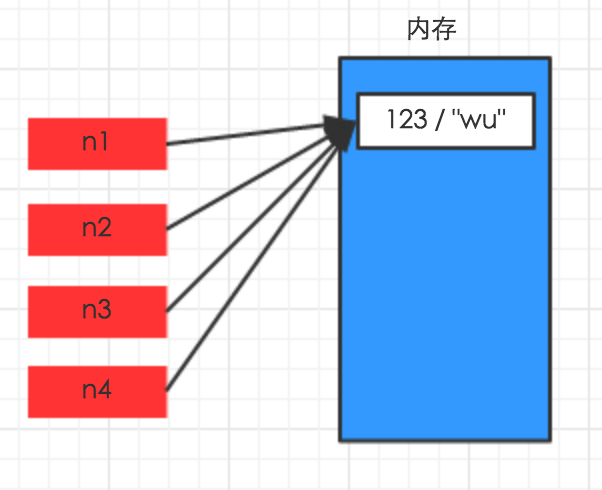
对于字典、元祖、列表 而言,进行赋值、浅拷贝和深拷贝时,其内存地址的变化是不同的。
赋值,只是创建一个变量,该变量指向原来内存地址,如:
1
2
3
|
n1 = { "k1" : "wu" , "k2" : 123 , "k3" : [ "alex" , 456 ]} n2 = n1 |
浅拷贝,在内存中只额外创建第一层数据
1
2
3
4
5
|
import copy n1 = { "k1" : "wu" , "k2" : 123 , "k3" : [ "alex" , 456 ]} n3 = copy.copy(n1) |
三、函数
在学习函数之前,一直遵循:面向过程编程,即:根据业务逻辑从上到下实现功能,其往往用一长段代码来实现指定功能,开发过程中最常见的操作就是粘贴复制,也就是将之前实现的代码块复制到现需功能处,如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
while True : if cpu利用率 > 90 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 if 硬盘使用空间 > 90 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 if 内存占用 > 80 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 |
腚眼一看上述代码,if条件语句下的内容可以被提取出来公用,如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
def 发送邮件(内容) #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 while True : if cpu利用率 > 90 % : 发送邮件( 'CPU报警' ) if 硬盘使用空间 > 90 % : 发送邮件( '硬盘报警' ) if 内存占用 > 80 % : |
函数的定义主要有如下要点:
- def:表示函数的关键字
- 函数名:函数的名称,日后根据函数名调用函数
- 函数体:函数中进行一系列的逻辑计算,如:发送邮件、计算出 [11,22,38,888,2]中的最大数等...
- 参数:为函数体提供数据
- 返回值:当函数执行完毕后,可以给调用者返回数据。
以上要点中,比较重要有参数和返回值:
1、返回值
函数是一个功能块,该功能到底执行成功与否,需要通过返回值来告知调用者。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
def 发送短信(): 发送短信的代码... if 发送成功: return True else : return False while True : # 每次执行发送短信函数,都会将返回值自动赋值给result # 之后,可以根据result来写日志,或重发等操作 result = 发送短信() if result = = False : 记录日志,短信发送失败... |
四、open函数
该函数用于文件处理,操作文件时,一般需要经历如下步骤:
- 打开文件
- 操作文件
1、打开文件
1
|
文件句柄 = open ( '文件路径' , '模式' ) |
打开文件时,需要指定文件路径和以何等方式打开文件,打开后,即可获取该文件句柄,日后通过此文件句柄对该文件操作。
打开文件的模式有:
- r,只读模式(默认)。
- w,只写模式。【不可读;不存在则创建;存在则删除内容;】
- a,追加模式。【可读; 不存在则创建;存在则只追加内容;】
"+" 表示可以同时读写某个文件
- r+,可读写文件。【可读;可写;可追加】
- w+,写读
- a+,同a
"U"表示在读取时,可以将 \r \n \r\n自动转换成 \n (与 r 或 r+ 模式同使用)
- rU
- r+U
"b"表示处理二进制文件(如:FTP发送上传ISO镜像文件,linux可忽略,windows处理二进制文件时需标注)
- rb
- wb
- ab
python走起之第三话的更多相关文章
- python走起之第八话
1. Socket介绍 概念 A network socket is an endpoint of a connection across a computer network. Today, mos ...
- python走起之第六话
面向过程 VS 面向对象 编程范式 编程是 程序 员 用特定的语法+数据结构+算法组成的代码来告诉计算机如何执行任务的过程 , 一个程序是程序员为了得到一个任务结果而编写的一组指令的集合,正所谓条条大 ...
- python走起之第四话
本节大纲: 一:双层装饰器:一个函数可以被多层装饰器进行装饰,函数渲染(编译)从下到上,函数执行从上到下.如下程序: 1 #!/usr/bin/env python 2 #-*-coding:utf- ...
- python走起之第五话
模块 1.自定义模块 自定义模块就是在当前目录下创建__init__.py这个空文件,这样外面的程序才能识别此目录为模块包并导入 上图中libs目录下有__init__.py文件,index.py程序 ...
- python走起之第十七话
选择器 #id 概述 根据给定的ID匹配一个元素. 使用任何的元字符(如 !"#$%&'()*+,./:;<=>?@[\]^`{|}~)作为名称的文本部分, 它必须被两个 ...
- python走起之第十三话
前景介绍 到目前为止,很多公司对堡垒机依然不太感冒,其实是没有充分认识到堡垒机在IT管理中的重要作用的,很多人觉得,堡垒机就是跳板机,其实这个认识是不全面的,跳板功能只是堡垒机所具备的功能属性中的其中 ...
- 进击的Python【第六章】:Python的高级应用(三)面向对象编程
Python的高级应用(三)面向对象编程 本章学习要点: 面向对象编程介绍 面向对象与面向过程编程的区别 为什么要用面向对象编程思想 面向对象的相关概念 一.面向对象编程介绍 面向对象程序设计(英语: ...
- 漫话JavaScript与异步·第三话——Generator:化异步为同步
一.Promise并非完美 我在上一话中介绍了Promise,这种模式增强了事件订阅机制,很好地解决了控制反转带来的信任问题.硬编码回调执行顺序造成的"回调金字塔"问题,无疑大大提 ...
- python列表和字符串的三种逆序遍历方式
python列表和字符串的三种逆序遍历方式 列表的逆序遍历 a = [1,3,6,8,9] print("通过下标逆序遍历1:") for i in a[::-1]: print( ...
随机推荐
- JS写的CRC16校验算法
var CRC = {}; CRC.CRC16 = function (data) { var len = data.length; if (len > 0) { var crc = 0xFFF ...
- Indexing and Hashing
DATABASE SYSTEM CONCEPTS, SIXTH EDITION11.1 Basic ConceptsAn index for a file in a database system wo ...
- Android如何通过shareduserid获取系统权限
[原文] android会为每个apk进程分配一个单独的空间(比如只能访问/data/data/自己包名下面的文件),一般情况下apk之间是禁止相互访问数据的.通过Shared User id,拥有同 ...
- js串讲回顾
注:1.xx.nextSibling.css.xxx->xx的下一个元素的css样式;2. window.opener.document.getElementById("cms&quo ...
- zepto源码--核心方法6(显示隐藏)--学习笔记
在不引入zepto插件模块fx_metho其他ds的情况下,zepto默认的显示隐藏的函数只有show, hide, toggle,这里解释有个前提条件,就是没有引入zepto的fx_methods插 ...
- canvas 实现 柱状图
define([],function(){ var myChart={ init:function(options){ this.ctx = options.ctx; this.data = opti ...
- Visual Studio 2012 常用快捷键
1. 强迫智能感知:Ctrl+J:2.强迫智能感知显示参数信息:Ctrl-Shift-空格:3.格式化整个块:Ctrl+K+F4. 检查括号匹配(在左右括号间切换): Ctrl +]5. 选中从光标起 ...
- iOS 宏(define)与常量(const)的正确使用
在iOS开发中,经常用到宏定义,或用const修饰一些数据类型,经常有开发者不知怎么正确使用,导致项目中乱用宏与const修饰 你能区分下面的吗?知道什么时候用吗? #define HSCoder @ ...
- Python基础(深、浅拷贝)
深.浅拷贝 基础:对象,引用,可变与可变 对于不可变的对象,如字符串.元组.数字深浅拷贝没有什么意义. 1.浅拷贝 浅拷贝只拷贝第一层对象(拷贝的实际是一个框子,拷贝过去的框子是不会变的,但是原先的框 ...
- [Android Tips] 4. Dismiss PopupWindow when touch outside
PopupWindow.setFocusable(true);