弄明白python reduce 函数
作者:Panda Fang
出处:http://www.cnblogs.com/lonkiss/p/understanding-python-reduce-function.html
原创文章,转载请注明作者和出处,未经允许不可用于商业营利活动
reduce() 函数在 python 2 是内置函数, 从python 3 开始移到了 functools 模块。
官方文档是这样介绍的
reduce(...)
reduce(function, sequence[, initial]) -> valueApply a function of two arguments cumulatively to the items of a sequence,
from left to right, so as to reduce the sequence to a single value.
For example, reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]) calculates
((((1+2)+3)+4)+5). If initial is present, it is placed before the items
of the sequence in the calculation, and serves as a default when the
sequence is empty.从左到右对一个序列的项累计地应用有两个参数的函数,以此合并序列到一个单一值。
例如,reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]) 计算的就是((((1+2)+3)+4)+5)。
如果提供了 initial 参数,计算时它将被放在序列的所有项前面,如果序列是空的,它也就是计算的默认结果值了
嗯, 这个文档其实不好理解。看了还是不懂。 序列 其实就是python中 tuple list dictionary string 以及其他可迭代物,别的编程语言可能有数组。
reduce 有 三个参数
function | 有两个参数的函数, 必需参数 |
sequence | tuple ,list ,dictionary, string等可迭代物,必需参数 |
initial | 初始值, 可选参数 |
reduce的工作过程是 :在迭代sequence(tuple ,list ,dictionary, string等可迭代物)的过程中,首先把 前两个元素传给 函数参数,函数加工后,然后把得到的结果和第三个元素作为两个参数传给函数参数, 函数加工后得到的结果又和第四个元素作为两个参数传给函数参数,依次类推。 如果传入了 initial 值, 那么首先传的就不是 sequence 的第一个和第二个元素,而是 initial值和 第一个元素。经过这样的累计计算之后合并序列到一个单一返回值
reduce 代码举例,使用REPL演示
>>> def add(x, y):
... return x+y
...
>>> from functools import reduce
>>> reduce(add, [1,2,3,4])
10
>>>
>>> sum([1,2,3,4])
10
>>>
很多教程只讲了一个加法求和,太简单了,对新手加深理解还不够。下面讲点更深入的例子
还可以把一个整数列表拼成整数,如下
>>> from functools import reduce
>>> reduce(lambda x, y: x * 10 + y, [1 , 2, 3, 4, 5])
12345
>>>
对一个复杂的sequence使用reduce ,看下面代码,更多的代码不再使用REPL, 使用编辑器编写
from functools import reduce
scientists =({'name':'Alan Turing', 'age':105},
{'name':'Dennis Ritchie', 'age':76},
{'name':'John von Neumann', 'age':114},
{'name':'Guido van Rossum', 'age':61})
def reducer(accumulator , value):
sum = accumulator['age'] + value['age']
return sum
total_age = reduce(reducer, scientists)
print(total_age)


from functools import reduce
scientists =({'name':'Alan Turing', 'age':105, 'gender':'male'},
{'name':'Dennis Ritchie', 'age':76, 'gender':'male'},
{'name':'Ada Lovelace', 'age':202, 'gender':'female'},
{'name':'Frances E. Allen', 'age':84, 'gender':'female'})
def reducer(accumulator , value):
sum = accumulator + value['age']
return sum
total_age = reduce(reducer, scientists, 0)
print(total_age)
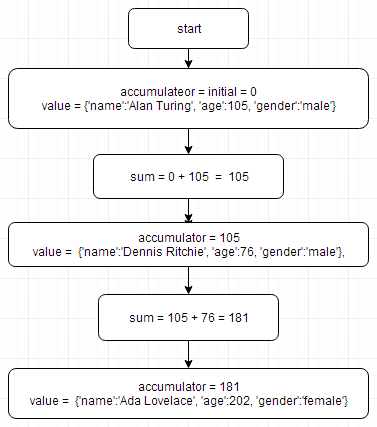
这个仍然也可以用 sum 来更简单的完成
sum([x['age'] for x in scientists ])
做点更高级的事情,按性别分组
from functools import reduce
scientists =({'name':'Alan Turing', 'age':105, 'gender':'male'},
{'name':'Dennis Ritchie', 'age':76, 'gender':'male'},
{'name':'Ada Lovelace', 'age':202, 'gender':'female'},
{'name':'Frances E. Allen', 'age':84, 'gender':'female'})
def group_by_gender(accumulator , value):
accumulator[value['gender']].append(value['name'])
return accumulator
grouped = reduce(group_by_gender, scientists, {'male':[], 'female':[]})
print(grouped)
输出
{'male': ['Alan Turing', 'Dennis Ritchie'], 'female': ['Ada Lovelace', 'Frances E. Allen']}
grouped = reduce(group_by_gender, scientists, collections.defaultdict(list))
当然 先要 import collections 模块
这当然也能用 pythonic way 去解决
import itertools
scientists =({'name':'Alan Turing', 'age':105, 'gender':'male'},
{'name':'Dennis Ritchie', 'age':76, 'gender':'male'},
{'name':'Ada Lovelace', 'age':202, 'gender':'female'},
{'name':'Frances E. Allen', 'age':84, 'gender':'female'})
grouped = {item[0]:list(item[1])
for item in itertools.groupby(scientists, lambda x: x['gender'])}
print(grouped)
再来一个更晦涩难懂的玩法。工作中要与其他人协作的话,不建议这么用,与上面的例子做同样的事,看不懂无所谓。
from functools import reduce
scientists =({'name':'Alan Turing', 'age':105, 'gender':'male'},
{'name':'Dennis Ritchie', 'age':76, 'gender':'male'},
{'name':'Ada Lovelace', 'age':202, 'gender':'female'},
{'name':'Frances E. Allen', 'age':84, 'gender':'female'})
grouped = reduce(lambda acc, val: {**acc, **{val['gender']: acc[val['gender']]+ [val['name']]}}, scientists, {'male':[], 'female':[]})
print(grouped)
**acc, **{val['gneder']... 这里使用了 dictionary merge syntax , 从 python 3.5 开始引入, 详情请看 PEP 448 - Additional Unpacking Generalizations 怎么使用可以参考这个python - How to merge two dictionaries in a single expression? - Stack Overflow
弄明白python reduce 函数的更多相关文章
- python reduce()函数
reduce()函数 reduce()函数也是Python内置的一个高阶函数.reduce()函数接收的参数和 map()类似,一个函数 f,一个list,但行为和 map()不同,reduce()传 ...
- py-day4-1 python reduce函数
from functools import reduse 从模块中导入 reduce函数: 处理一个序列,然后把序列进行合并操作 #**** 问题:求1+2+3+100的和是多少? # 一,原始 ...
- Python中的map()函数和reduce()函数的用法
Python中的map()函数和reduce()函数的用法 这篇文章主要介绍了Python中的map()函数和reduce()函数的用法,代码基于Python2.x版本,需要的朋友可以参考下 Py ...
- python Map()和reduce()函数
Map()和reduce()函数 map() 会根据提供的函数对指定序列做映射. 第一个参数 function 以参数序列中的每一个元素调用 function 函数,返回包含每次 function 函 ...
- Python的map、filter、reduce函数 [转]
1. map函数func作用于给定序列的每个元素,并用一个列表来提供返回值. map函数python实现代码: def map(func,seq): mapped_seq = [] fo ...
- python的reduce()函数
reduce()函数也是Python内置的一个高阶函数. reduce()函数接收的参数和 map()类似,一个函数 f,一个list,但行为和 map()不同,reduce()传入的函数 f 必须接 ...
- Python lambda和reduce函数
看到一篇博文写lambda和reduce函数.笔者小痒了一下,用Python实现一下: #! /usr/bin/env python # -*-coding:utf-8-*- import time ...
- Python自学笔记-map和reduce函数(来自廖雪峰的官网Python3)
感觉廖雪峰的官网http://www.liaoxuefeng.com/里面的教程不错,所以学习一下,把需要复习的摘抄一下. 以下内容主要为了自己复习用,详细内容请登录廖雪峰的官网查看. Python内 ...
- python 中 reduce 函数的使用
reduce()函数也是Python内置的一个高阶函数. reduce()函数接收的参数和 map()类似,一个函数 f,一个list,但行为和 map()不同,reduce()传入的函数 f 必须接 ...
随机推荐
- Tomcat 笔记-设置虚拟主机
通过作用虚拟主机,可以使多个不同域名的网站共存于一个Tomcat中 在tomcat的server.xml文件中添加主机名: <Host name="hostname" app ...
- IDEA + Maven + JavaWeb项目搭建
前言:在网上一直没找到一个完整的IDEA+Maven+Web项目搭建,对于IDEA和Maven初学者来说,这个过程简单但是非常痛苦的,对中间的某些步骤不是很理解,导致操作错误,从而项目发布不成功,一直 ...
- base64减少图片请求
1. 使用base64减少 a) 2. 页面解析 CSS 生成的 CSSOM 时间增加 Base64 跟 CSS 混在一起,大大增加了浏览器需要解析CSS树的耗时.其实解析CSS ...
- vue数据请求
我是vue菜鸟,第一次用vue做项目,写一些自己的理解,可能有些不正确,欢迎纠正. vue开发环境要配置本地代理服务.把config文件加下的index.js里的dev添加一些内容, dev: { e ...
- expected single matching bean but found 2
org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'acc ...
- Vue源码后记-其余内置指令(1)
把其余的内置指令也搞完吧,来一个全家桶. 案例如下: <body> <div id='app'> <div v-if="vIfIter" v-bind ...
- sqoop1.4.6从mysql导入hdfs\hive\hbase实例
//验证sqoop是否连接到mysql数据库sqoop list-tables --connect 'jdbc:mysql://n1/guizhou_test?useUnicode=true& ...
- Path.Combine 合并两个路径字符串,会出现的问题
Path.Combine(path1,path2) 1.如果path2字符串,以 \ 或 / 开头,则直接返回 path2
- Scala从入门到精通之四-映射和元组
在Scala中映射之键值对的集合,元组是n个对象的聚集,但是对象的类型不一定相同 本节内容要点 Scala中映射的创建,遍历和查询 如何从可变和不可变映射中做出选择 Scala映射和Java映射见的互 ...
- no suitable driver found for jdbc:mysql//localhost:3306/..
出现这样的情况,一般有四种原因(网上查的): 一:连接URL格式出现了问题(Connection conn=DriverManager.getConnection("jdbc:mysql ...