URAL 2034 : Caravans
Description
Input
Output
input | output |
---|---|
7 7 |
2 |
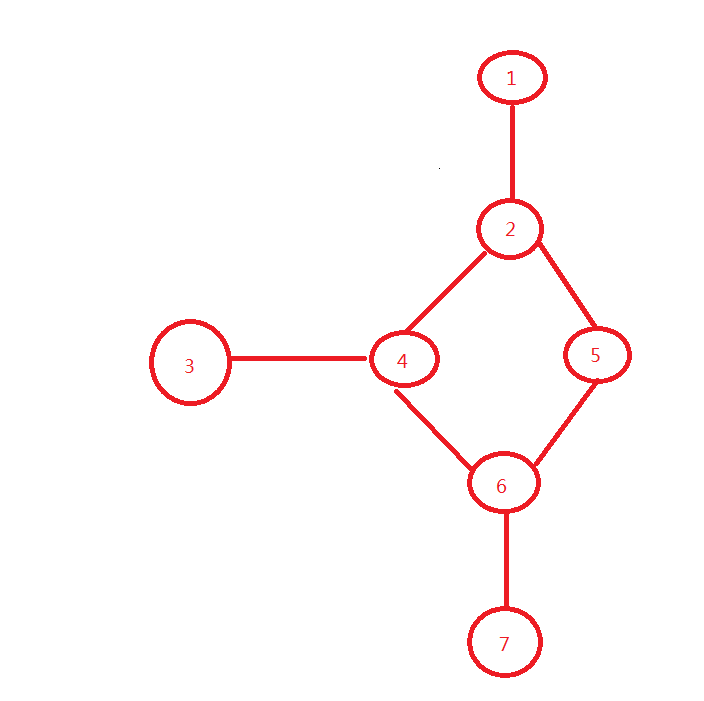
#include <cstdio>
#include <vector>
#include <queue>
#include <cstring>
using namespace std;
const int maxn = 1e5 + ;
#define max(x,y) ((x)>(y)?(x):(y))
#define min(x,y) ((x)<(y)?(x):(y))
vector<int>e[maxn];
int dp[maxn] , d[maxn] , maxv[maxn], n , m , s , f , r , used[maxn] ;
queue<int>q; void solve()
{
memset(dp , , sizeof(dp));memset(d,-,sizeof(d));memset(maxv,-,sizeof(maxv));memset(used,,sizeof(used));
d[f] = ;
q.push(f);
while(!q.empty())
{
int x = q.front();q.pop();
for(int i = ; i < e[x].size() ; ++ i)
{
int v = e[x][i];
if (d[v] == -)
{
d[v] = d[x] + ;
q.push(v);
}
}
}
maxv[r] = ;
q.push(r);
while(!q.empty())
{
int x = q.front();q.pop();
for(int i = ; i < e[x].size() ; ++ i)
{
int v = e[x][i];
if (maxv[v] == -)
{
maxv[v] = maxv[x] + ;
q.push(v);
}
}
}
dp[s] = maxv[s];used[s] = ;
q.push(s);
while(!q.empty())
{
int x = q.front();q.pop();
maxv[x] = min(maxv[x],dp[x]);
for(int i = ; i < e[x].size() ; ++ i)
{
int v = e[x][i];
if (d[x] - d[v] == )
{
dp[v] = max(dp[v],maxv[x]);
if (!used[v])
{
q.push(v);
used[v] = ;
}
}
}
}
printf("%d\n",maxv[f]);
} int main(int argc,char *argv[])
{
scanf("%d%d",&n,&m);
while(m--)
{
int u ,v ;
scanf("%d%d",&u,&v);u--,v--;
e[u].push_back(v);e[v].push_back(u);
}
scanf("%d%d%d",&s,&f,&r);s--,f--,r--;
solve();
return ;
}
URAL 2034 : Caravans的更多相关文章
- URAL 2034 Caravans(变态最短路)
Caravans Time limit: 1.0 secondMemory limit: 64 MB Student Ilya often skips his classes at the unive ...
- URAL
URAL 2035 输入x,y,c, 找到任意一对a,b 使得a+b==c&& 0<=a<=x && 0<=b<=y 注意后两个条件,顺序搞错 ...
- hdu 2034人见人爱A-B
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=2034 解题思路:set的基本用法 #include<iostream> #include& ...
- 后缀数组 POJ 3974 Palindrome && URAL 1297 Palindrome
题目链接 题意:求给定的字符串的最长回文子串 分析:做法是构造一个新的字符串是原字符串+反转后的原字符串(这样方便求两边回文的后缀的最长前缀),即newS = S + '$' + revS,枚举回文串 ...
- ural 2071. Juice Cocktails
2071. Juice Cocktails Time limit: 1.0 secondMemory limit: 64 MB Once n Denchiks come to the bar and ...
- ural 2073. Log Files
2073. Log Files Time limit: 1.0 secondMemory limit: 64 MB Nikolay has decided to become the best pro ...
- ural 2070. Interesting Numbers
2070. Interesting Numbers Time limit: 2.0 secondMemory limit: 64 MB Nikolay and Asya investigate int ...
- ural 2069. Hard Rock
2069. Hard Rock Time limit: 1.0 secondMemory limit: 64 MB Ilya is a frontman of the most famous rock ...
- ural 2068. Game of Nuts
2068. Game of Nuts Time limit: 1.0 secondMemory limit: 64 MB The war for Westeros is still in proces ...
随机推荐
- 简单使用NSURLConnection、NSURLRequest和NSURL
以下是代码,凝视也写得比較清楚: 头文件须要实现协议NSURLConnectionDelegate和NSURLConnectionDataDelegate // // HttpDemo.h // My ...
- Vitamio 多媒体框架 介绍
功能 Vitamio 是一款 Android 与 iOS 平台上的全能多媒体开发框架,全面支持硬件解码与 GPU 渲染.Vitamio 凭借其简洁易用的 API 接口赢得了全球众多开发者的青睐.到目前 ...
- nyoj 2
#include <iostream> #include <stack> #include <string.h> #include <stdio.h> ...
- C# 导出word文档及批量导出word文档(3)
在初始化WordHelper时,要获取模板的相对路径.获取文档的相对路径多个地方要用到,比如批量导出时要先保存文件到指定路径下,再压缩打包下载,所以专门写了个关于获取文档的相对路径的类. #regio ...
- oracle单行函数之类型转换
oracle数据类型转换:显示转换盒隐式转换 oracle自动完成转换
- 使用Scanner来解析文件
前面的流是全部流进来再处理,空间换取时间 我们用Scanner来解析文件,先处理再输入数据,时间换取空间 两种方法 Scanner scanner1=new Scanner(file1); for(; ...
- C/C++安全编码-字符串
1 字符串 1.1 字符串基础 字符串提供命令行参数.环境变量.控制台输入.文本文件及网络连 接,提供外部输入方法来影响程序的行为和输出,这也是程序容易出错的地方.字符串是一个概念,并不是C/ ...
- android JNI (二) 第一个 android工程
下载NDK 后 它自带有 sample,初学者 可以导入Eclipse 运行 这里 我是自己创建的一个新工程 第一步: 新建一个Android工程 jni_test(名字自取) 第二步:为工程添加 本 ...
- DEDE常见问题(转)
问题1. 把数据保存到数据库附加表 `dede_addonvisa` 时出错,请把相关信息提交给DedeCms官方.Unknown column 'redirecturl' in 'field lis ...
- php生成table表格
function getTable($arrTh, $arrTr){ $s = '<table class="tbData">'; $s .= '<tr>' ...